To list open ports in PowerShell, you can use the following command that retrieves active TCP connections along with the listening ports. Here's the code snippet:
Get-NetTCPConnection | Select-Object LocalPort, State | Where-Object { $_.State -eq 'Listen' }
Understanding Open Ports
What are Open Ports?
Open ports refer to the communication endpoints on a device that are actively listening for incoming connections. Each port is associated with a specific protocol, often TCP or UDP, which are used for different types of data transmission. The ports are identified by unique port numbers ranging from 0 to 65535, with certain numbers designated for specific services (e.g., HTTP uses port 80, HTTPS uses port 443).
Why List Open Ports?
Listing open ports is crucial for several reasons:
- Security Considerations: Knowing which ports are open allows network administrators to secure vulnerable services and prevent unauthorized access.
- Troubleshooting Network Issues: If an application is not functioning correctly, checking open ports can help identify whether a required service is running.
- Managing Services and Applications: By monitoring open ports, one can effectively manage and optimize resource allocation.

PowerShell Basics for Networking
Getting Started with PowerShell
PowerShell is a powerful command-line tool that allows system administrators to automate tasks and manage systems effectively. To open PowerShell in Windows, simply type "PowerShell" in the Start menu search bar and select the application.
Essential PowerShell Cmdlets for Network Management
Among the useful cmdlets for network management, `Get-NetTCPConnection` and `Get-NetUDPEndpoint` are key commands that help in listing active connections and endpoints in your system.
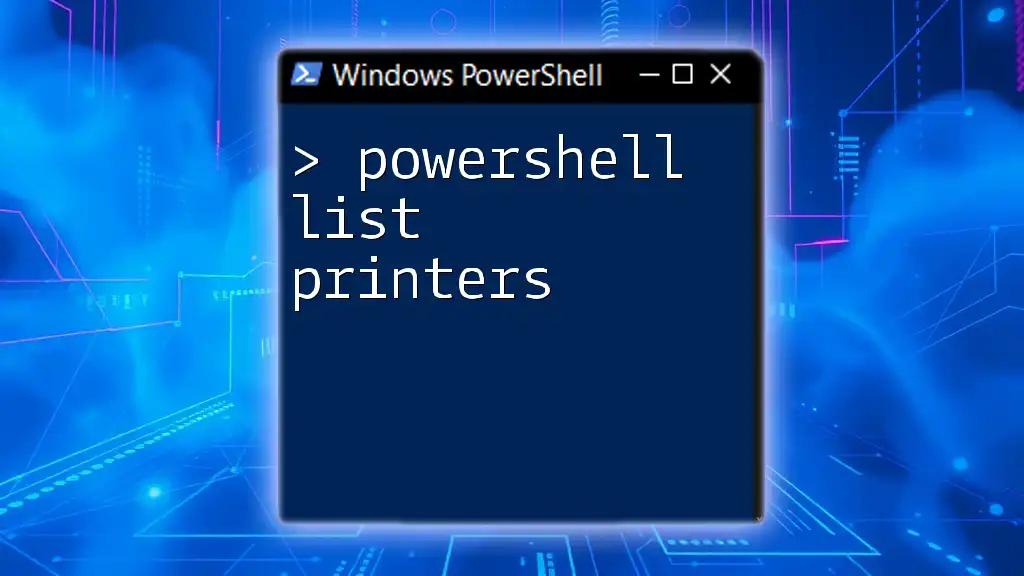
Listing Open Ports in PowerShell
Using `Get-NetTCPConnection`
The `Get-NetTCPConnection` cmdlet provides detailed information about TCP connections on a system. This command retrieves current TCP connections and displays useful details such as local port, state, and remote address.
Code Snippet:
Get-NetTCPConnection | Select-Object LocalPort, State, RemoteAddress
This command selects specific properties—`LocalPort`, `State`, and `RemoteAddress`—to create a simplified view of the current TCP connections. Understanding the output helps identify which ports are being used and their respective states.
Using `Get-NetUDPEndpoint`
To list open UDP ports, you can use the `Get-NetUDPEndpoint` cmdlet. Unlike TCP, UDP is connectionless and is often used for services that require faster data transmission.
Code Snippet:
Get-NetUDPEndpoint | Select-Object LocalPort, LocalAddress
Using this command allows system administrators to monitor UDP traffic, which is vital when analyzing services like DNS and streaming applications.
Combining TCP and UDP Output
Using `ConvertFrom-Json` to Combine Outputs
For a more comprehensive overview, you can combine TCP and UDP outputs to gain insights into all listening ports.
Code Snippet:
$tcp = Get-NetTCPConnection | Select-Object LocalPort, State, RemoteAddress
$udp = Get-NetUDPEndpoint | Select-Object LocalPort, LocalAddress
$result = @($tcp, $udp)
$result
This script merges the outputs from both cmdlets into a single array, allowing for a unified view of both TCP and UDP active connections.
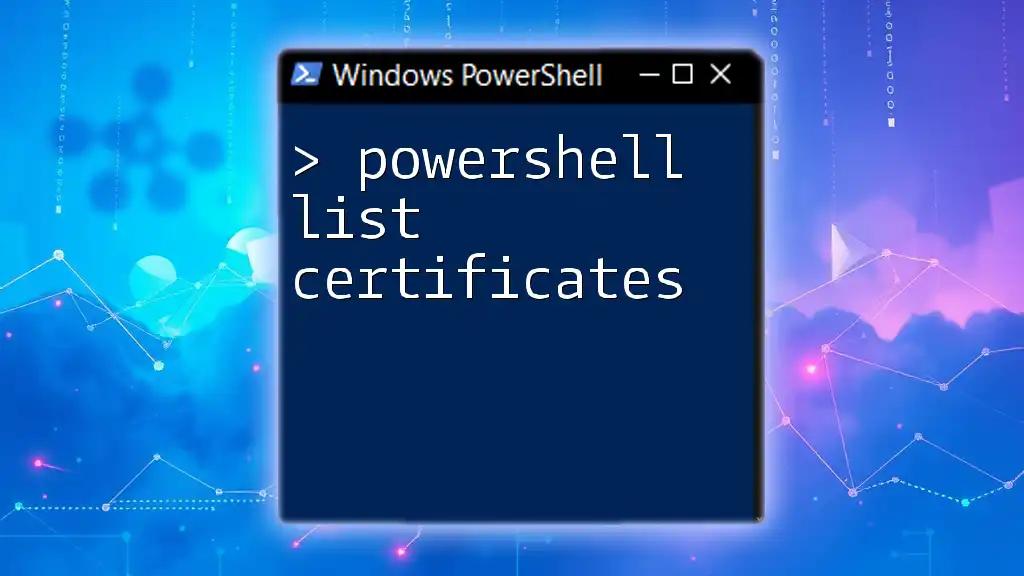
Filtering Open Ports
Filtering by Local Port
If you want to focus on a specific port, you can filter the results using the `Where-Object` cmdlet. This is useful for tracking services running on particular ports.
Code Snippet:
Get-NetTCPConnection | Where-Object { $_.LocalPort -eq 80 }
This command will return any TCP connections using port 80, which is commonly used for web services.
Filtering by State
To filter by the state of the ports (such as 'Listen'), you can use a similar approach:
Code Snippet:
Get-NetTCPConnection | Where-Object { $_.State -eq 'Listen' }
This command identifies all ports actively listening for incoming connections, providing crucial data for security assessments.
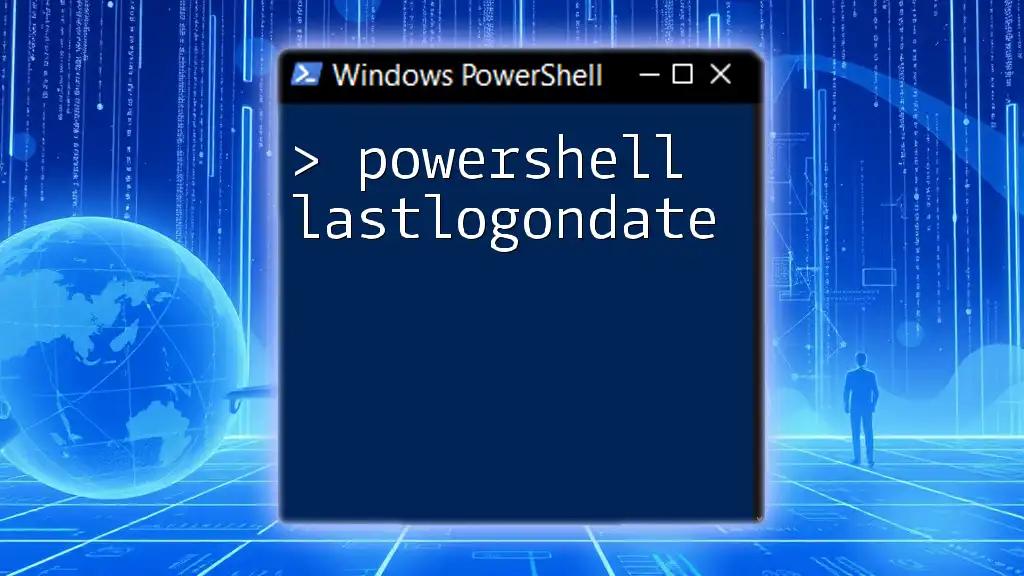
Exporting Open Ports to a File
Saving the Output
Documenting the list of open ports can enhance security management efficiency. Exporting the output of port listings can be achieved easily with the `Export-Csv` cmdlet.
Code Snippet:
Get-NetTCPConnection | Export-Csv -Path C:\open_ports.csv -NoTypeInformation
This command saves the current list of TCP connections to a CSV file, allowing for easy sharing and record-keeping.
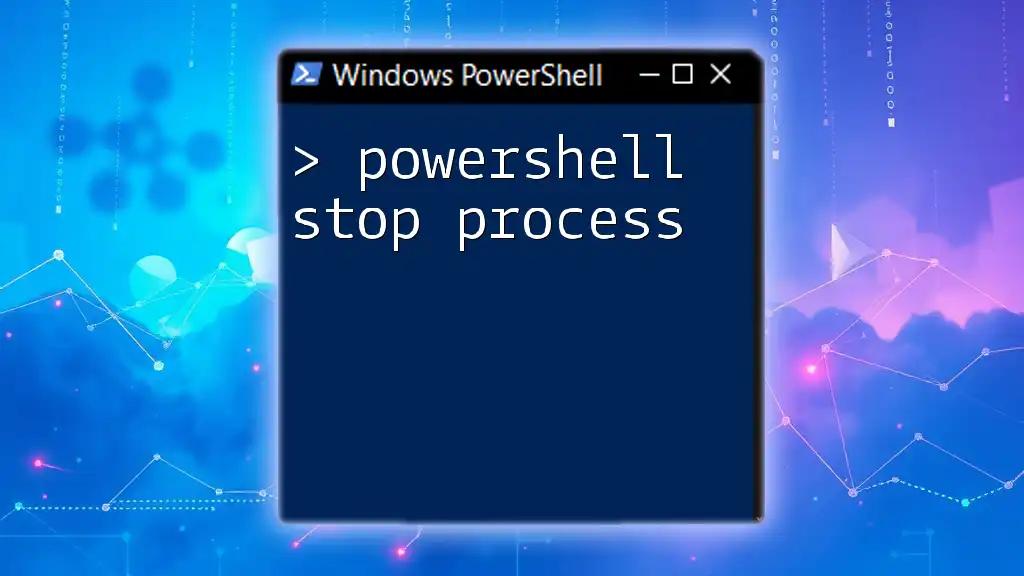
Monitoring Open Ports
Creating a Script for Regular Checks
To maintain ongoing visibility into open ports, you might consider creating a script that captures this information regularly.
Code Snippet:
$monitoringScript = {
Get-NetTCPConnection | Out-File C:\port_monitor_log.txt -Append
}
Utilizing this block within a scheduled task allows you to automate logging TCP connections, which can be essential for identifying changes over time.

Common Issues and Troubleshooting
Potential Errors in Port Listing
When attempting to list open ports, a few common issues may arise. Firewall restrictions could block PowerShell from accessing certain information. Additionally, lacking permission to execute certain commands may impede users who are not running PowerShell as an administrator.
When to Seek Further Help
If the built-in cmdlets don't suffice for your needs, consider exploring third-party tools like Nmap for more extensive scanning capabilities. Online communities and forums are also invaluable for troubleshooting specific issues.

Conclusion
Knowing how to powershell list open ports is an essential skill for managing network security and troubleshooting in any IT environment. By utilizing PowerShell commands effectively, you can enhance your system's security and performance routines, allowing for greater visibility into network activity.
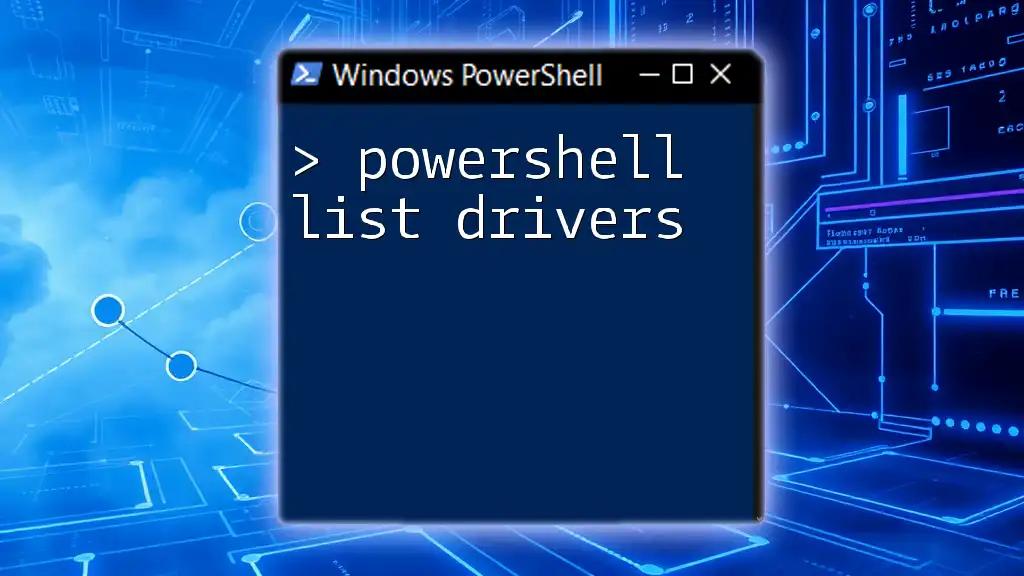
Additional Resources
Recommended Reading and Tutorials
For those looking to deepen their understanding of PowerShell, the official Microsoft PowerShell documentation offers extensive resources. Additionally, consider enrolling in online courses that focus on PowerShell networking for hands-on learning experiences.

Call to Action
Explore our PowerShell courses to further enhance your skills! Become proficient in managing and securing your networks with quick and effective command-line techniques.