The `StartsWith` method in PowerShell is used to determine if a string begins with a specified substring, allowing for efficient filtering and checking of string data.
Here’s an example code snippet demonstrating its usage:
# Check if the string starts with "Hello"
$string = "Hello, World!"
if ($string.StartsWith("Hello")) {
Write-Host 'The string starts with "Hello".'
} else {
Write-Host 'The string does not start with "Hello".'
}
Understanding PowerShell String Manipulation
What is String Manipulation?
String manipulation refers to the ability to change, parse, and analyze strings in programming languages. It is a critical skill that enables developers to handle text data efficiently. In PowerShell, string manipulation plays a pivotal role in tasks such as filtering data, validating input, and automating repetitive operations.
Why Use PowerShell for String Manipulation?
PowerShell is a powerful scripting language built on the .NET framework, specifically designed for system administration and automation. It provides a rich set of features that simplify string manipulation tasks, allowing users to write concise scripts quickly. The ability to manipulate strings effectively means less code and faster results.
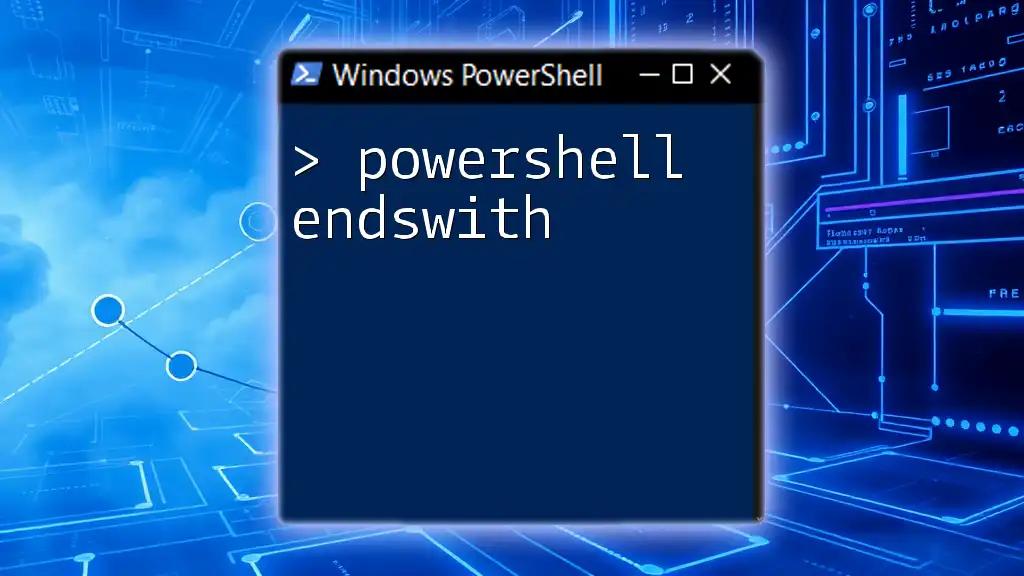
PowerShell StartsWith: An Overview
What is `StartsWith` in PowerShell?
The `StartsWith` method in PowerShell is a function used to determine if a particular string starts with a specified substring. This method is essential for scenarios where you want to validate or conditionally handle data based on its initial characters, such as validating filenames, URLs, or commands.
Syntax of `StartsWith` in PowerShell
The basic syntax of the `StartsWith` method is as follows:
$string.StartsWith($substring)
- $string: The string you want to check.
- $substring: The substring you want to compare with the start of the string.
The method returns `True` if the string starts with the specified substring and `False` otherwise.
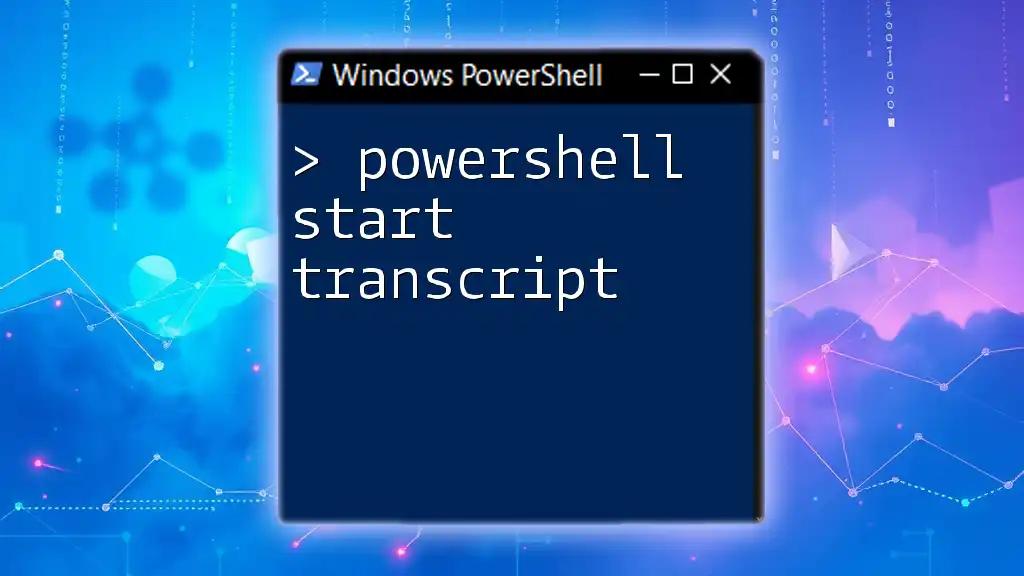
Using `StartsWith` with String Objects
Basic Examples of `StartsWith`
Let's take a look at a simple example:
$greeting = "Hello, World!"
$result = $greeting.StartsWith("Hello")
In this case, the variable `$result` will contain `True` because the string stored in `$greeting` indeed starts with "Hello".
Case Sensitivity in `StartsWith`
One crucial aspect to keep in mind is that `StartsWith` is case-sensitive by default. This means that "Hello" and "hello" are treated as different strings.
$greeting.StartsWith("hello") # returns $false
If you need to perform a case-insensitive check, you can use the `StringComparison` enumeration:
$result = [string]::StartsWith($greeting, "hello", [StringComparison]::CurrentCultureIgnoreCase) # returns $true
This method allows you to specify how you want PowerShell to compare the strings, making it a robust solution for various scenarios.
Combining `StartsWith` with Conditional Logic
Using `If` Statements with `StartsWith`
One common use case is to include `StartsWith` in conditional statements to make decisions based on string content. For example:
if ($greeting.StartsWith("Hello")) {
Write-Output "Greeting starts with Hello."
}
In this snippet, if `$greeting` starts with "Hello", it outputs the message confirming that condition.
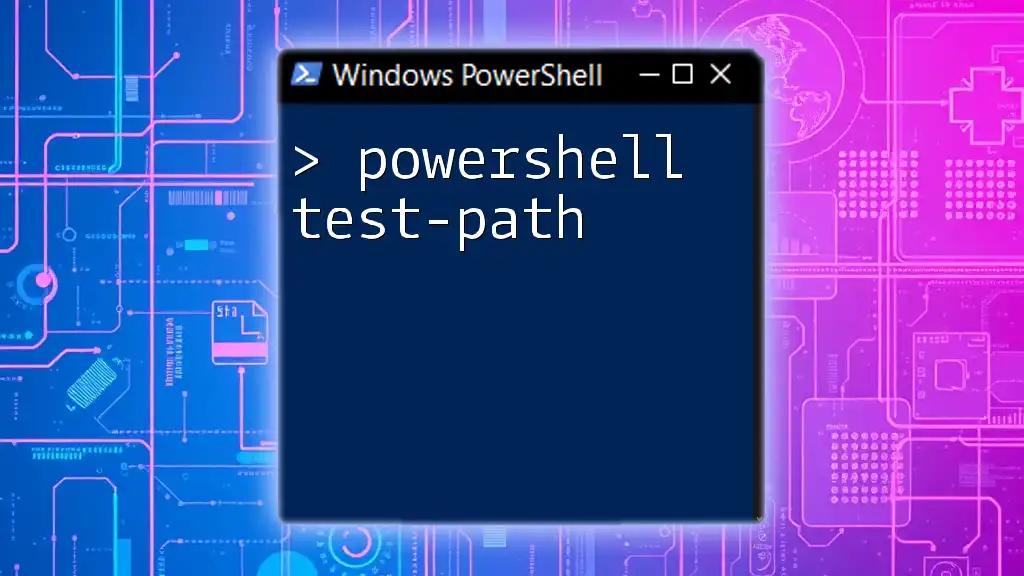
Advanced Usage of `StartsWith`
Working with Arrays of Strings
In scenarios where you need to check multiple strings, `StartsWith` can be effectively used within loops. For example:
$words = @("Apple", "Banana", "Cherry")
$prefix = "B"
foreach ($word in $words) {
if ($word.StartsWith($prefix)) {
Write-Output "$word starts with $prefix."
}
}
This script checks each word in the array and outputs those that start with the prefix "B," showcasing how `StartsWith` allows batch processing of strings.
String Comparison with Different Cultures
When localizing applications or scripts, culture-specific string comparisons can be vital. Using culture-aware string manipulation ensures that your scripts behave correctly across different languages.
$stringInfo = New-Object System.Globalization.CultureInfo('en-US')
$result = [string]::StartsWith("café", "caf", [StringComparison]::CurrentCultureIgnoreCase)
In this example, using `CurrentCultureIgnoreCase` ensures that "café" is evaluated correctly, accounting for locale-specific characters.
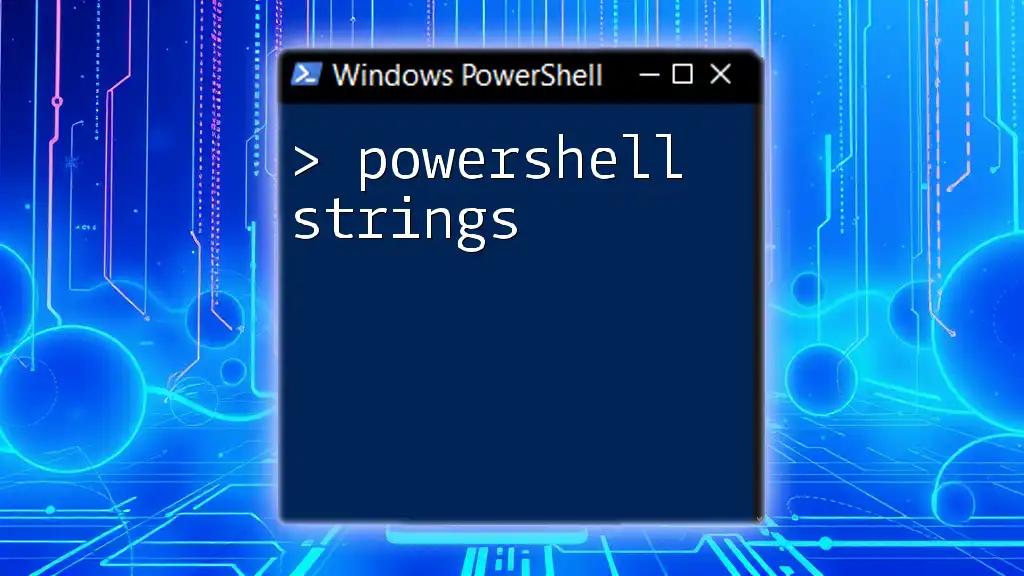
Performance Considerations
When to Use `StartsWith`
Using `StartsWith` is particularly advantageous when you need to perform quick checks at the beginning of strings. It excels in scenarios where the position of your substring is critical, such as filtering logs or validating inputs before processing them further.
Alternatives to `StartsWith`
While `StartsWith` is powerful, there are alternative string methods you might consider, such as `Contains` or `IndexOf`. These methods, however, check for substrings without focusing solely on the starting position.
$example1 = "Hello World"
$example1.Contains("Hello") # returns $true
$example1.IndexOf("Hello") -eq 0 # returns $true
This comparison shows that while `Contains` checks for the presence of "Hello" anywhere in the string, `IndexOf` checks whether it starts at position zero, thereby achieving the same intent within a broader scope.
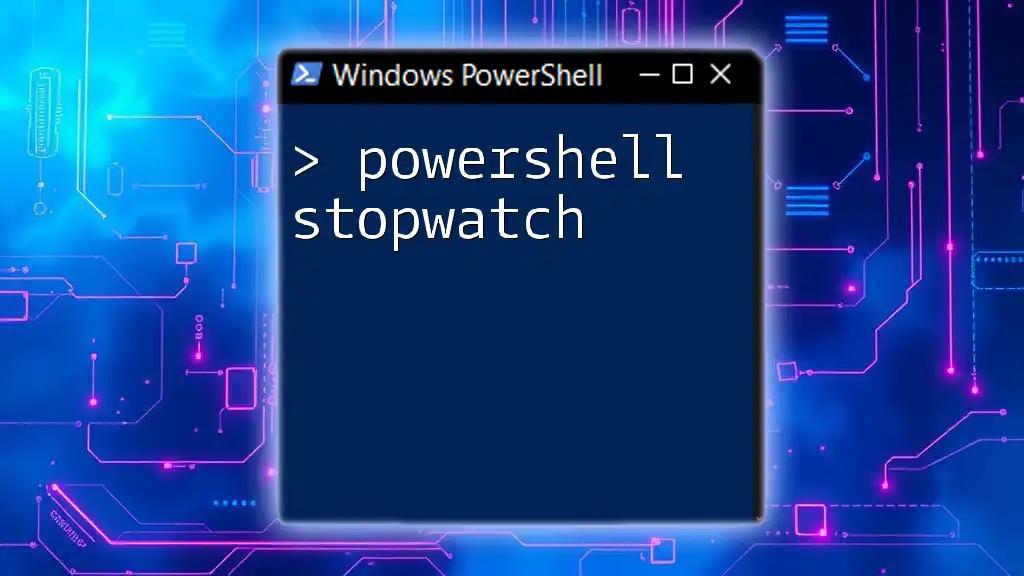
Troubleshooting Common Issues
Common Errors with `StartsWith`
Common mistakes when using `StartsWith` include incorrect casing or typographical errors in the substring. Always ensure your input is accurate, and consider the case sensitivity of the method.
Best Practices for Using `StartsWith`
To optimize the use of `StartsWith`:
- Double-check the string casing before performing checks.
- Use `StringComparison` to avoid pitfalls with case sensitivity.
- Group inputs logically to simplify checks and improve readability in your scripts.
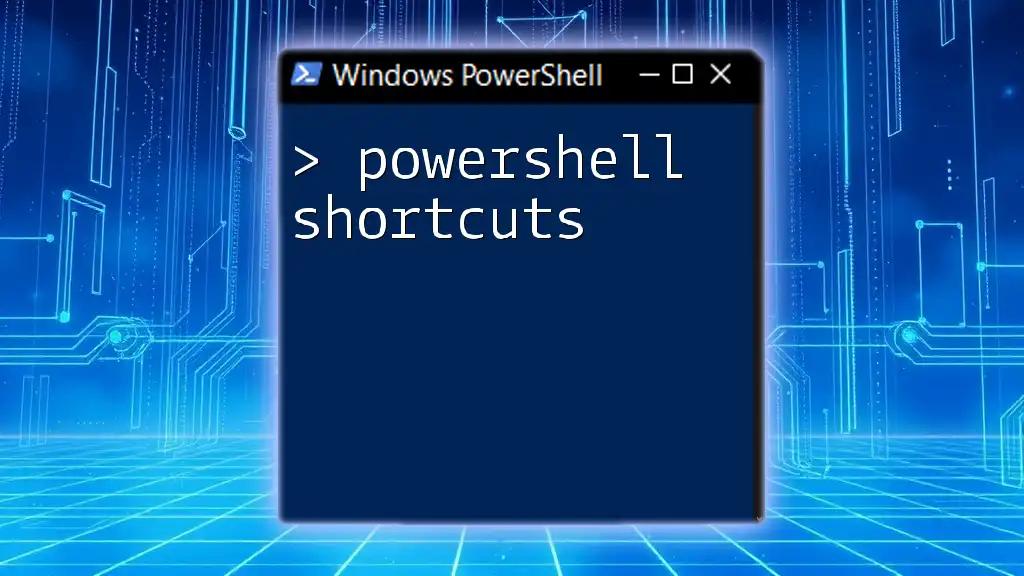
Conclusion
Recap of Key Takeaways
The `StartsWith` method is a vital tool in PowerShell for string manipulation, enabling users to efficiently validate and handle strings based on their initial characters. By understanding its features, such as case sensitivity and culture considerations, you can implement powerful scripts that streamline your workflows.
Encouragement to Experiment
Now that you have an understanding of how to use `StartsWith` effectively, try incorporating this method into your own scripts! Explore other string manipulation features in PowerShell to become even more proficient in automating your tasks.