The `EndsWith` method in PowerShell is used to determine whether a given string ends with a specified substring, enabling easy string manipulation and validation.
Here's a quick code snippet demonstrating its usage:
$string = "Learn PowerShell"
$endsWith = $string.EndsWith("PowerShell")
Write-Host $endsWith # Outputs: True
Overview of String Methods in PowerShell
PowerShell offers a range of string manipulation methods that allow users to handle text efficiently. Among these, the EndsWith method stands out as a vital tool for checking whether a string concludes with a specific substring. This capability is crucial in various scripts, especially when validating file types or formats.
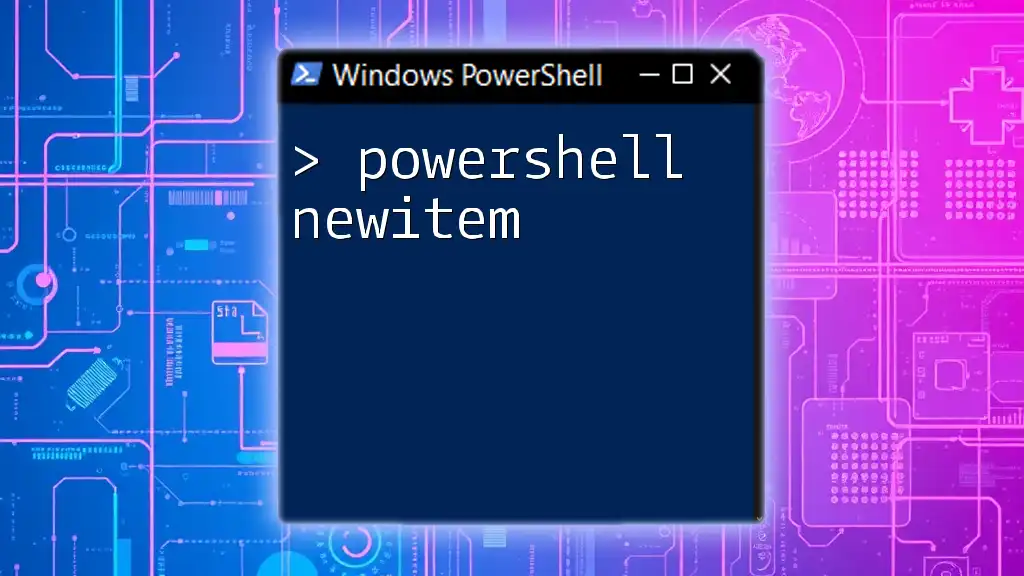
Understanding the EndsWith Method
Definition of EndsWith
The EndsWith method is designed to determine if a given string ends with a particular sequence of characters, referred to as a substring. This method is particularly valuable for verifying file extensions, handling data input validation, and more.
Syntax of the EndsWith Method
The structure of the EndsWith command is straightforward. It can be executed as follows:
$string.EndsWith($value)
In this command:
- $string represents the string you want to check.
- $value is the substring you are checking for at the end of the string.
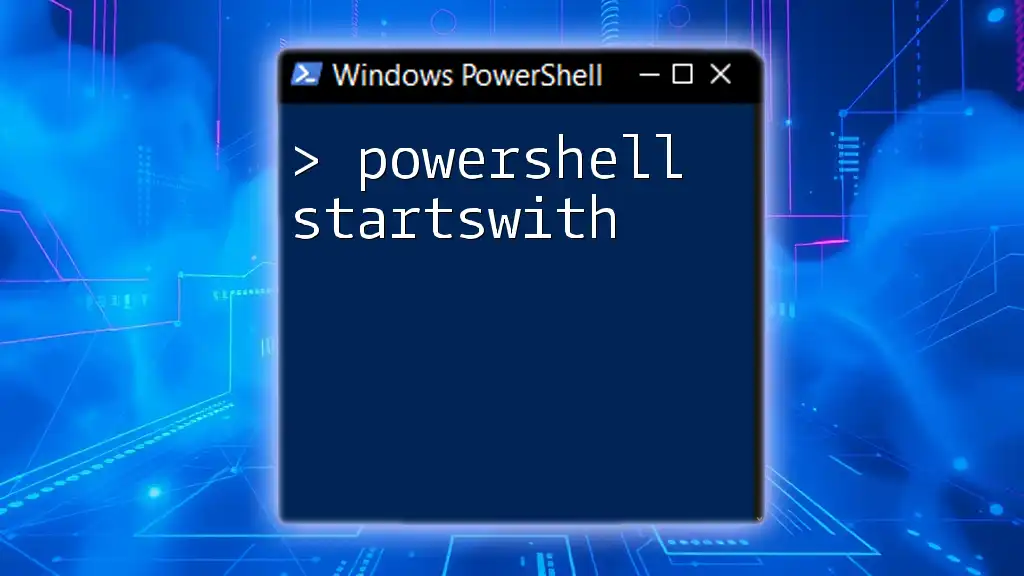
Parameters of the EndsWith Method
Value Parameter
The $value parameter is essential as it defines the substring you're searching for. If this substring appears at the end of your string, the EndsWith method will return True. If not, it will return False.
Comparison Type Parameter
There's also an optional parameter that allows you to specify whether the comparison should be case-sensitive or not. This can be an important feature when distinguishing file types that are case-sensitive.
The full syntax, including the comparison parameter, looks like this:
$string.EndsWith($value, $ignoreCase)
Where:
- $ignoreCase is a Boolean option; if set to True, the comparison will ignore case.
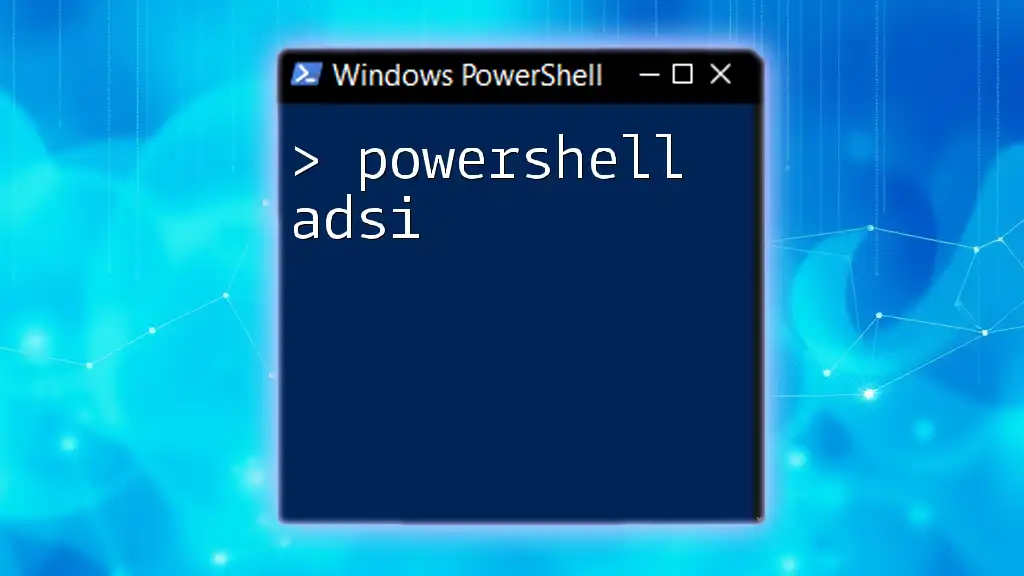
Practical Examples of Using EndsWith
Basic Example
To demonstrate the simplicity and effectiveness of the EndsWith method, consider the following example. Suppose you want to check if a filename indicates a Word document:
$filename = "report.docx"
if ($filename.EndsWith(".docx")) {
"This file is a Word document."
}
In this code snippet, the message will be displayed if the $filename indeed ends with ".docx".
Using EndsWith with Different Comparisons
Another scenario involves case sensitivity. Here’s how you can check a filename while disregarding case:
$filename = "IMAGE.PNG"
if ($filename.EndsWith(".png", $true)) {
"This file is a PNG image."
} else {
"This file is not a PNG image."
}
In this case, even if the input has uppercase letters, the code will accurately identify it as a PNG image due to the $ignoreCase parameter set to True.
Working with Collections of Strings
You can also use the EndsWith method to process multiple strings in a collection. Here’s an example that checks a list of file names for a specific extension:
$files = @("photo.jpg", "document.pdf", "spreadsheet.csv")
foreach ($file in $files) {
if ($file.EndsWith(".pdf")) {
"Found a PDF file: $file"
}
}
This loop iterates through each file in the $files array, checking for a .pdf extension and printing a message for each match.
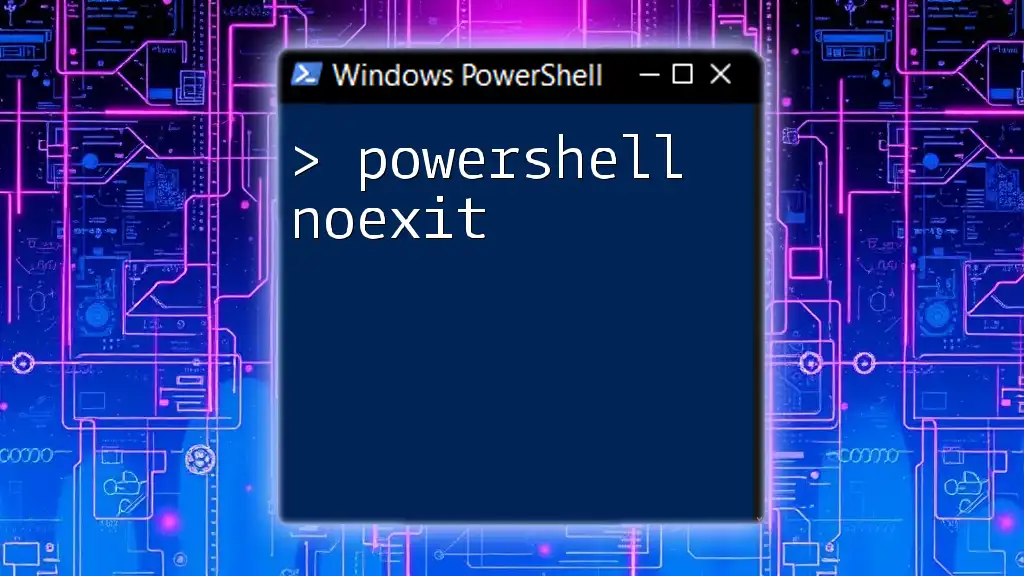
Use Cases of EndsWith in Real-World Scenarios
File Processing
A common application of the EndsWith method is in file processing tasks, especially when filtering files by their extensions. When automating workflows or scripts that involve file management, you can easily verify that files conform to expected types.
Data Validation
The EndsWith method proves particularly helpful in validating specific formats for inputs in scripts. For instance, ensuring that user input matches the expected format can prevent errors and enhance the overall robustness of your code.
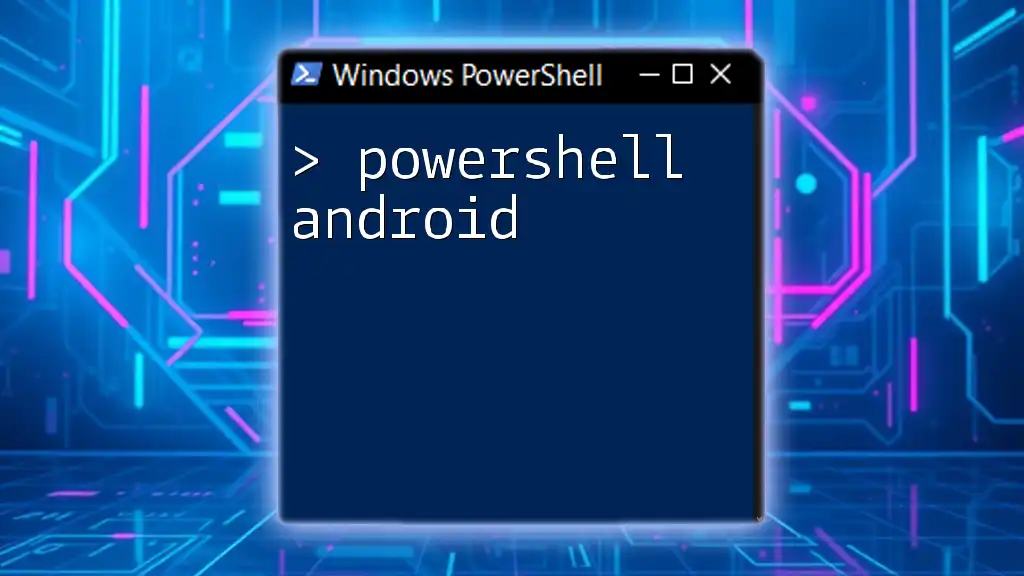
Best Practices When Using EndsWith
Avoiding Common Pitfalls
While using the EndsWith method, it’s easy to mistakenly assume that it performs a case-insensitive check unless specified. Therefore, always clarify the comparison type to avoid unexpected results.
Performance Considerations
In most cases, the performance of the EndsWith method is efficient. However, if you are working with a very large number of strings or complex conditions, consider whether it might be beneficial to explore regular expressions or other methods for validation.
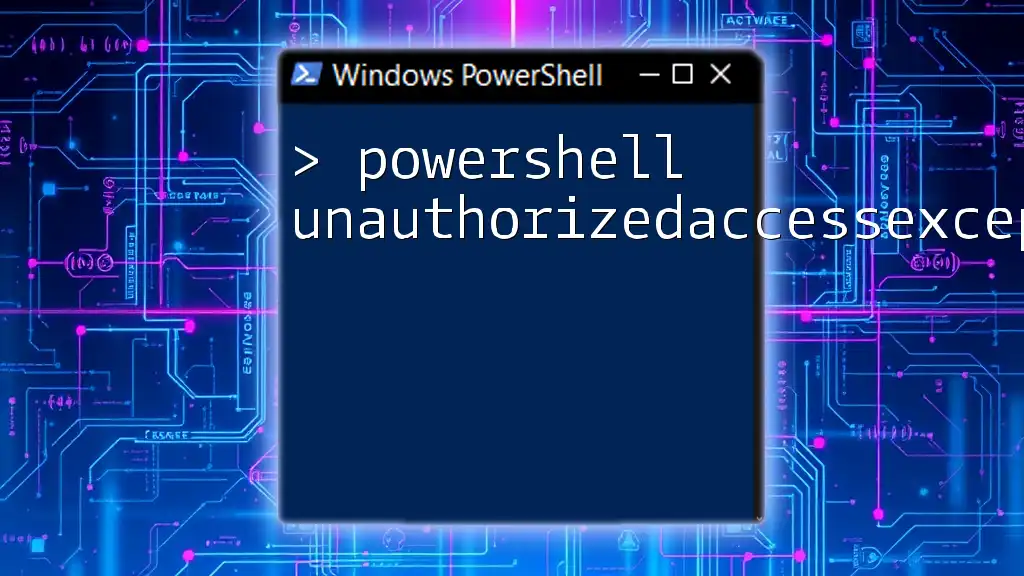
Conclusion
In summary, the PowerShell EndsWith method is an essential string manipulation tool that empowers users to validate, filter, and process strings effectively. Its straightforward syntax and flexible parameters make it a useful addition to any PowerShell script. Users are encouraged to practice using EndsWith in different contexts to fully leverage its capabilities, and consider exploring other string methods offered by PowerShell for even deeper functionality.