The `Instr` function in PowerShell is used to determine the position of a substring within a string, returning the index of its first occurrence or zero if it isn't found.
Here’s a simple code snippet demonstrating its use:
$string = "Hello, PowerShell World!"
$substring = "PowerShell"
$position = $string.IndexOf($substring) + 1 # Add 1 for 1-based index
Write-Host "The substring '$substring' is found at position: $position"
What is the `-in` Operator?
The `-in` operator is a powerful tool in PowerShell that checks for membership within collections. In other words, it evaluates whether a specific value exists within an array or a collection of items. This operator is particularly useful for making decisions in scripts, allowing for concise conditional checks in your code.

Understanding the Basics of the `-in` Operator
What Does `-in` Do?
Simply put, the `-in` operator performs a membership test. It returns true if the value on the left side exists in the collection on the right side.
Syntax of the `-in` Operator
The syntax for using the `-in` operator is straightforward:
Value -in Collection
In this structure:
- Value is what you're searching for.
- Collection is the array, list, or a set of items you're checking against.
When to Use `-in`
You would typically use the `-in` operator when:
- You need to verify membership of a value in a collection.
- You are filtering data or making decisions based on whether a certain condition holds.
The `-in` operator can greatly simplify your scripts, making them more readable and efficient.
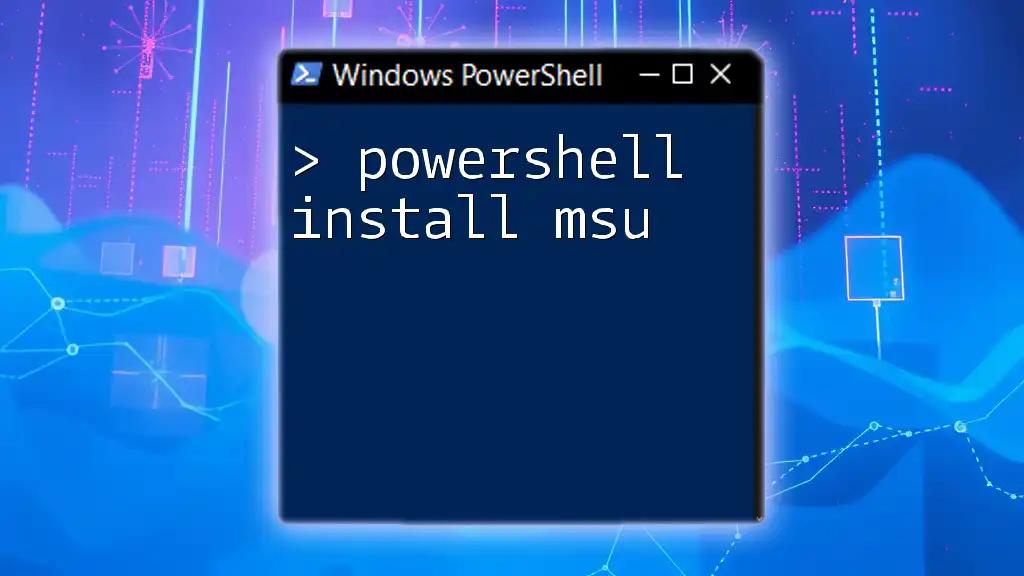
Practical Examples of Using the `-in` Operator
Example 1: Simple Value Check
Consider a scenario where you have a collection of fruits:
$fruits = 'apple', 'banana', 'cherry'
'banana' -in $fruits
In this example, the output will be true as `'banana'` exists within the `$fruits` array. This simple check can be used in conditional statements or scripts to streamline functionality.
Example 2: If Statement with `-in`
The `-in` operator shines in conditional statements. Here's an example involving user access:
$allowedUsers = 'alice', 'bob', 'charlie'
$currentUser = 'bob'
if ($currentUser -in $allowedUsers) {
Write-Host "Access granted."
} else {
Write-Host "Access denied."
}
In this code snippet, if `$currentUser` is found within `$allowedUsers`, the script outputs "Access granted." Otherwise, it will say "Access denied." This demonstrates how you can easily manage access controls using the `-in` operator.
Example 3: Using `-in` with Arrays
PowerShell also allows you to use the `-in` operator with arrays directly:
$colors = @('red', 'green', 'blue')
$inputColor = 'green'
if ($inputColor -in $colors) {
"The color is in the array."
} else {
"The color is not in the array."
}
In this example, the output confirms that the input color `'green'` is indeed in the `$colors` array. Using the `-in` operator in this way streamlines code and enhances clarity.
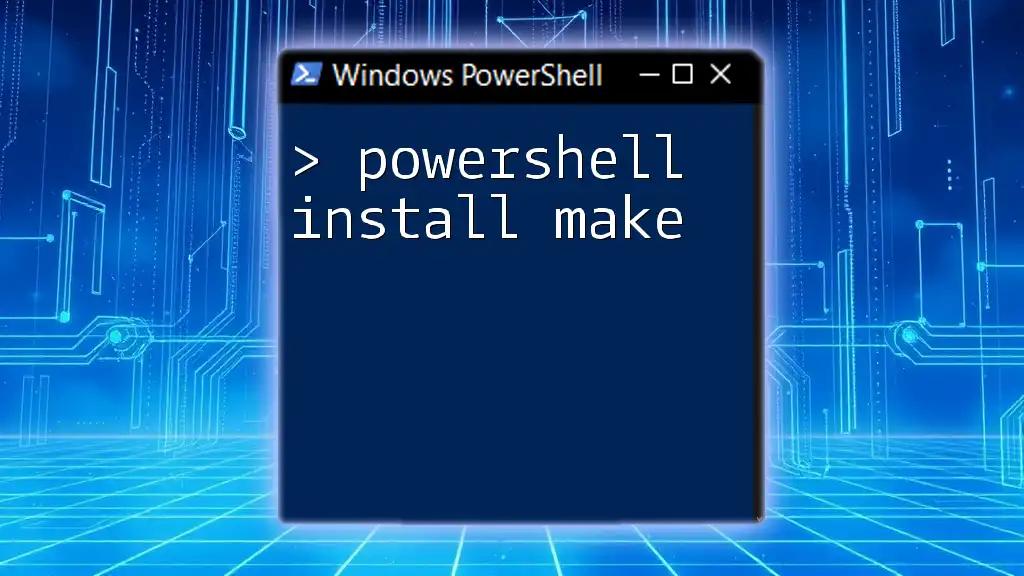
Advanced Use Cases for the `-in` Operator
Working with Hash Tables
The `-in` operator can also be applied within hash tables. For instance:
$userPermissions = @{
'alice' = 'admin'
'bob' = 'user'
'charlie' = 'guest'
}
if ('bob' -in $userPermissions.Keys) {
"Bob has permissions."
}
In this case, the script checks if `'bob'` is one of the keys in the `$userPermissions` hash table. This is useful when you want to validate user roles or permissions.
Using `-in` in Pipelines
You can use the `-in` operator with pipelines as well, enhancing its utility in data manipulation:
Get-Process | Where-Object { $_.Name -in @('explorer', 'notepad') }
Here, the script retrieves running processes and filters them based on whether their names match either `'explorer'` or `'notepad'`. This use case illustrates how `-in` can make filtering operations efficient and expressive.
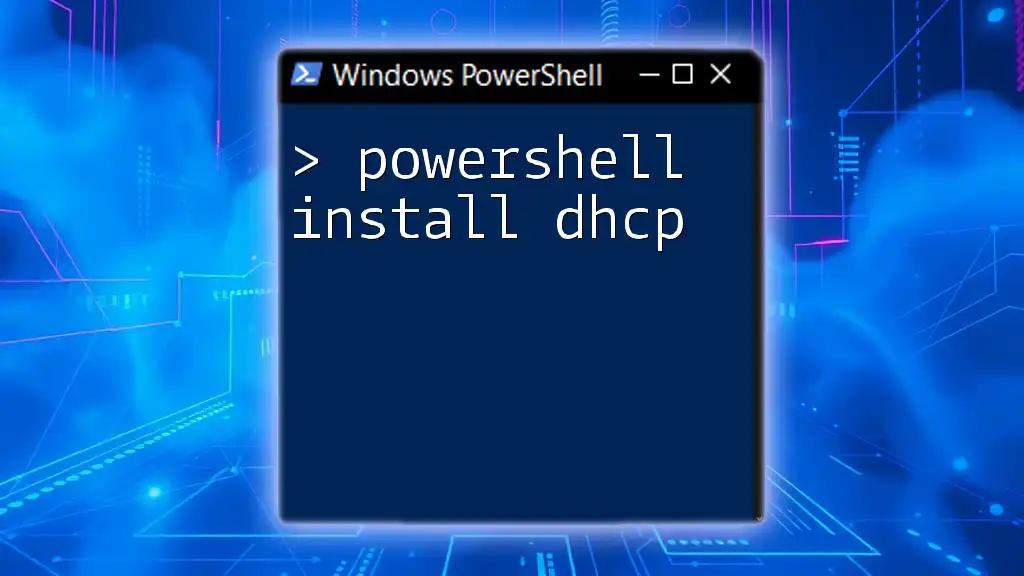
Troubleshooting Common Issues
Common Errors with `-in`
While the `-in` operator is intuitive, mistakes can occur. A common error includes checking against non-collection types. For instance, attempting to check if `'apple' -in 'fruit'` will yield an error since a string is not a collection.
Debugging Tips
For troubleshooting, consider using `Write-Host` to print intermediate values:
# Debugging example
$currentUser = 'bob'
$allowedUsers = 'alice', 'bob', 'charlie'
Write-Host "Checking user: $currentUser"
if ($currentUser -in $allowedUsers) {
Write-Host "User is allowed."
} else {
Write-Host "User is not allowed."
}
This practice can clarify data flow and help understand where an error might have occurred.
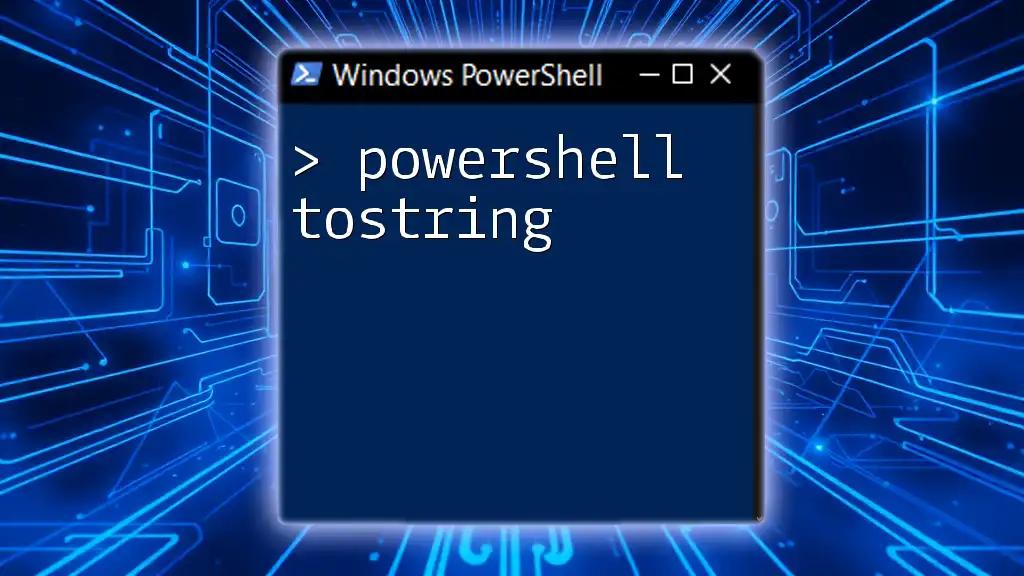
Performance Considerations
Efficiency of Using `-in`
When using the `-in` operator, it's crucial to grasp the performance implications. For small collections, it works efficiently. However, as the size of the collection grows, you may want to evaluate alternatives like hashing collections that can improve lookup times.
Best Practices
For optimal performance when using `-in`:
- Always ensure the collection is strictly necessary, avoiding large, unfiltered lists.
- Use hash tables when feasible, as they provide faster lookups compared to arrays.
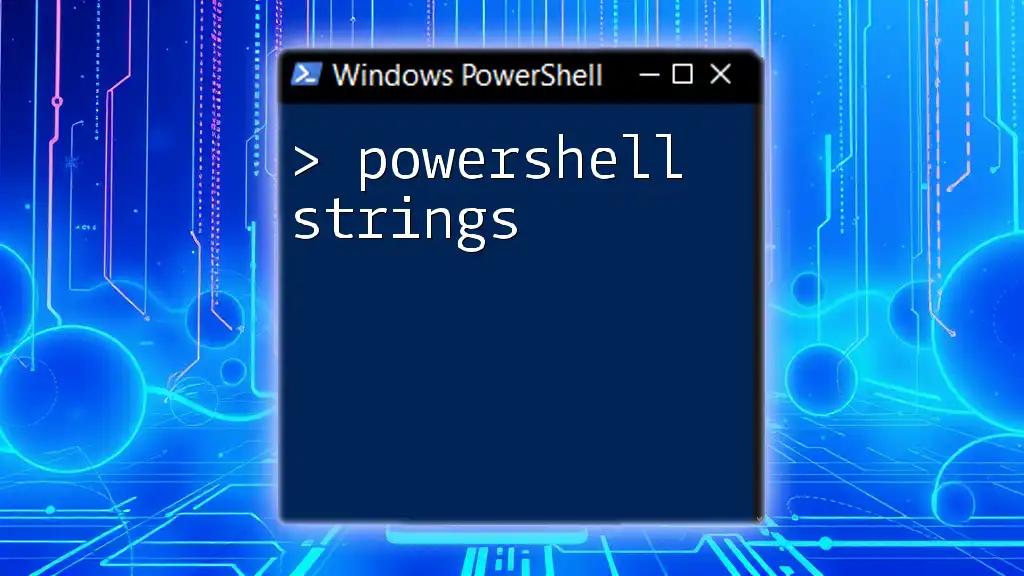
Conclusion
By leveraging the `-in` operator in PowerShell, you can perform tailored membership tests that enhance the logic within your scripts. It not only streamlines code but also allows for clear readability and efficient data handling.
Encouragement to Practice
We encourage you to experiment with the `-in` operator in your own scripts. The more you practice its use, the more adept you will become at handling complex conditions and enhancing your PowerShell proficiency.
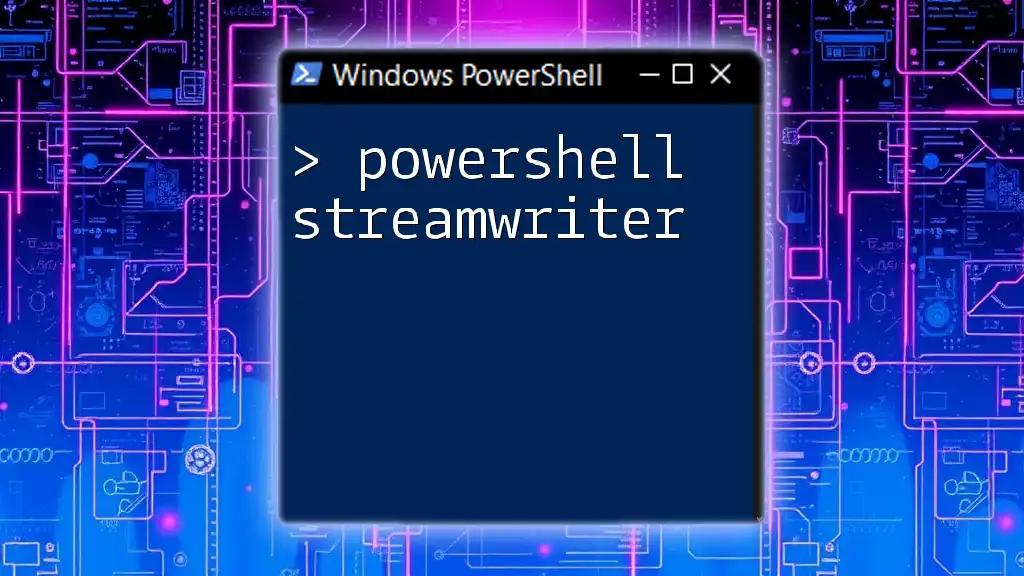
Additional Resources
For those looking to expand their knowledge further, consider exploring Microsoft’s official PowerShell documentation, community blogs, and forums dedicated to scripting and automation challenges. Engaging with these resources will deepen your understanding and help you stay updated on the latest tips and best practices in PowerShell scripting.