"Powershell in string refers to the ability to embed and manipulate strings in PowerShell scripts, allowing for dynamic data handling and output."
Here’s a code snippet demonstrating the use of strings in PowerShell:
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding Strings in PowerShell
What is a String?
A string in programming is a sequence of characters used to represent text. In PowerShell, strings are a fundamental data type and are crucial for managing textual data in scripts. Understanding how strings work is essential for effective PowerShell scripting.
String Types in PowerShell
PowerShell supports various types of strings, each serving distinct purposes for string manipulation.
Single-Quoted Strings
Single-quoted strings treat everything within the quotes as literal text. They do not allow for variable expansion or escape sequences. For example:
'$hello world'
In this case, `$hello world` is treated as plain text.
Double-Quoted Strings
Double-quoted strings allow for variable expansion and interpret escape sequences. This makes them highly versatile for generating dynamic texts. For example:
$name = "World"
"$hello $name"
This will output `Hello World`. The variable `$name` is expanded within the string.
Here-Strings
Here-strings are a convenient way to define multiline strings. They can make your code more readable, especially when dealing with long texts. A here-string is defined using `@"` to start and `"@` to end, like so:
$multiline = @"
This is a here-string.
It can span multiple lines.
"@
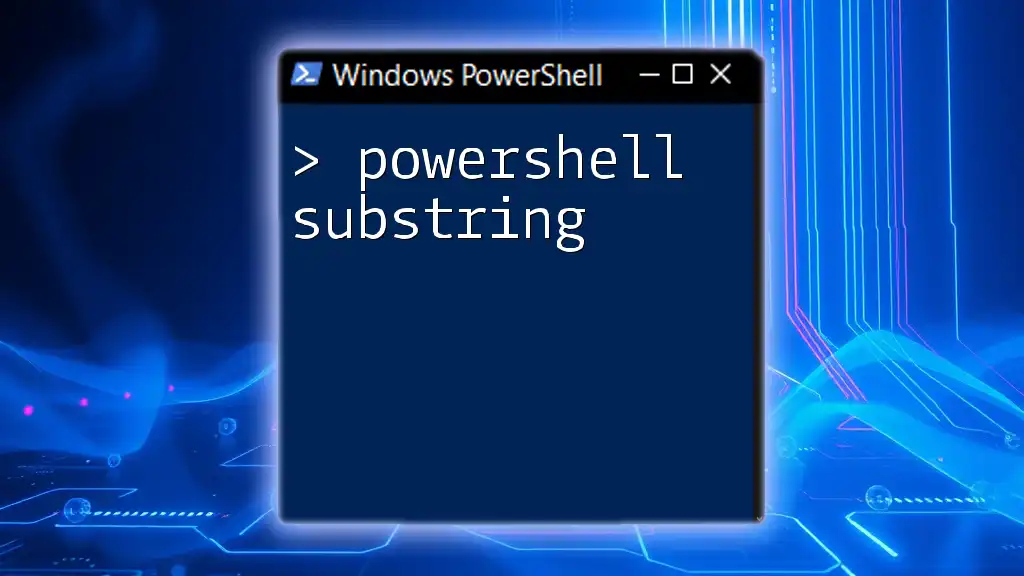
Creating Strings in PowerShell
Basic String Creation
Creating strings in PowerShell is straightforward. You can assign a string to a variable easily:
$greeting = "Hello, PowerShell!"
The variable `$greeting` now holds the string value.
String Interpolation
String interpolation allows you to include variables and expressions directly within your strings. This feature simplifies dynamic string creation. For example:
$name = "John"
$message = "Hello, $name!"
Here, the value of `$name` is injected into the string, resulting in `Hello, John!`.
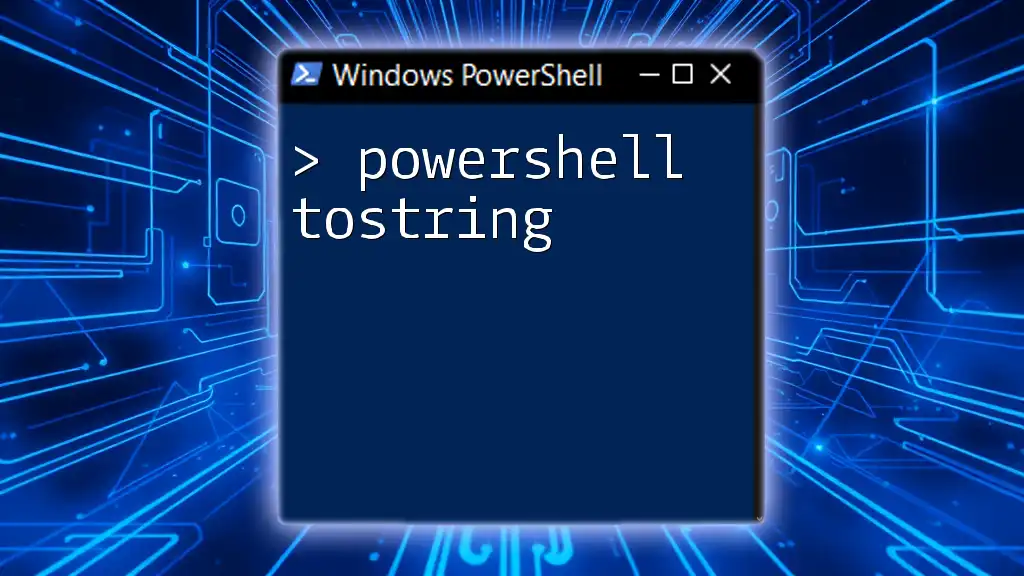
String Methods and Properties
Built-in String Methods
PowerShell provides numerous built-in string methods to facilitate string manipulation.
.Length
The `.Length` property returns the number of characters in a string. For example:
$string = "PowerShell"
$string.Length
This command would output `10`, representing the length of the string.
.ToUpper() and .ToLower()
These methods allow you to convert strings to uppercase or lowercase, respectively. For instance:
$string = "Hello"
$string.ToUpper() # Outputs "HELLO"
$string.ToLower() # Outputs "hello"
.Substring(startIndex, length)
The `.Substring()` method extracts a specific portion of a string. For example:
$string = "PowerShell"
$subString = $string.Substring(0, 5) # Outputs "Power"
.Replace(oldValue, newValue)
This method replaces occurrences of a specified substring with another substring:
$string = "Hello, World!"
$newString = $string.Replace("World", "PowerShell") # Outputs "Hello, PowerShell!"
.Trim()
Trimming is essential for removing whitespace from the start and end of strings. For example:
$string = " Hello "
$trimmedString = $string.Trim() # Outputs "Hello"
String Properties
PowerShell strings come with several useful properties that enhance their functionality. You can access individual characters using an index:
$string = "PowerShell"
$firstCharacter = $string[0] # Outputs "P"
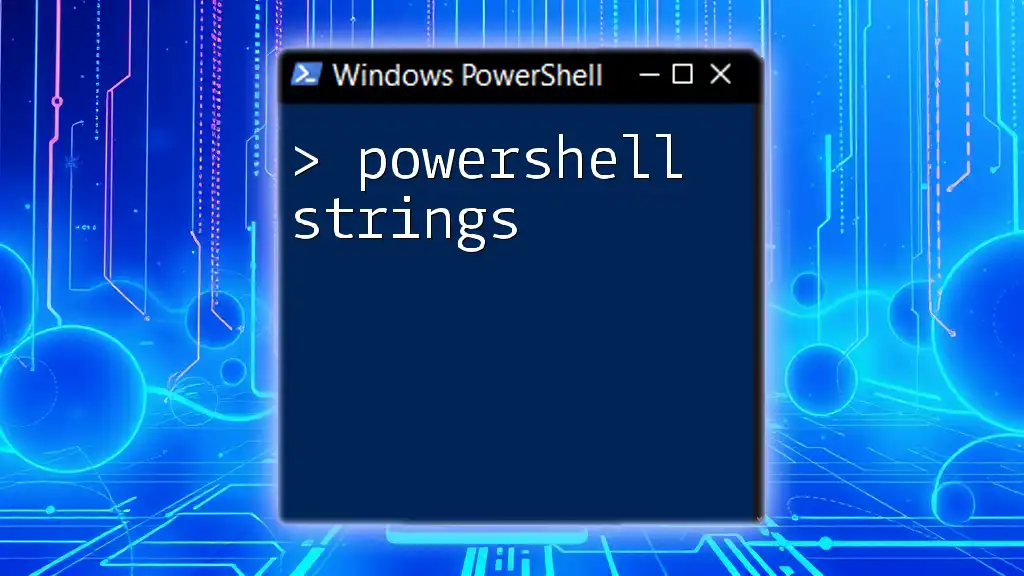
Working with String Arrays
Creating String Arrays
An array is a collection of items, and PowerShell allows you to create string arrays using the `@()` syntax. For example:
$fruits = @("Apple", "Banana", "Cherry")
Accessing Array Elements
You can easily access individual elements in a string array by referencing their index. For example:
$fruits[1] # Outputs "Banana"
Joining and Splitting Strings
Join-Strings
You can combine elements of an array into a single string using the `-join` operator. For instance:
$fruits -join ", " # Outputs "Apple, Banana, Cherry"
Split String
The `.Split()` method allows you to split a string into an array based on a delimiter. For example:
$string = "One,Two,Three"
$array = $string.Split(",") # Outputs an array: "One", "Two", "Three"
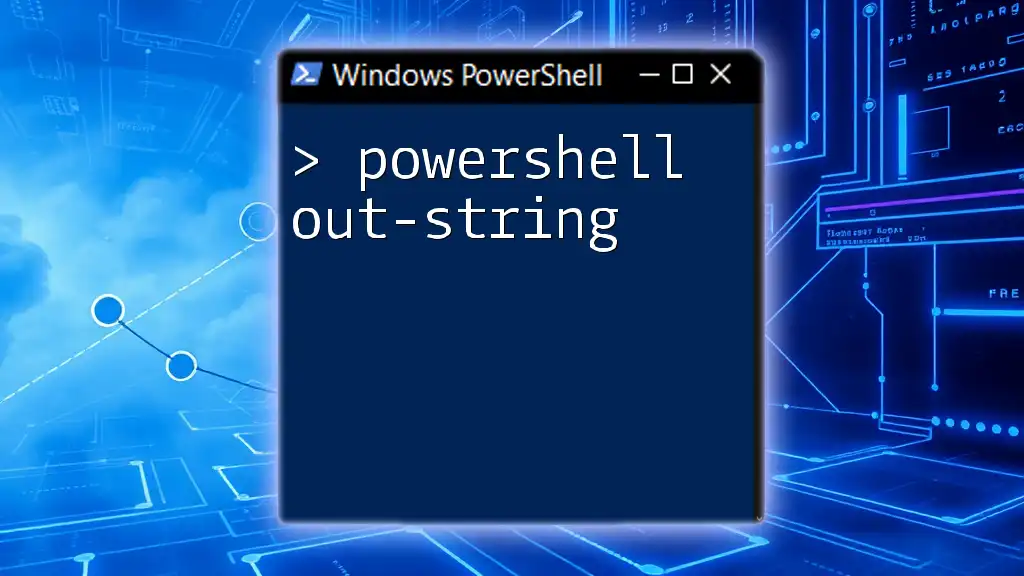
String Formatting
String Format Operator - `-f`
The format operator `-f` allows you to create formatted strings by substituting values into a predefined template. For example:
$name = "Alice"
$greeting = "Hello, {0}!" -f $name # Outputs "Hello, Alice!"
Using Composite Formatting
You can pass multiple values to format multiple placeholders. This is useful for creating complex messages:
$age = 30
"{0} is {1} years old." -f $name, $age # Outputs "Alice is 30 years old."
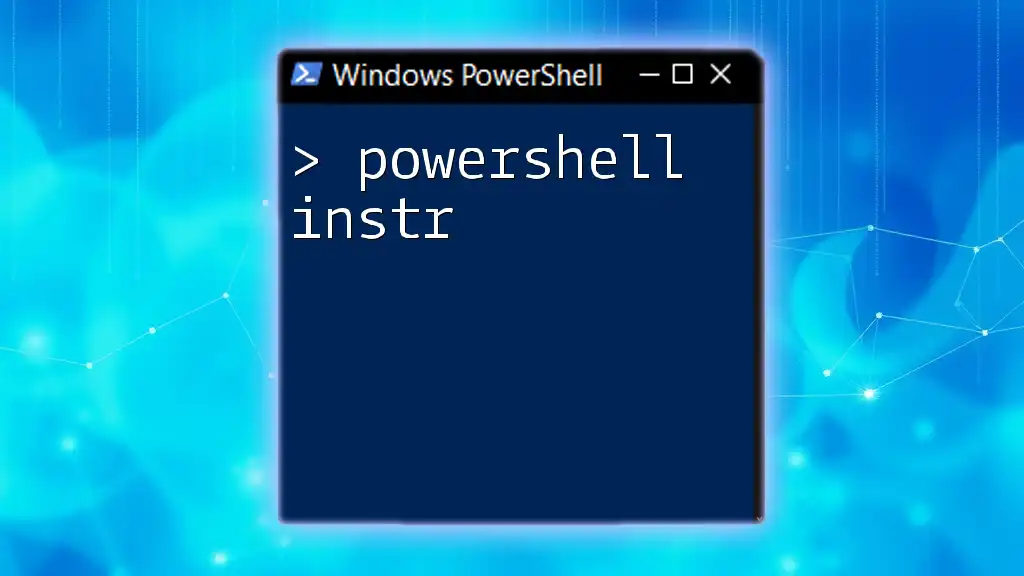
Special Characters and Escape Sequences
Common Special Characters
PowerShell strings can contain special characters that serve particular purposes (e.g., newline `n`, tab `t`). Understanding these is crucial for managing string outputs effectively.
Using Backticks for Escaping
In PowerShell, you can use the backtick character (`) to escape special characters and ensure they are treated as literals. For example:
$path = "C:\Program Files\MyApp\"
$escapedPath = "C:`\Program Files\MyApp\"
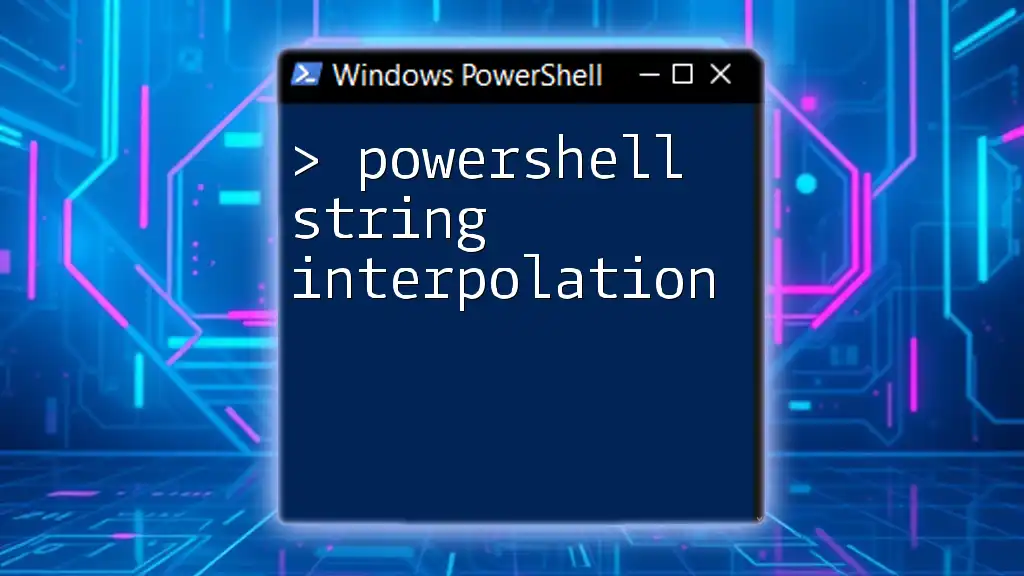
Practical Applications of String Manipulation
Real-World Use Cases
Logging
Strings are often used in logging operations. You can create informative logs using string manipulation to capture system activities or errors.
Generating Dynamic Messages
In automation scripts, you may need to generate dynamic notifications or messages based on user input or system status. String manipulation enables you to form these messages effectively.
Data Retrieval
Strings are crucial for retrieving and formatting data from text files, JSON responses, or API calls. Understanding string handling can enhance your data processing skill set significantly.
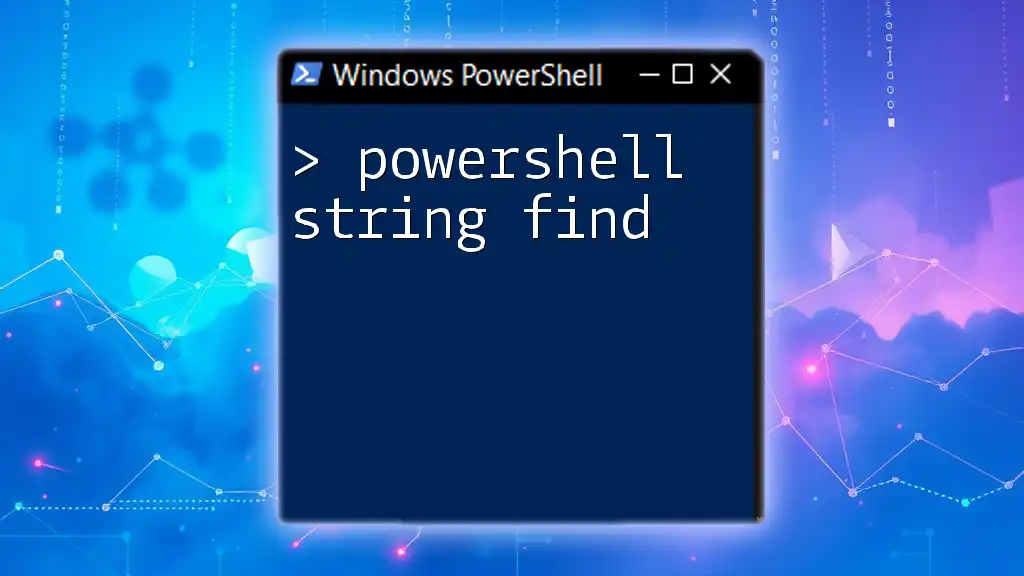
Conclusion
Mastering how to manipulate strings in PowerShell is vital for optimizing your scripting capabilities. Whether you're managing variables, formatting text, or processing data, understanding strings will improve your efficiency and effectiveness as a PowerShell user. Continue to explore and practice string operations to refine these skills in your scripting endeavors.
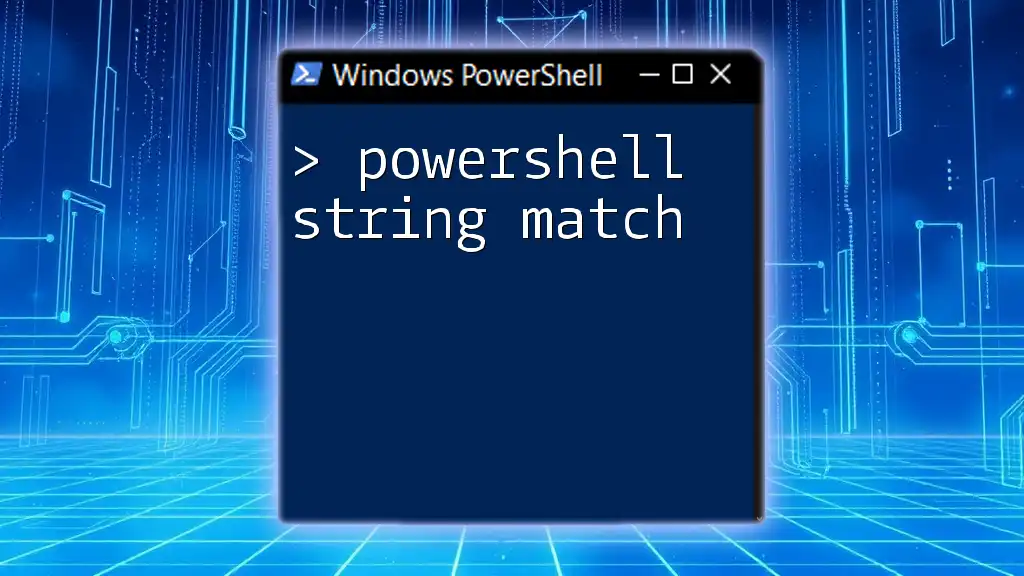
Additional Resources
To deepen your understanding, consider exploring the official PowerShell documentation and engaging with community forums for practical tips and tricks related to string manipulation. Practice is key—experiment with different string methods and properties to enhance your PowerShell prowess.