In PowerShell, you can determine the length of a substring by specifying the start index and the number of characters you want to extract from a string using the `Substring()` method.
Here's a code snippet demonstrating how to use it:
$originalString = "Hello, World!"
$substring = $originalString.Substring(0, 5) # Extracts "Hello"
$length = $substring.Length # Gets the length of the substring
Write-Host "The substring is '$substring' and its length is $length."
Understanding Substrings
What is a Substring?
A substring is a contiguous sequence of characters within a string. Essentially, it is a part of a parent string from which it is derived. For instance, in the string "PowerShell," the substring "Power" is formed from the first five characters.
Why Use Substrings in PowerShell?
Strings are prevalent in scripting and command-line tasks. The ability to manipulate these strings using substrings allows you to extract or modify specific parts, making it easier to process text data effectively. Common use cases include data parsing from log files, extracting usernames from email addresses, or manipulating CSV content. Understanding how to handle substrings can significantly speed up your scripting capabilities.

The Substring Method in PowerShell
Syntax of the Substring Method
The PowerShell `Substring` method is a simple yet powerful tool for extracting parts of strings. Its basic syntax is as follows:
$substring = $string.Substring(startIndex, length)
Here, `startIndex` represents the position from which to start extracting the substring, and `length` indicates how many characters to take from that position.
Practical Examples
Basic Example of Substring
To understand the fundamental operation of the `Substring` method, consider the following example:
$string = "Hello, PowerShell!"
$substring = $string.Substring(7, 11)
Write-Output $substring # Outputs: PowerShell
In this example, the method extracts 11 characters starting from index 7, resulting in "PowerShell."
Extracting Specific Parts of a String
Substrings are incredibly useful for pulling specific sections from strings. For instance, extracting a username from an email address can be elegantly accomplished like this:
$email = "user@example.com"
$username = $email.Substring(0, $email.IndexOf("@"))
Write-Output $username # Outputs: user
Here, we use `IndexOf` to find the position of the "@" symbol and extract everything to the left of it.

Determining the Length of a Substring
Using the Length Property
Understanding the length of strings is critical in substring operations. Each string in PowerShell has a `.Length` property that can be leveraged. For example:
$length = $substring.Length
Example of Length Calculation
To demonstrate how to calculate the length of a substring, observe the following code snippet:
$string = "PowerShell Scripting"
$substring = $string.Substring(0, 10)
$length = $substring.Length
Write-Output $length # Outputs: 10
In this instance, the length of the substring "PowerShell" is calculated to be 10 characters.
Handling Edge Cases
Substring Out of Range
One common pitfall when using substrings is specifying an invalid `startIndex` or `length`. PowerShell will throw an exception if these values are out of bounds. For example:
# Trying to extract from index 20 will cause an exception
$errorSubstring = $string.Substring(20, 5) # Index out of range
Careful validation before using indices can prevent such errors.
Default Behavior of Length in Substring
If you omit the length parameter from the `Substring` method, PowerShell extracts from the specified `startIndex` to the end of the string:
$substring = $string.Substring(6) # Starts from index 6 to the end
This can be handy when you want the remainder of the string without needing to calculate its total length.
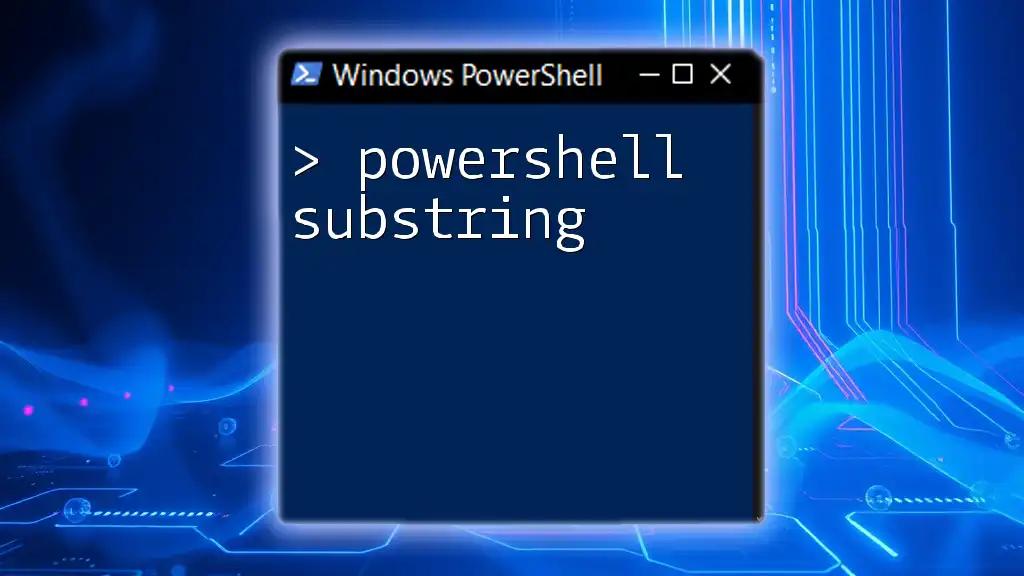
Advanced String Manipulation
Combining Substring with Other Methods
Using `IndexOf` Method
Integrating the `IndexOf` method with `Substring` enhances your ability to extract data. For instance:
$string = "Find the position in PowerShell"
$startIndex = $string.IndexOf("in")
$substring = $string.Substring($startIndex, 2)
Write-Output $substring # Outputs: in
This example showcases how to locate the position of a substring within a string dynamically.
Real-World Applications
The versatility of substrings proves invaluable in a range of data processing scenarios. For instance, you might extract dates from a log file or parse delimiters in a CSV file. Being proficient in substring manipulation allows for efficient data sanitization and presentation.

Best Practices for Using Substrings
Avoiding Common Mistakes
To ensure smooth substring operations, always validate your indices against the string's length. Using conditional checks can prevent runtime errors and preserve script integrity.
Performance Considerations
If you're working with large strings or performing operations on many substrings, be mindful of performance. Inefficient substring extraction can lead to slower script execution. It’s often useful to minimize redundant calculations by storing frequently accessed lengths or indices.
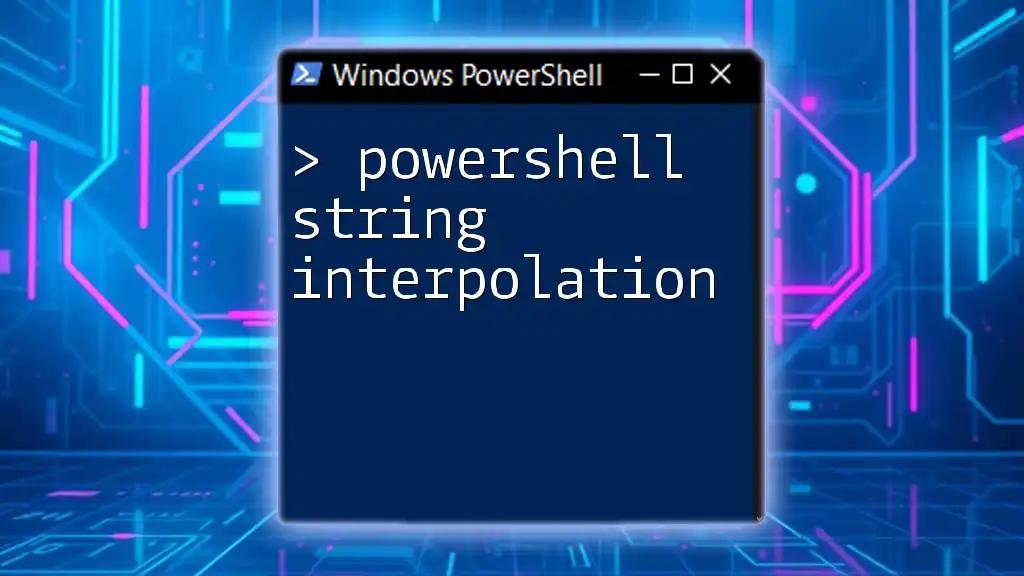
Conclusion
In summary, understanding the concept of PowerShell substring length is a crucial component of effective string manipulation in scripting. With effective use of the `Substring` method and its associated properties, you can enhance your PowerShell scripts significantly. Practice extracting and manipulating substrings in various scenarios to become proficient in efficient scripting.
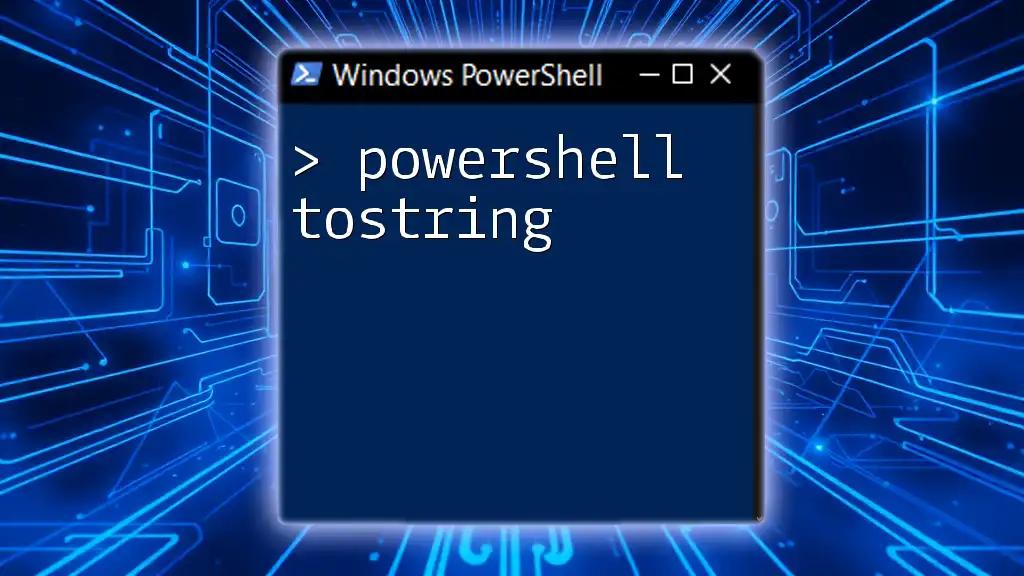
References and Further Reading
For more in-depth exploration, you may want to consult the official Microsoft documentation on [PowerShell String Manipulation](https://docs.microsoft.com/powershell/scripting/learn/deep-dives/strings?view=powershell-7.1), offering a wealth of examples and guidance. Additionally, consider engaging with online courses or tutorials to deepen your understanding of PowerShell and its capabilities.
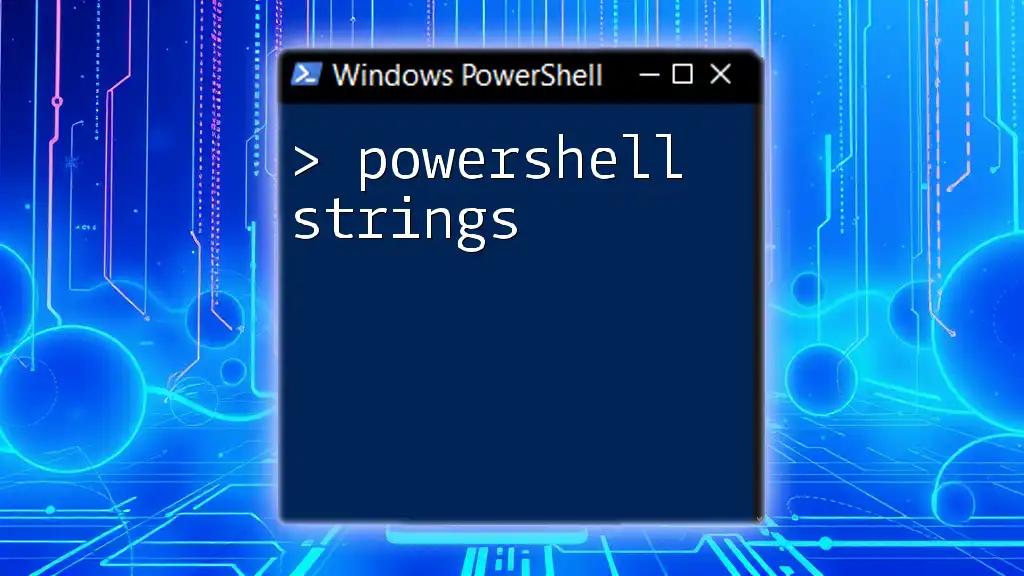
Call to Action
Ready to take your PowerShell skills to the next level? Sign up for our courses today to explore various PowerShell topics and enhance your string manipulation techniques with practical examples and expert guidance!