The `LastWriteTime` property in PowerShell allows you to retrieve the last modification date and time of a file, which can be accessed through the `Get-Item` or `Get-ChildItem` cmdlets.
Here’s a simple example of how to use it:
Get-Item "C:\Path\To\Your\File.txt" | Select-Object LastWriteTime
Understanding LastWriteTime in PowerShell
What is LastWriteTime?
LastWriteTime refers to the timestamp that indicates the last time a file was modified. It is a crucial part of file management, allowing users to track changes made to files over time. Understanding LastWriteTime is essential, especially for tasks such as file backups, version control, and even system administration. This timestamp helps in deciding which versions of files should be retained or discarded based on recency.
How LastWriteTime Works
Each file in a file system possesses a few key timestamps: CreationTime, LastAccessTime, and LastWriteTime. The LastWriteTime specifically updates every time the file's content changes, making it a reliable reference point for authors, developers, and IT professionals.
When you manipulate files in Windows—saving, editing, or deleting them—Windows automatically updates the LastWriteTime, reflecting the last moment the file was altered. Grasping how this works helps in better management of file systems and can offer insights into working habits or system performance.
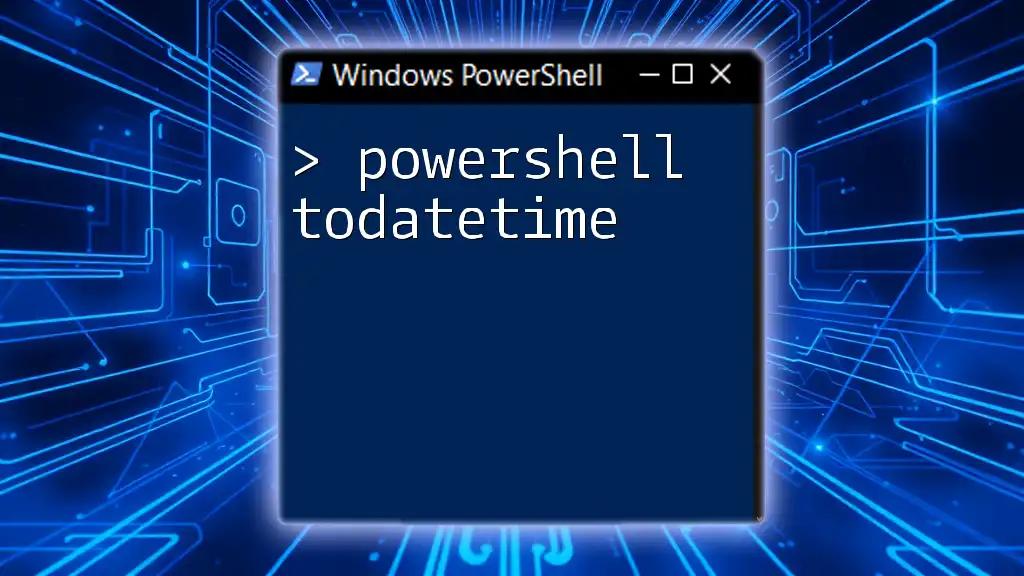
Retrieving LastWriteTime with PowerShell
Using Get-Item and Get-ChildItem
One of the primary ways to fetch LastWriteTime is by using the Get-Item or Get-ChildItem cmdlets in PowerShell.
To get the LastWriteTime of a single file, you can use the following command:
$file = Get-Item "C:\example\file.txt"
$file.LastWriteTime
This command retrieves the specified file and outputs its LastWriteTime directly. The output will show the precise date and time the file was last modified, providing immediate insights into the file's recency.
Listing LastWriteTime for Multiple Files
To gather information on the LastWriteTime for multiple files within a directory, Get-ChildItem is the way to go. The following example lists all files in a directory along with their LastWriteTime:
Get-ChildItem "C:\example" | Select-Object Name, LastWriteTime
You can also filter files by specific types. If you only want to see `.txt` files in the directory, you can use:
Get-ChildItem "C:\example" -Filter "*.txt" | Select-Object Name, LastWriteTime
This command will display the names and LastWriteTimes of all text files in the specified directory. It is particularly helpful for auditing or managing files based on their last modified dates.
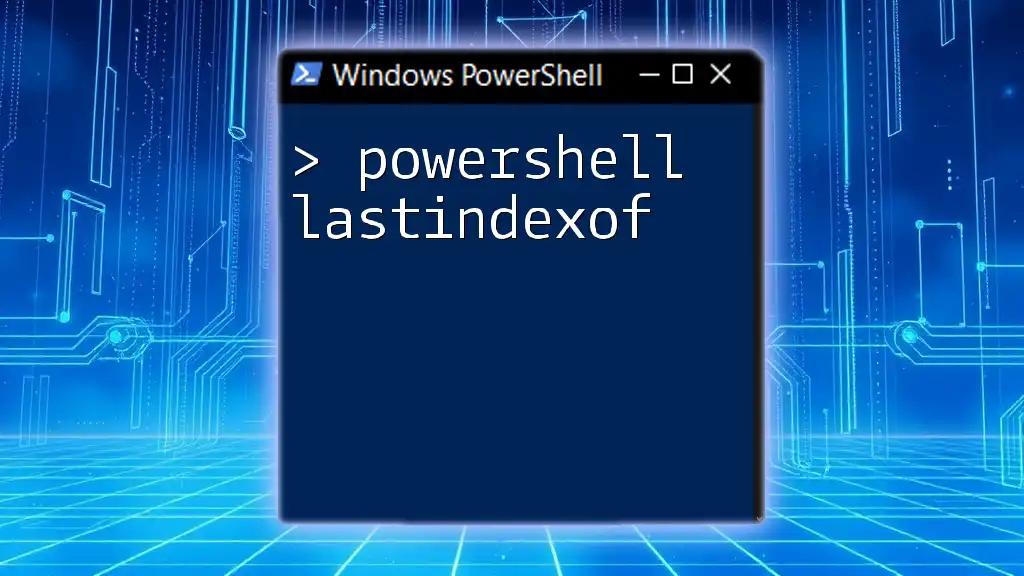
Manipulating LastWriteTime in PowerShell
Changing LastWriteTime of a File
Sometimes, you may need to update a file's LastWriteTime manually. This can be useful for testing or auditing purposes. You can change a file's LastWriteTime like this:
(Get-Item "C:\example\file.txt").LastWriteTime = "2023-10-01 10:00:00"
With this command, you're setting the LastWriteTime to a specified date and time. Be cautious: altering a file's LastWriteTime can potentially disrupt version control systems or auditing processes that rely on timestamps for accuracy.
Resetting LastWriteTime to Current Time
To reset the LastWriteTime to the current date and time, you can use the `Get-Date` cmdlet. This is useful for indicating that a file has just been created or downloaded.
(Get-Item "C:\example\file.txt").LastWriteTime = Get-Date
By executing this command, you’ll refresh the LastWriteTime to the moment the command is run, representing its current state.
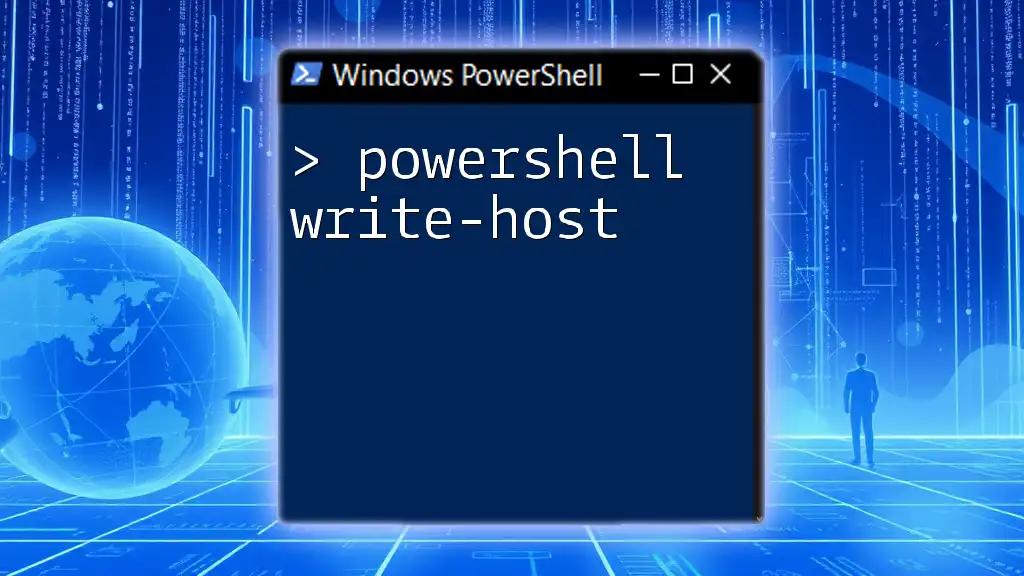
Practical Use Cases of LastWriteTime
File Backup and Version Control
LastWriteTime serves a vital role in effective file backup and version control. By tracking when files were modified, organizations can identify which versions of files are the most current and eliminate redundant or older backups. For example, if you're in a content management system, comparing LastWriteTimes can help manage document revisions and ensure that team members are working on the latest version.
Automating File Management Tasks
You can also automate file management tasks using LastWriteTime. A common scenario involves logging the LastWriteTime of files for monitoring purposes. The following script logs the LastWriteTime for all files in a specific directory into a text file:
$files = Get-ChildItem "C:\example"
foreach ($file in $files) {
"$($file.Name): $($file.LastWriteTime)" | Out-File "C:\logs\file_log.txt" -Append
}
This script iterates through each file in the specified directory and appends the name and LastWriteTime to a log file. This can be an invaluable tool for system administrators who need to monitor file activity and maintain records over time.
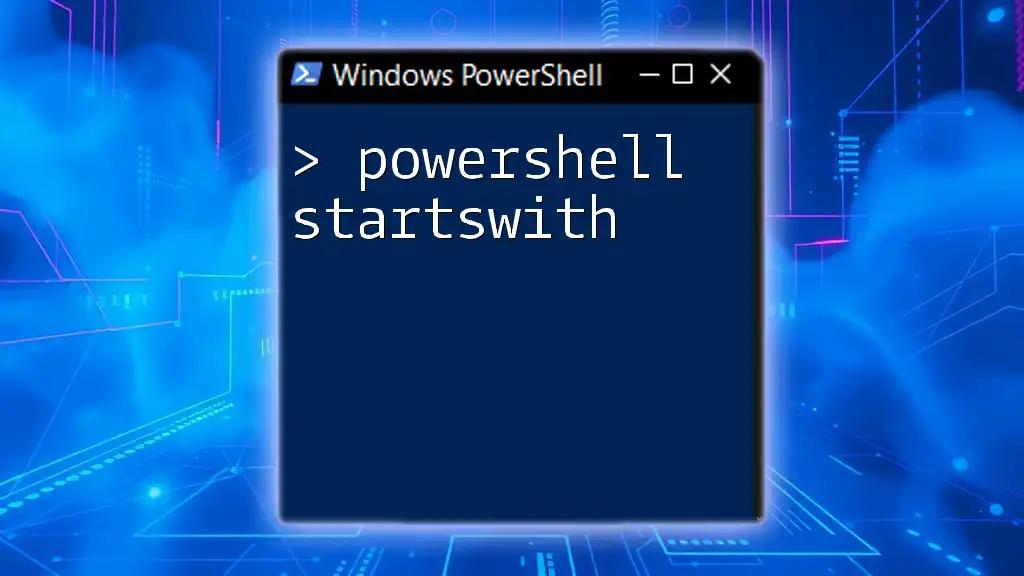
Best Practices for Working with LastWriteTime
Validating Changes
When you perform operations that affect LastWriteTime, always validate the changes afterward. A useful practice is to immediately fetch and verify the LastWriteTime of a file post-modification to ensure that the changes were applied correctly. This helps maintain the integrity of your files.
Performance Considerations
When querying large directories, consider that extensive retrieval commands may impact system performance. To optimize, use filtering options wisely, and limit the number of properties returned. Using the `-Recurse` parameter may slow the process when operating on extensive file trees, so judicious use is advisable.
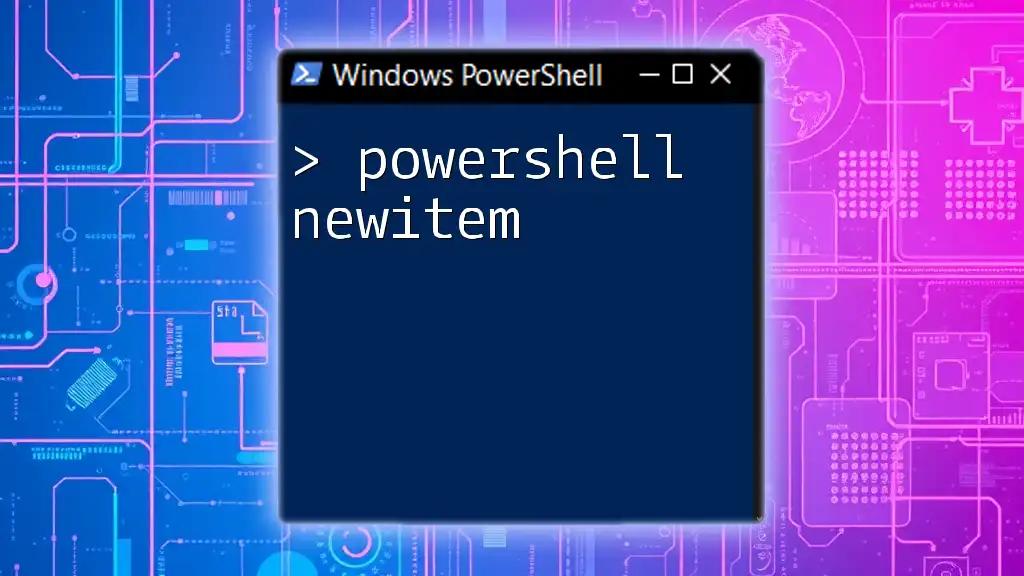
Conclusion
Recap of Key Concepts
Understanding and effectively utilizing PowerShell LastWriteTime opens numerous avenues for efficient file management. From retrieval and manipulation to monitoring and auditing, mastering this concept can streamline many processes.
Next Steps for Learning More
To deepen your knowledge of PowerShell and LastWriteTime, explore various resources available online, including Microsoft's documentation and community forums. Hands-on practice with different cmdlets and scenarios will enhance your learning significantly.
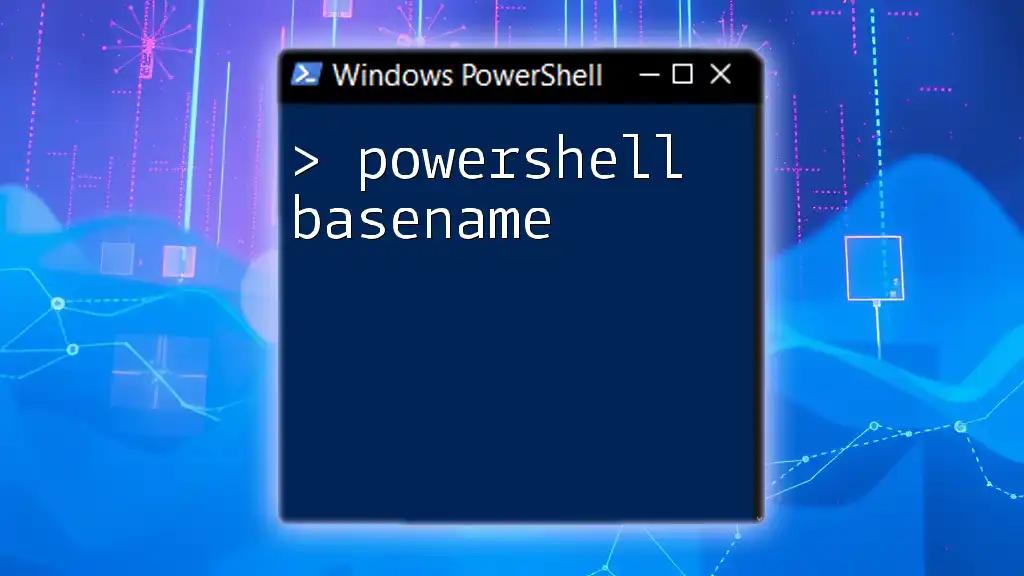
Frequently Asked Questions (FAQ)
Can I retrieve LastWriteTime for files on remote systems?
Yes, using PowerShell Remoting with Invoke-Command allows you to run commands that can return LastWriteTime from files stored on remote machines.
What if LastWriteTime is incorrect?
Incorrect LastWriteTime can stem from system errors, improper file operations, or human error. If needed, you can adjust the timestamp to reflect the correct date and time.
How to compare LastWriteTime between files?
You can compare LastWriteTime by retrieving it for multiple files and using conditional statements to identify differences. A simple example might involve storing the LastWriteTime properties in variables and comparing them with basic if-else logic.
By leveraging the capabilities of PowerShell LastWriteTime, you'll empower your file management processes and enhance your overall productivity.