In PowerShell, you can overwrite a file by using the `Set-Content` cmdlet, which allows you to replace the existing content of the file with new data.
Set-Content -Path "C:\path\to\your\file.txt" -Value "This is the new content."
Understanding File Overwriting
Definition of Overwriting a File
Overwriting a file means replacing the content of an existing file with new data. When you overwrite a file, the original content is deleted and can no longer be retrieved unless a backup exists. This action can be critical in scenarios such as automated logging, where outdated log files need to be refreshed without cluttering the storage with multiple versions.
Risks of Overwriting Files
Overwriting files carries inherent risks, primarily the potential for data loss. If an important document is overwritten by mistake, retrieving it may be impossible without a backup. Therefore, it's crucial to adopt best practices for safe overwriting, such as:
- Always create backups of critical files before overwriting them.
- Ensure proper error handling in your scripts to avoid unintentional overwrites.
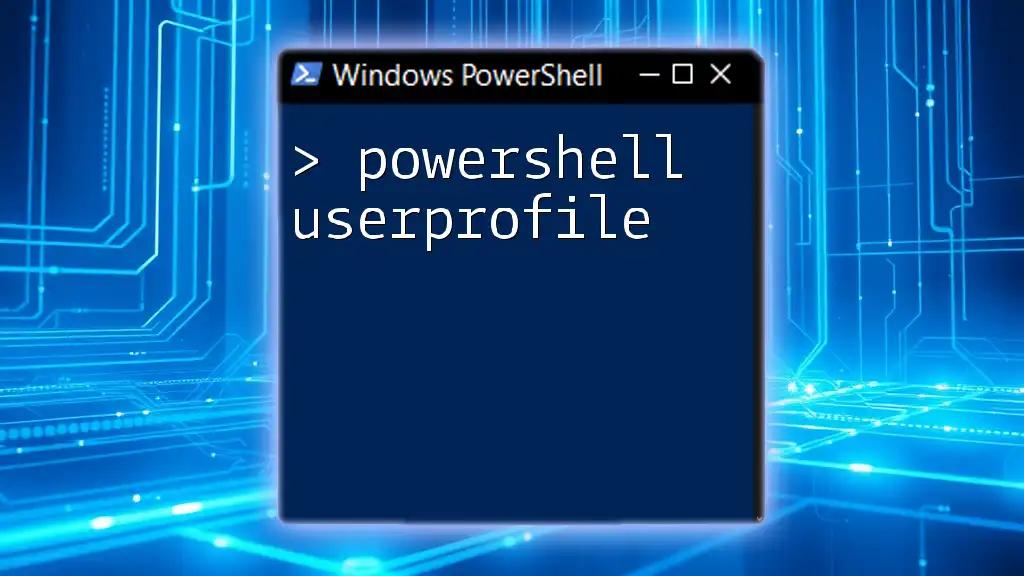
PowerShell Commands for File Overwriting
Using the `Set-Content` cmdlet
The `Set-Content` cmdlet is one of the primary methods for overwriting files in PowerShell. It allows you to specify a file path and the new content you wish to write.
Syntax Overview:
Set-Content -Path "filepath" -Value "new content"
Example: To overwrite a text file named `sample.txt` with new content, use the following command:
Set-Content -Path "C:\example\sample.txt" -Value "This is new content"
Upon execution, the previous content of the file will be erased, and the new text will be written in its place.
Using the `Add-Content` cmdlet
While `Add-Content` is typically used for appending data to a file, knowing the distinction is essential. If you need to add content without replacing the current data, `Add-Content` would be appropriate. For example:
Add-Content -Path "C:\example\sample.txt" -Value "This will be added without deleting existing content."
However, if you specifically want to overwrite a file, stick with `Set-Content`.
Using the `Out-File` cmdlet
Another option for file manipulation is the `Out-File` cmdlet. It writes data to a specified file and can also be used to overwrite it.
Syntax Overview:
Out-File -FilePath "filepath" -InputObject "new content"
Example: To overwrite `sample.txt` using `Out-File`:
"Overwrite this content" | Out-File -FilePath "C:\example\sample.txt"
This command uses PowerShell's pipeline feature to send the string directly to `Out-File`, resulting in overwriting the existing file content.
Using Redirection Operators
Redirection operators are a more concise way to overwrite files in PowerShell.
The Greater Than Operator (`>`)
The greater than operator (`>`) is a simple and effective method for overwriting files. It takes output from a command and directs it to a file.
Example:
"This is the new content of the file" > "C:\example\sample.txt"
This command completely replaces the current contents of the file with the specified text. It's essential to remember that this action is immediate and replaces without confirmation.
The Double Greater Than Operator (`>>`)
In contrast, the double greater than operator (`>>`) is used for appending content to a file rather than overwriting it. For example:
"This will be added to the existing file." >> "C:\example\sample.txt"
When using this operator, previous content remains unaffected, which is useful when you want to retain old data.
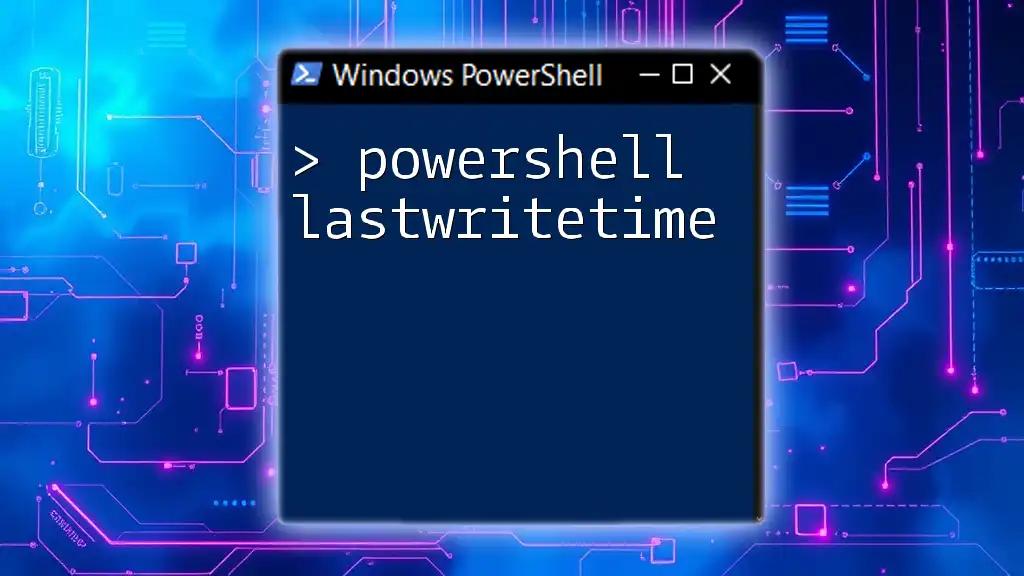
Safeguards for Overwriting Files
Backing Up Files Before Overwriting
Always prioritize backing up files before performing any overwrite actions. You can use the `Copy-Item` cmdlet to create a backup:
Copy-Item -Path "C:\example\sample.txt" -Destination "C:\example\backup_sample.txt"
This practice ensures that you have a safety net in case of accidental overwrites.
Implementing User Confirmation
To further mitigate risks, consider scripting a user confirmation prompt before overwriting files. Here’s an example code snippet:
$confirm = Read-Host "Are you sure you want to overwrite the file? (Y/N)"
if ($confirm -eq 'Y') {
Set-Content -Path "C:\example\sample.txt" -Value "This is new content"
}
This simple script asks the user for confirmation, adding an extra layer of security to the overwriting process.
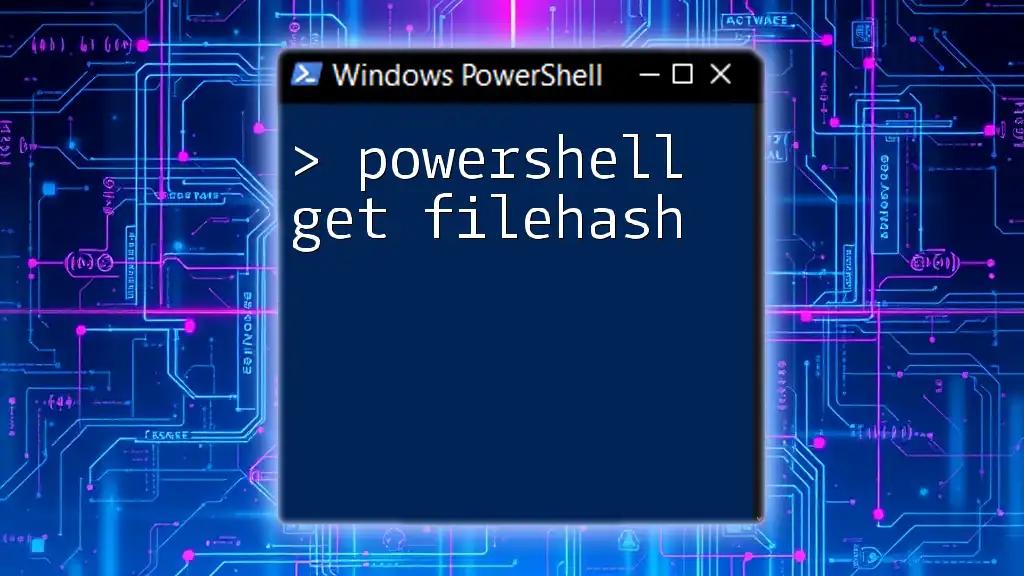
Additional Examples and Use Cases
Scheduled Tasks for File Overwriting
PowerShell can be particularly powerful in scheduled tasks where regular updates to logs or reports are needed. For instance, you might set up a daily task that overwrites a log file with new data.
Overwriting Multiple Files
You can also use loops to overwrite multiple files in one go. Utilize `Get-ChildItem` to target multiple files based on criteria. An example is as follows:
$files = Get-ChildItem "C:\example\*.txt"
foreach ($file in $files) {
Set-Content -Path $file.FullName -Value "New content for multiple files."
}
This script goes through all `.txt` files in the specified directory and overwrites their content, demonstrating the scalability of PowerShell commands.
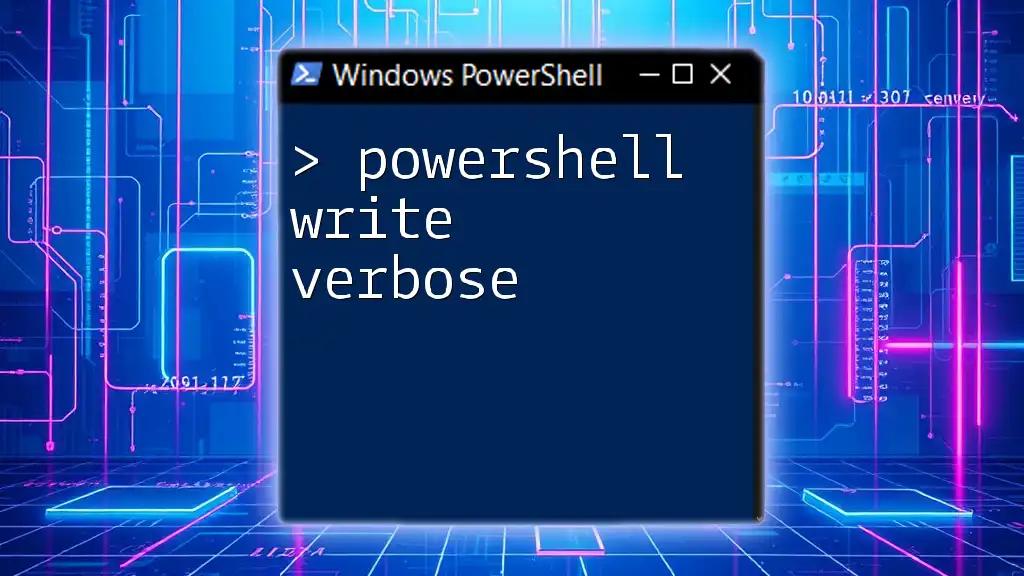
Conclusion
In conclusion, mastering how to powershell overwrite file actions is an essential skill for anyone engaged in scripting or automation. By using the appropriate cmdlets, understanding redirection, and implementing safeguards, you can perform file manipulation tasks efficiently and safely. Always ensure to practice safe overwriting techniques and explore further resources to dive deeper into PowerShell scripting.