In PowerShell, the `Write-Verbose` command allows you to output detailed information to the console when the `$VerbosePreference` variable is set to 'Continue', providing additional context during script execution for better debugging and understanding.
Write-Verbose 'This is a verbose message' -Verbose
Understanding Verbosity in PowerShell
What is Verbosity?
In the context of scripting, verbosity refers to the level of detail provided in output messages. In PowerShell scripts, verbose output serves as a crucial tool for enhancing clarity, particularly during testing and debugging.
The Role of Write-Verbose
The `Write-Verbose` cmdlet in PowerShell allows developers to send detailed messages to the output stream when executing a script. Unlike `Write-Output`, which displays content directly or as part of object pipeline processing, or `Write-Host`, which simply prints to the console without contributing to the output stream, `Write-Verbose` serves a different purpose.
Verbosity preference variable plays a significant role in determining whether verbose messages are displayed. By default, verbose messages are suppressed unless the user explicitly includes the `-Verbose` flag during execution.
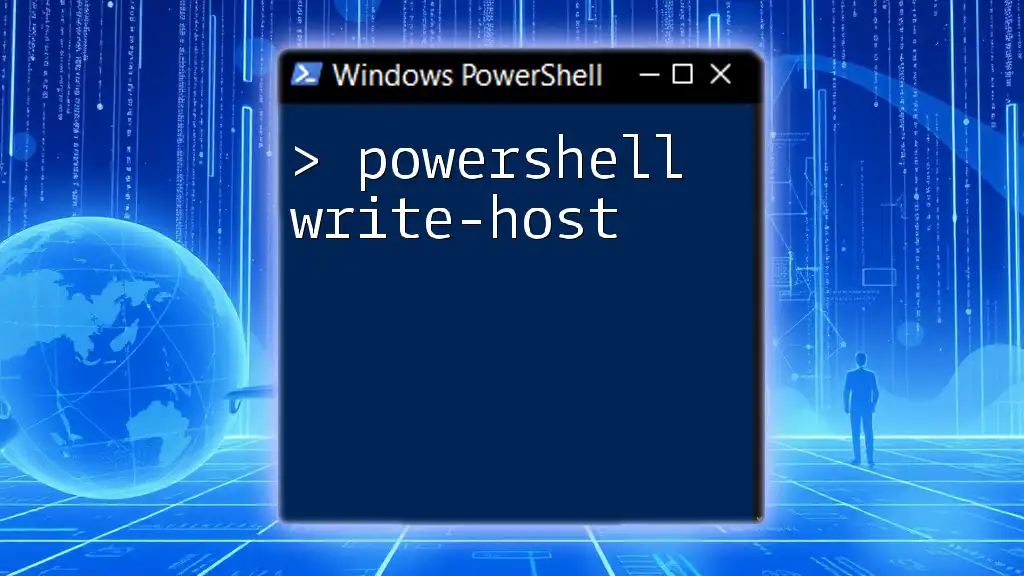
How to Use Write-Verbose
Basic Syntax
The basic syntax for `Write-Verbose` is quite straightforward. Here’s a simple example:
Write-Verbose "This is a verbose message" -Verbose
In this command, `"This is a verbose message"` will only appear in the output if the `-Verbose` switch is applied.
Enabling Verbose Output
To enable verbose output in your PowerShell scripts, you simply need to use the `-Verbose` switch with the cmdlet or function call. For instance, when you run a command like the following:
Get-Process -Verbose
You'll receive detailed information about the processes being fetched, which can be especially invaluable during troubleshooting scenarios.
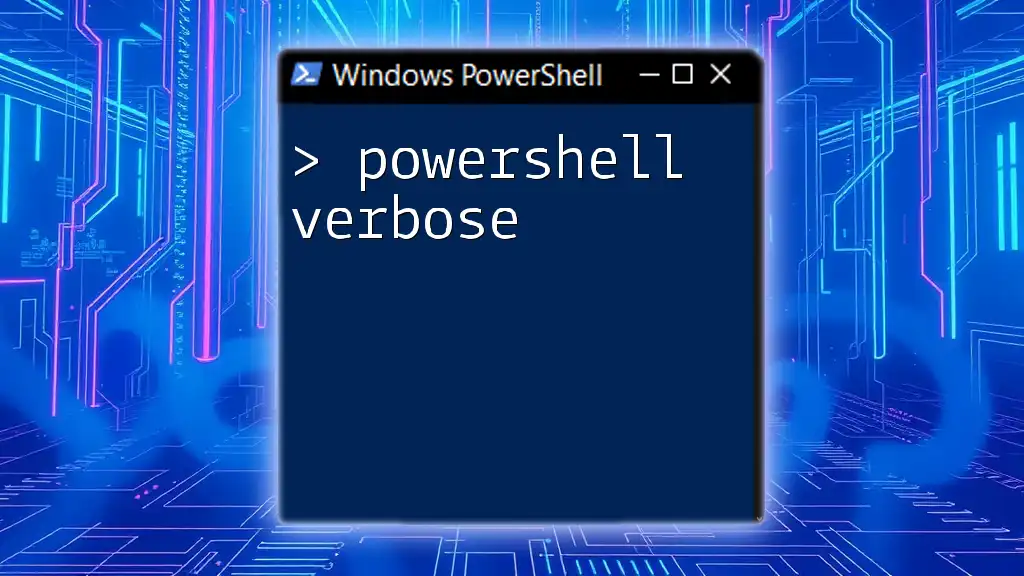
Practical Use Cases for Write-Verbose
Debugging Scripts
Verbose messages can significantly aid in debugging scripts by providing insights into the script execution process. For instance:
function Test-Script {
[CmdletBinding()]
param (
[string]$Name
)
Write-Verbose "Starting the Test-Script function."
Write-Verbose "Received name: $Name"
# Further processing...
Write-Verbose "Ending the Test-Script function."
}
Test-Script -Name "Example" -Verbose
In this example, `Test-Script` not only processes the name but also outputs informative messages, giving the debugger clarity on the function's progression.
Provides Feedback to Users
Using `Write-Verbose` can enhance user experience by offering feedback throughout lengthy processes. For instance, consider a loop where each iteration requires time to complete:
for ($i = 1; $i -le 5; $i++) {
Write-Verbose "Iteration $i started."
Start-Sleep -Seconds 1 # Simulating a time-consuming task
Write-Verbose "Iteration $i completed."
}
Here, users receive real-time updates about the script's progress, improving engagement and understanding.
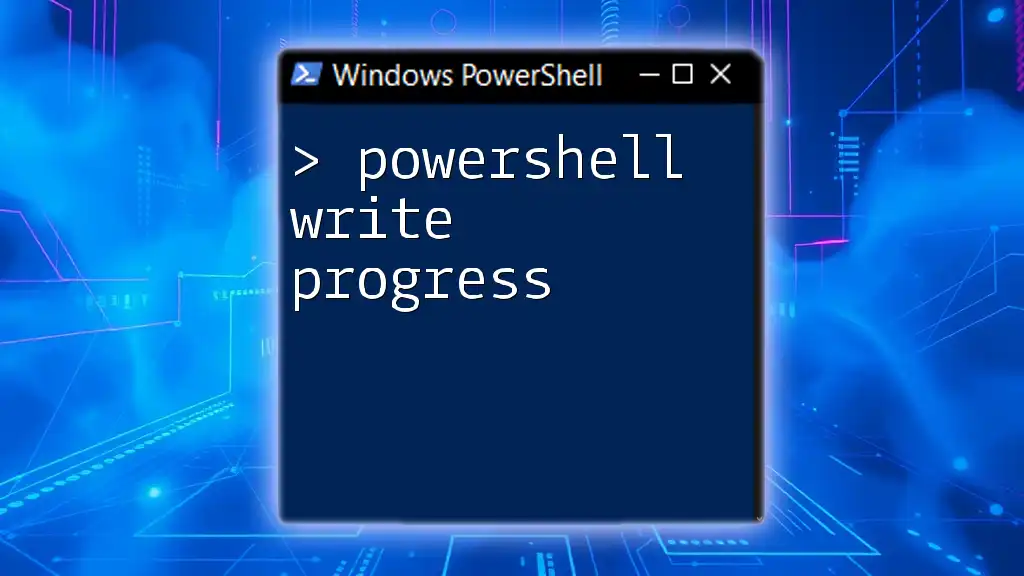
Best Practices for Using Write-Verbose
Keeping Messages Concise
When writing verbose messages, keep them concise and relevant. Clarity is critical — verbose output should enhance understanding rather than overwhelm it. For example, rather than saying "Processing data for user with ID: 12345...", you may say "Processing data for User ID 12345."
Avoiding Overuse
While verbose messaging is beneficial, overuse can lead to clutter. It can create confusion, especially in large scripts with many verbose messages. Strive to maintain balance by ensuring that only critical information is conveyed.
Integrating with Other Cmdlets
Combining `Write-Verbose` with complementary cmdlets, such as `Try/Catch`, provides robust error handling:
try {
Write-Verbose "Attempting to fetch data..."
Get-Content "path/to/file.txt" -ErrorAction Stop
Write-Verbose "Data fetched successfully."
} catch {
Write-Verbose "An error occurred: $_"
}
This block not only attempts to get the specified content but also provides verbose information if the operation fails, helping both developers and users to pinpoint issues.
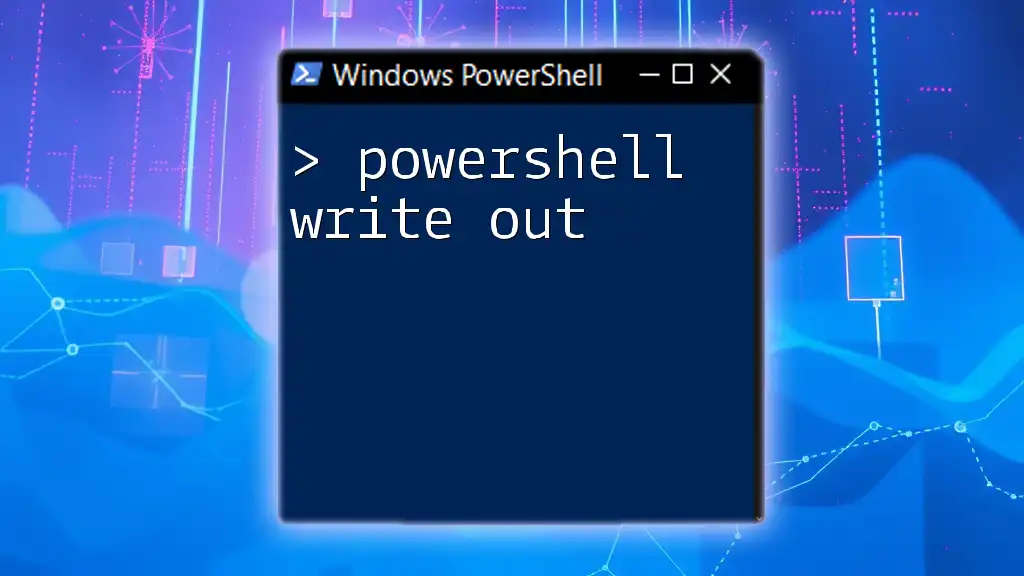
Conclusion
In summary, PowerShell Write-Verbose offers a crucial method for sending detailed messages, facilitating effective communication during script execution. By implementing these practices, not only can you enhance clarity in your scripts, but you can also significantly simplify the debugging process. The ability to inform users during script execution is invaluable, ensuring they remain informed and engaged.
Keep practicing and integrating `Write-Verbose` into your scripts, and you'll appreciate its power in creating clear, user-friendly PowerShell routines.
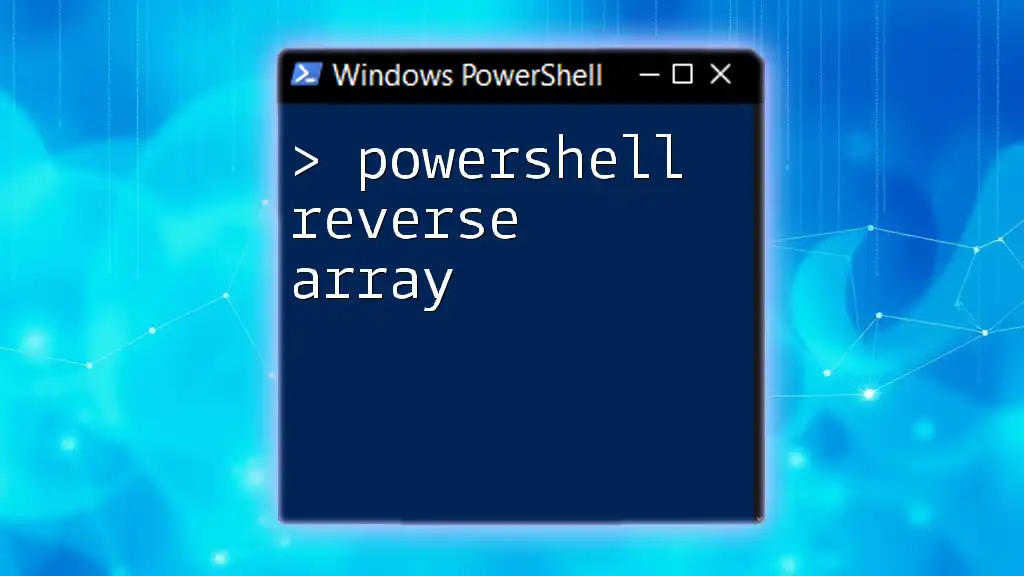
Additional Resources
For further exploration of `Write-Verbose`, consult the official PowerShell documentation for a deeper understanding and discover community resources, tutorials, and forums that can augment your PowerShell knowledge.