In PowerShell, you can reverse an array using the `Reverse()` method, which rearranges the elements in the opposite order.
Here's the code snippet:
$array = 1, 2, 3, 4, 5
$reversedArray = [array]::Reverse($array)
$reversedArray
This will output: `5, 4, 3, 2, 1`
Understanding Arrays in PowerShell
What is an Array?
In PowerShell, an array is a collection of items stored in a single variable. Arrays allow you to group related data together, making it easier to manage and access. This is particularly important in scripting and automation tasks, where you often work with multiple data points at once.
Creating Arrays
To create an array in PowerShell, you use the `@()` syntax. Here's a simple example:
$myArray = @('Apple', 'Banana', 'Cherry')
In this example, `@()` defines the array, and the elements `'Apple'`, `'Banana'`, and `'Cherry'` are assigned as its contents. Arrays can hold any type of data, including numbers, strings, or even other arrays.
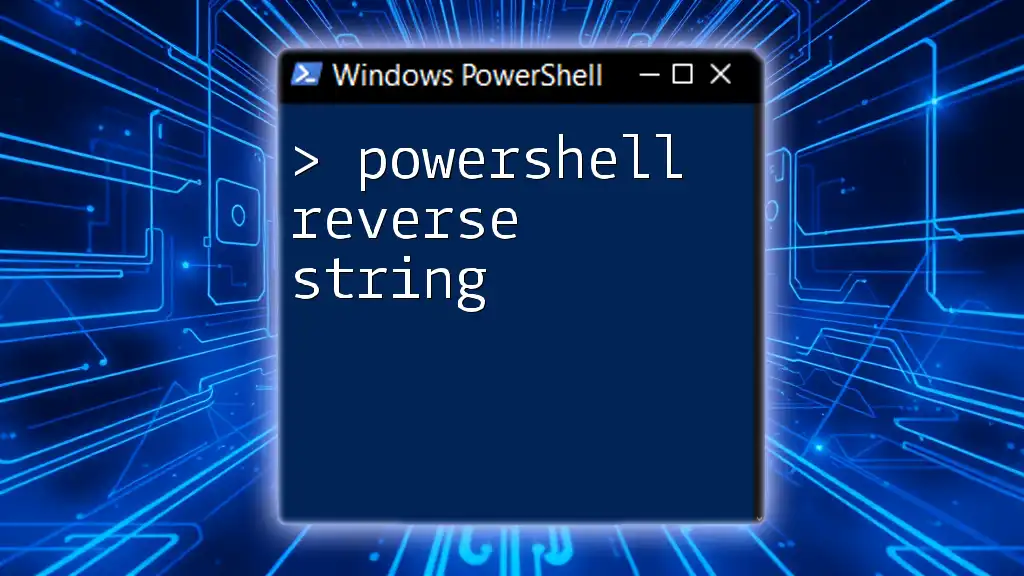
The Concept of Reversing an Array
What Does Reversing an Array Mean?
Reversing an array means altering its order so that the first element becomes the last and the last element becomes the first. For instance, if you have an array like `@('Apple', 'Banana', 'Cherry')`, reversing it would result in `@('Cherry', 'Banana', 'Apple')`. This concept is particularly useful in various data processing scenarios, such as when you need to display data in a different order or when time-based metrics need to be reported from the most recent to the oldest.
Benefits of Reversing an Array
Understanding how to reverse an array can enhance your data manipulation skills. Reversing can:
- Facilitate data output in the desired order, crucial in report generation.
- Enhance readability by presenting data from the latest to the earliest, especially in log files or time-series data.
- Simplify complex transformations and algorithms by allowing for backward iterations.
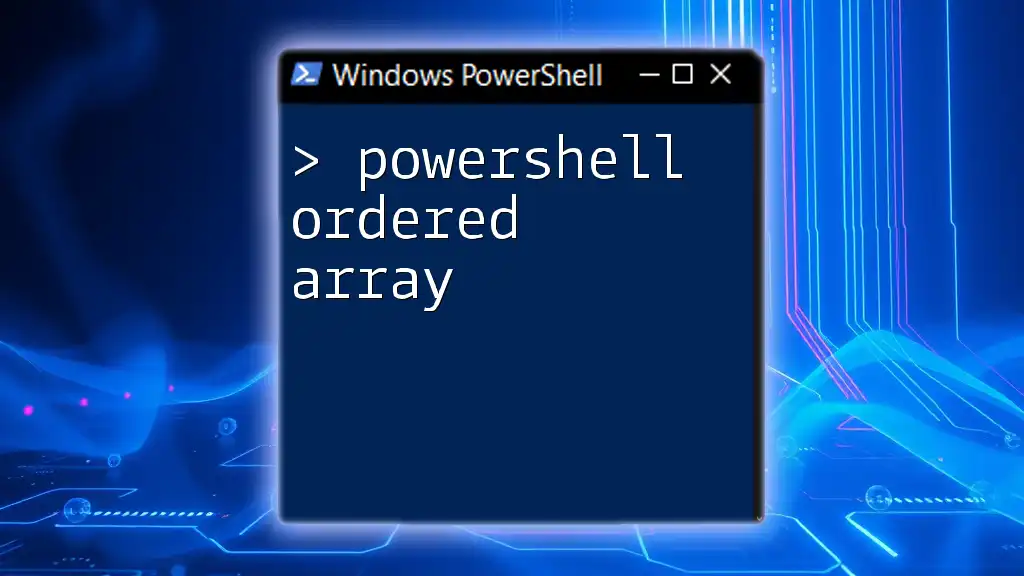
Methods to Reverse an Array in PowerShell
Using the `Reverse()` Method
One of the most straightforward ways to reverse an array in PowerShell is by using the `.NET` method `[array]::Reverse()`. This method modifies the original array in place, making it simple and efficient. Here's how you can do it:
$myArray = @('Apple', 'Banana', 'Cherry')
[array]::Reverse($myArray)
After running this code, `$myArray` will contain:
Cherry
Banana
Apple
The `Reverse()` method is quick and does not require additional variables or complex logic, making it a popular choice among PowerShell scripters.
Using Array Indexing
If you prefer a more hands-on approach, you can manually reverse an array using indexing. This method gives you complete control over the reversal process. Here’s a step-by-step guide on how to implement it:
$originalArray = @('Apple', 'Banana', 'Cherry')
$reversedArray = @()
for ($i = $originalArray.Length - 1; $i -ge 0; $i--) {
$reversedArray += $originalArray[$i]
}
In this example, we create an empty array called `$reversedArray` and loop through `$originalArray` from the last index to the first. Each element is appended to `$reversedArray`, resulting in:
Cherry
Banana
Apple
This method is particularly useful if you want to apply additional transformations while reversing the array.
Using PowerShell Pipeline
Using the PowerShell pipeline to reverse an array is another elegant solution. This method showcases PowerShell's ability to handle data streams smoothly. Here’s how it can be accomplished:
$myArray = @('Apple', 'Banana', 'Cherry') | ForEach-Object { $_ } | Sort-Object { $myArray.Count - $_.IndexOf($_) }
In this snippet, we utilize the pipeline to process each item in the array, allowing the contents to be manipulated as they flow through the different commands. This method can enhance readability and demonstrates PowerShell's versatility.
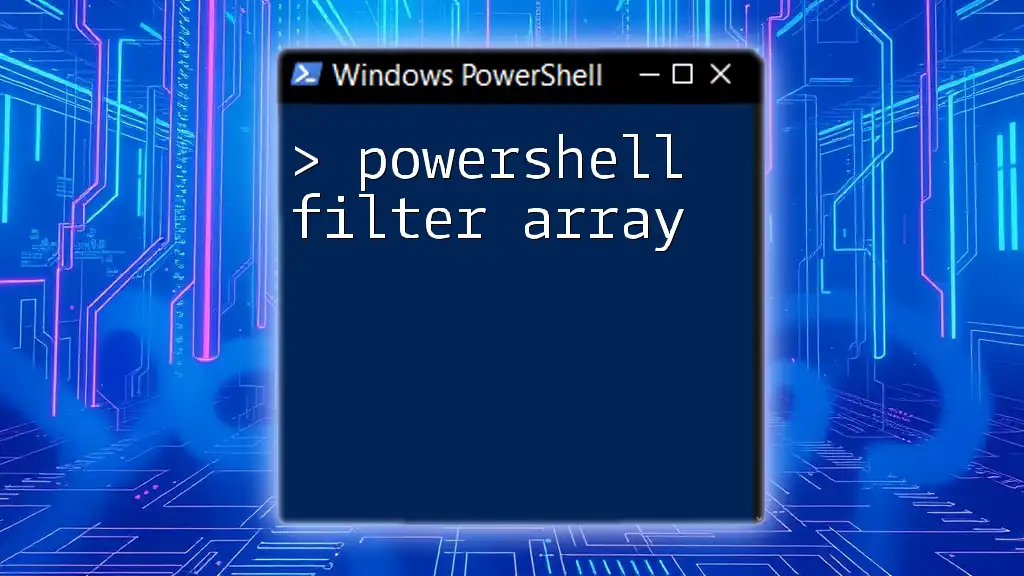
Practical Applications of Reversing an Array
Example Use Cases
Reversing an array can be beneficial in several scenarios:
- Data Formatting: When exporting data for reports, reversing an array allows you to present entries in a more user-friendly order.
- Backup Procedures: You can reverse data collections that require processing from the newest entry to the oldest.
Real-World Situations
Consider a scenario where you’re analyzing log files. Often, logs are generated chronologically; however, you might need to inspect the most recent entries first. Reversing the array allows you to view the relevant information straight away, streamlining your workflow.
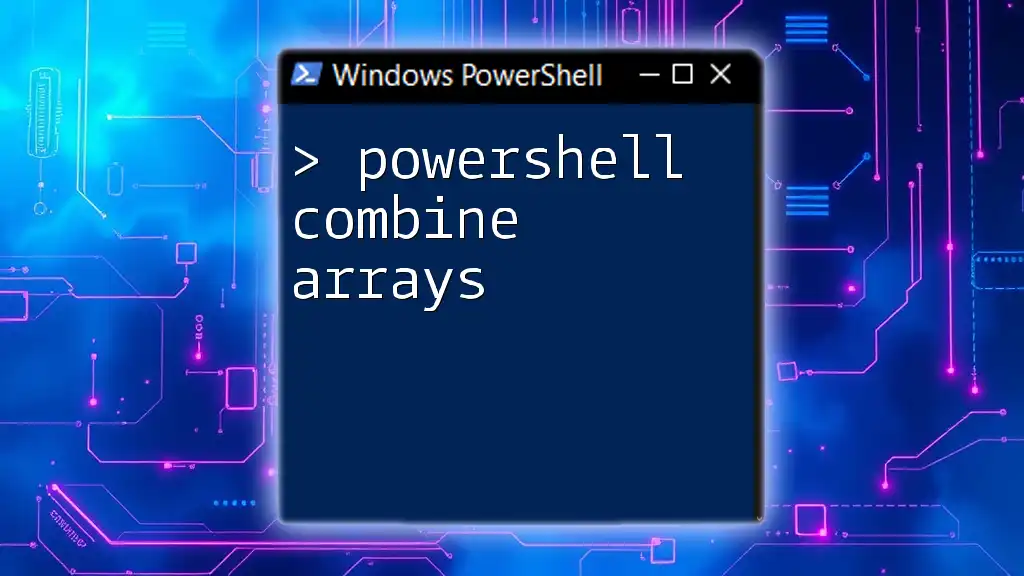
Common Pitfalls and Troubleshooting
What to Watch Out For When Reversing Arrays
While reversing arrays is generally straightforward, there are a few common pitfalls to be aware of:
- Immutable Behavior: Methods that return a new array without altering the original can be confusing. Familiarize yourself with whether a method modifies the array in place or creates a new one.
- Index Errors: When implementing manual reversal using indexing, ensure that your loops reference the correct indices to avoid out-of-bounds errors.
Tips for Troubleshooting Common Errors
If you encounter issues while reversing arrays:
- Validate Input: Make sure the input is indeed an array and not `null` or another data type.
- Error Messages: Pay close attention to error messages in PowerShell; they often provide clues on what went wrong.
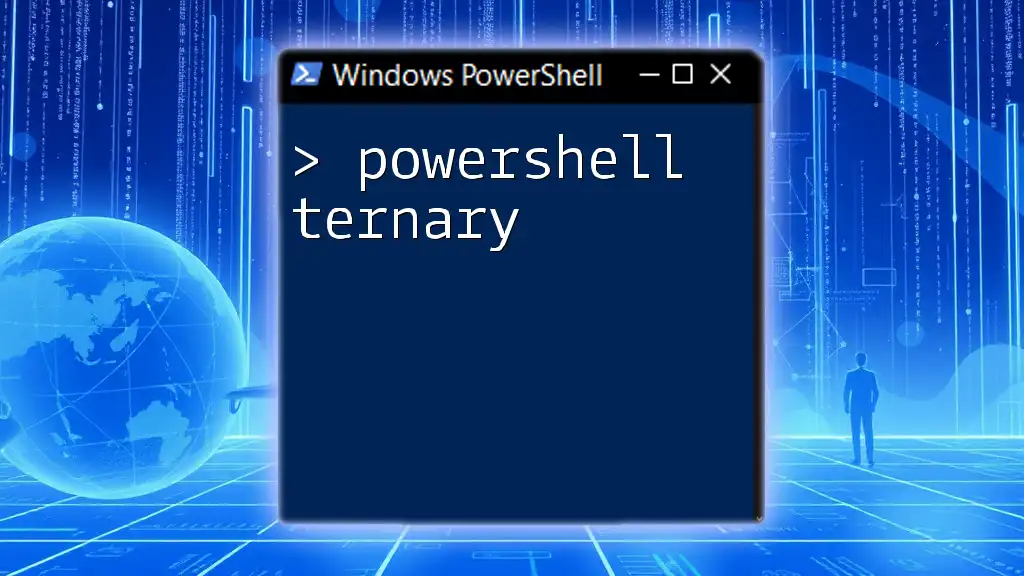
Conclusion
Understanding how to effectively reverse arrays in PowerShell enhances your scripting capabilities. It allows for better data management and manipulation, leading to improved productivity. By mastering techniques like using the `Reverse()` method, array indexing, and the PowerShell pipeline, you empower yourself to handle data more effectively and creatively.
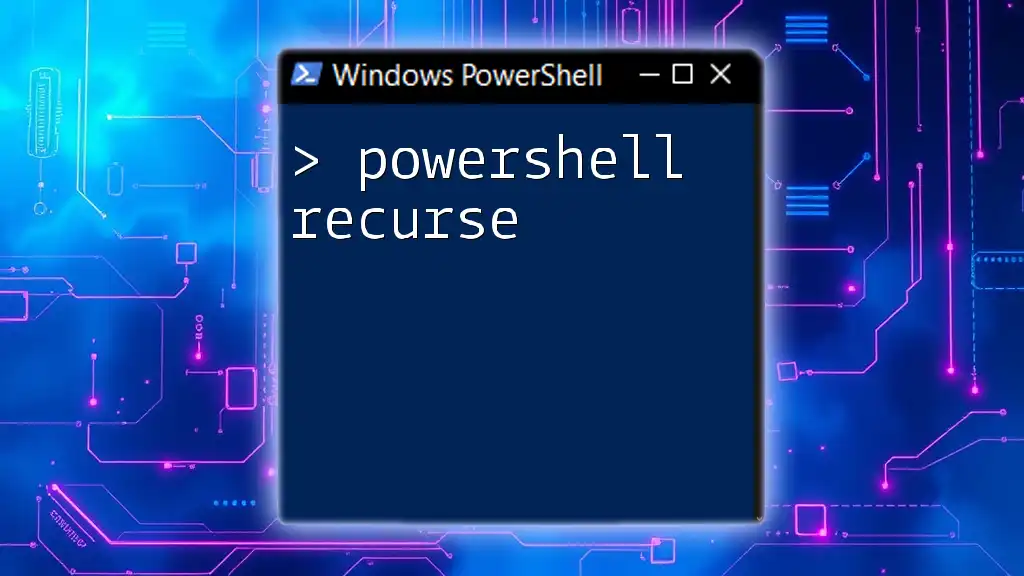
Resources and Further Reading
To deepen your understanding of PowerShell arrays and further enhance your skills, consider exploring:
- The official PowerShell documentation for arrays and collections.
- Online tutorials focused on data manipulation in PowerShell.
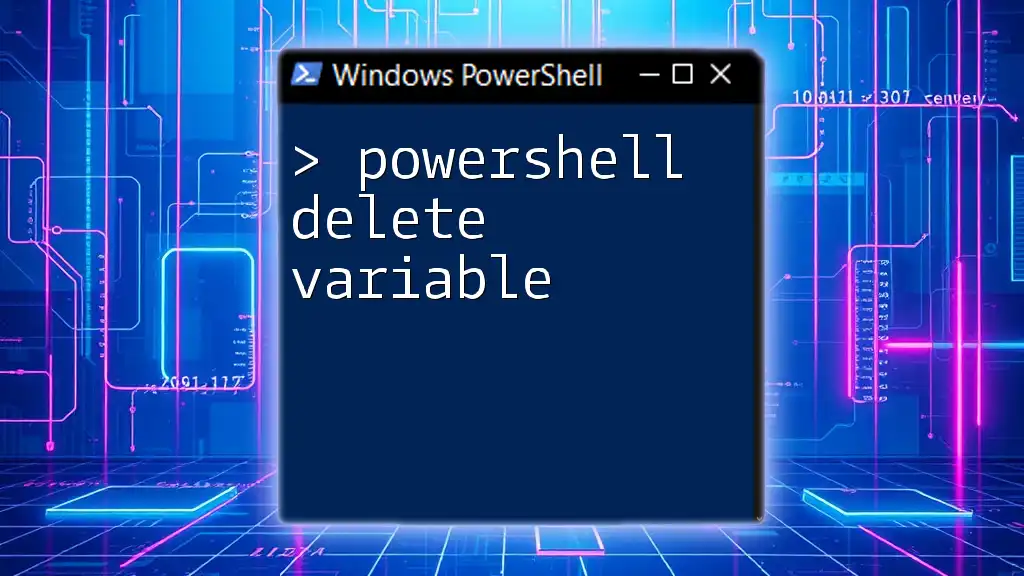
Call to Action
We’d love to hear your experiences using PowerShell arrays! Share your insights or questions in the comments below. Explore our courses and materials to deepen your knowledge and gain hands-on experience in PowerShell.