An ordered array in PowerShell is a collection that maintains the order of elements as they are added, allowing for indexed access to items based on their position in the collection.
Here's a code snippet demonstrating how to create an ordered array in PowerShell:
$orderedArray = [ordered]@{
'One' = 1
'Two' = 2
'Three' = 3
}
$orderedArray
Understanding PowerShell Arrays
What is an Array?
An array is a data structure that can hold multiple values in a single variable. It organizes data in a manner that allows easy access and manipulation. Arrays are used extensively in programming for tasks such as storing collections of items and managing data efficiently. The advantages of using arrays include constant time complexity for access and the ability to store a vast amount of data under a single variable name.
Types of Arrays in PowerShell
PowerShell supports various types of arrays, but primarily we work with:
- Standard Arrays: These are traditional arrays that allow you to store indexed collections of items.
- Ordered Arrays: A specialized type of collection that maintains the order of elements based on the sequence they are added.
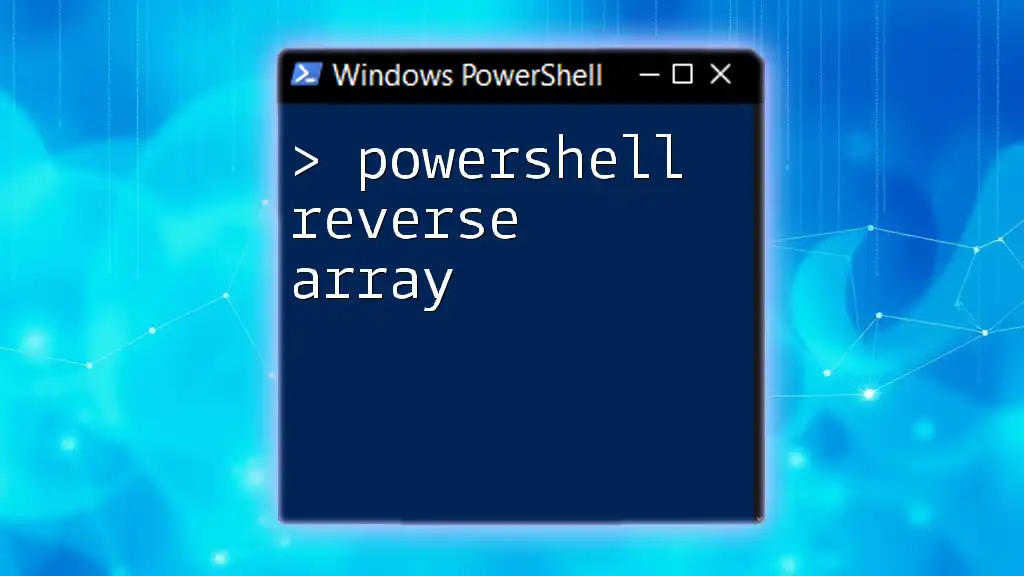
What is an Ordered Array in PowerShell?
Definition and Characteristics
An ordered array in PowerShell is a hash table that preserves the order of its keys. This means that when you retrieve items from an ordered array, you access them in the same order they were added. This is a significant feature for scenarios where maintaining sequence is crucial.
When to Use Ordered Arrays
You should consider using ordered arrays in scenarios where the order in which elements are entered is significant. For instance, if you are storing configuration data, user inputs, or any entities where the chronological order is critical, ordered arrays will be advantageous. They enhance clarity and coherence in scripts, contributing to easier debugging and understanding of data flow.
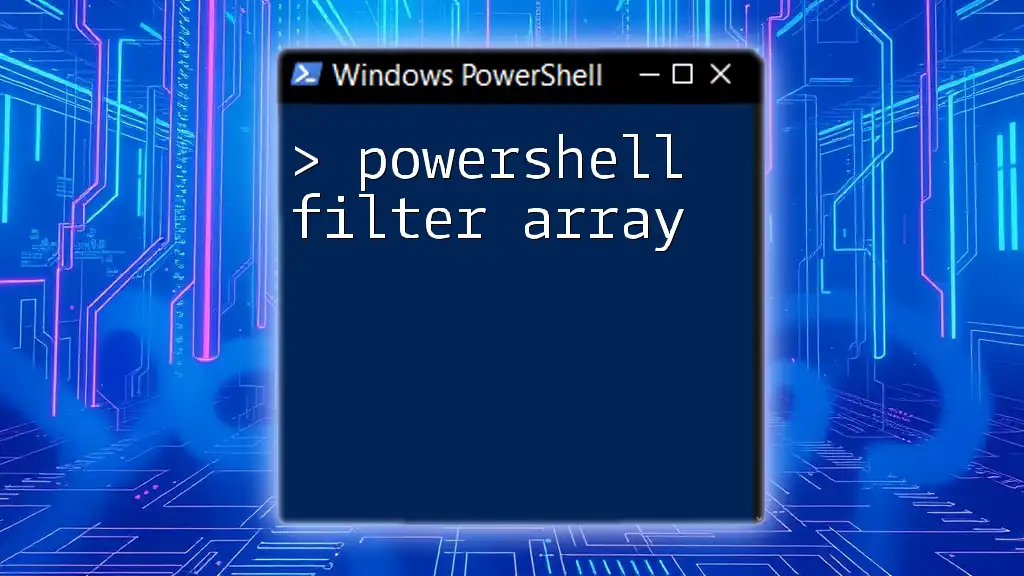
Creating Ordered Arrays in PowerShell
Syntax Overview
Creating an ordered array in PowerShell involves using the `[ordered]` attribute in conjunction with a hash table. The syntax is simple, allowing easy implementation.
Example:
To create an ordered array, you can use the following code:
$orderedArray = [ordered]@{
Name = 'John'
Age = 30
Country = 'USA'
}
Adding Items to an Ordered Array
Once you've created an ordered array, you can easily add or modify elements. Adding new elements doesn't disrupt the order of existing keys.
Example:
To add a new item representing occupation to the ordered array:
$orderedArray['Occupation'] = 'Developer'
Accessing Elements in Ordered Arrays
Accessing elements from an ordered array is straightforward. You can retrieve items directly by referencing their keys.
Example:
To fetch the occupation you recently added, you would use:
$occup = $orderedArray['Occupation']
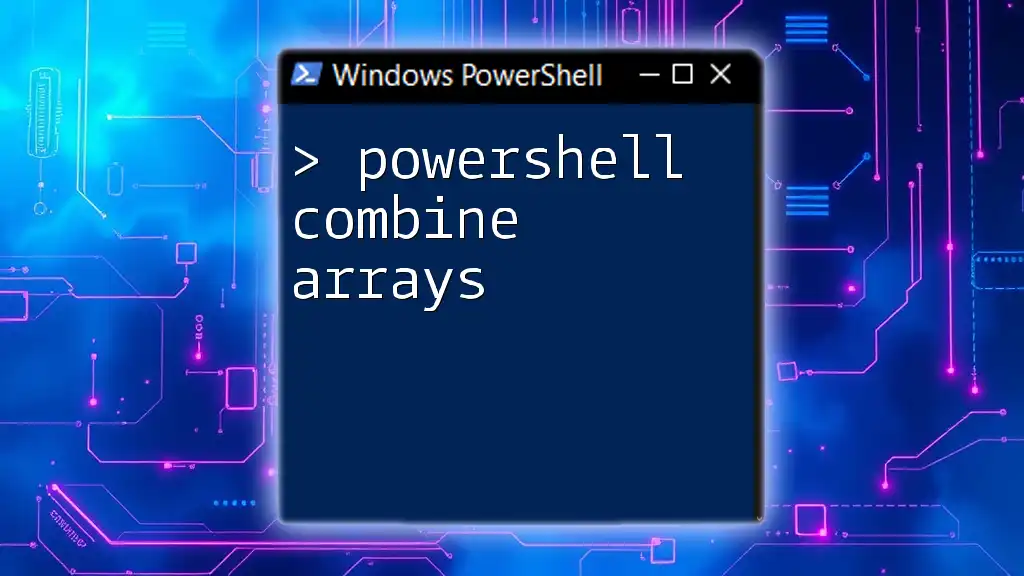
Manipulating Ordered Arrays
Iterating Through an Ordered Array
Iterating through an ordered array can be accomplished using a loop. This enables you to perform operations on each element sequentially.
Example: Using `foreach`
Here’s how you can iterate through the keys in the ordered array:
foreach ($key in $orderedArray.Keys) {
Write-Output "$key: $($orderedArray[$key])"
}
Sorting Ordered Arrays
While ordered arrays maintain the initial insertion order, there are situations when you might want specific ordering based on certain attributes or values.
Example:
If you have keys that need sorting, you can achieve this as follows:
$orderedArray = [ordered]@{
b = 2
a = 1
c = 3
}
$sortedArray = $orderedArray.GetEnumerator() | Sort-Object Name
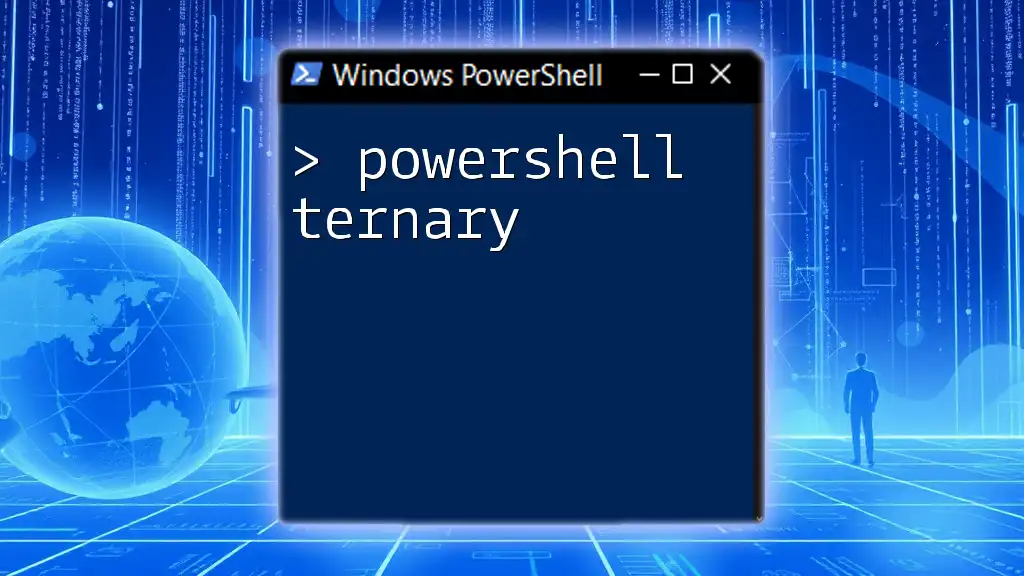
Differences Between Ordered and Non-Ordered Arrays
Performance Implications
In terms of performance, ordered arrays can provide better management for scenarios that require sequential access. Their ability to maintain order typically results in higher efficiency when you need to retrieve elements in the same order they were added.
Data Integrity
Ordered arrays help preserve data integrity since the order of insertion matters and is reflected when you retrieve the data.
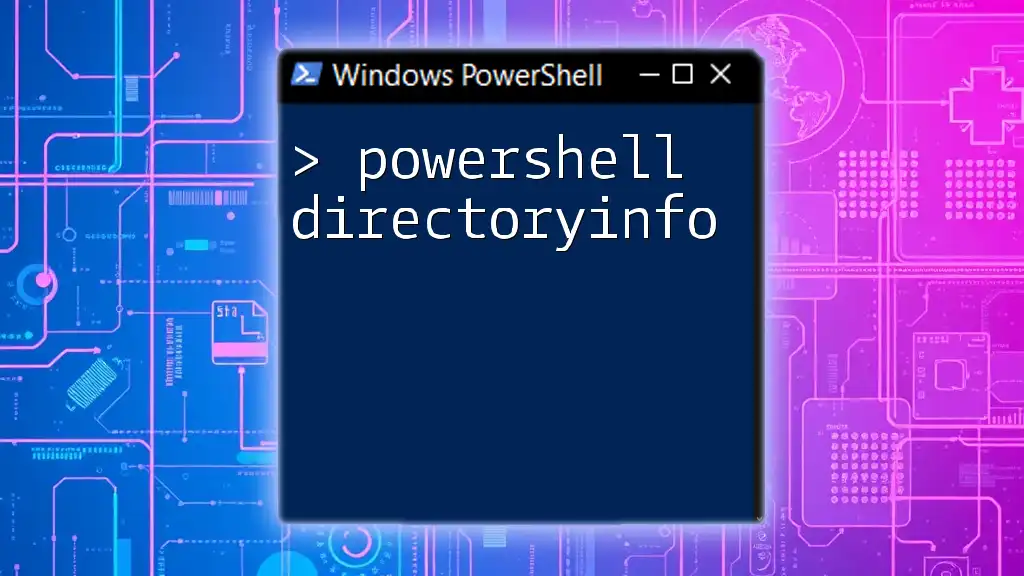
Best Practices for Using Ordered Arrays
When to Prefer Ordered Arrays Over Hash Tables
If you frequently require the order of items to remain intact, ordered arrays should be your data structure of choice over standard hash tables. Hash tables do not guarantee any particular order of items.
Tips for Managing Large Ordered Arrays
When handling large collections in ordered arrays, consider ways to efficiently manipulate your data. It may be beneficial to regularly check for duplicates, optimize access routes to the elements, and utilize appropriate looping constructs that minimize resource consumption.
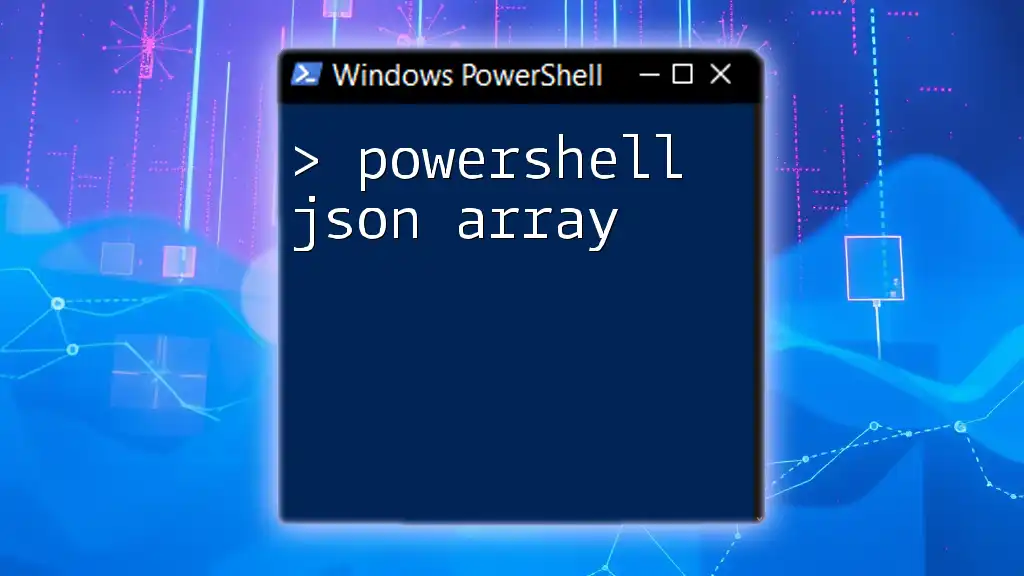
Common Pitfalls and Troubleshooting
Errors in Creating or Manipulating Ordered Arrays
One common mistake is forgetting to use the `[ordered]` attribute when declaring an ordered array. This will lead to standard unordered behavior. Always ensure you verify the type at the beginning to avoid potential issues down the road.
If you encounter unexpected results, check your element definitions for typos, and ensure that your keys are unique, as duplicate keys will overwrite previous entries.
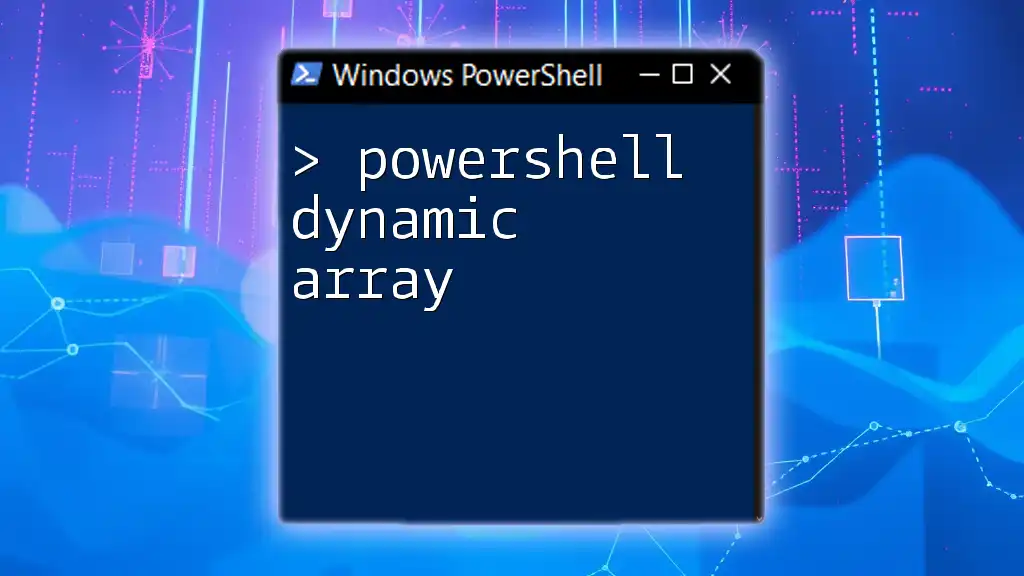
Conclusion
In summary, PowerShell ordered arrays provide a flexible and efficient way to maintain and manipulate collections of data where order matters. Their easy syntax and functionality make them an essential tool in the PowerShell scripting arsenal. Embrace mastering ordered arrays to enhance your scripting solutions, and don’t hesitate to experiment with various commands and examples to deepen your understanding.
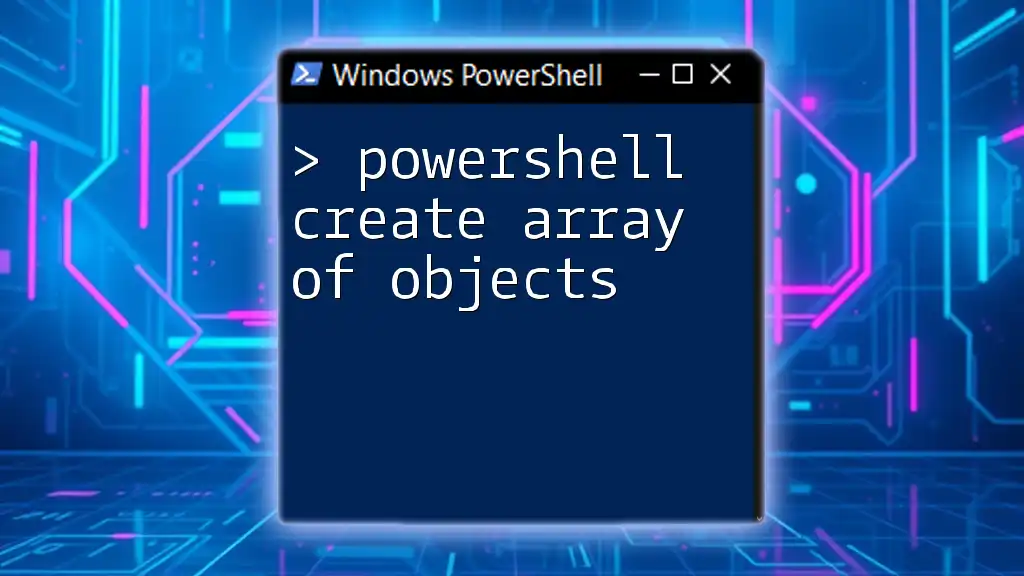
Additional Resources
To further explore and expand your knowledge on ordered arrays, refer to the official Microsoft PowerShell documentation. Participating in PowerShell forums and exploring tutorials can provide practical insights and community support as you refine your skills.