In PowerShell, you can create a variable by prefixing a name with a dollar sign ($) and assigning a value using the equals sign (=).
$greeting = 'Hello, World!'
Understanding Variables in PowerShell
What is a Variable?
In PowerShell, a variable is a data structure used to store information. This information can be anything from numbers and strings to arrays and even complex objects. Variables allow you to temporarily retain data during the execution of a script, making them fundamental for automation and dynamic data manipulation.
Types of Variables
PowerShell supports various data types. Each type serves different purposes, which include:
- String: Used for text.
- Integer: For whole numbers.
- Boolean: Represents true or false values.
- Array: A collection of items stored in a single variable.
- HashTable: A collection of key-value pairs for storing data more flexibly.
Understanding the differences between these types allows you to choose the most suitable variable type for your needs.
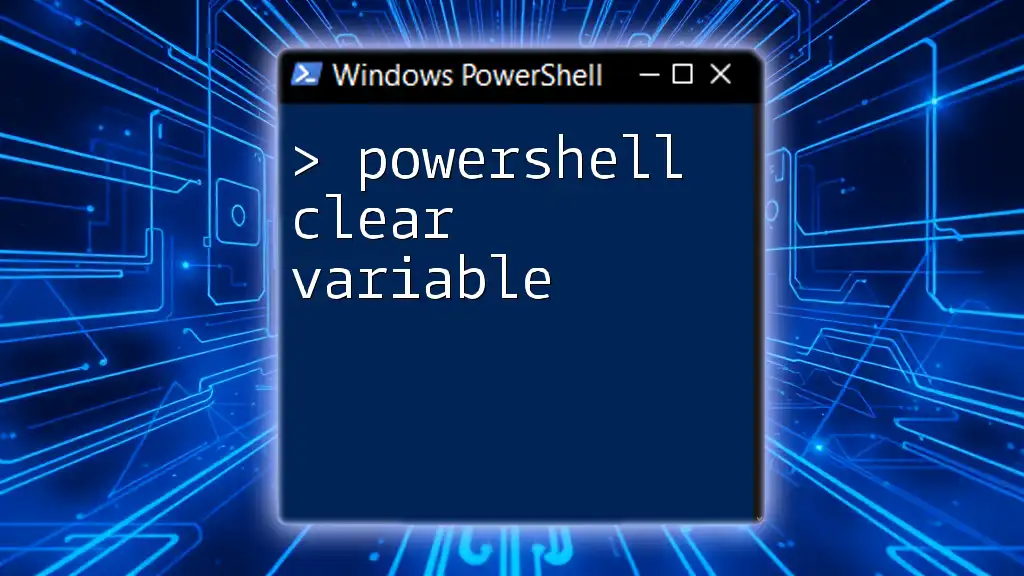
Declaring a Variable in PowerShell
Syntax for Declaring Variables
To create a variable in PowerShell, you use the dollar sign ($) followed by the variable name, an equal sign, and then the value you want to assign. The general syntax is:
$variableName = value
For example, if you want to store a name, you could do it as follows:
$name = "John Doe"
Best Practices for Variable Naming
When declaring variables, it's essential to follow best practices for naming:
- Avoid spaces and special characters: Use underscores or camel case (e.g., `$firstName`, `$last_name`).
- Descriptive names: Choose names that clearly describe the variable's purpose (e.g., `$userCount` instead of `$x`).
Good variable names improve the readability of your scripts and help others understand your code at a glance.
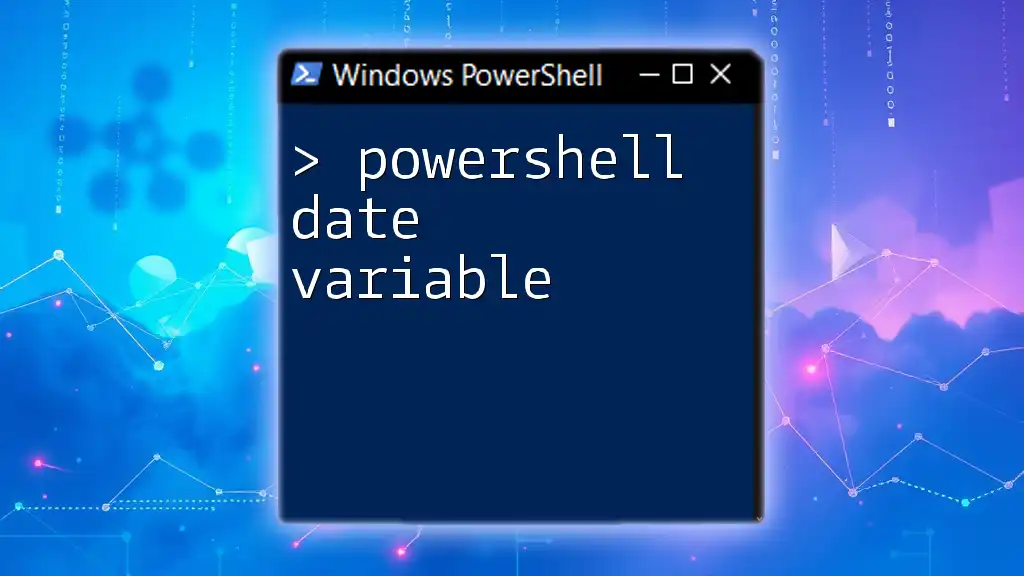
PowerShell Assign Variable
Assigning Values to Variables
You can assign various types of values to variables in PowerShell, whether they are simple strings, integers, or complex structures. For example:
$age = 30
$hobbies = @("Reading", "Traveling", "Gaming")
This gives `$age` a numeric value while `$hobbies` holds an array of strings.
Reassigning Variables
Once a variable is declared, you can change its value at any time. For example, if you initially set:
$age = 30
You can later change it to:
$age = 31 # Reassigning the age
This ability to reassign makes variables flexible and adaptable as your scripts run.
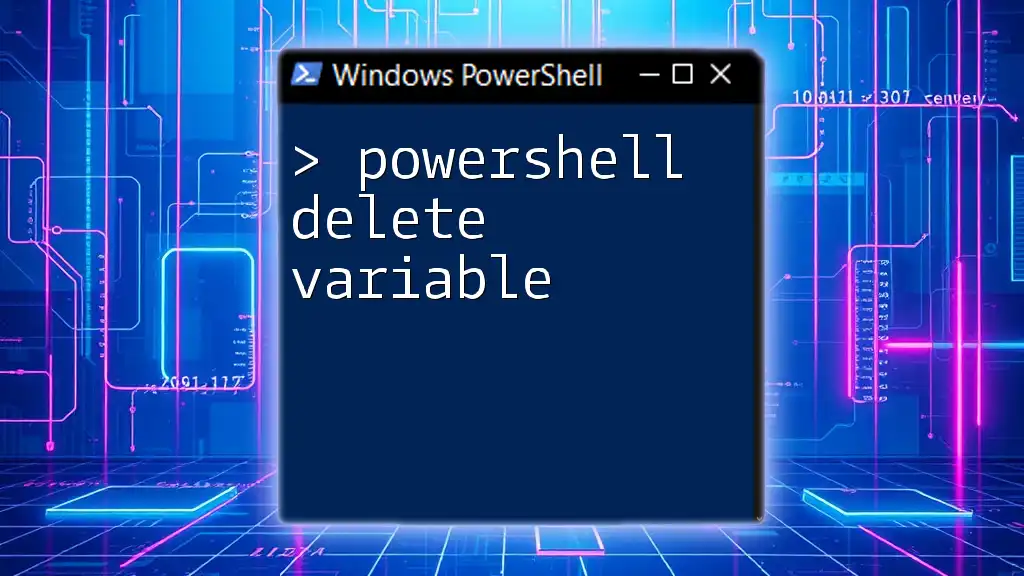
Declaring Variables in PowerShell
Using Different Data Structures
PowerShell allows you to leverage different data structures when creating variables, enhancing the way you can organize and manipulate your data. Two common structures are arrays and hashtables.
Array Example: An array can be declared and initialized as follows:
$numbers = @(1, 2, 3, 4)
Hashtable Example: A hashtable, which is more structured, can be created like this:
$person = @{ Name = "John Doe"; Age = 30 }
Using these structures lets you group related data effectively.
Scoped Variables
One of the more advanced aspects of PowerShell is managing the scope of your variables. There are three primary types of scopes you will encounter:
- Local: Accessible only in the current script or block.
- Global: Accessible from anywhere in the script session.
- Script: Accessible only within the script file itself.
For example, to create a globally accessible variable, you can use the following syntax:
$global:myVar = "I am global!"
Understanding variable scopes is crucial for avoiding naming conflicts and managing data visibility correctly.
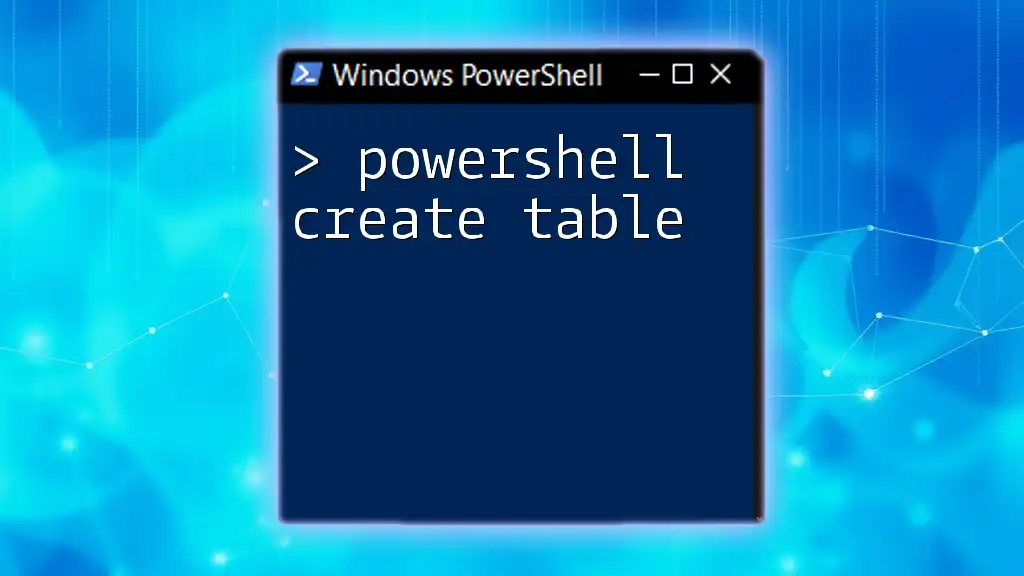
Working with Variable Content
Accessing Variables
Retrieving the contents of a variable in PowerShell is straightforward. Simply use the variable name, prefixed by a dollar sign. For instance, to output the value of a variable, you can do it like this:
Write-Output $name # Output: John Doe
Outputting Variables
There are several methods to display variable values, including `Write-Host` and `Write-Output`. It's important to know when to use each. `Write-Host` directly communicates to the console, while `Write-Output` sends the data down the output pipeline, which can be useful in more complex scripts.
For example:
Write-Host "Hello, $name!" # Displays: Hello, John Doe!
Write-Output "Your age is $age." # Sends output down the pipeline
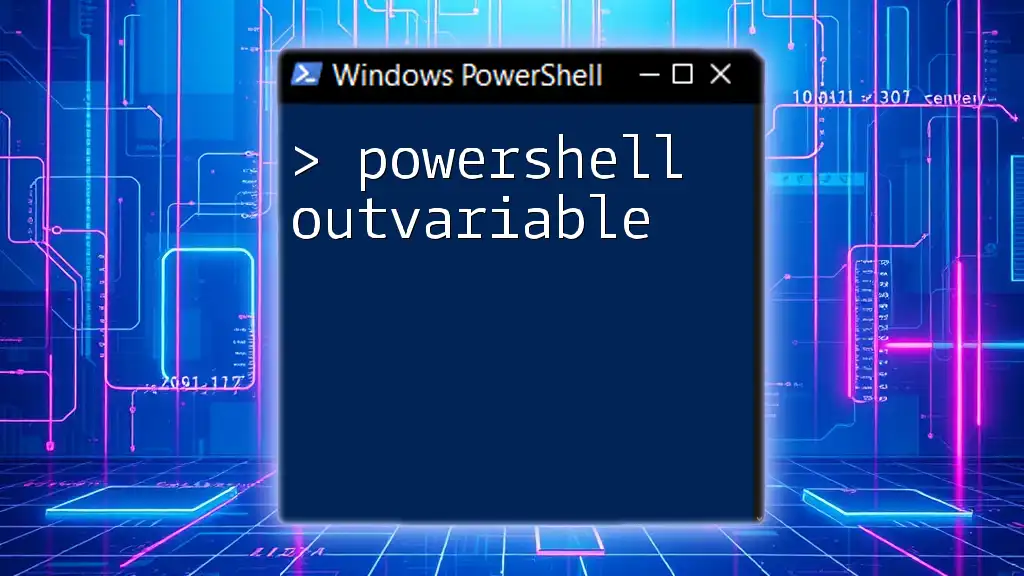
Common Errors and Troubleshooting
Error Messages Related to Variables
While working with variables, you might encounter errors, especially if a variable hasn't been initialized. For example, attempting to access a variable that is `$null` can lead to issues.
To avoid such scenarios, always check if a variable is initialized before using it:
if ($null -eq $myVar) {
Write-Host "myVar is not initialized"
}
Additionally, make use of `Get-Type` to verify what type of data your variable holds, which helps in debugging.
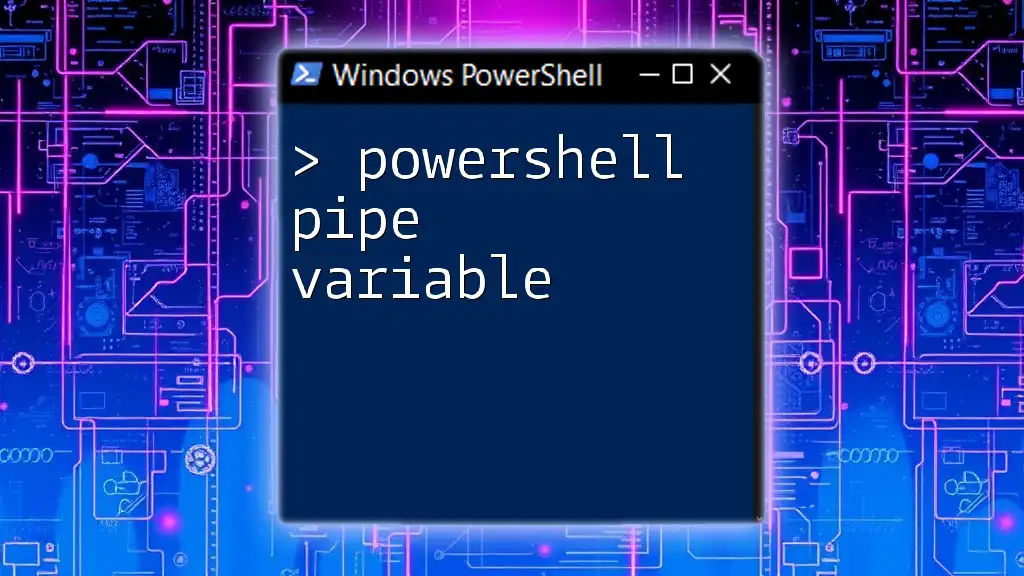
Conclusion
In summary, understanding how to create variables in PowerShell is crucial for effective scripting and automation. Variables help in storing temporary data and allow for dynamic manipulation of information. By practicing how to declare, assign, and manage variables, you enhance your PowerShell proficiency, making script execution smoother and more efficient.
Remember to adhere to best practices, experiment with different data types and structures, and utilize scopes strategically. As you continue your PowerShell journey, you'll find that mastering variable usage opens the door to more complex and efficient scripting capabilities.
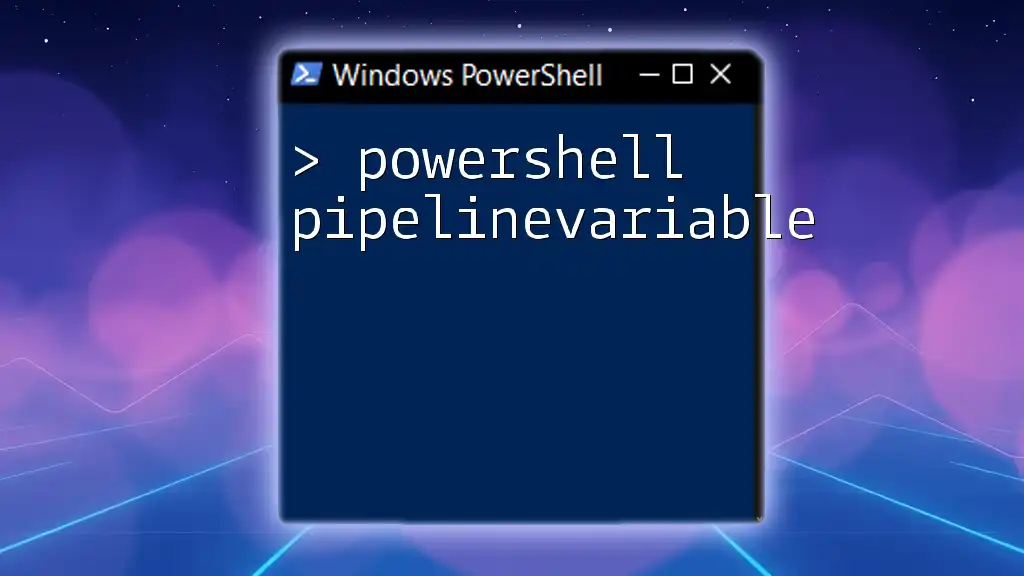
Additional Resources
For further learning, explore community forums, specialized books on PowerShell scripting, and engage with online courses that delve deeper into the nuances of PowerShell. If you're interested in personalized guidance, feel free to contact us!