In PowerShell, you can display the value of a variable using the `Write-Host` command to print output to the console.
Here's a code snippet that demonstrates how to echo a variable value:
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding PowerShell Variables
PowerShell variables are used to store data that can be referenced and manipulated throughout your script. To create a variable in PowerShell, you use the `$` symbol followed by the variable name. The syntax is simple:
$myVariable = "Hello, World!"
Types of Variables
PowerShell supports several types of variables, including:
- String Variables: Used to store text data. Example: `"$name"`
- Array Variables: A collection of items stored in a single variable. Example: `@("Item1", "Item2")`
Understanding the type of variable you're working with is essential because it can influence how you echo the variable value.
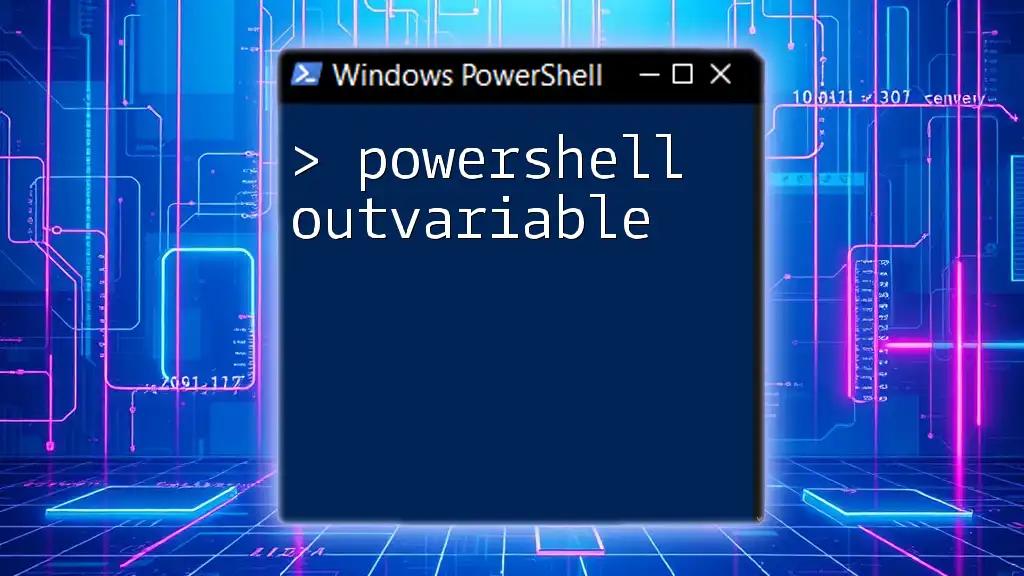
Using the Echo Command in PowerShell
The term echo in PowerShell refers to outputting the value of a variable to the screen. It's essential to understand that echo doesn't have a dedicated command in PowerShell. Instead, there are multiple cmdlets that serve the purpose of displaying variable content.
What Does Echo Mean in PowerShell?
In standard terms, echoing a variable means displaying its contents. However, PowerShell uses `Write-Host`, `Write-Output`, and even the built-in output behavior for this purpose. This multiplicity can confuse newcomers, but each method has its context and use.
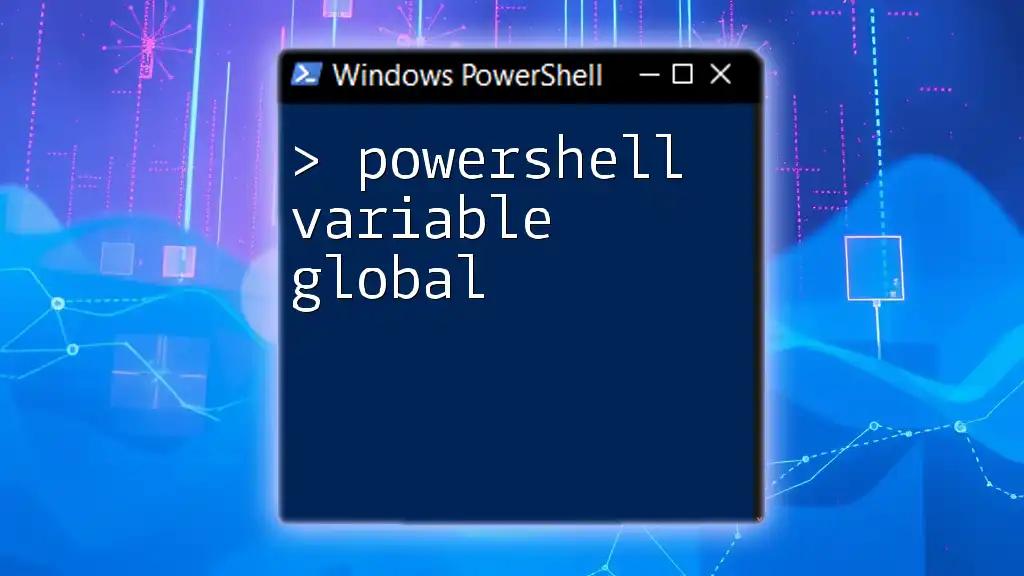
Echoing Variable Values in PowerShell
Basic Echoing of a Variable
One of the most common methods to echo a variable in PowerShell is using `Write-Host`. This cmdlet outputs the value directly to the console.
Example:
$myVariable = "Hello, World!"
Write-Host $myVariable
In this example, the string "Hello, World!" is displayed in the console.
Alternative Methods
Using Write-Output
Another method for echoing variable values is `Write-Output`. Unlike `Write-Host`, which directly writes to the console, `Write-Output` sends the data "down the pipeline", which can be useful if you are chaining commands.
Example:
Write-Output $myVariable
When executed, this will produce the same output as before, but the data can now be further processed if needed.
Using the Built-in Output Behavior
PowerShell has an implicit output behavior. If you simply type the variable name in the console or script and do not use any cmdlet, PowerShell automatically outputs the value.
Example:
$myVariable
In this case, the contents of `$myVariable` will be echoed without any cmdlet, utilizing PowerShell's ability to recognize the last expression.
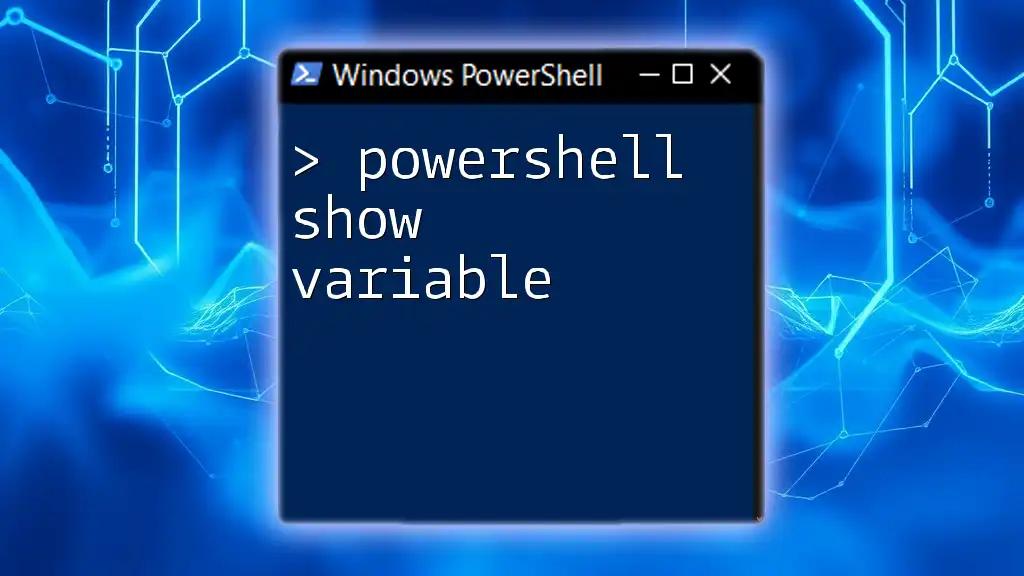
Practical Examples
Example 1: Echo a Simple String Variable
If you want to echo a simple string variable, `Write-Host` is a straightforward choice.
Code Snippet:
$greeting = "Hello, PowerShell!"
Write-Host $greeting
This will display "Hello, PowerShell!" on the console.
Example 2: Echo a Variable Within a String
PowerShell allows you to include variables within strings, giving you flexibility when you want to compose messages.
Code Snippet:
$name = "John"
Write-Host "Hello, $name!"
This will output "Hello, John!" by interpolating the variable `$name` into the string.
Example 3: Echoing Multiple Variables
Echoing multiple variables at once can be done seamlessly.
Code Snippet:
$firstName = "Jane"
$lastName = "Doe"
Write-Host "$firstName $lastName"
This will result in "Jane Doe" being displayed on the console.

Best Practices for Echoing Variables
When to Use Write-Host vs. Write-Output: It’s important to choose the right cmdlet based on your needs. Use `Write-Host` when you want to display messages that don't require further processing. On the other hand, opt for `Write-Output` when you plan to use the output in a pipeline, allowing for greater flexibility in your scripts.
Debugging with Echo
Echoing variable values is a powerful debugging technique. When scripts don’t behave as expected, inserting `Write-Host` or `Write-Output` statements can provide insight into the current state of your variables, helping you diagnose issues quickly.
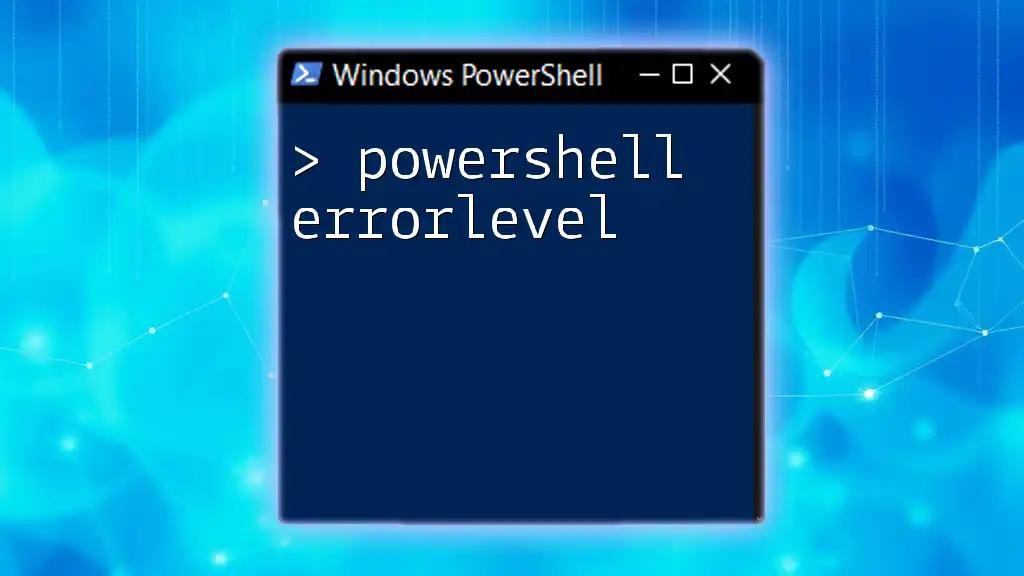
Troubleshooting Common Issues
Common Errors When Echoing Variables
One common mistake is trying to echo a variable that hasn’t been initialized. Another frequent mishap is mixing up `Write-Host` and `Write-Output`, which can lead to unexpected results depending on the context.
Solutions and Tips
To troubleshoot issues with echoing variables:
- Always ensure your variable is initialized before attempting to use or output it.
- If you’re using `Write-Host`, remember that this method doesn’t send output down the pipeline; it simply displays it in the console.
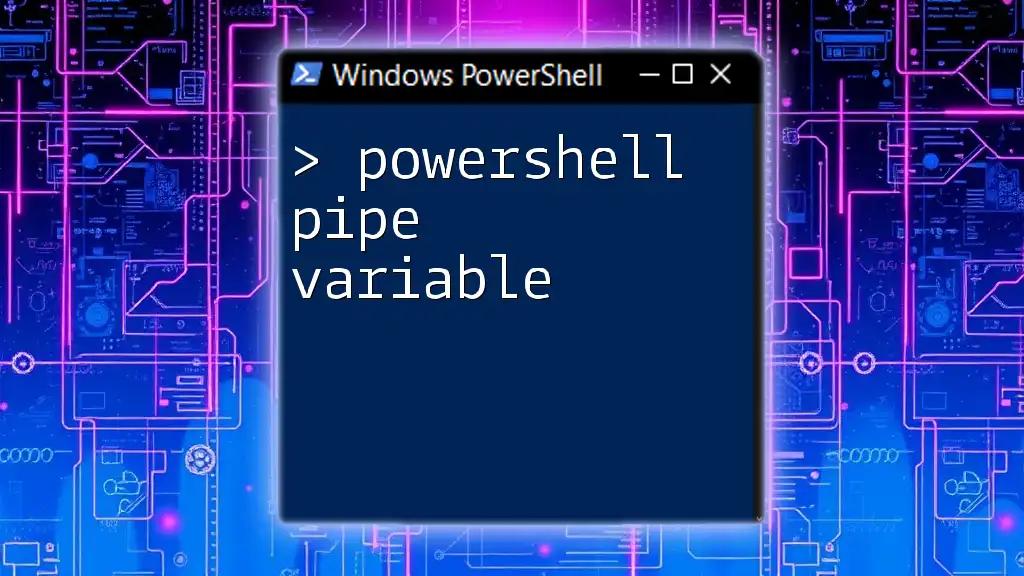
Conclusion
Echoing variable values in PowerShell is a fundamental skill that enhances your scripting abilities. Learning when and how to use different commands effectively can streamline your workflows and make debugging easier. With practice, you'll be able to implement these techniques in real-life scenarios confidently.
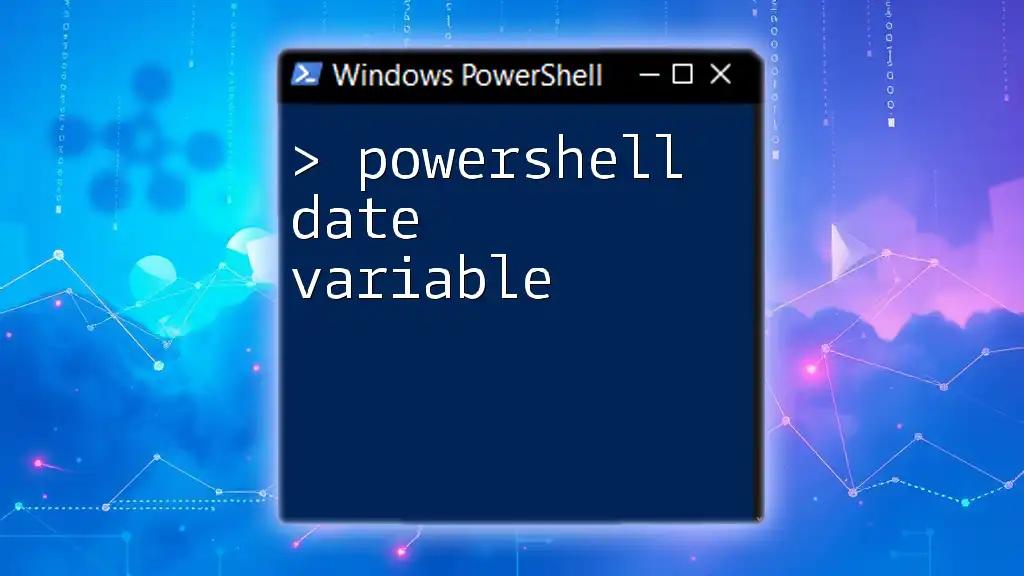
Call to Action
Now that you have a solid understanding of PowerShell echo variable value, it's time to put this knowledge into practice. Try writing your own scripts that incorporate variable echoing to see how it can improve your workflow. Don't forget to subscribe for more PowerShell tips and tricks!