In PowerShell, the absolute value of a number can be obtained using the `Math` class's `Abs()` method, which returns the non-negative value of a given number.
Here’s how you can use it in a code snippet:
$number = -42
$absoluteValue = [Math]::Abs($number)
Write-Host "The absolute value of $number is $absoluteValue"
Understanding Absolute Value
Definition of Absolute Value
At its core, the absolute value of a number refers to its non-negative magnitude, irrespective of its sign. In mathematical terms, it means that the absolute value of both `3` and `-3` is `3`. This concept is vital in programming because it allows programmers to handle numbers uniformly without worrying about their signs.
Why Use Absolute Value in PowerShell?
Using absolute value in PowerShell can enhance data processing capabilities. It plays a critical role in several applications, including:
- Data Manipulation: Often, data has fluctuations that lead to negative numbers. Using absolute values lets you focus on the magnitude of the data.
- Error Handling: In scripts where invalid inputs can arise, applying absolute value can streamline comparisons and validations, making your scripts more robust.
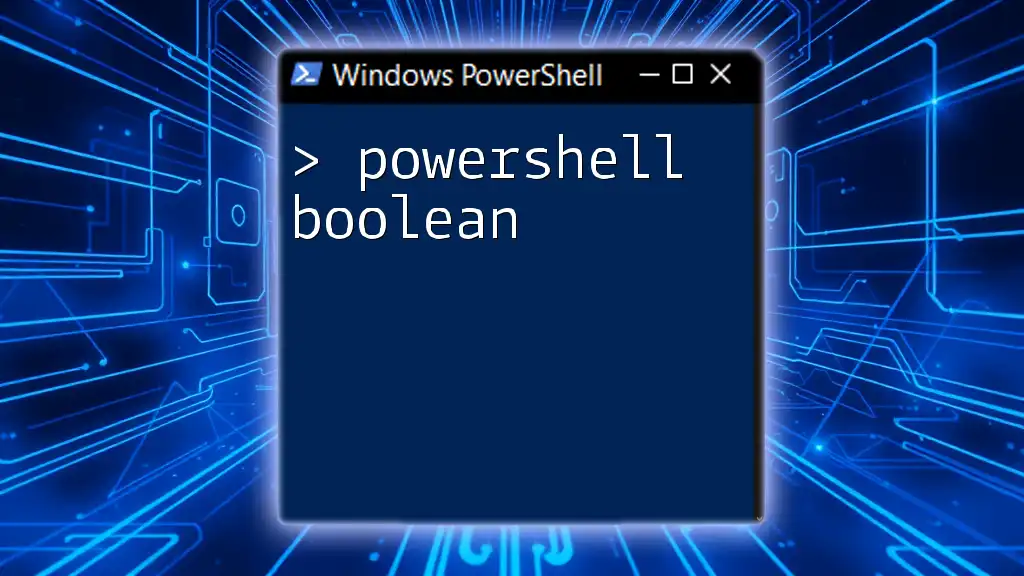
PowerShell Commands for Absolute Value
Using the Math Class
PowerShell, being built on the .NET framework, allows you to leverage the Math class for mathematical operations, including absolute values. The `Abs` method is straightforward to use.
Example Code Snippet:
$number = -42
$absoluteValue = [math]::Abs($number)
Write-Output $absoluteValue
In this example, we invoke the `Abs` method from the `Math` class to obtain the absolute value of `-42`. The output will be `42`. This method works seamlessly regardless of whether the input number is an integer, floating-point, or a variable holding these values.
Custom Function for Absolute Value
Creating a custom function in PowerShell adds reusability and clarity to your scripts. Below is a simple custom function that computes the absolute value.
Example Code Snippet:
function Get-AbsoluteValue {
param (
[double]$value
)
return [math]::Abs($value)
}
# Usage Example
Get-AbsoluteValue -value -15.7
In this snippet:
- We define a function `Get-AbsoluteValue` that takes a parameter `$value`.
- Inside the function, we return the absolute value using `[math]::Abs($value)`.
This approach not only encapsulates the logic but also enables you to perform further operations or enhancements.
Error Handling with Absolute Value
Robust scripts include error handling to deal with unexpected input. Below is an enhanced function that integrates input validation:
Example Code Snippet:
function Safe-AbsoluteValue {
param (
[Parameter(Mandatory=$true)]
[double]$value
)
if ($value -is [double]) {
return [math]::Abs($value)
} else {
throw "Input must be a numeric value."
}
}
# Usage Example
Safe-AbsoluteValue -value -20
In this function:
- The `Mandatory` attribute ensures the function cannot be called without a value.
- We check if the provided value is of type `double`. If not, we throw an error.
Using this method, we ensure that the script behaves predictably and that the input is validated, thereby enhancing the user experience.
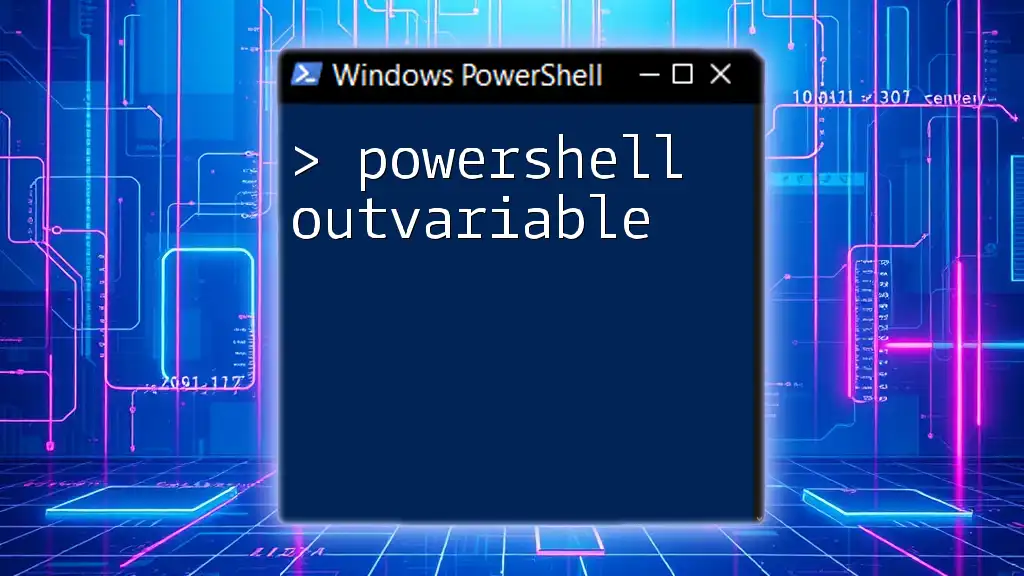
Practical Applications of Absolute Value in PowerShell
Use Cases in Data Analysis
In data analysis scenarios, absolute values can help manage datasets more effectively. For instance, when analyzing prices, absolute values can help determine variations regardless of direction.
Example Scenario: Analyzing price differences. Example Code Snippet:
$prices = @(10, -20, 30, -40)
$absolutePrices = $prices | ForEach-Object { [math]::Abs($_) }
# Output absolute values
$absolutePrices
Here, we loop through an array of prices and apply the `Abs` method, resulting in the output as follows: `10, 20, 30, 40`. This allows you to analyze the financial data without the bias introduced by negative values.
Comparisons and Conditional Logic
Absolute values also play a significant role in conditional checks. They can simplify logical conditions that involve comparing values against a threshold.
Example Scenario: Checking if values deviate under a threshold. Example Code Snippet:
$threshold = 15
$value = -18
if ([math]::Abs($value) -lt $threshold) {
Write-Output "Value is within the threshold."
} else {
Write-Output "Value exceeds the threshold."
}
In this example, the condition checks if the absolute value of `-18` is less than `15`. The output will correctly reflect that it exceeds the threshold, making absolute comparison straightforward.
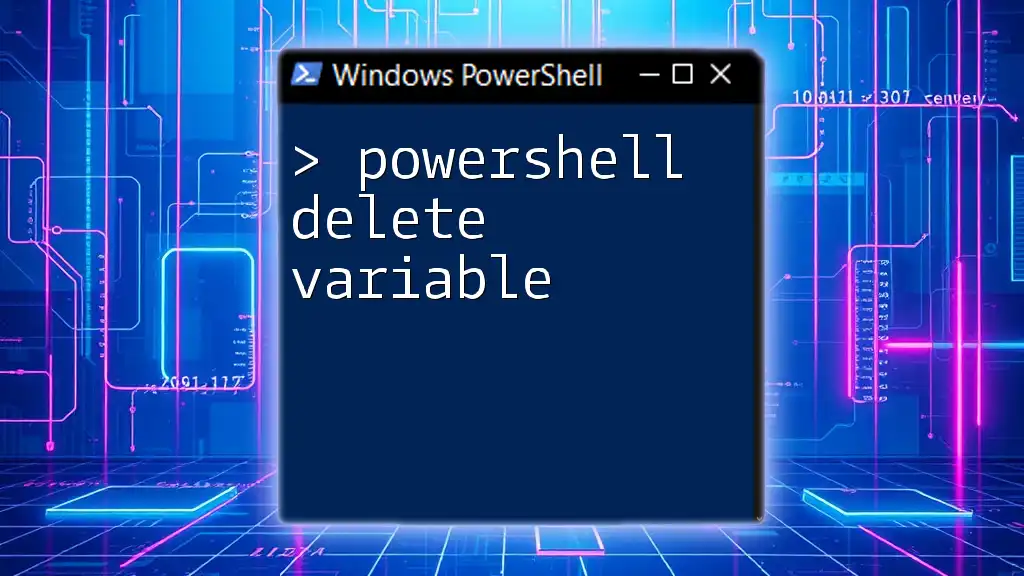
Tips for Using Absolute Value in PowerShell
Best Practices
- Consistency in Variable Naming: Maintain a clear naming convention that reflects the meaning of your variables for better readability and maintainability.
- Commenting for Clarity: Use comments to explain complex logic, making it easier for others (or yourself in the future) to understand the code.
Common Pitfalls to Avoid
- Forgetting to Validate Non-Numeric Input: Always ensure that your functions can only accept the correct data types to avoid runtime errors.
- Using Incorrect Data Types: Ensure you use appropriate types (like `double` for numeric calculations) to avoid unexpected behavior during operations.
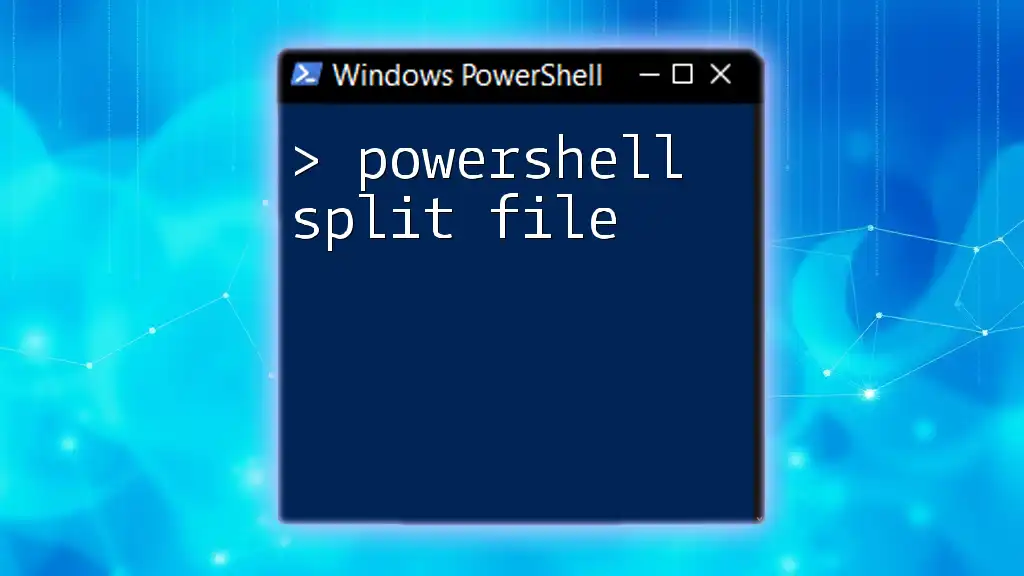
Conclusion
Understanding and using PowerShell absolute value helps streamline data manipulation and enhance logical evaluations within your scripts. By implementing the techniques discussed in this article, you can handle numerical data effectively, which is critical in various scripting tasks. Embrace these concepts and explore more PowerShell functionalities to become proficient in your scripting endeavors.
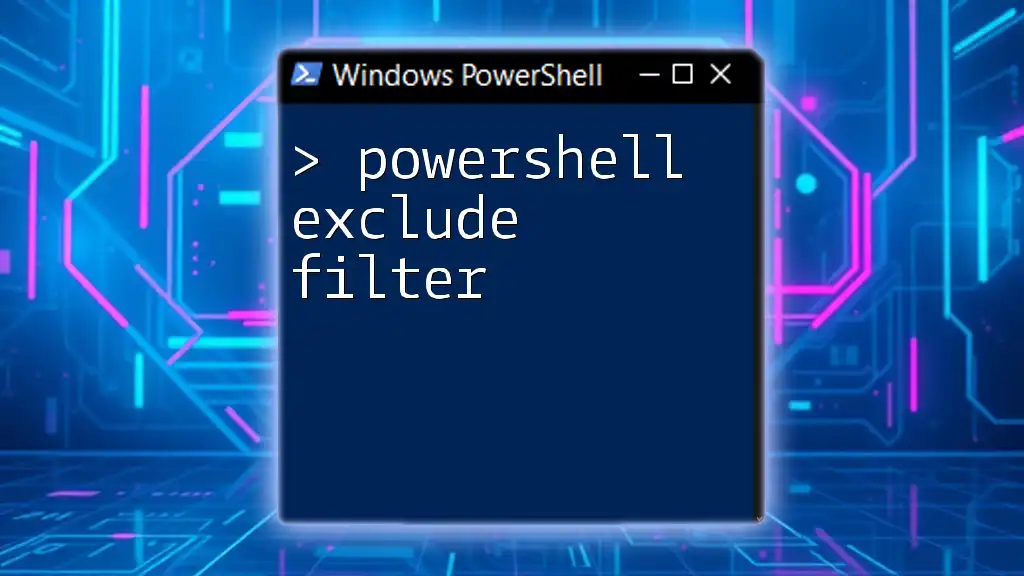
Additional Resources
For further learning, consider exploring books on PowerShell scripting, the official Microsoft documentation, and community forums where you can discuss best practices and advanced techniques with fellow enthusiasts.