In PowerShell, a boolean is a data type that represents one of two values: `$true` or `$false`, which are often used to control the flow of execution in scripts and commands.
# Example of a boolean evaluation
$IsActive = $true
if ($IsActive) {
Write-Host 'The condition is true.'
} else {
Write-Host 'The condition is false.'
}
What are Booleans?
Booleans are fundamental data types used in programming to represent truth values, specifically true and false. In PowerShell, as in many programming languages, booleans are crucial for controlling the flow of a script by enabling conditions, branching, and logical operations.
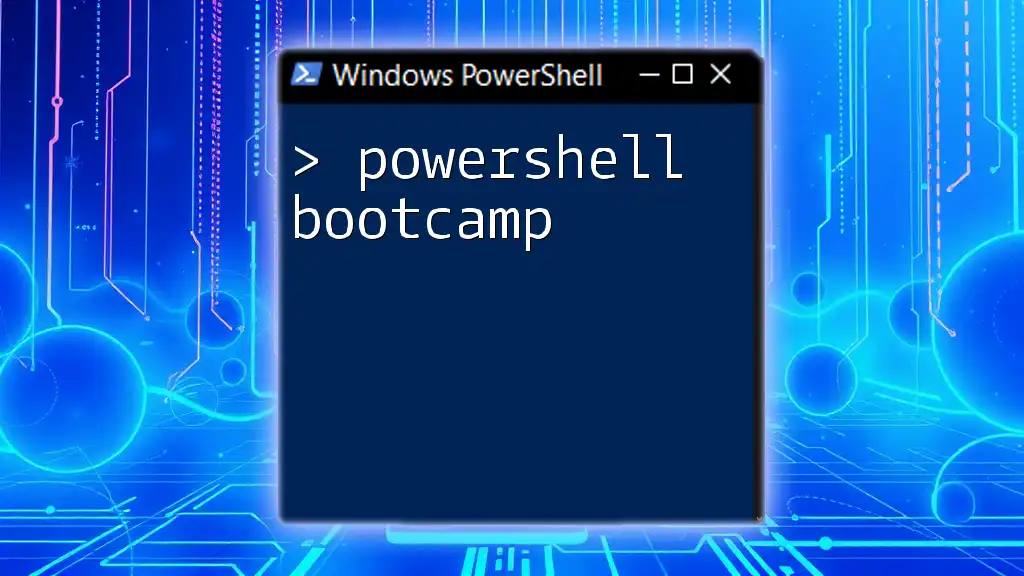
Understanding PowerShell Variables
Before diving into PowerShell booleans, it's essential to understand how variables work. In PowerShell, a variable is a container for storing data that can be used and manipulated during a script's execution. With variable types including strings, integers, arrays, and more, boolean variables specifically handle true/false values.
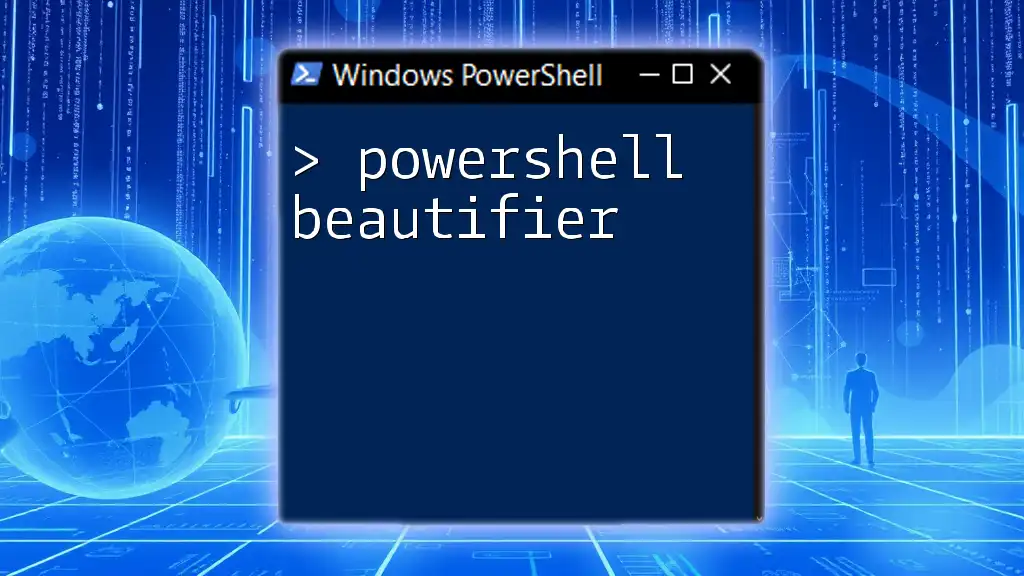
PowerShell Boolean Variables
Creating a PowerShell Boolean Variable
Creating a boolean variable in PowerShell is straightforward. You define a variable using the `$` sign followed by the variable name and assign it a boolean value, either `$true` or `$false`. The syntax is as follows:
$myBool = $true
Types of Boolean Values
In PowerShell, the two primary boolean values are `$true` and `$false`. These are the default representations of true and false in the environment. Using boolean variables effectively can streamline your code and improve readability.
Consider this example:
$isReady = $false
if ($isReady) {
"The system is ready!"
} else {
"The system is not ready yet."
}
In this snippet, the condition checks the boolean variable `$isReady`. Since its value is set to `$false`, the output will affirm that the system is not ready.
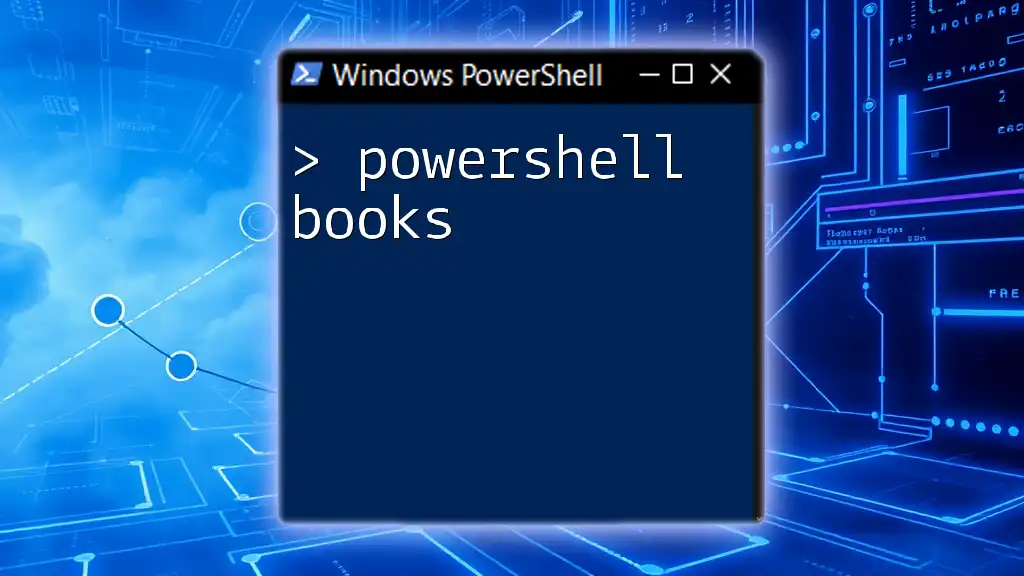
Working with Booleans in PowerShell
Using Boolean Logic
PowerShell provides logical operators—AND, OR, and NOT—that enable the manipulation of boolean values. Understanding how to leverage these operators is vital for effective scripting.
Examples of Each Operator:
-
AND Operator (`-and`): This operator returns true only if both operands are true.
$a = $true $b = $false $andResult = $a -and $b # $andResult is false
-
OR Operator (`-or`): Returns true if at least one of the operands is true.
$orResult = $a -or $b # $orResult is true
-
NOT Operator (`-not`): Reverses the boolean value of its operand.
$notResult = -not $a # $notResult is false
Boolean Expressions in Conditions
Using boolean variables in conditionals is a fundamental aspect of scripting in PowerShell. The `if` statement allows for conditional execution based on the outcome of a boolean expression. Here's an example:
if ($myBool -eq $true) {
"Condition is true!"
} else {
"Condition is false!"
}
In this snippet, the script checks if `$myBool` is equal to `$true`. Based on this check, it executes the corresponding block of code.
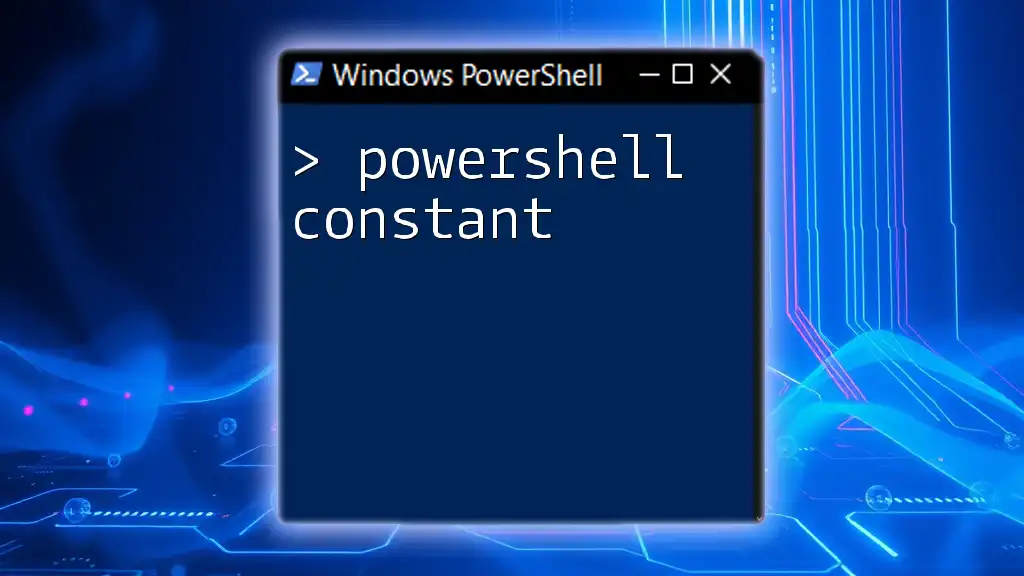
Boolean Functions and Methods
Common Functions Involving Booleans
Several built-in PowerShell functions return boolean values, such as `Test-Path`, which checks for the existence of a file or directory. The ability to use these functions efficiently allows you to build conditional logic that relies on external conditions.
For instance:
if (Test-Path "C:\example.txt") {
"File exists."
} else {
"File does not exist."
}
In this example, `Test-Path` evaluates whether the specified path exists, returning a `true` or `false` value based on the result.
Creating Custom Boolean Functions
You can also create custom functions that return boolean values in PowerShell. This flexibility allows you to encapsulate logic and reuse it throughout your scripts for improved organization.
Here’s an example of a simple custom function that checks if a number is even:
function IsEven {
param ($number)
return ($number % 2 -eq 0)
}
if (IsEven 4) {
"4 is even."
} else {
"4 is odd."
}
In this case, the function `IsEven` will return `$true` if the provided number is even, and it can be utilized in conditions as shown.
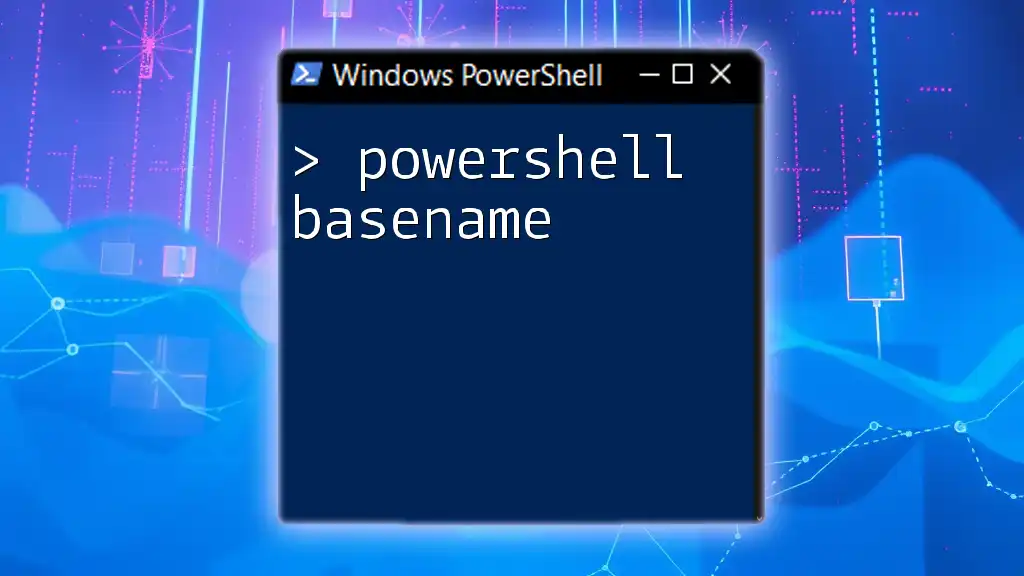
Best Practices for Using Booleans
Consistent Use of Boolean Values
To maintain clarity in your scripts, it's essential to consistently use boolean values. This includes clearly distinguishing when to use `$true` versus `$false` and ensuring that your expressions are straightforward.
Debugging Boolean Logic
When working with booleans, you may encounter challenges, especially in complex expressions. Here are some tips for troubleshooting:
-
Use Verbose Output: Incorporate `Write-Host` or `Write-Output` statements to track the flow and see actual values during script execution.
-
Simplify Conditions: If a boolean expression isn't returning as expected, break it down into simpler components for easier testing.
-
Logical Flow: Ensure that your logical flow aligns with the intended outcomes, examining each branch of your conditionals thoroughly.
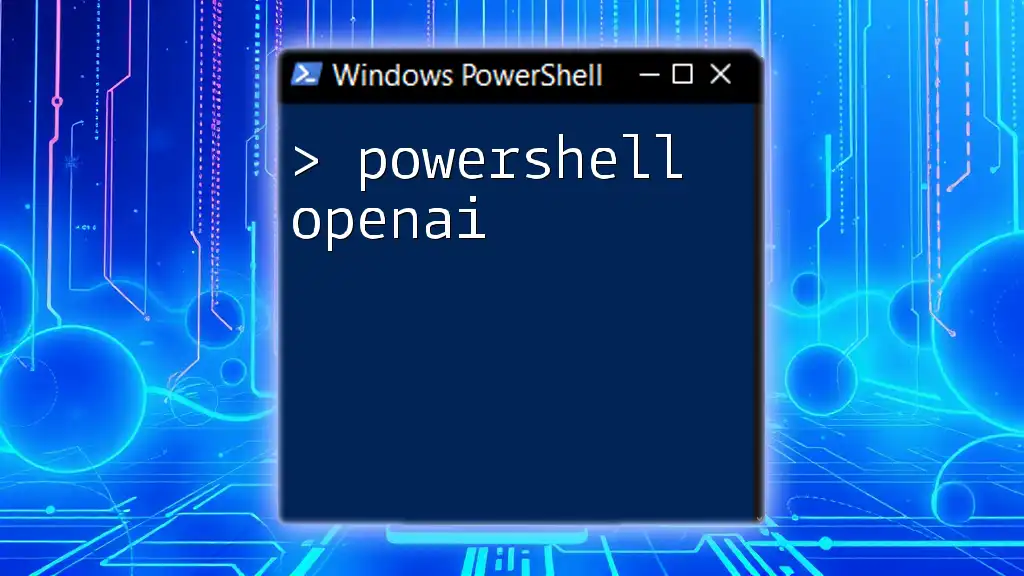
Conclusion
In summary, mastering PowerShell booleans is essential for anyone looking to script efficiently in PowerShell. Understanding how to create, manipulate, and use boolean variables and expressions will empower you to write clearer and more effective scripts.
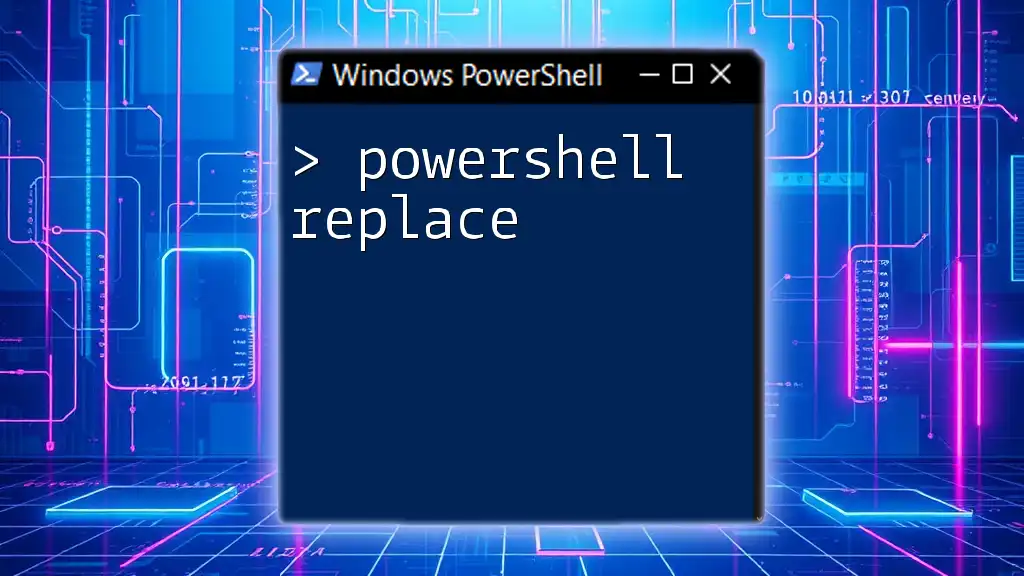
Additional Resources
For those interested in delving deeper into the topic, plenty of documentation and learning materials exist online. Engaging with the community can also provide additional insights and shared experiences, enhancing your PowerShell journey.