In PowerShell, an object is an instance of a class that can hold data and methods, allowing users to manipulate and interact with complex data structures efficiently.
Here's a simple example of creating and displaying a PowerShell object:
$person = New-Object PSObject -Property @{ Name = 'John Doe'; Age = 30 }
$person | Format-List
Understanding PowerShell Objects
What is an Object in PowerShell?
In PowerShell, an object is a data structure that encapsulates both data and behavior. Each object consists of attributes, known as properties, which describe the object, and methods, which define actions the object can perform. Understanding objects is crucial for harnessing the full potential of PowerShell.
The Relationship Between Objects and Classes
Objects are instances of classes, which define the blueprint for the attributes (properties) and methods an object will possess. Here’s a simple illustration of creating a basic class in PowerShell:
class Person {
[string]$Name
[int]$Age
Person([string]$name, [int]$age) {
$this.Name = $name
$this.Age = $age
}
}
$john = [Person]::new("John Doe", 30)
In this example, `Person` is a class that contains two properties, `Name` and `Age`. The `$john` variable is an instance of this class, representing a specific person.
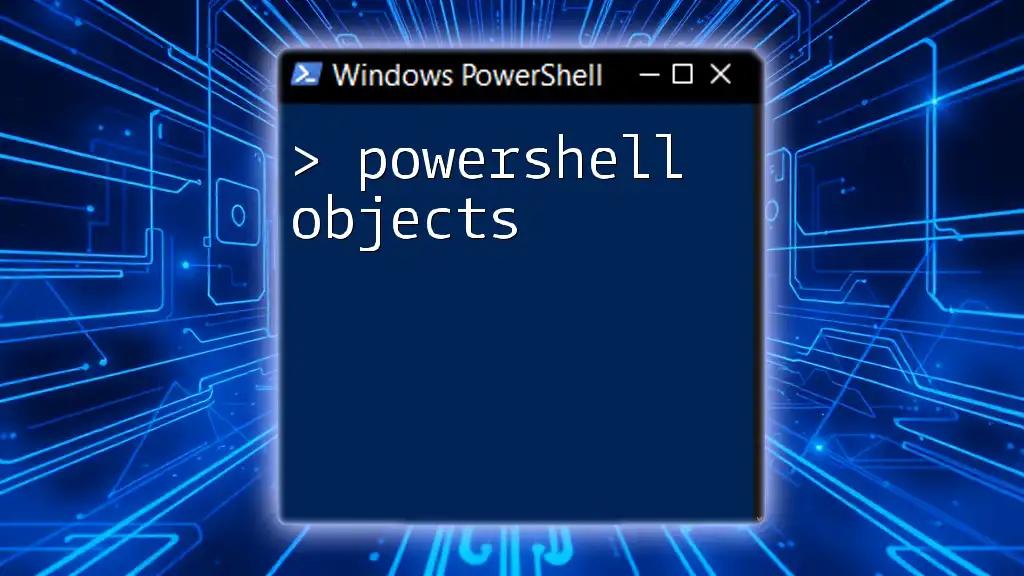
Properties of PowerShell Objects
What are Properties?
Properties are the characteristics or attributes of an object. They hold data related to the object, and can be accessed and modified. Here’s how to retrieve the properties of a process object:
$process = Get-Process | Where-Object { $_.Name -eq 'notepad' }
$process | Select-Object Name, Id, WorkingSet
In this command, we use the `Select-Object` cmdlet to access the `Name`, `Id`, and `WorkingSet` properties of the Notepad process.
How to Create and Modify Object Properties
Creating and modifying properties can enhance the usefulness of objects. You can easily define a new object and update its properties as follows:
$myCar = New-Object PSObject -Property @{
Make = "Toyota"
Model = "Camry"
}
$myCar.Make = "Honda" # Modifying existing property
Here, we created a car object with properties `Make` and `Model`. Later, we modified the `Make` property to reflect a change.
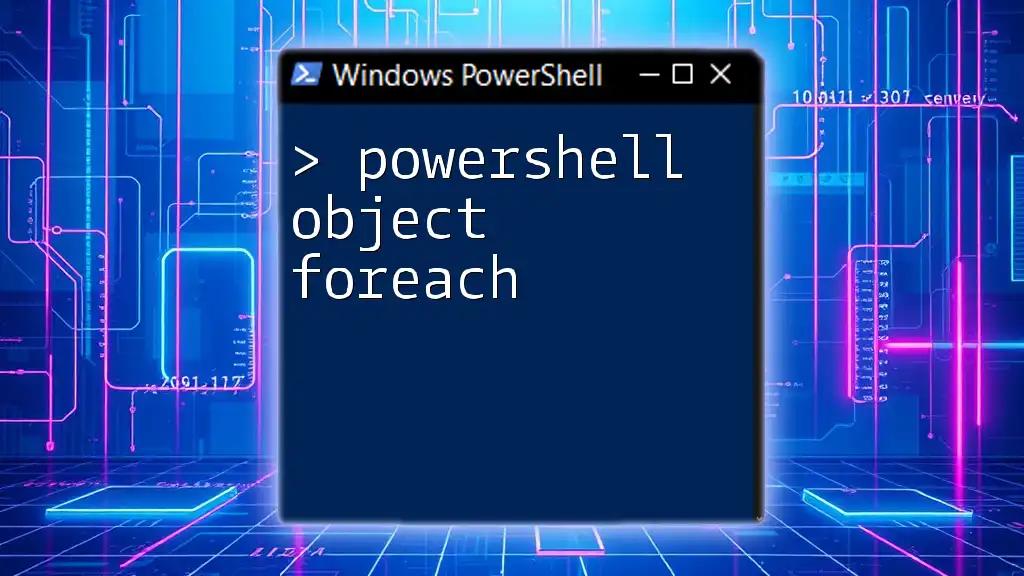
Methods of PowerShell Objects
What are Methods?
Methods are functions associated with objects that define the operations the objects can perform. They play a vital role in manipulating data in PowerShell.
Calling Methods on Objects
You can call methods on objects as shown in the following example:
$myArrayList = New-Object System.Collections.ArrayList
$myArrayList.Add("Item1")
$myArrayList.Add("Item2")
$myArrayList.Remove("Item1") # Calling a method
In this example, we've instantiated an ArrayList and used the `Add` method to insert items. Later, we called the `Remove` method to delete an item.
Creating Custom Methods
Defining and implementing your own methods within a PowerShell class allows for specialized functionality. Here’s an example of a basic calculator class:
class Calculator {
[int] Add([int]$a, [int]$b) {
return $a + $b
}
}
$calc = [Calculator]::new()
$calc.Add(5, 10) # Calling the custom method
In this snippet, the `Add` method inside the `Calculator` class demonstrates how you can create reusable code for summing two integers.

Working with Common PowerShell Objects
Built-in PowerShell Objects
PowerShell comes with several built-in objects that are fundamental to scripting. Examples include `PSObject`, `PSCustomObject`, and `Hashtable`. Understanding these objects is key to writing effective scripts.
Examples of PowerShell Objects in Action
To see PowerShell objects in action, consider the following example that retrieves the status of running processes:
Get-Service | Where-Object { $_.Status -eq 'Running' }
This command effectively filters the service objects, returning only those with a Running status.

Creating and Using Custom Objects
Using `New-Object` to Create Objects
Custom objects can be created using the `New-Object` cmdlet. This can be particularly useful for scenarios where you need to aggregate data.
Using `Select-Object` and Custom Properties
You can also create custom objects by using `Select-Object` to extract and customize properties. For example:
Get-Process | Select-Object Name, Id, @{Name='Working Set (MB)';Expression={[math]::round($_.WorkingSet/1MB,2)}}
In this example, we create a custom property that converts the `WorkingSet` to megabytes and rounds it to two decimal places.
Understanding `PSCustomObject`
`PSCustomObject` is a flexible way to create simple objects, allowing for easy definition of properties and values. Here’s how:
$myCustomObject = [PSCustomObject]@{
Name = "Alice"
Age = 25
}
This creates a lightweight object with easily accessible properties.
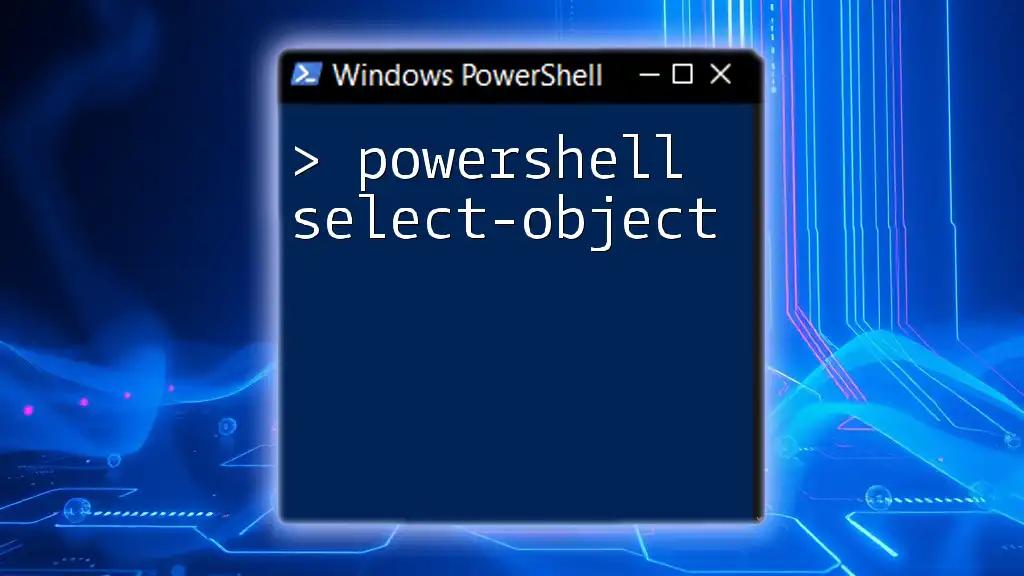
Managing Object Pipelines
Object Pipeline Basics
In PowerShell, objects can be passed through a pipeline, enabling powerful and succinct scripting capabilities. The pipeline takes the output of one cmdlet and uses it as the input for another.
Advanced Pipeline Techniques
More complex object manipulations can be done through the pipeline, facilitating streamlined data transformations.
Get-Process | Where-Object { $_.CPU -gt 1 }
This command filters processes by CPU usage. Here, the output of `Get-Process` is passed through the pipeline to `Where-Object`, which selects the relevant processes based on a condition.
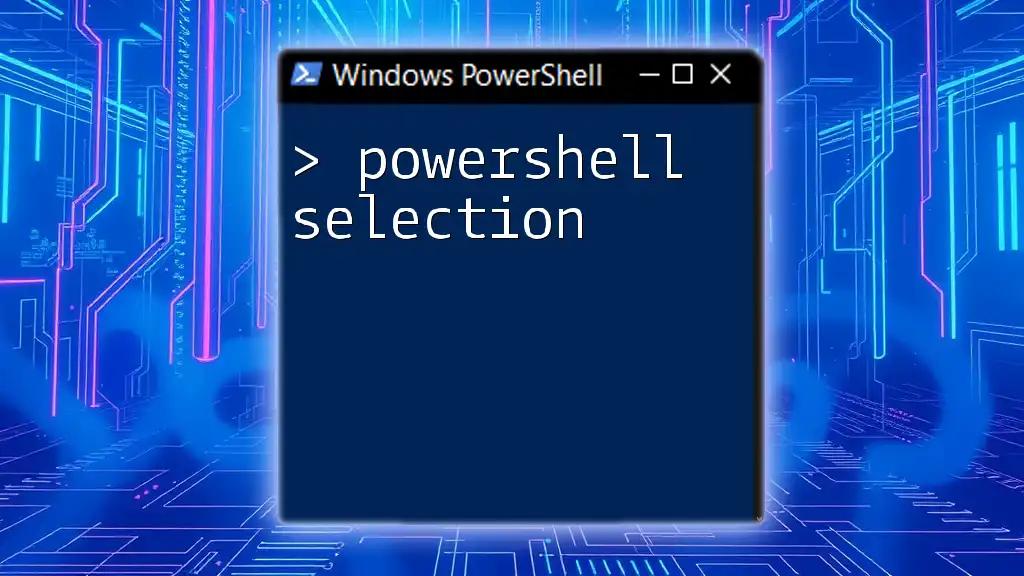
Conclusion
Understanding PowerShell objects is crucial for anyone looking to leverage PowerShell for automation and system administration tasks. With their flexible nature and capability to hold data and methods, objects are the cornerstone upon which PowerShell operates.
As you explore these concepts, practice using objects in your scripts to enhance productivity and efficiency. For further learning, consider accessing additional resources or attending workshops focused on mastering PowerShell.