PowerShell checksum refers to the process of generating a unique hash value for a file, which helps verify its integrity and ensure it hasn't been altered.
Here's a simple code snippet to calculate the checksum of a file using PowerShell:
Get-FileHash -Path "C:\path\to\your\file.txt" -Algorithm SHA256
Understanding Checksums in PowerShell
What is a Checksum?
A checksum is a string of characters generated from a set of data, usually used to verify the integrity of that data. Its specific role is to help detect errors that may have occurred during data transmission or storage. Essentially, it performs a quick mathematical operation on the content of a file and produces a fixed-size string that summarizes that content.
Common uses of checksums include:
- File Transfers: Ensuring that files sent over a network are not corrupted.
- Backups: Verifying that backups remain intact and unaltered over time.
- Security: Checking for unauthorized modifications or tampering.
Why Use Checksums in PowerShell?
The importance of data integrity cannot be overstated, particularly in environments where files are transmitted across networks or stored for extended periods. Checksums help guarantee that the data you are working with remains unchanged, providing confidence in your operations.
Additionally, checksums aid in error detection. When a checksum is calculated and saved upon the initial creation or transfer of a file, it can be recalculated later to check if the file has remained unchanged. If they don't match, you can infer that the file has either been corrupted or intentionally altered.
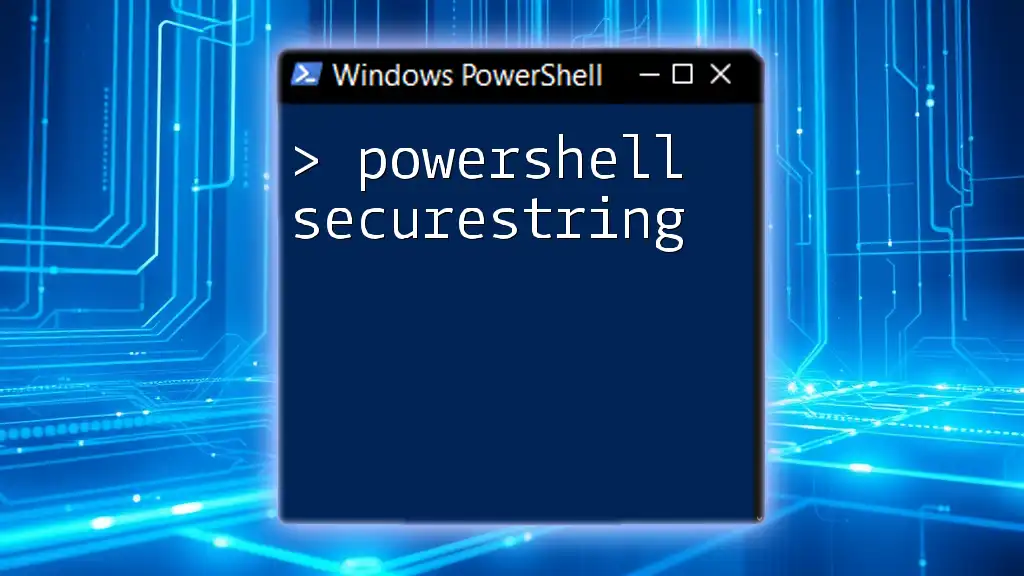
Common Checksum Algorithms in PowerShell
Overview of Checksum Algorithms
PowerShell supports several algorithms for generating checksums, which are crucial for different use cases:
-
MD5: This algorithm produces a 128-bit hash value. While it is fast, it is widely considered insecure due to vulnerabilities that can allow for hash collisions (different input producing the same output).
-
SHA-1: Producing a 160-bit hash value, SHA-1 is more secure than MD5 but has also been found to have vulnerabilities. Still, it is often used in various applications.
-
SHA-256: Part of the SHA-2 family, SHA-256 generates a 256-bit hash and is highly recommended for modern applications requiring strong security.
Comparing Checksum Algorithms
When selecting a checksum algorithm, one must consider the balance between speed and security. Generally, faster algorithms like MD5 may suit applications where speed is crucial, but they come with increased risk. On the other hand, SHA-256 offers much greater security at the expense of performance.
To choose the right algorithm, consider your specific needs:
- If data integrity is paramount, opt for SHA-256.
- For scenarios requiring fast verification with acceptable risk, MD5 or SHA-1 might suffice.
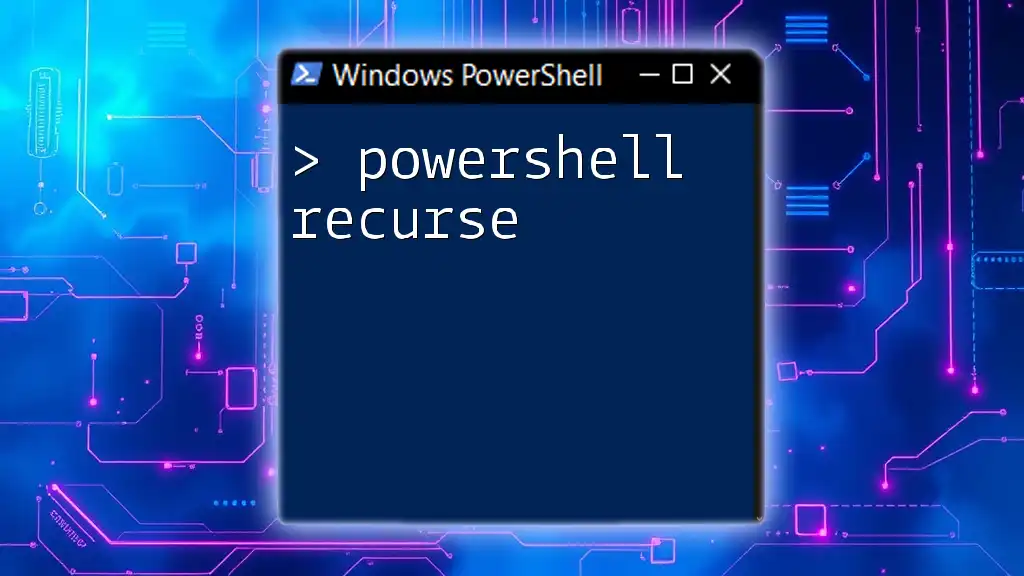
Generating Checksums Using PowerShell
Using Get-FileHash cmdlet
PowerShell provides a straightforward way to compute checksums using the `Get-FileHash` cmdlet. This cmdlet enables users to perform hash calculations on files without needing any external libraries or tools.
Basic Syntax
The syntax is incredibly intuitive:
Get-FileHash -Path "C:\path\to\your\file.txt"
This command generates a hash for the specified file, utilizing the default SHA-256 algorithm.
Example
As an example, generate an SHA-256 checksum for a file named `example.txt`:
Get-FileHash -Path "C:\path\to\example.txt" -Algorithm SHA256
This command returns the hash value, allowing you to verify that the file has not been altered since the last calculation.
Generating Multiple Checksums
When you want to calculate checksums for many files within a directory, you can automate the process using a loop:
$files = Get-ChildItem "C:\path\to\your\directory\*"
foreach ($file in $files) {
Get-FileHash -Path $file.FullName -Algorithm SHA256
}
This snippet retrieves all files in a specified directory and generates an SHA-256 checksum for each file, allowing for bulk calculations, saving considerable time in the process.
Custom Checksum Functions in PowerShell
To make your checksum operations reusable, you can define a custom function. Here's how you can create a function called `Get-Checksum`:
function Get-Checksum {
param (
[string]$Path,
[string]$Algorithm = "SHA256"
)
Get-FileHash -Path $Path -Algorithm $Algorithm
}
With this function, you can easily compute checksums by just calling `Get-Checksum -Path "C:\myfile.txt"` while specifying the desired algorithm if needed.
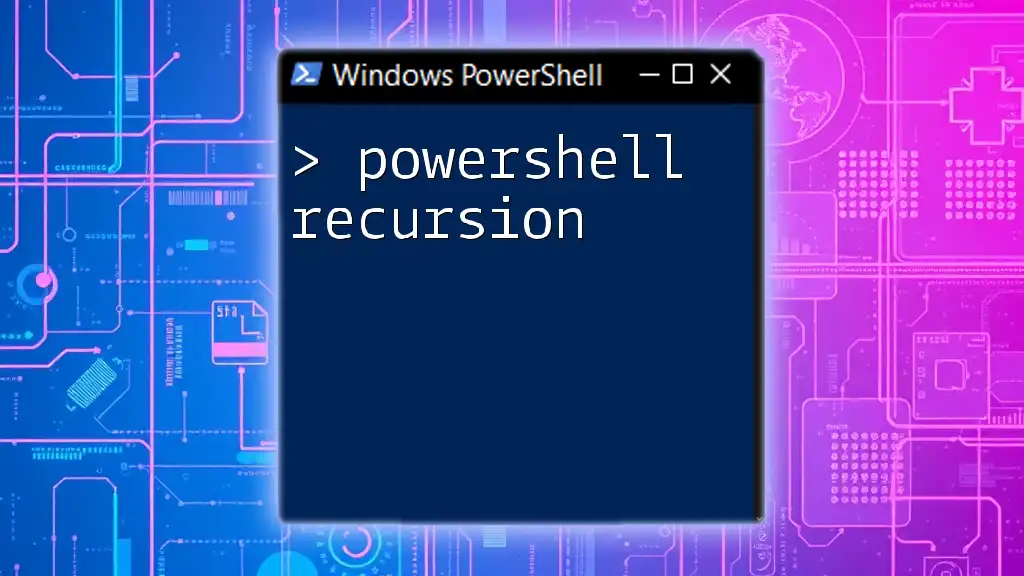
Verifying Checksums in PowerShell
How to Verify a Checksum
Verifying checksums is critical, especially after downloading or transferring files. The steps are straightforward: calculate the checksum of the file in question and compare it to a known value.
Example of Checksum Verification
Let’s say you have a known checksum for a file and want to verify whether the file has remained intact:
$originalChecksum = "your_known_checksum"
$calculatedChecksum = (Get-FileHash -Path "C:\path\to\downloaded_file.txt").Hash
if ($calculatedChecksum -eq $originalChecksum) {
"Checksum verified successfully."
} else {
"Checksum verification failed."
}
In this example, the script compares the calculated checksum to a known good checksum. If they match, the integrity of the file is confirmed.
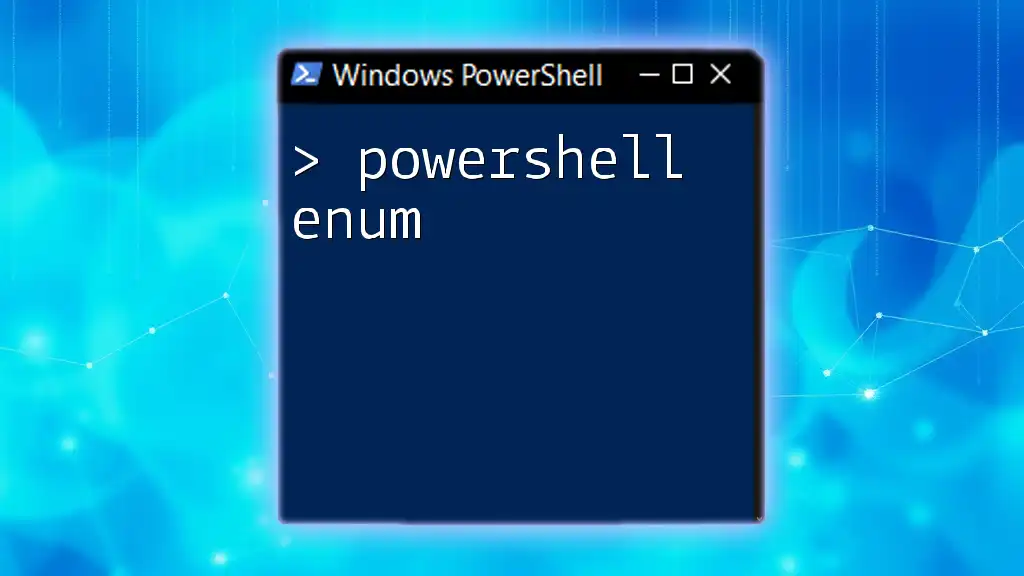
Advanced Checksum Operations
Creating Checksum Files
A useful feature of PowerShell is the ability to generate a checksum file that lists checksums for multiple files, which is particularly effective for backup or verification processes. Here’s how you can do that:
Get-ChildItem "C:\path\to\your\directory\*" | Get-FileHash -Algorithm SHA256 | Export-Csv "C:\path\to\checksum_file.csv" -NoTypeInformation
This command creates a CSV file containing the checksum for each file in the specified directory, enabling easy comparisons later.
Comparing Checksums of Different Files
Sometimes it's necessary to compare checksums from different versions of a single file. Here’s a simple way to do that:
$original = Get-FileHash -Path "C:\path\to\original_file.txt"
$updated = Get-FileHash -Path "C:\path\to\updated_file.txt"
if ($original.Hash -eq $updated.Hash) {
"Files are identical."
} else {
"Files differ."
}
This script will help you quickly ascertain if any changes have occurred between two versions of a file.
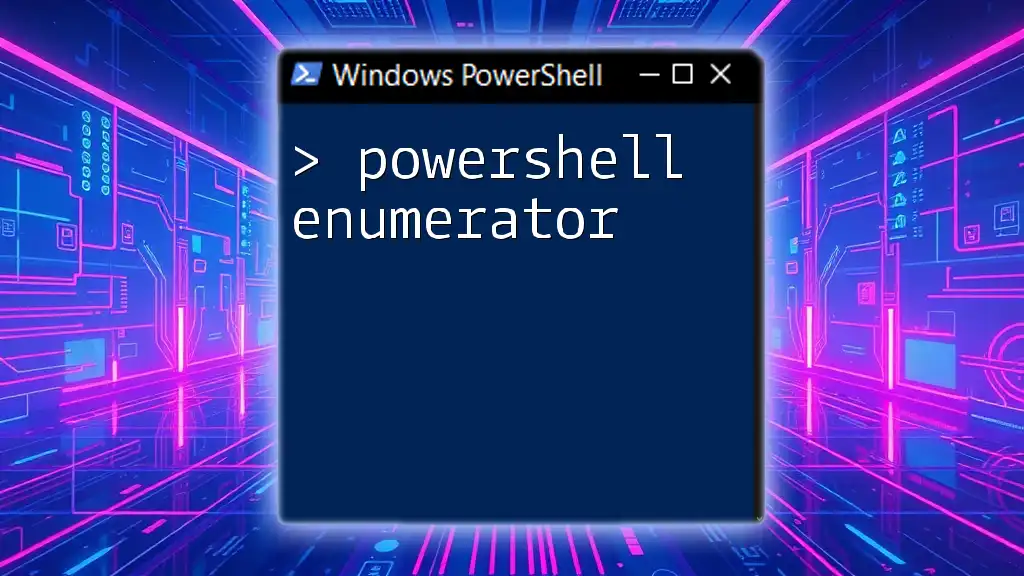
Best Practices for Using Checksum in PowerShell
Regular Checks
It is a good practice to routinely verify checksums for crucial files, especially those that undergo frequent updates or are critical to operations. Scheduling these checks can help catch and resolve issues proactively.
Security Considerations
While MD5 and SHA-1 are widely recognized, their vulnerabilities necessitate caution. It’s advisable to avoid these weaker algorithms unless absolutely necessary. Instead, prioritize algorithms with stronger security guarantees, like SHA-256.
Furthermore, when storing checksum values, consider using secure methods to prevent tampering or unauthorized access.
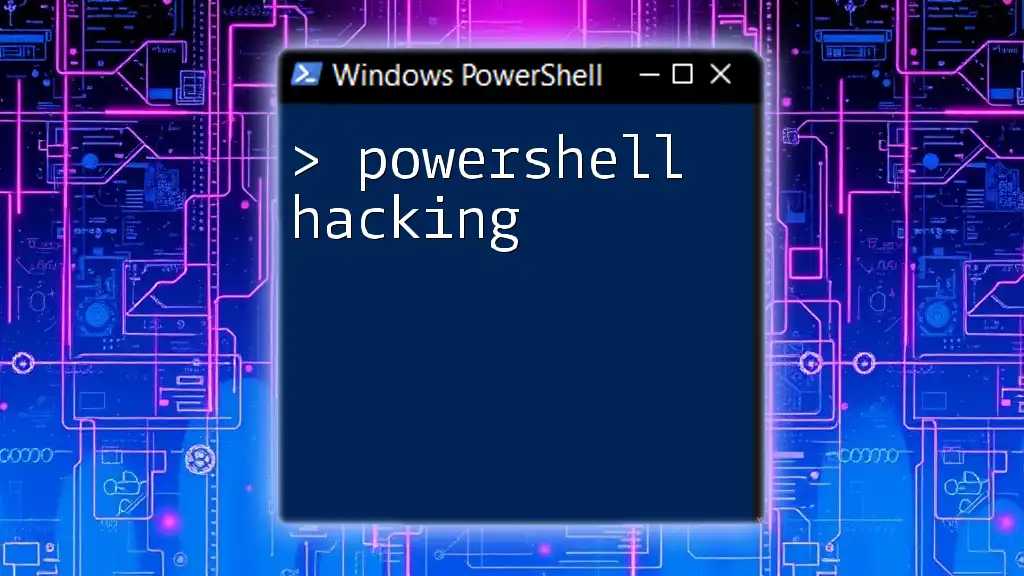
Conclusion
Utilizing PowerShell to compute and verify checksums not only aids in maintaining data integrity but also enhances security protocols in your computing environment. By incorporating the practices discussed in this guide, you can effectively safeguard your data, ensuring consistency and reliability in your file operations. Implement these methods into your daily routines, and you'll significantly bolster your data management efforts.
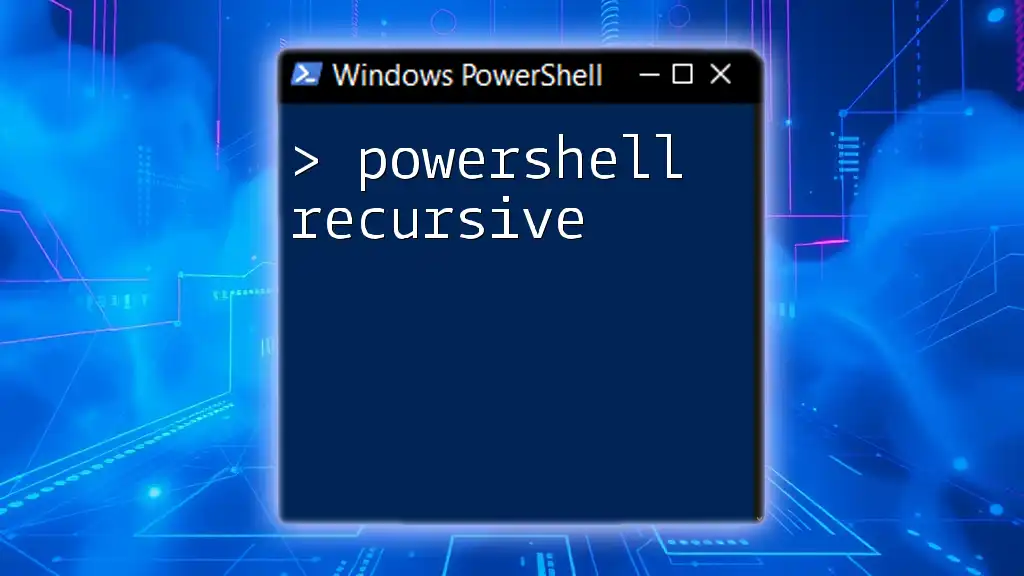
Additional Resources
To dive deeper into PowerShell and checksum verification methods, refer to official Microsoft documentation on PowerShell cmdlets and hash algorithms. Engaging with community forums may also provide useful insights and real-world applications. Share your experiences and continue learning to enhance your PowerShell mastery!