To check if a folder exists in PowerShell, you can use the `Test-Path` cmdlet, which returns `True` if the path exists and `False` otherwise.
Here's a code snippet to demonstrate this:
$folderPath = "C:\YourFolderName"
if (Test-Path $folderPath) {
Write-Host "The folder exists."
} else {
Write-Host "The folder does not exist."
}
Understanding PowerShell and File System Operations
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed for system administration tasks and automation. It enables users to execute commands, manage system resources, and automate repetitive tasks across both local and remote systems. By utilizing cmdlets and scripts, system administrators can achieve greater efficiency and control over the Windows environment.
PowerShell and File System Commands
PowerShell provides a rich set of commands for file system operations, making it an ideal choice for managing files and directories. A common scenario in file management is determining whether a specific folder or directory exists before executing subsequent commands—this is where using PowerShell to check if a folder exists becomes essential.
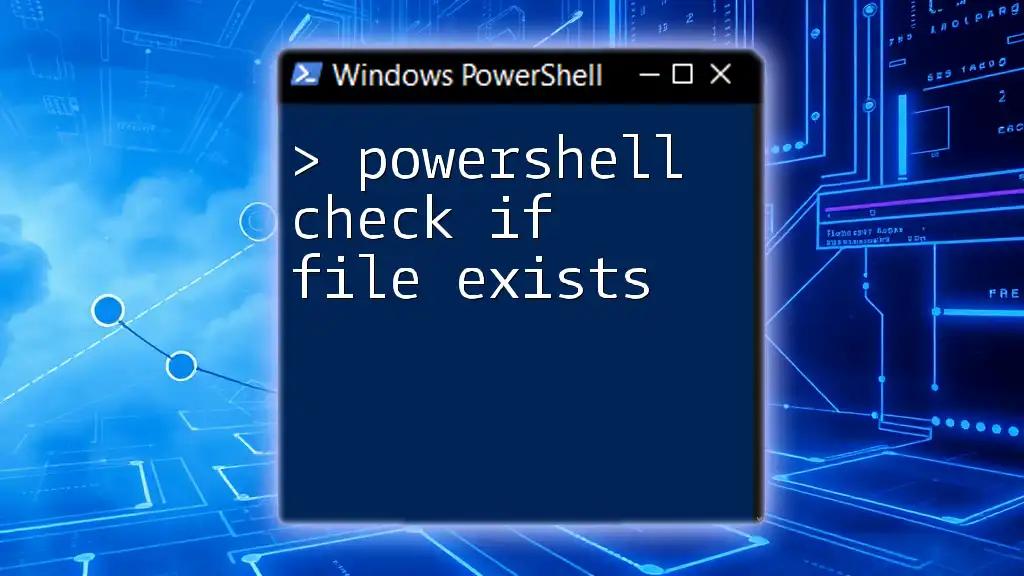
Checking If a Folder Exists in PowerShell
The `Test-Path` Cmdlet
`Test-Path` is a core cmdlet in PowerShell used to check the existence of files and folders. It returns a Boolean value indicating whether the specified path is valid. Understanding how to use this cmdlet effectively is crucial for any PowerShell script that interacts with the file system.
Syntax of `Test-Path`
The basic structure of the `Test-Path` cmdlet is:
Test-Path <path>
You replace `<path>` with the actual path to the folder you want to check.
Example: Basic Folder Existence Check
Consider the following PowerShell script that checks if a folder exists:
$folderPath = "C:\ExampleFolder"
if (Test-Path $folderPath) {
Write-Host "Folder exists."
} else {
Write-Host "Folder does not exist."
}
In this snippet, we assign the path we want to check to the `$folderPath` variable. The `if` statement evaluates whether the folder exists. If it does, it outputs "Folder exists." Otherwise, it informs the user that the folder does not exist.
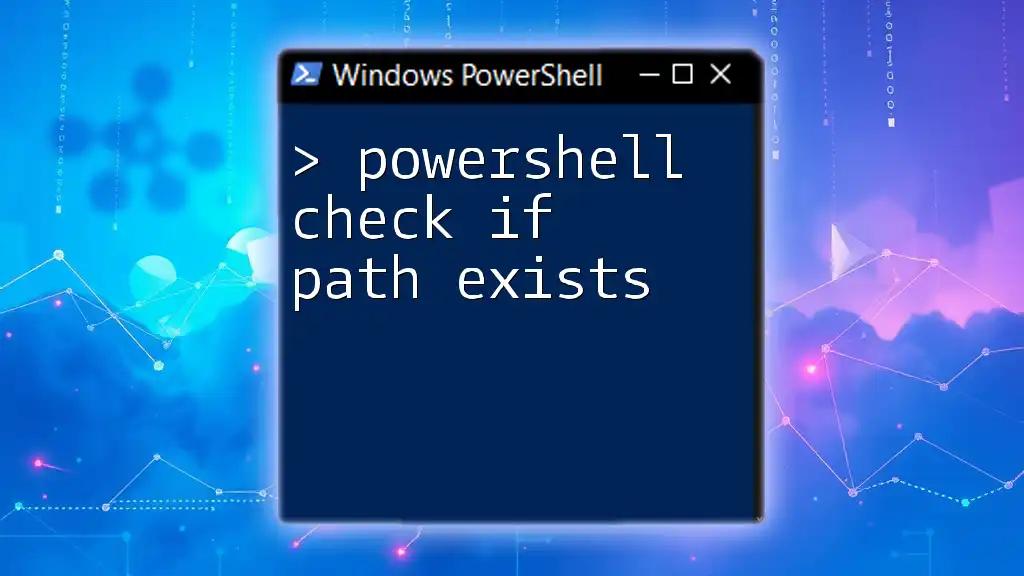
Using Conditional Statements with Folder Checks
Implementing `if` Statements
The `if` statement can be combined with the `Test-Path` cmdlet to perform more complex logic. This allows for the creation of folders only if they do not already exist, thereby preventing errors in automation scripts.
Example: Create Folder If It Doesn't Exist
$folderPath = "C:\TestFolder"
if (Test-Path $folderPath) {
Write-Host "The folder exists."
} else {
Write-Host "The folder does not exist. Creating folder..."
New-Item -ItemType Directory -Path $folderPath
}
In this code example, if the specified folder does not exist, the script will create it using the `New-Item` cmdlet. This demonstrates not only how to check for the existence of a folder but also to take action based on that condition.

Advanced Techniques for Folder Existence
Checking for Subfolders
Sometimes, it is essential to verify the existence of subfolders within a parent directory. This requires a slight modification to the path being checked.
Example: Check for Subfolder
$subfolderPath = "C:\ExampleFolder\SubFolder"
if (Test-Path $subfolderPath) {
Write-Host "Subfolder exists."
} else {
Write-Host "Subfolder does not exist."
}
In this example, we specify the path to a subfolder. The same logic applies as before—`Test-Path` checks the specified path, and the output informs the user of the result.
Combining with Other Cmdlets
For more comprehensive checks or to perform operations on multiple folders, you can combine `Test-Path` with other cmdlets. For instance, using `Get-ChildItem` allows you to retrieve directory contents and verify folder existence dynamically.
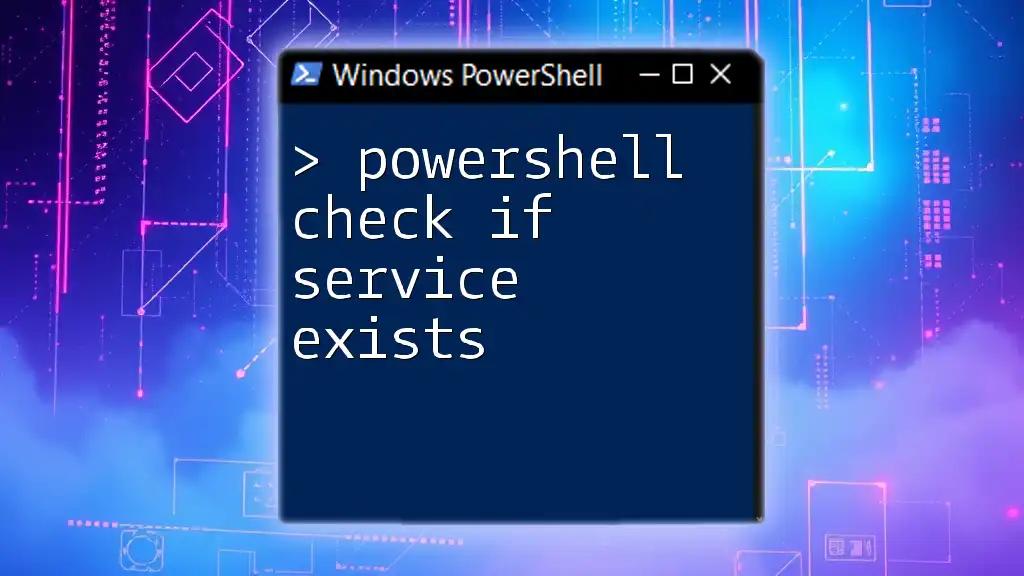
Error Handling in Folder Checks
Using Try-Catch with Folder Checks
Error handling is a vital part of scripting that helps manage unexpected issues that might occur during execution. PowerShell supports error handling via the try-catch mechanism.
Example: Error Handling
try {
if (-Not (Test-Path $folderPath)) {
throw "Folder does not exist."
}
} catch {
Write-Host "Error: $_"
}
This example checks for the existence of the folder and throws an error if it is absent. The catch block captures that error and outputs a message. This form of error handling improves the robustness of your scripts.

Tips for Effective Folder Checks
Best Practices
When automating PowerShell scripts that check for folder existence, consider using environment variables for dynamic paths. This approach ensures that scripts can adapt to different systems without needing hardcoded paths.
Performance Considerations
For scripts that might check a large number of folders, be mindful of performance impacts. If speed is a concern, consider using parallel processing to run checks for multiple folders simultaneously using the `ForEach-Object -Parallel` feature, which significantly boosts execution speed.

Conclusion
Understanding how to check if a folder exists using PowerShell empowers system administrators to create more reliable and efficient scripts. With the `Test-Path` cmdlet, combined with conditional statements and error handling, you can manage file system operations confidently and effectively.
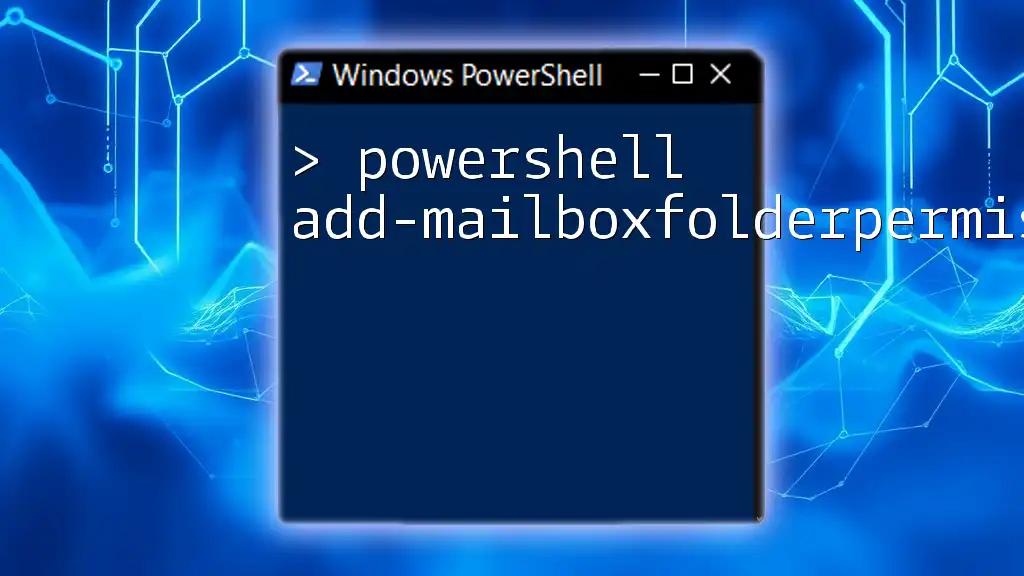
Additional Resources
For further learning, consult the official PowerShell documentation and explore community forums for tips and troubleshooting assistance. These resources are invaluable when developing and refining your scripting skills.

Call to Action
Now that you’re equipped with the knowledge of checking for folder existence, try implementing these examples in your own environment and explore how they can be integrated into your automation workflows. For more insights and advanced techniques, visit our website for comprehensive tutorials and guidance.