To check if a file exists in PowerShell, you can use the `Test-Path` cmdlet followed by the file path as shown below:
Test-Path 'C:\path\to\your\file.txt'
Understanding the Basics of File Existence in PowerShell
What Does "Check If File Exists" Mean?
When we discuss checking if a file exists in PowerShell, we refer to the process of verifying whether a specified file is present in a given location on the filesystem. This fundamental operation is vital for ensuring your scripts run smoothly without attempting actions on non-existent files, which could lead to runtime errors or unexpected behavior.
Why Check If a File Exists?
Verifying the presence of a file before performing operations on it serves various essential purposes, including:
- Error Prevention: Preventing your scripts from failing due to missing files.
- Data Integrity: Ensuring that the necessary files are available for the execution of specific tasks, preventing data loss.
- Conditional Operations: Allowing scripts to execute alternative paths based on the existence of files, enhancing flexibility.
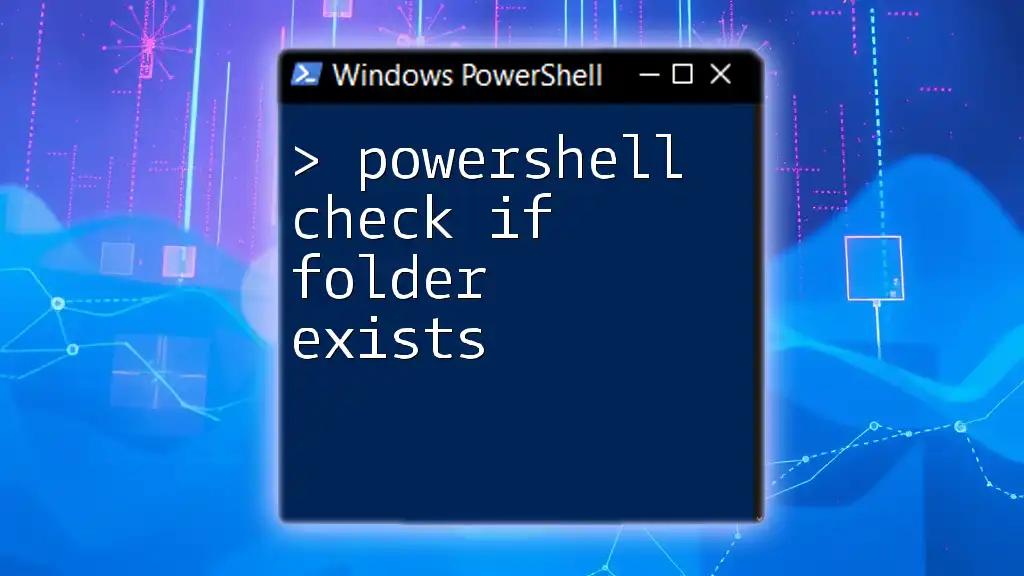
PowerShell Syntax for Checking File Existence
Introduction to cmdlets
PowerShell utilizes various cmdlets to perform tasks seamlessly. One of the most important cmdlets for checking file existence is `Test-Path`, which evaluates whether a specified path exists.
Using the Test-Path Cmdlet
The `Test-Path` cmdlet is straightforward to use. Its basic syntax is as follows:
Test-Path <path>
This command returns a Boolean value (`$true` or `$false`) based on whether the specified file or directory exists at the provided path.
Example of Test-Path
Basic Example
To illustrate how to use `Test-Path`, consider the following example where we check if a file exists:
$filePath = "C:\example\file.txt"
if (Test-Path $filePath) {
Write-Host "File exists at path."
} else {
Write-Host "File does not exist."
}
In this example, we define a variable `$filePath` that holds the path to a file. The `if` statement utilizes `Test-Path` to check whether the file exists. Depending on the result, it outputs an appropriate message. This simple check is a foundational building block for more complex scripts.
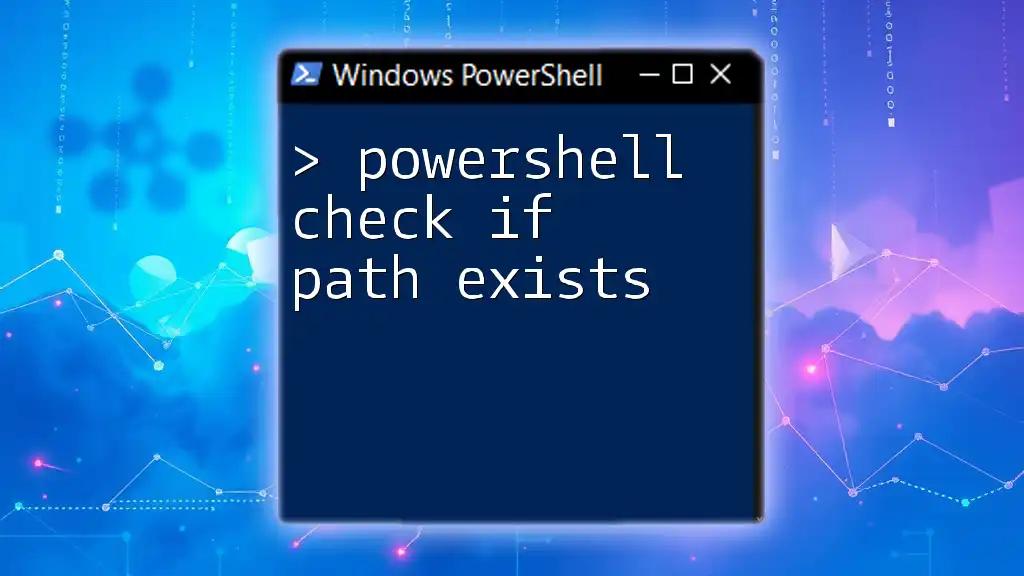
Advanced Techniques in Checking File Existence
Using If-Else Statements
To streamline conditional logic in your scripts, you can leverage if-else statements along with `Test-Path`. This allows you to execute specific code blocks based on the existence of the file.
if (Test-Path $filePath) {
# Code to execute if file exists
} else {
# Code to execute if file does not exist
}
This construct is essential in automating tasks where you need to decide whether to proceed based on the file's presence or absence.
Nested Conditions
Checking Multiple Files
You can also check for the existence of multiple files by using arrays to hold the paths of those files. This approach is efficient for automating batch operations.
$filePaths = @("C:\example\file1.txt", "C:\example\file2.txt")
foreach ($file in $filePaths) {
if (Test-Path $file) {
Write-Host "$file exists."
} else {
Write-Host "$file does not exist."
}
}
In this code snippet, we iterate through an array of file paths, checking the existence of each one. This method is particularly useful when managing groups of files in your scripts.
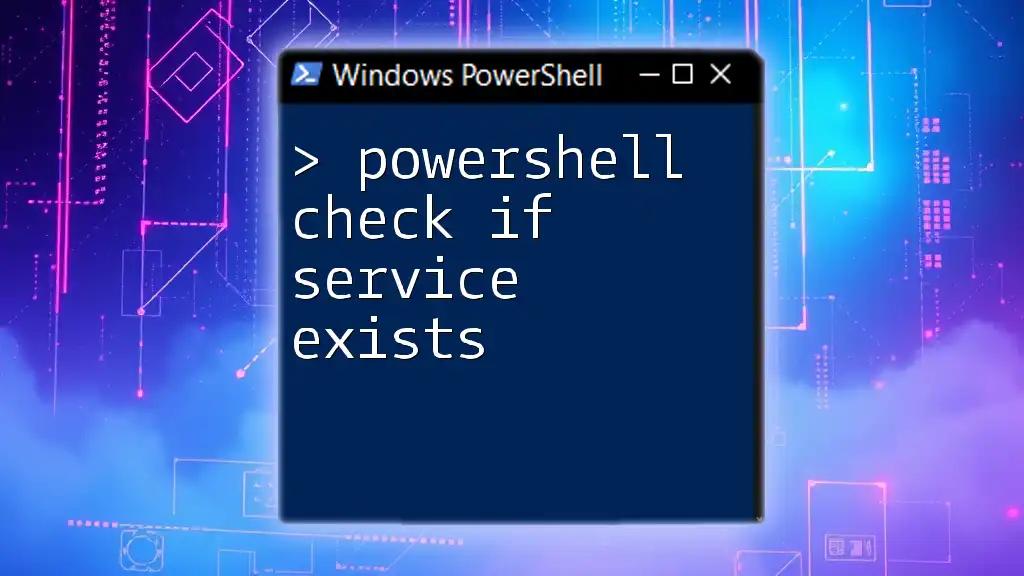
Common Use Cases for Checking File Existence
Automating Backups
One of the most practical applications of checking if a file exists is in backup scripts. Before initiating a backup, it’s crucial to confirm that the source files exist to avoid unnecessary complications.
File Processing Scripts
When processing files, checking for their existence allows your scripts to gracefully skip over missing files, thus preventing errors during execution. This ensures a smoother workflow and better end-user experiences.

Best Practices When Checking File Existence in PowerShell
Using Full Paths vs. Relative Paths
When specifying paths in your scripts, utilizing full paths minimizes confusion and potential errors. Relative paths may lead to issues based on the current working directory, while full paths provide clarity and certainty.
Handling Exceptions
It's also vital to anticipate unexpected issues while checking for file existence. Using a `try` and `catch` block allows you to manage errors effectively, ensuring your script doesn’t fail unexpectedly.
try {
if (Test-Path $filePath) {
# Process file
}
} catch {
Write-Host "An error occurred: $_"
}
In this construction, any error that arises will be caught, allowing alternative actions to be pursued or informative messages to be displayed.

PowerShell File Existence Checking in Scripts
Integrating into Scripts for Automation
Incorporating file existence checks into larger automation scripts enhances their reliability and efficiency. Here's a comprehensive script example that utilizes such checks:
# Backup Script
$sourcePath = "C:\example\data.txt"
$backupPath = "C:\backups\data_backup.txt"
if (Test-Path $sourcePath) {
Copy-Item $sourcePath $backupPath
Write-Host "Backup completed successfully."
} else {
Write-Host "Source file does not exist. Backup aborted."
}
In this script, we check if a source file exists before performing the backup. This method ensures that actions occur only when it is safe to do so, thereby enhancing the overall functionality of the script.
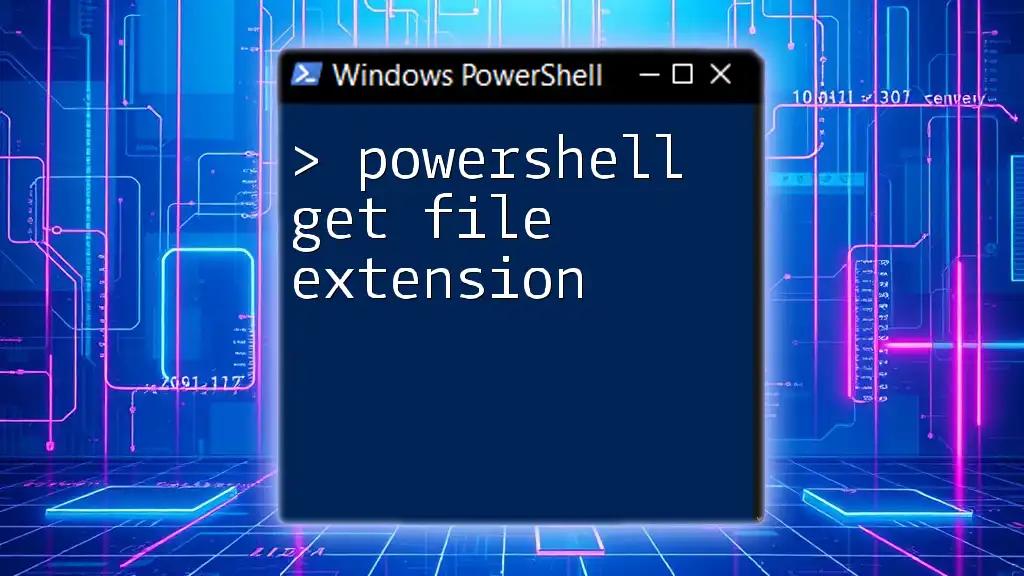
Conclusion
Checking if a file exists in PowerShell is a vital skill for anyone scripting with this powerful tool. By understanding how to effectively use `Test-Path` and implementing conditional logic in your scripts, you can significantly optimize your automation tasks. Mastering this technique will not only prevent common errors but also lead to more efficient and robust PowerShell scripts, making your coding endeavors smoother and more professional.
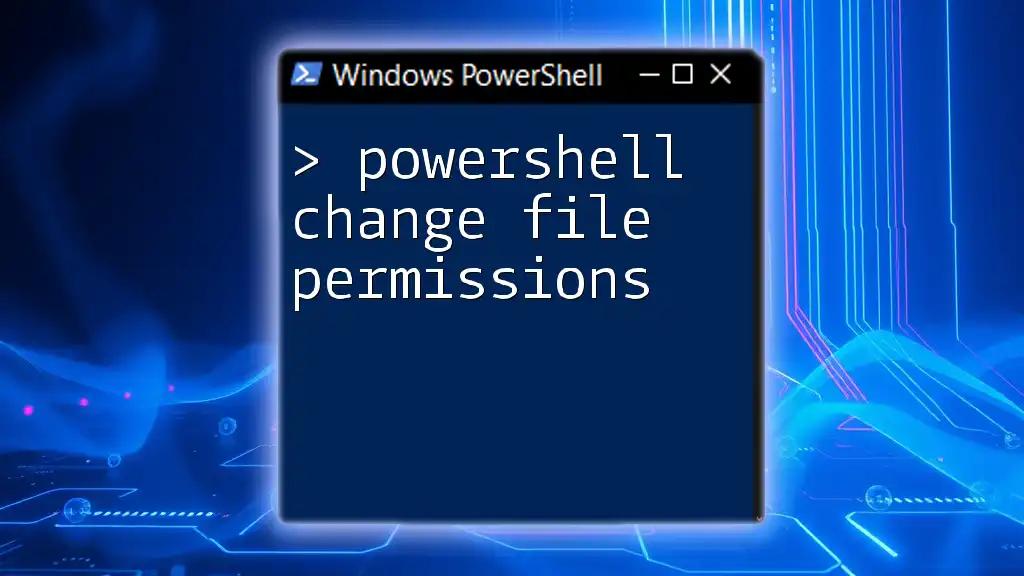
Further Reading
For more in-depth exploration of PowerShell commands and best practices, consider reviewing the official PowerShell documentation. Additionally, look into specialized books and online tutorials that focus on advanced PowerShell scripting techniques.

FAQ Section
What is the difference between Test-Path and other commands for checking file existence?
`Test-Path` is specifically designed to evaluate the existence of file and directory paths. Other commands may be intended for different functions, making `Test-Path` the most straightforward choice for checking file presence.
How can I check if a folder exists using PowerShell?
You can use the same `Test-Path` cmdlet with the path of the folder instead. The syntax remains the same, and it will accurately assess whether the specified folder exists.
Can I check for file existence on remote paths?
Yes, you can use `Test-Path` to check for files on remote paths as long as you have the necessary permissions and the required network path is accessible.