To check if a PowerShell module is installed, you can use the `Get-Module -ListAvailable` command to verify its presence and conditionally respond based on the result.
if (Get-Module -ListAvailable -Name "YourModuleName") { Write-Host "Module is installed." } else { Write-Host "Module is not installed." }
Understanding PowerShell Modules
What is a PowerShell Module?
A PowerShell module is essentially a package that contains PowerShell functions, cmdlets, variables, and even workflows. They provide a way to group related elements for better organization and ease of use. These modules can be built-in, custom-made, or sourced from third-party developers.
Why Use PowerShell Modules?
PowerShell modules significantly enhance the power and usability of the command line. By utilizing modules, users can automate tedious tasks, manage systems more efficiently, and leverage existing functions for faster workflows. For instance, system administrators often use modules to streamline the management of servers and services, while developers may utilize modules to handle deployment processes.
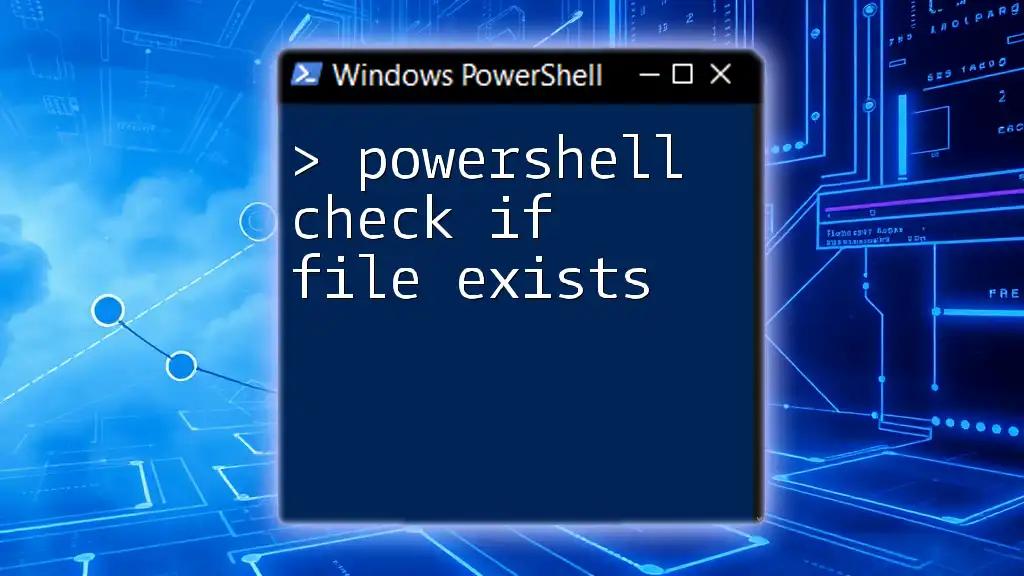
Checking Installed Modules in PowerShell
Using `Get-Module` to List Installed Modules
To check if a module is installed, one of the most effective methods is to use the `Get-Module` command. This command retrieves information about the modules that are currently imported into your PowerShell session.
The basic syntax for using `Get-Module` is:
Get-Module -ListAvailable
When you run this command, PowerShell will return a list of all available modules, along with their names, versions, and other relevant details. Here’s a sample output you might see:
ModuleType Version Name ExportedCommands
---------- ------- ---- ----------------
Script 1.0.0 MyModule {Get-Foo, Set-Foo}
Binary 2.1.3 AnotherModule {Get-Bar, Set-Bar}
This output provides a comprehensive overview of what modules are installed on your system, making it easy to identify whether a particular module exists.
Filtering Installed PowerShell Modules
Using `Where-Object`
If you want to narrow down the results to check for specific modules, you can use the `Where-Object` cmdlet in conjunction with `Get-Module`. This allows you to filter the list according to your criteria.
For example, to check if a module named "ModuleName" is installed, you can execute:
Get-Module -ListAvailable | Where-Object {$_.Name -eq "ModuleName"}
This command will return details about "ModuleName" if it exists, or no output if it does not.
Checking Specific Version of a Module
In scenarios where the version of a module is crucial, you can check for both the name and version using the following code snippet:
Get-Module -ListAvailable | Where-Object {$_.Name -eq "ModuleName" -and $_.Version -eq "1.0.0"}
This command ensures that not only is the module present, but it also confirms that it matches the required version.

Methods to Verify Module Installation
Using `Select-Object` for Detailing Module Info
For further detail about installed modules, you can pipe the output of `Get-Module` to `Select-Object`. This command allows you to specify which properties you want to see, enabling you to customize the displayed output to your needs. Here’s how to get a more detailed view:
Get-Module -ListAvailable | Select-Object Name, Version, Author
This command lists the names, versions, and authors of all installed modules, giving you a richer context about what you have available.
Using `Export-ModuleMember` to Check Availability
For a more programmatic approach, you can use an if-statement to check if a module is installed. This is useful in scripts where you may need to handle conditions based on the presence of a module. Consider the following snippet:
if (Get-Module -ListAvailable | Where-Object {$_.Name -eq "ModuleName"}) {
"Module is installed"
} else {
"Module is not installed"
}
This simple check will provide a clear output indicating whether the specified module is installed or not.
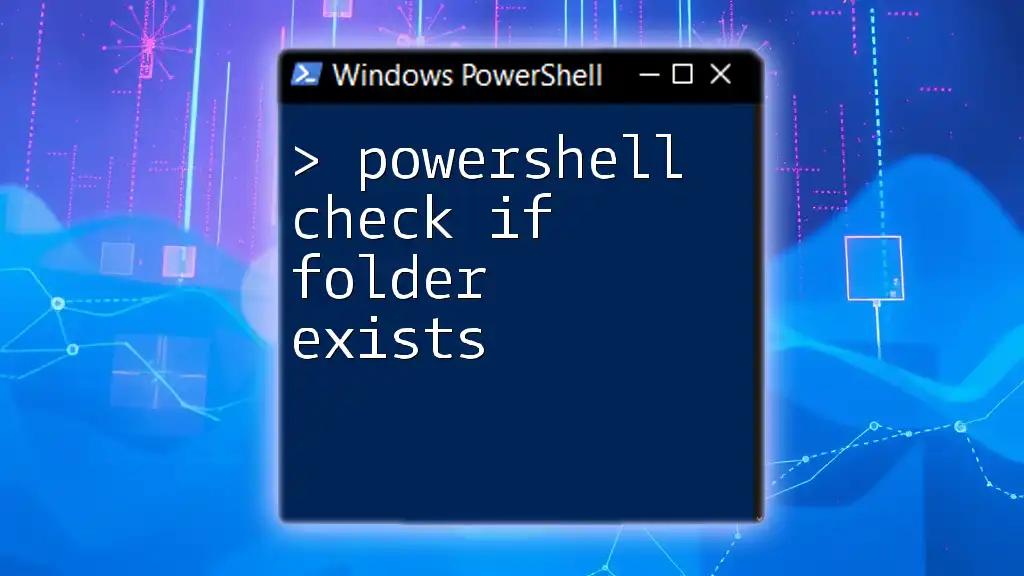
Handling Missing Modules
How to Install Missing Modules
If you determine that a necessary module is missing, you can easily install it using the `Install-Module` cmdlet. This command caters to modules available in the PowerShell Gallery, a repository of PowerShell content. Here’s an example of how to install a module:
Install-Module -Name "ModuleName"
Running this command will prompt you for confirmation if it is the first time you are installing from the Gallery, ensuring that you agree to any license terms.
Troubleshooting Common Issues
When working with PowerShell modules, you might encounter some common issues:
- Permissions Issues: Ensure that you have administrative rights if you're trying to install modules or write to certain system directories.
- Network Connectivity Issues: If you cannot access the PowerShell Gallery, check your internet connection and any firewall settings that might be blocking access.
- Module Dependencies: Some modules depend on others. If a module fails to install, check for any prerequisites.

Conclusion
In summary, the ability to check if a module is installed in PowerShell is invaluable for effective system management. Whether using `Get-Module`, filtering results, or checking versions, PowerShell provides a robust suite of tools for module management. By understanding how to leverage these commands, you enhance your workflow, ensuring that you always have the necessary modules at your disposal.
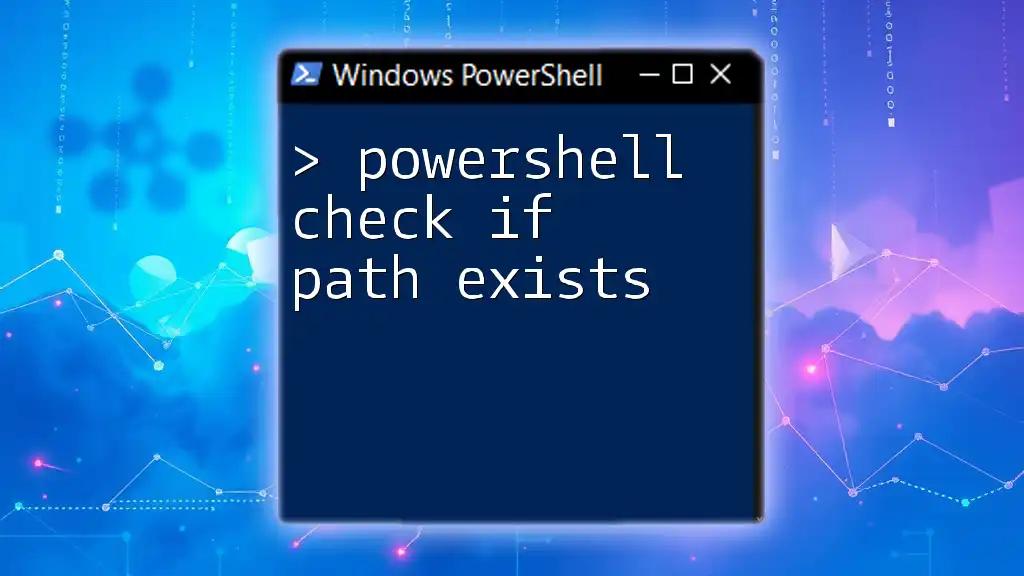
Additional Resources
For further reading and to deepen your understanding of PowerShell modules, consult the official Microsoft documentation, explore third-party resources, and engage with community forums dedicated to PowerShell. They can provide a wealth of information and support as you navigate the world of PowerShell scripting and automation.