A PowerShell module builder allows you to create reusable collections of functions, cmdlets, and variables that can be easily shared and imported into your PowerShell environment.
Here’s a code snippet demonstrating how to create a simple PowerShell module:
# Save this code as MyModule.psm1
function Get-Greeting {
param (
[string]$Name = "World"
)
Write-Host "Hello, $Name!"
}
To use the module, you would import it in your PowerShell session with:
Import-Module 'Path\To\MyModule.psm1'
Get-Greeting -Name 'Student' # Output: Hello, Student!
Understanding PowerShell Modules
What is a PowerShell Module?
PowerShell modules are collections of related functionalities packed together for easy distribution, sharing, and reusability. Modularity allows you to organize your functions, cmdlets, and resources, making your code cleaner and easier to maintain. Instead of writing long scripts, you can create specific modules that serve distinct purposes, keeping your workflow efficient.
Types of PowerShell Modules
Binary Modules
Binary modules are compiled and packaged as dynamic link libraries (DLLs). They are primarily written in languages like C# or VB.NET and are useful when performance is crucial or when leveraging .NET libraries. These modules allow complex operations while maintaining high performance, making them ideal for heavy computational tasks.
Script Modules
Script modules are easier to create and are typically saved with the `.psm1` extension. They consist of PowerShell script files that include functions and variables. These modules provide a lightweight approach to encapsulating code. A script module is handy for developers focusing on quick script-based automation without the need for external compilation.
Manifest Files
A module manifest file, with the `.psd1` extension, is a hash table that stores metadata about the module. This file provides essential information to users and PowerShell itself, such as module version, authorship, and dependencies. Having a manifest is crucial for maintaining version control and ensuring proper loading of your module.
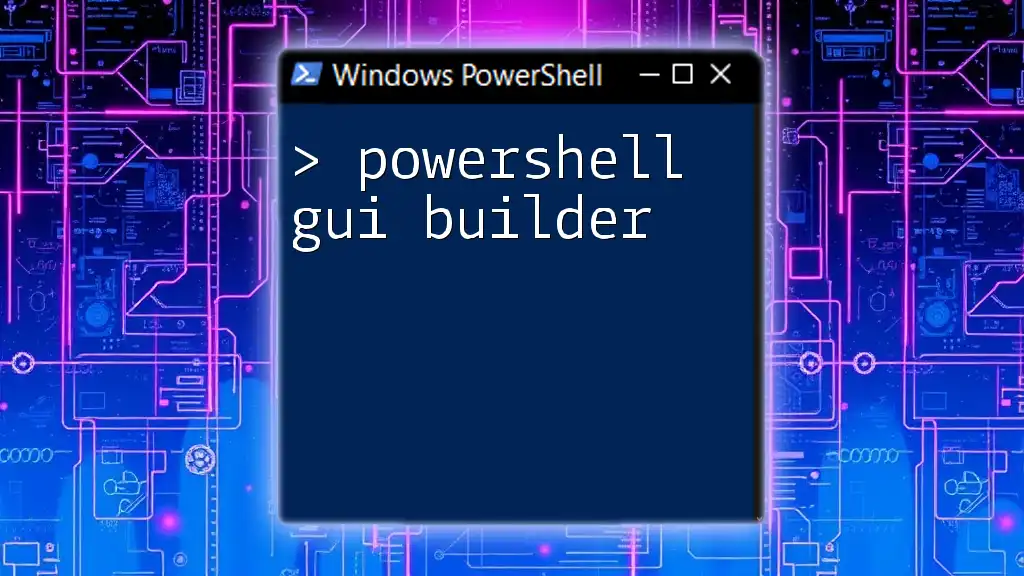
Setting Up Your Environment
Prerequisites
Before you dive into building a PowerShell module, ensure you have the latest version of PowerShell installed on your machine. You can download it from the official [PowerShell GitHub](https://github.com/PowerShell/PowerShell) page.
Integrated Development Environment (IDE) Options
While you can write PowerShell code in any text editor, using an Integrated Development Environment (IDE) like Visual Studio Code can significantly enhance your productivity. It offers features like syntax highlighting, IntelliSense, and debugging tools which simplify the coding process.
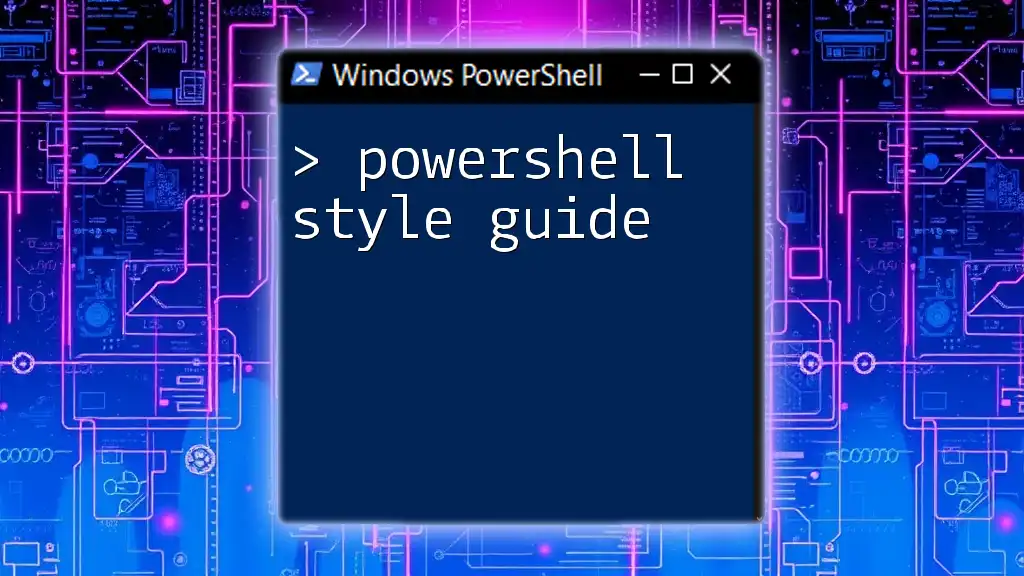
Creating Your First PowerShell Module
Step-by-Step Guide to Building a Module
Step 1: Plan Your Module
Before jumping into coding, it's vital to define the purpose of your module clearly. Ask yourself what functionality you want to offer. This planning stage helps in organizing your code and aligning it with specific use cases.
Step 2: Create Your Module Structure
A well-structured module facilitates easier maintenance and updates. A suggested folder structure might look like this:
MyModule/
|- MyModule.psm1
|- MyModule.psd1
|- Functions/
|- Resources/
Organizing your functions and resources into separate subfolders makes your module cleaner and more manageable.
Step 3: Writing Functions
With your module structure in place, it's time to write the functions. Functions encapsulate reusable code blocks. For instance:
function Get-Hello {
param (
[string]$Name = "World"
)
Write-Output "Hello, $Name!"
}
This simple function demonstrates taking a parameter and outputting a greeting. You can enhance your functions further by adding error handling and validation to improve their robustness.
Step 4: Defining the Module Manifest
A manifest file is essential to describe your module comprehensively. Here's a minimalistic example of what the `.psd1` file might include:
@{
ModuleVersion = '1.0'
GUID = '12345678-1234-1234-1234-123456789012'
Author = 'Your Name'
Description = 'A module for greeting purposes'
}
The GUID is unique to your module, ensuring there's no conflict with other modules. Including a description and version helps users understand your module's purpose and its evolution.
Step 5: Exporting Functions
When you build a module, you might want some functions to be publicly accessible while keeping others private. You can specify which functions are exported using the `Export-ModuleMember` cmdlet:
Export-ModuleMember -Function Get-Hello
This choice allows you to maintain cleaner code while emphasizing the core functionalities your module offers.
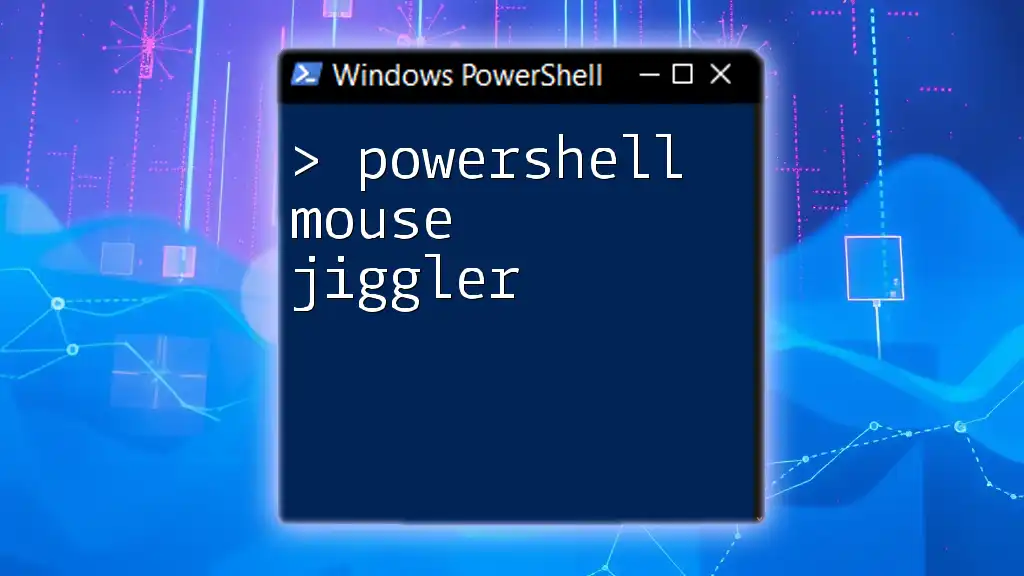
Testing Your Module
Running the Module
After defining your module and functions, it's time to test. Import your module into your PowerShell session using the `Import-Module` command:
Import-Module ./MyModule/MyModule.psm1
Get-Hello -Name "PowerShell"
This command should return "Hello, PowerShell!" if everything is correctly set up. Testing your functions regularly ensures they behave as expected.
Debugging Common Issues
Despite best efforts, you may encounter errors while testing your module. Common pitfalls include incorrect paths, missing dependencies, or syntax errors. Always read the error messages carefully; they often point you directly to the issue. Utilize the PowerShell ISE or Visual Studio Code's debugging features to step through your code and identify problems systematically.
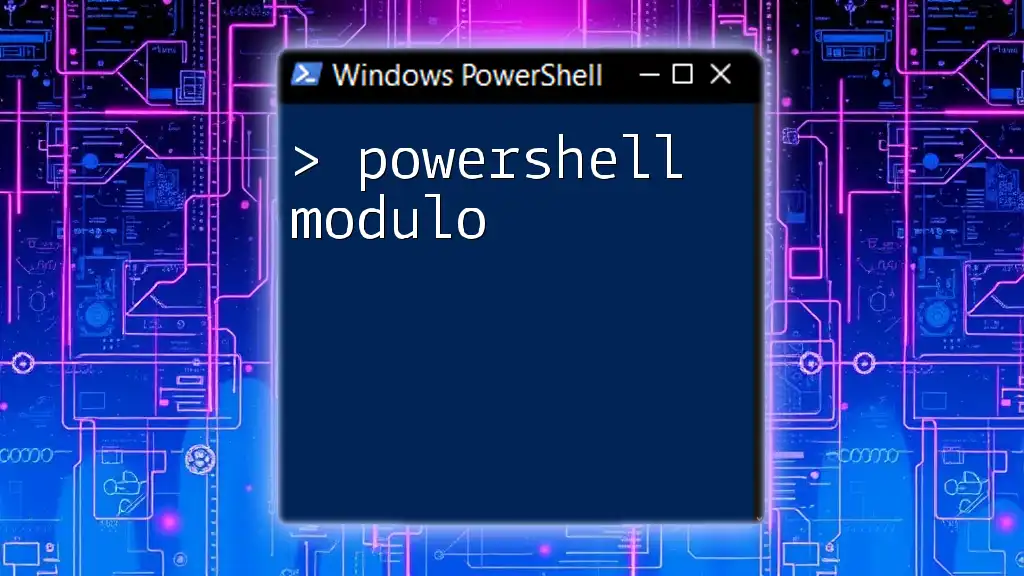
Publishing Your PowerShell Module
Best Practices for Publishing
Once your module is ready for public use, you can publish it on platforms like the PowerShell Gallery. This platform makes sharing easy and allows users to install your module with simple commands. Include clear documentation to help users understand how to use your module effectively.
Version Control
Using a version control system like Git is highly recommended for managing your module’s changes. It allows you to track modifications and collaborate with others efficiently. When you make changes to your module, tag your commits and maintain a changelog to inform users about new features or bug fixes.
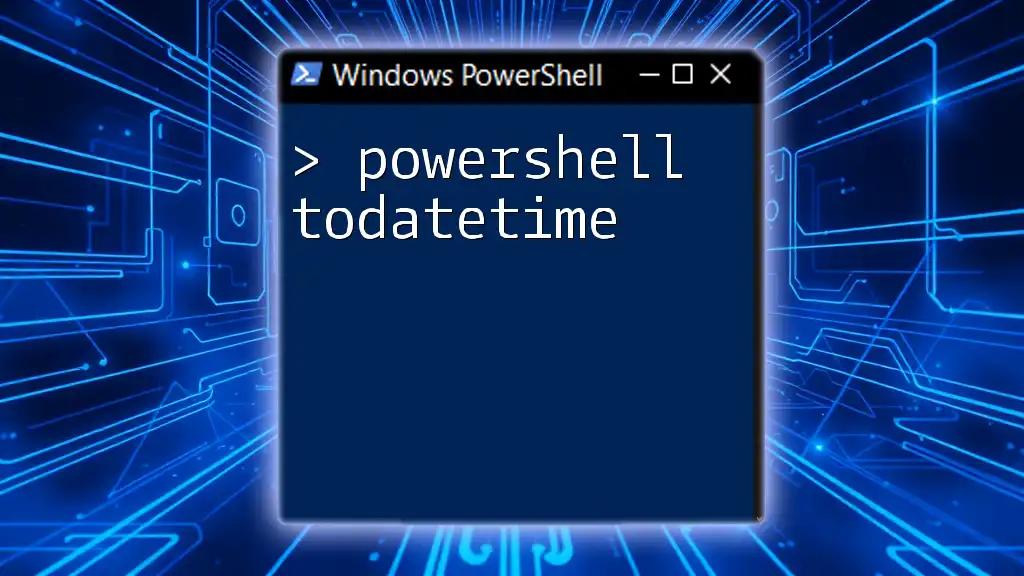
Maintaining Your PowerShell Module
Updating Your Module
As your module evolves, update it with new features or improvements. Applying semantic versioning helps users understand the significance of updates – whether they include new features, bug fixes, or breaking changes.
Gathering Feedback and Iterating
Encourage users to provide feedback on your module. Whether through forums or comments, user input is invaluable for understanding the real-world use cases of your module. Regularly iterate based on this feedback to improve your offerings continuously.
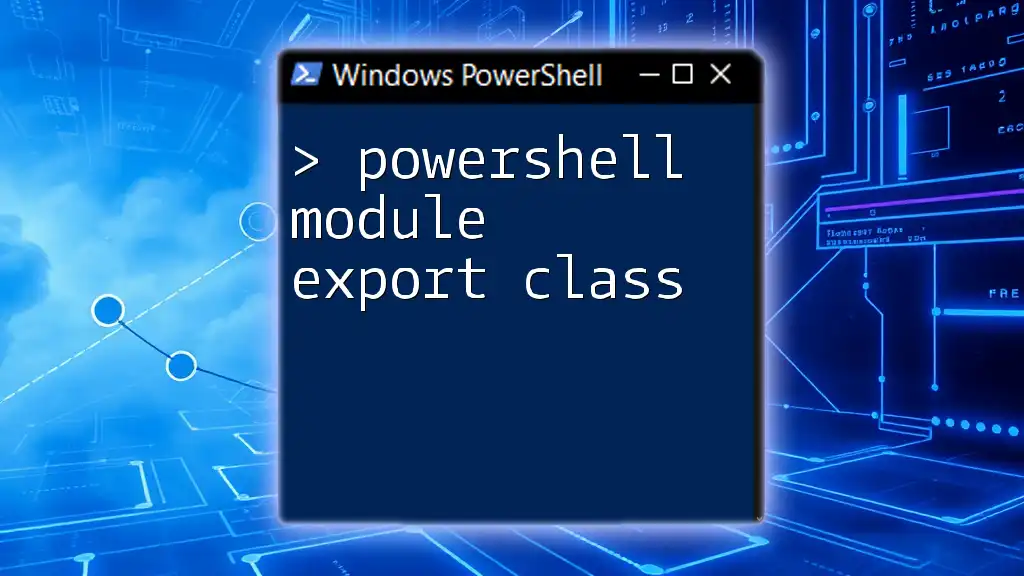
Conclusion
Creating a PowerShell module is a rewarding endeavor that promotes code organization, reusability, and community sharing. By understanding the types of modules, planning your project thoroughly, and following best practices, you can become a proficient PowerShell module builder. Remember to keep learning, engaging with the community, and iterating on your designs, ensuring your modules remain relevant and impactful.