The `Export-ModuleMember` cmdlet in PowerShell is used within a module to specify which functions, variables, or cmdlets are accessible to users of the module, thereby controlling the module's public interface.
Here’s a simple example:
function Get-Greeting {
param (
[string]$Name
)
"Hello, $Name!"
}
# Export the Get-Greeting function
Export-ModuleMember -Function Get-Greeting
Understanding PowerShell Modules
What is a PowerShell Module?
A PowerShell module is a collection of related PowerShell functions, cmdlets, and resources that are bundled together for ease of use. They serve the purpose of encapsulating functionality into reusable units, making it easier to manage complex scripts and applications in a modular fashion. There are three main types of PowerShell modules:
- Script modules: These consist of PowerShell scripts (.psm1 files) and are the most common type.
- Binary modules: These are compiled .NET assemblies that provide a richer set of functionalities through API calls.
- Manifest modules: These use a module manifest file (.psd1) to describe the module and its contents, enhancing the description and versioning of your code.
Modules provide significant benefits, such as code reusability, maintainability, and the ability to share code easily across different scripts and environments.
Structure of a PowerShell Module
To create a structured and organized module, it’s essential to understand its components. A typical module might include a module manifest file, which contains metadata about the module, as well as your PowerShell scripts. The manifest file plays a crucial role in ensuring that all dependencies are acknowledged and tracked, thus preventing runtime errors.
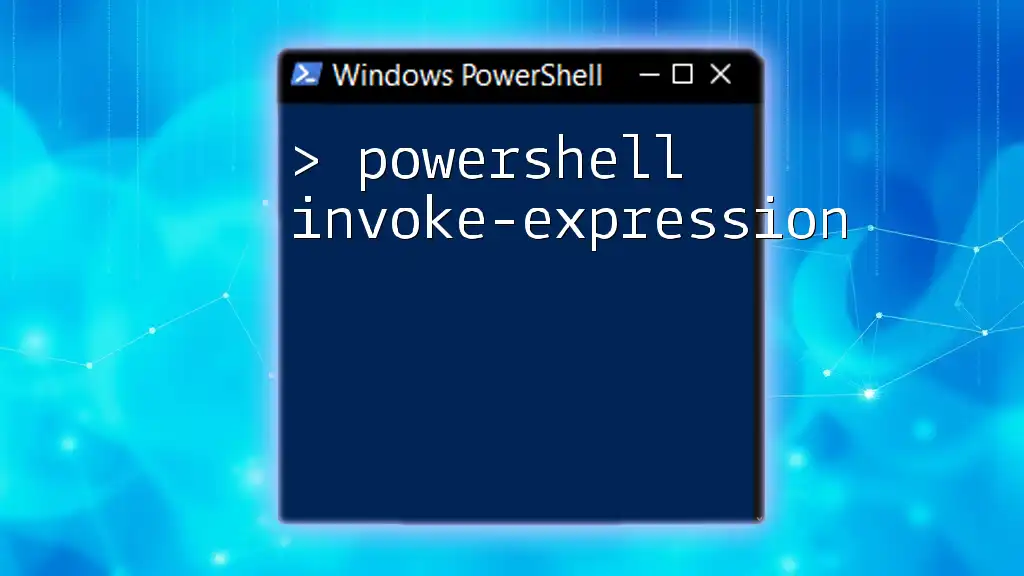
Introduction to Export Classes
What is an Export Class in PowerShell?
An export class in PowerShell refers to a class that is explicitly defined within a module and intended for use by users of that module. This concept arises from object-oriented programming, allowing you to define a blueprint for creating objects with specific attributes and methods.
Why Use Export Classes?
Using export classes in your modules provides several advantages:
- Encapsulation: Group related functions and data together, promoting better organization of your code.
- Simplicity: Users can interact with a single object rather than needing to understand many individual functions.
In short, export classes help streamline the user experience while also maintaining a clear code structure internally.
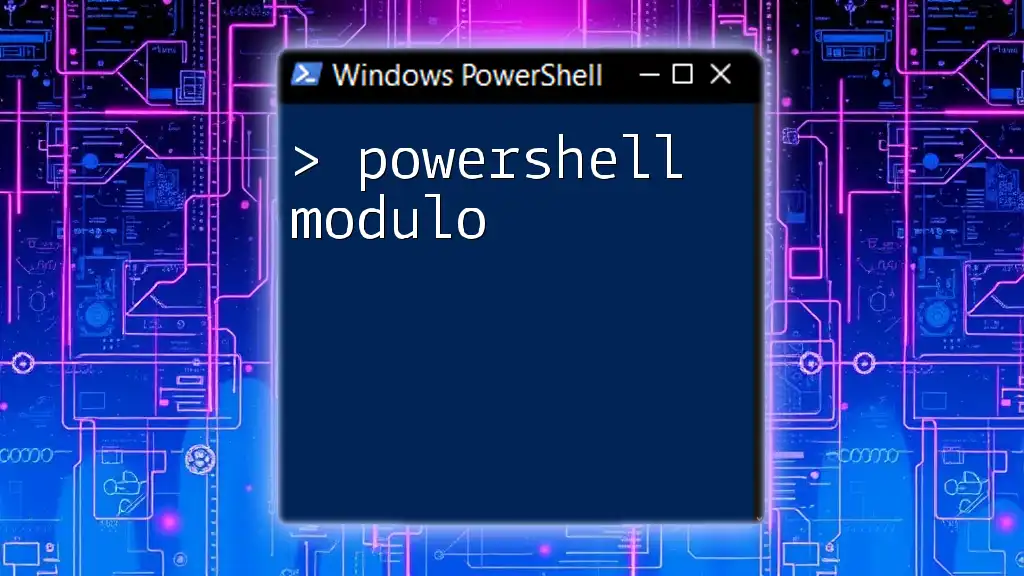
Creating a PowerShell Module with Export Class
Setting Up Your Development Environment
Before diving into module creation, ensure that you have PowerShell installed on your system. For Windows users, PowerShell comes pre-installed, while Mac and Linux users can install it from the official PowerShell GitHub page.
Defining Your Module
To create a new module, start by creating a folder that will hold your module files. Inside this folder, you will create a PowerShell script file and a module manifest file:
- Create a folder: Name it appropriately for your module, e.g., `MyModule`.
- Create the module manifest file: This can be accomplished using the `New-ModuleManifest` cmdlet and will create a `.psd1` file where you can specify metadata such as the module version, author, and description.
Implementing the Export Class
Declaring the Export Class
Now, you’ll declare your export class in the `.psm1` file of your module. The syntax in PowerShell for declaring a class is straightforward. Here’s a simple example of an export class:
class MySampleClass {
[string]$Name
MySampleClass([string]$name) {
$this.Name = $name
}
[void] Greet() {
Write-Host "Hello, $($this.Name)!"
}
}
In this example, we’re defining a class `MySampleClass` that includes a property `$Name` and a method `Greet()`. The class constructor initializes the name when a new object is created.
Exporting the Class in the Module
Once you have defined your class, you need to make it available for use outside of the module. This is done by using the `Export-ModuleMember` cmdlet:
Export-ModuleMember -Function MySampleClass
This line will ensure that users of your module can access `MySampleClass` when they import the module.
Utilizing the Export Class from Other Modules
After exporting your class, users can load your module and instantiate the class as shown below:
Import-Module MyModule
$sample = [MySampleClass]::new("Alice")
$sample.Greet()
In this snippet, the user imports `MyModule`, creates a new instance of `MySampleClass`, and calls the `Greet` method, resulting in a personalized greeting.
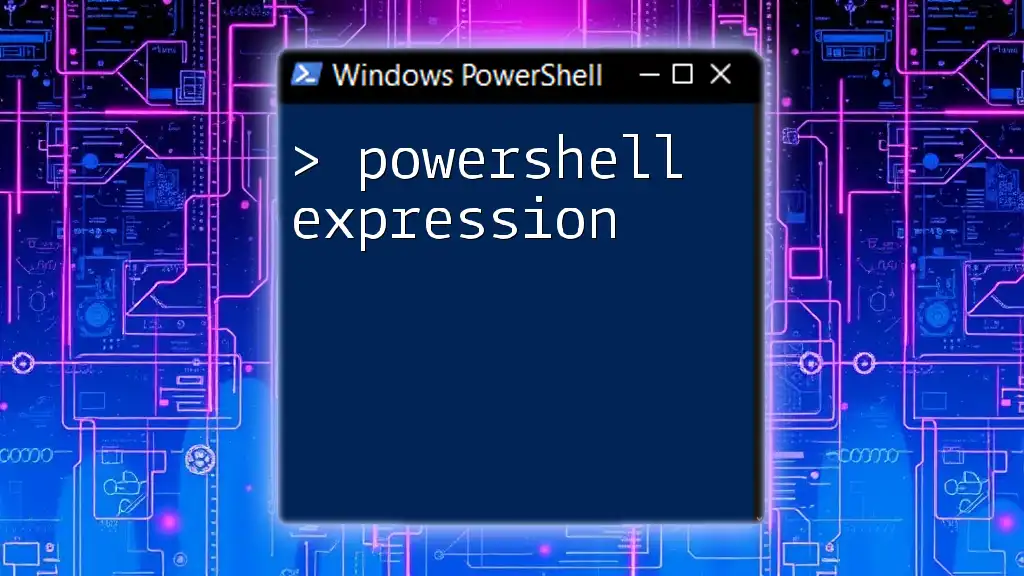
Best Practices for Using Export Classes
Structuring Your Code for Readability
When designing your module, structure your code for clarity and maintainability. Follow consistent naming conventions for your classes, methods, and properties. Comment your code to clarify complex logic, making it easier for both you and others to follow along in the future.
An exemplary module layout could be:
MyModule/
├── MyModule.psd1
├── MyModule.psm1
└── MyExportClass.ps1
Ensuring Reusability and Maintenance
Writing modular code is essential not only for maintenance but also for future enhancements. As you add or improve functionalities, consider how changes can impact existing code. Version your modules effectively by updating the version number in your manifest file every time you make significant changes to the module.
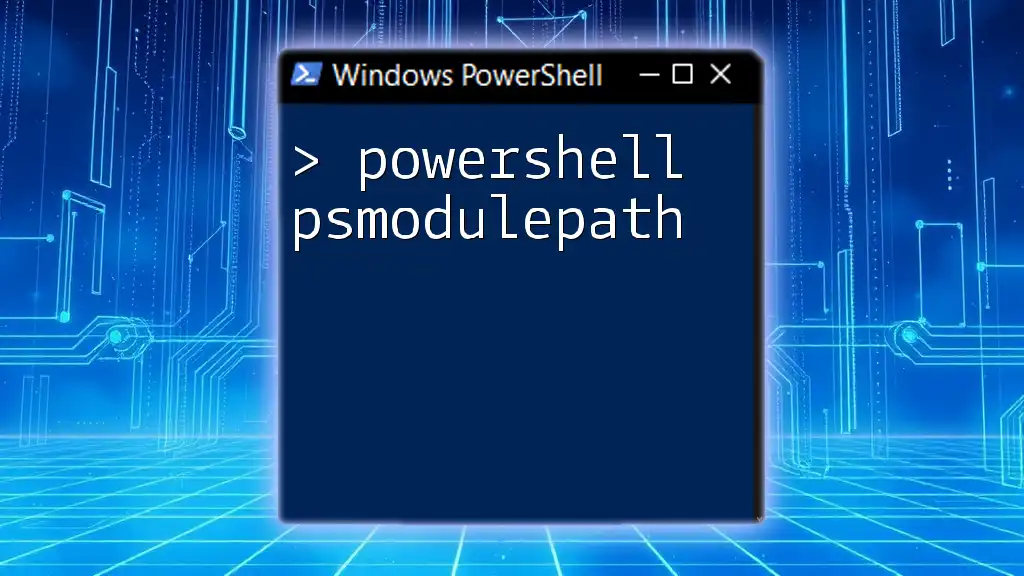
Troubleshooting Common Issues
Common Errors when Exporting Classes
When working with PowerShell module export classes, you might encounter typical pitfalls. Common errors include:
- Forgetting to use `Export-ModuleMember`, resulting in users being unable to access your classes.
- Namespace conflicts if multiple modules define classes with the same name. Use unique class names to mitigate this.
Debugging Techniques
To troubleshoot and debug your module, you can enhance the error handling in your classes. Utilize `try-catch` blocks, making it easier to identify potential issues. Moreover, PowerShell has built-in debugging tools such as breakpoints and the `Set-PSBreakpoint` cmdlet that help trace through the execution flow.
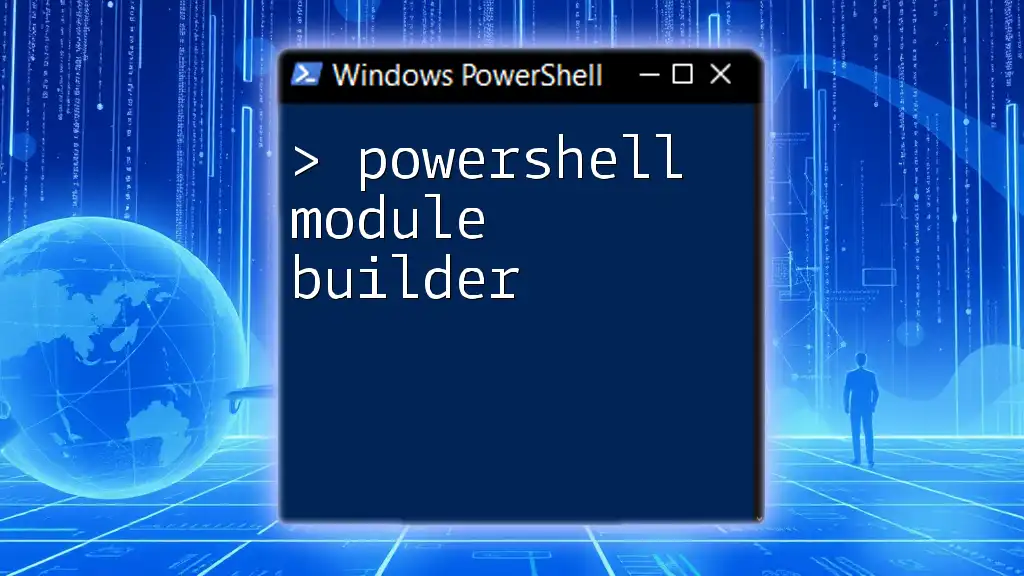
Conclusion
In summary, understanding how to create a PowerShell module export class is a crucial skill for any PowerShell user looking to enhance their scripts and modules. Through careful structuring and method implementation, you can develop robust code that promotes ease of use and maintenance. With these best practices and foundational knowledge, you’re well-prepared to get started on creating your own PowerShell modules and export classes!
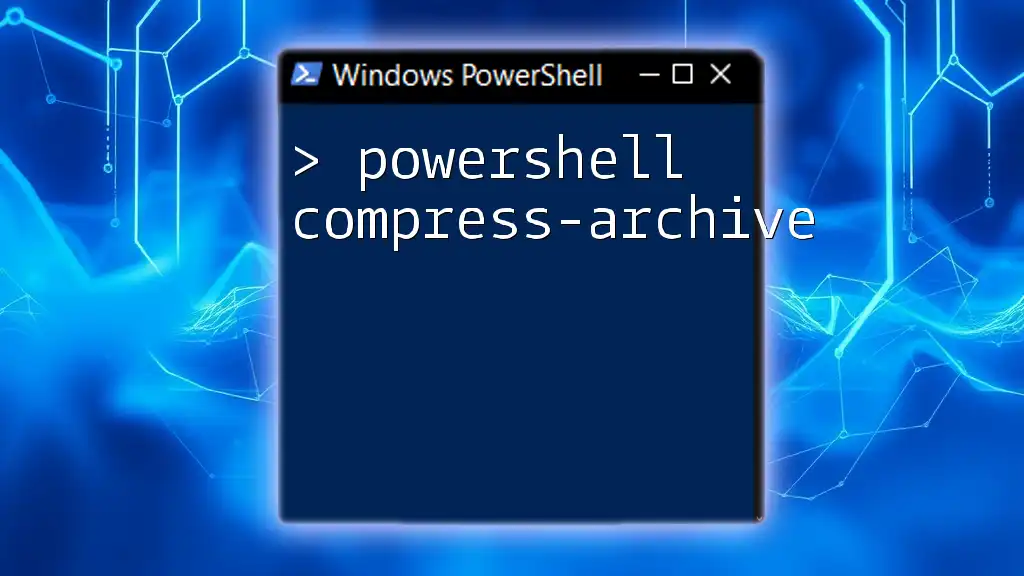
Additional Resources
As you continue your journey into PowerShell module development, consider referencing the official PowerShell documentation and engaging with community forums for additional support and ideas. Happy coding!