In PowerShell, the `Import-Csv` cmdlet is used to read data from a CSV file and convert it into a series of PowerShell objects for easy manipulation and analysis.
$csvData = Import-Csv -Path 'C:\Path\To\Your\File.csv'
Understanding CSV Format
What is a CSV File?
A CSV (Comma-Separated Values) file is a simple text format used to represent tabular data. This file format organizes data in rows and columns, with each value separated by a comma. While the name suggests commas, delimiters can also be semicolons or tabs. CSV files are widely used due to their simplicity and compatibility with numerous applications, particularly spreadsheets and databases.
Common use cases for CSV files include data export/import between applications, data storage for simple database entries, and configuration management—making CSV files an integral part of data management processes.
Why Use PowerShell for CSV Manipulation?
PowerShell streamlines the manipulation of CSV files by providing built-in cmdlets like `Import-Csv` and `Export-Csv`. Automating repetitive tasks with PowerShell not only saves time but also ensures consistency and reduces human error. By integrating PowerShell scripts with existing systems, you can effectively manage and transform data across different environments.

Getting Started with Importing CSV in PowerShell
Prerequisites
Before diving into CSV importation, ensure that you have the correct version of PowerShell installed. For optimal functionality, using Windows PowerShell 5.1 or later is recommended. Administrative permissions might also be necessary to interact with specific files or directories, depending on your organizational policies.
Basic Syntax of Importing CSV
To import a CSV file in PowerShell, you will mainly utilize the `Import-Csv` cmdlet. The general structure is as follows:
Import-Csv -Path "yourfile.csv"
This command fetches the contents of the specified CSV file and converts it into PowerShell objects, allowing you to manipulate and analyze the data effectively.
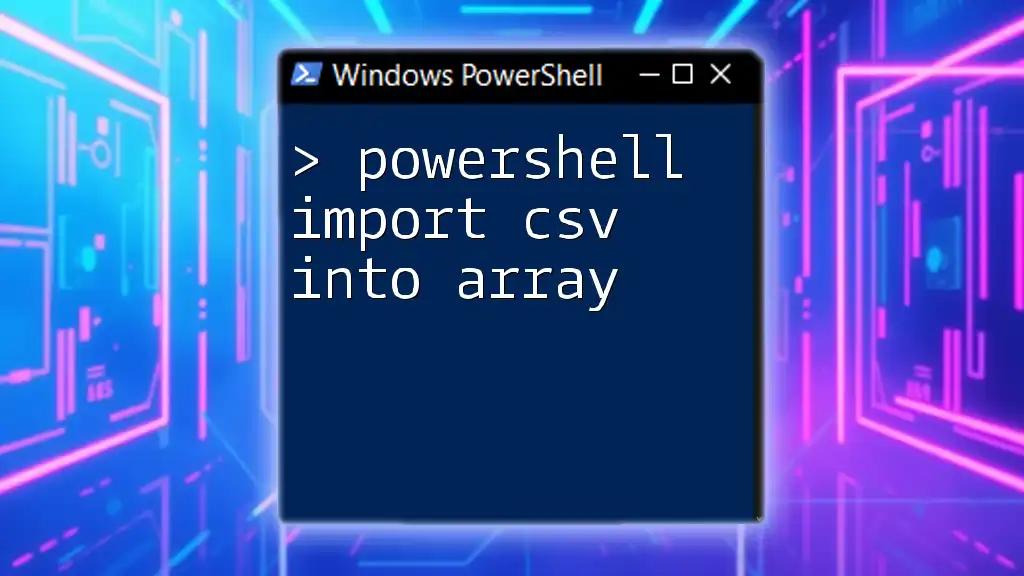
Importing CSV Files
Using Import-Csv Cmdlet
Once you are ready to proceed, the `Import-Csv` cmdlet becomes your primary tool. To use it, simply refer to the full path of the CSV file you wish to import:
$data = Import-Csv -Path "C:\data\sample.csv"
This command assigns the imported data to the variable `$data`, allowing you to work with the table-like structure of the CSV within PowerShell.
Understanding the Output
When you import a CSV file, each row turns into a PowerShell object, with the headers of your CSV file becoming the properties of that object. This means you can access the data using property names directly:
foreach ($row in $data) {
Write-Output $row.ColumnName
}
In this example, replace `ColumnName` with the actual header name of the column you want to access.
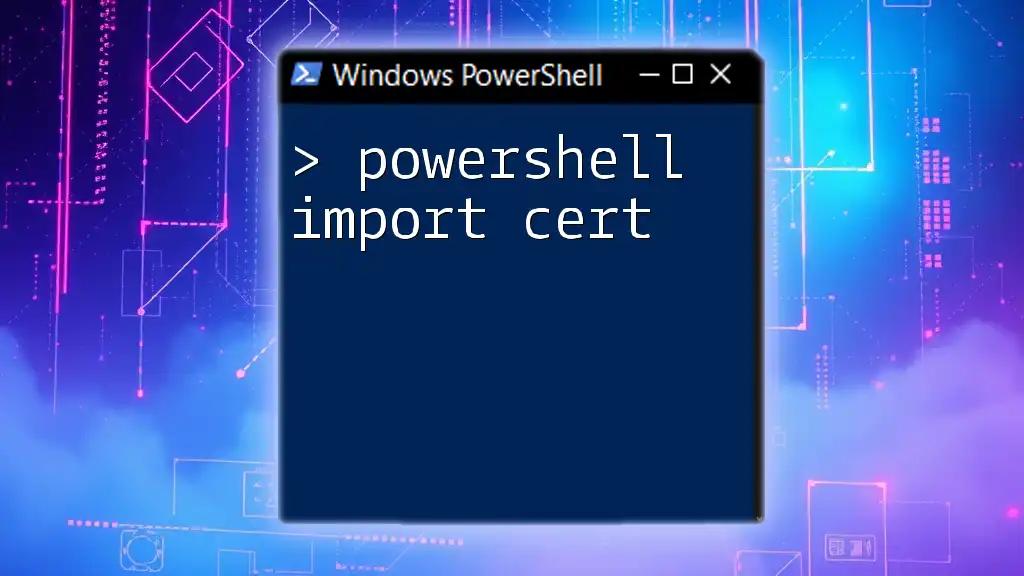
Advanced Usage of Import-Csv
Specifying Delimiters
While CSV files typically use commas, you may encounter files with different delimiters (such as tabs or semicolons). PowerShell allows you to specify a custom delimiter using the `-Delimiter` parameter. For instance, if dealing with a TSV (Tab-Separated Values) file, you can use:
$data = Import-Csv -Path "C:\data\sample.tsv" -Delimiter "`t"
This command informs PowerShell to interpret the TSV file appropriately.
Working with Headers
In some cases, a CSV file may lack headers. You can address this by specifying custom headers using the `-Header` parameter. Here’s how you can replace the default behavior:
$data = Import-Csv -Path "C:\data\noheader.csv" -Header "Column1", "Column2", "Column3"
In this example, three headers are assigned to the imported data, enabling you to manipulate the data according to your schema.
Filtering Data During Import
You might want to only work with a subset of your data immediately after import. Harnessing the `Where-Object` cmdlet allows you to filter the imported data efficiently. For example:
$filteredData = Import-Csv -Path "C:\data\sample.csv" | Where-Object { $_.Age -gt 30 }
This command filters the imported data, retaining only those entries where the Age field is greater than 30.
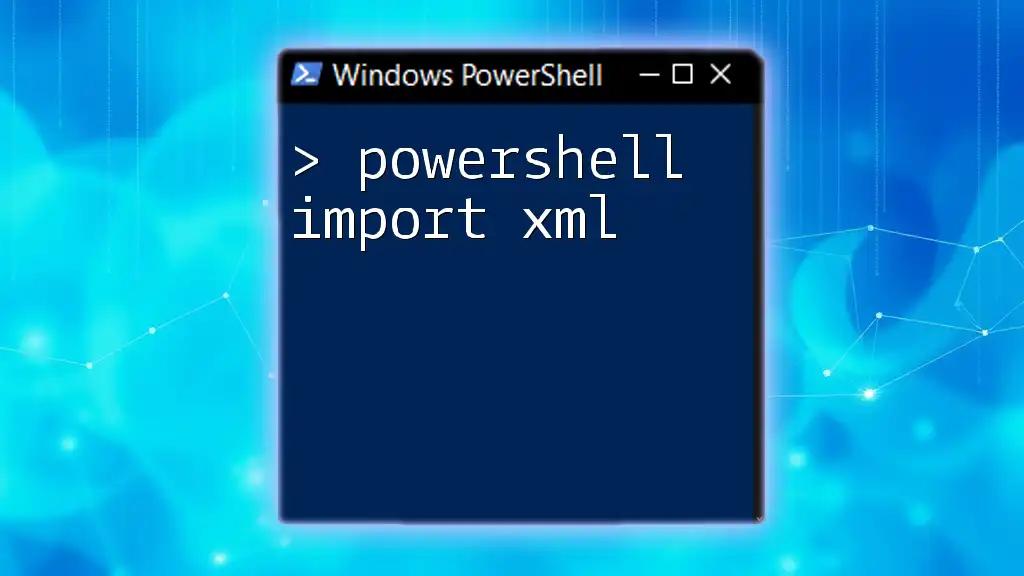
Manipulating Imported Data
Accessing Columns by Name
Once your data is imported and stored as objects, you can access specific columns easily. Here’s an example of accessing and displaying the "Name" column:
foreach ($row in $data) {
Write-Output $row.Name
}
This iterates through each row and prints the values of the "Name" column to the console.
Modifying Imported Data
Manipulating the data to fit your needs is straightforward. Suppose you want to increment Age by one year for each record; you can do so with:
foreach ($row in $data) {
$row.Age = $row.Age + 1
}
This updates the Age property in each object within your data collection.
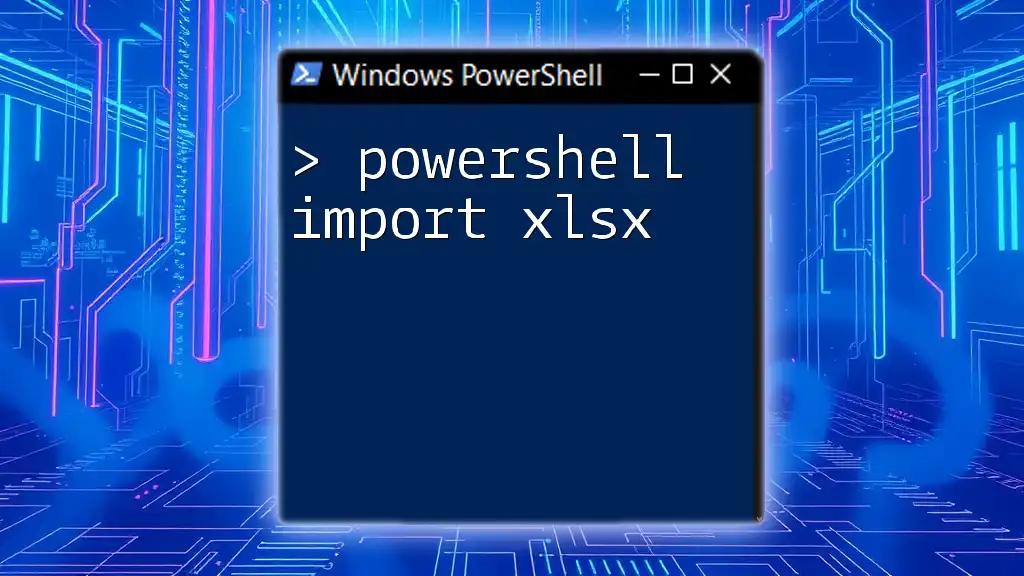
Exporting Modified Data Back to CSV
Using Export-Csv Cmdlet
After working with your data, you may need to export it back to a CSV file. The `Export-Csv` cmdlet is utilized for this purpose. The basic syntax for exporting data is as follows:
$data | Export-Csv -Path "C:\data\modified.csv" -NoTypeInformation
In this example, the data is piped into `Export-Csv`, creating a new file called `modified.csv`.
Best Practices for Exporting
When exporting, pay attention to how quotations and delimiters are handled. By using the `-NoTypeInformation` parameter, you avoid unnecessary type metadata being added as the first line in your output file.
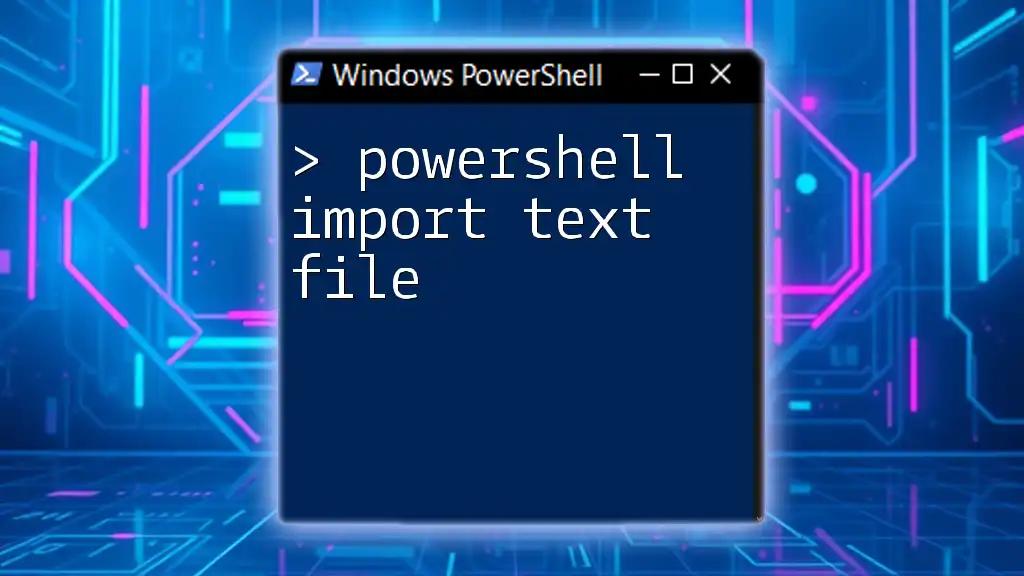
Real-World Applications
Automating Report Generation
One useful application of the `Import-Csv` command is producing automated reports. For instance, if you regularly receive a monthly CSV report, you can craft a PowerShell script that imports the file, processes the data, and sends an email summary to stakeholders—all without manual intervention.
Data Migration Tasks
Moving data between systems is another vital use case for PowerShell and CSV. When migrating records from an old system to a new one, you can import the existing CSV data, transform it as needed, and then export it in the format necessary for the new system.

Troubleshooting Common Issues
Common Errors and How to Fix Them
When working with `Import-Csv`, you might encounter common issues such as file-not-found errors. Ensure the file path is correct and check permissions if necessary. Other issues may arise from unexpected data formats; verify that your CSV is formatted correctly.
Using PowerShell Debugging Techniques
If you face script errors, employing debugging techniques can be invaluable. Utilize `Write-Debug` or `Write-Host` to track variable values and flow through your script. These methods can help identify where a problem lies in your data processing.
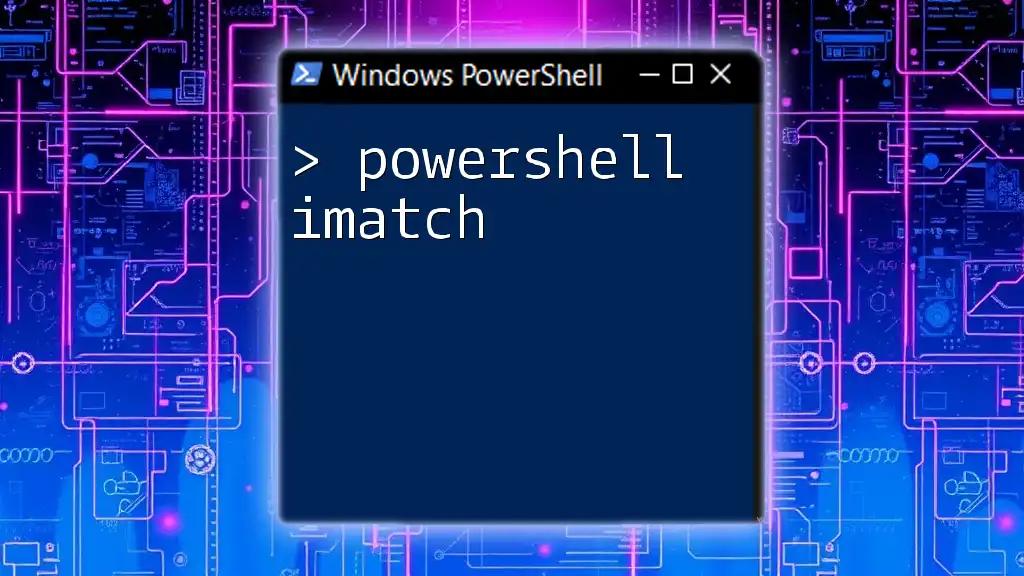
Conclusion
Mastering the `Import-Csv` command in PowerShell equips you with a powerful tool for data management. By leveraging this functionality, you can efficiently manipulate, analyze, and report on data, significantly enhancing your productivity and streamlining your workflows.
Take the time to practice these commands, experiment with different datasets, and develop your PowerShell skills further. By doing so, you will unlock countless possibilities in data handling and automation within your work environment.
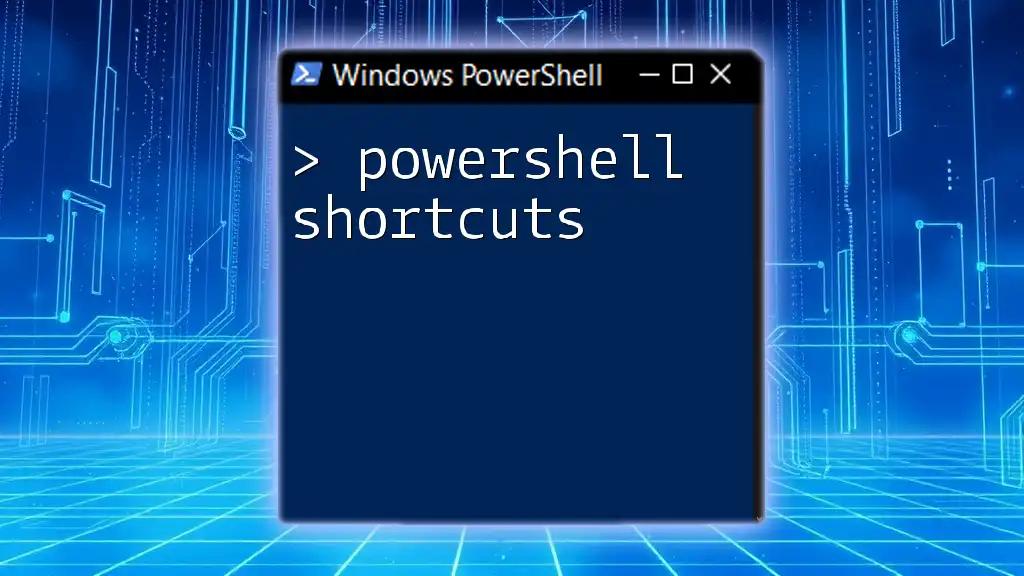
Additional Resources
For those looking to deepen their understanding of PowerShell and CSV manipulation, consider exploring the official Microsoft documentation on `Import-Csv`, as well as engaging with PowerShell community forums. Keep an eye out for upcoming workshops or tutorials that dive deeper into the intricacies of PowerShell scripting for more advanced learning opportunities.