In PowerShell, the `Import-Csv` cmdlet is used to read a CSV file and the `ForEach-Object` cmdlet allows you to iterate through each row of the imported data, enabling efficient processing of each record.
Here’s a basic example:
Import-Csv -Path 'path\to\your\file.csv' | ForEach-Object { Write-Host $_.ColumnName }
Make sure to replace `ColumnName` with the actual header name of the column you want to process.
Understanding CSV and PowerShell
What is a CSV File?
CSV, or Comma-Separated Values, is a simple file format used to store tabular data, such as spreadsheets or databases. The data in a CSV file is structured as lines of text, where each line corresponds to a record, and each record consists of fields separated by commas. This format is commonly used for data exchange across different applications, making it an essential component when handling large data sets.
Why Use PowerShell for CSV Import?
PowerShell offers robust capabilities for automation and data manipulation, making it an ideal choice for managing CSV files. The `Import-Csv` cmdlet allows users to quickly read data from a CSV file and converts it into PowerShell objects. This enables easy access to individual records and fields for further manipulation. By using PowerShell, you can automate repetitive tasks, saving valuable time and minimizing the potential for human error.
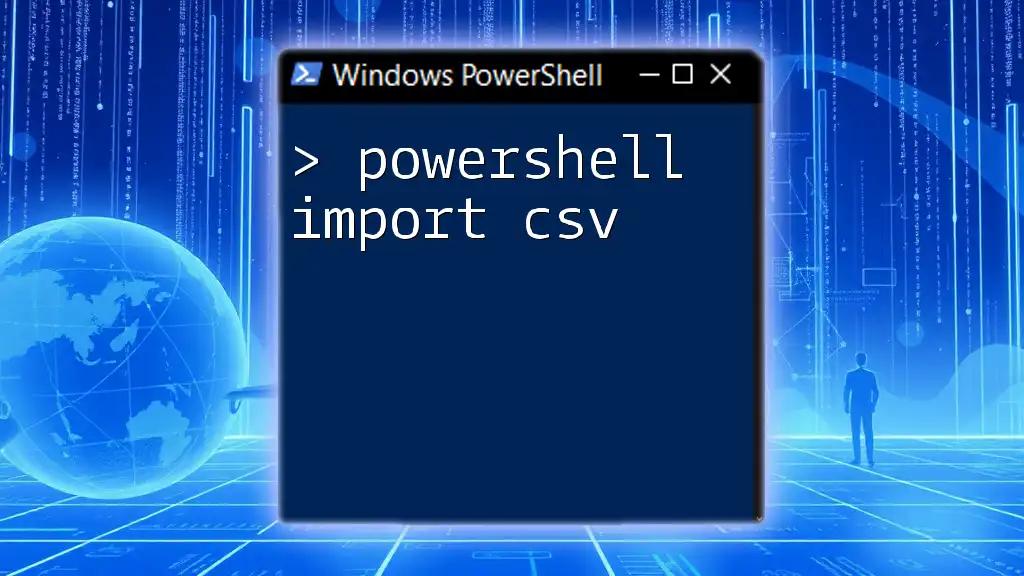
Getting Started with Import-Csv
How to Use Import-Csv in PowerShell
The `Import-Csv` cmdlet is straightforward to implement. Here’s the basic syntax to import data from a CSV file:
Import-Csv -Path 'path\to\your\file.csv'
In this command, replace `'path\to\your\file.csv'` with the actual file path of your CSV file. This command reads the CSV data and outputs it as PowerShell objects.
Basic Example of Import-Csv
Here’s a simple example demonstrating how to import data from a CSV file:
$data = Import-Csv -Path 'C:\Data\example.csv'
$data
This command assigns the imported data to the variable `$data`, which can then be used for further processing. If your CSV contains headers, PowerShell will automatically create properties based on these headers, making it easy to reference specific columns in later commands.
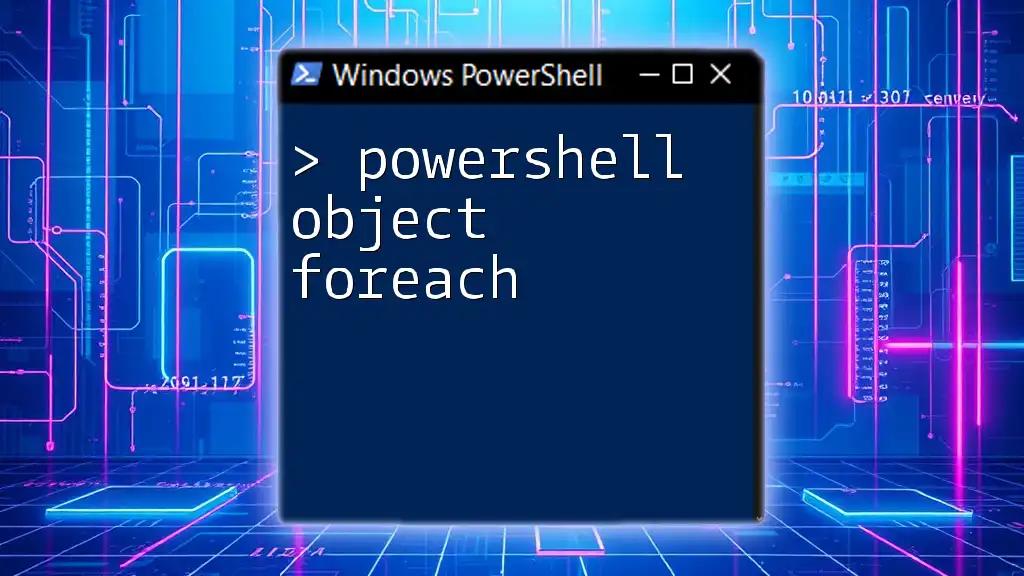
Leveraging ForEach with Import-Csv
Understanding ForEach in PowerShell
The `ForEach` construct in PowerShell is essential for iterating over collections, such as arrays or objects. Using `ForEach` allows you to perform actions on each item in a collection, which is particularly useful when dealing with the records imported from a CSV file.
Using ForEach with Import-Csv
To process each row of a CSV file, you combine `Import-Csv` with `ForEach-Object`. The syntax resembles the following:
Import-Csv -Path 'path\to\file.csv' | ForEach-Object { /* code here */ }
For example, suppose you want to display a message for each entry in your CSV file:
Import-Csv -Path 'C:\Data\example.csv' | ForEach-Object {
Write-Host "Processing: $($_.ColumnName)"
}
In this case, `ColumnName` should be replaced with the actual name of the header in the CSV file. The expression `$($_.ColumnName)` allows you to access the value associated with that column for each record.
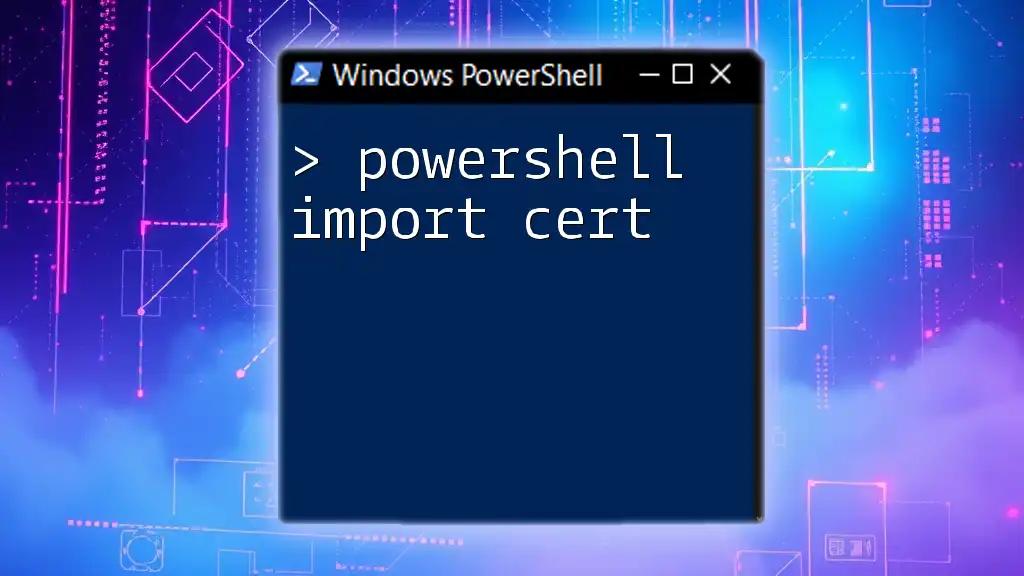
Real-World Applications of Import-Csv and ForEach
Automating Data Processing Tasks
Imagine you have a CSV file containing employee information. You can easily iterate through the records and produce a report:
Import-Csv -Path 'C:\Data\employees.csv' | ForEach-Object {
$name = $_.Name
$title = $_.Title
Write-Host "$name is a $title"
}
This loop not only makes the process faster but also reduces human error, allowing your reports to be generated accurately and swiftly.
Modifying Data within CSV Files
With PowerShell, you can also modify values in the CSV. Here's how you can change the status of all records and export the updated data into a new CSV file:
$data = Import-Csv -Path 'C:\Data\example.csv'
$data | ForEach-Object {
$_.Status = "Processed"
}
$data | Export-Csv -Path 'C:\Data\example_processed.csv' -NoTypeInformation
In this example, every record’s `Status` field is updated to "Processed," and the modified data is exported to a new CSV file. The `-NoTypeInformation` parameter removes additional type metadata from the output file.

Common Use Cases
Importing and Filtering Data
PowerShell allows you to filter records on the fly using `Where-Object`, making it particularly useful for extracting specific data based on conditions. For example, if you want to display only high-value sales from a CSV file, you could use the following command:
Import-Csv -Path 'C:\Data\sales.csv' | Where-Object { $_.Amount -gt 1000 } | ForEach-Object {
Write-Host "High sale: $($_.Product)"
}
This command filters records where the `Amount` field is greater than 1000, providing a way to quickly assess high-value transactions.
Aggregating Data from CSV
If you need to summarize data, PowerShell can group and aggregate entries effectively. For instance, suppose you want to count the number of transactions per category:
Import-Csv -Path 'C:\Data\transactions.csv' |
Group-Object -Property Category |
ForEach-Object {
Write-Host "Category: $($_.Name), Count: $($_.Count)"
}
In this example, `Group-Object` organizes the records by `Category`, and `ForEach-Object` generates a count for each group, offering a clear overview of the transaction volume across categories.

Best Practices for Using Import-Csv and ForEach
Error Handling Best Practices
When working with external files, it's crucial to anticipate potential issues, such as missing files or incorrect formats. Wrapping your CSV import and processing in a `try-catch` block provides a safety net:
try {
Import-Csv -Path 'C:\Data\example.csv' | ForEach-Object {
# Processing code here
}
} catch {
Write-Host "Error: $_"
}
This approach ensures that you handle errors gracefully and outputs a meaningful message if something goes wrong.
Optimizing Performance
When dealing with large CSV files, performance can become an issue. To optimize processing, consider:
- Only importing the necessary columns.
- Limiting the operations performed within the `ForEach` loop to minimize overhead.
- Using filtering before the import to reduce the number of records loaded into memory.
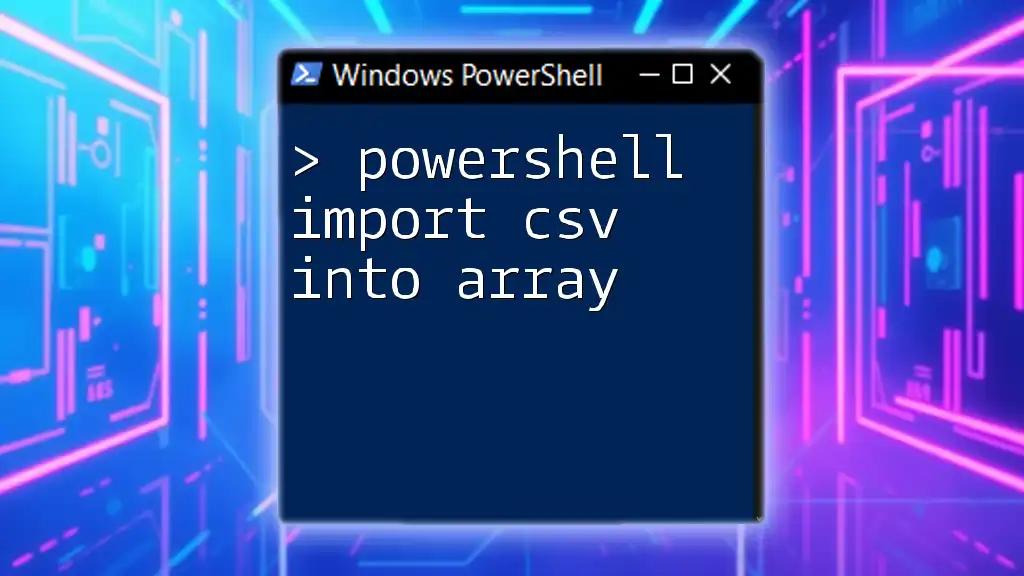
Conclusion
Combining `Import-Csv` with `ForEach` in PowerShell provides powerful tools for managing and manipulating data efficiently. By leveraging these cmdlets, you can automate mundane tasks, perform complex data transformations, and even generate insightful reports. Use the examples and techniques covered in this guide to harness PowerShell's capabilities and streamline your data workflows.