In PowerShell, the pipe (`|`) operator allows you to pass the output of one command directly into another, and using `ForEach-Object` enables iteration over each item in that output.
Here’s a code snippet demonstrating the use of the pipe with `ForEach-Object`:
Get-Process | ForEach-Object { Write-Host "Process Name: $($_.Name)" }
Understanding the Basics of PowerShell Pipe
What is a Pipe in PowerShell?
A pipe, denoted by the symbol `|`, is a powerful feature in PowerShell that allows you to send the output of one command directly into another command as input. This facilitates the creation of complex command sequences by chaining commands together in a more efficient manner.
For example, when you run:
Get-Process | Sort-Object Name
Here, the output of `Get-Process` is being sent to `Sort-Object`, which then sorts the processes by their names.
Benefits of Using Pipes
Utilizing pipes in PowerShell has several advantages:
- Streamlining Commands: By chaining commands, you can accomplish more with less code, improving efficiency.
- Improving Readability: Piping commands can make scripts easier to read and understand at a glance.
- Enhancing Performance: Pipes allow for the direct transfer of data between commands, which can often speed up processing times compared to intermediate variable assignments.
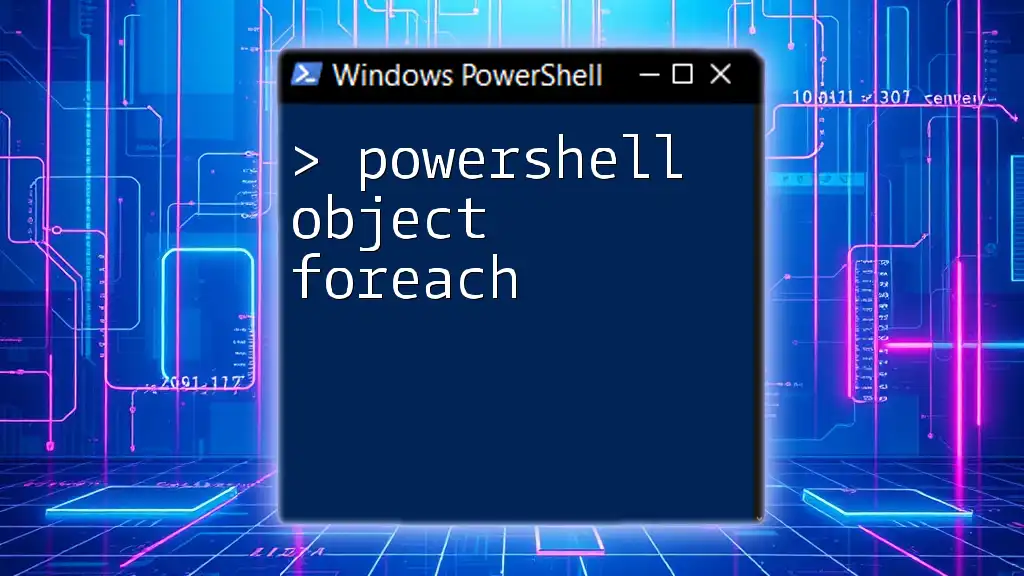
The Foreach Cmdlet in PowerShell
What is the Foreach Cmdlet?
The `Foreach` cmdlet is a looping structure that iterates over a collection, executing a specified block of code for each item in that collection. It is particularly useful for processing arrays and collections of objects.
Syntax of Foreach
The basic syntax of the `Foreach` cmdlet requires a collection to iterate over and a script block containing the actions to perform for each item. It looks like this:
ForEach ($item in $collection) {
# Actions performed on $item
}
In this structure, `$item` represents the current element being processed, allowing you to leverage its properties within the loop.
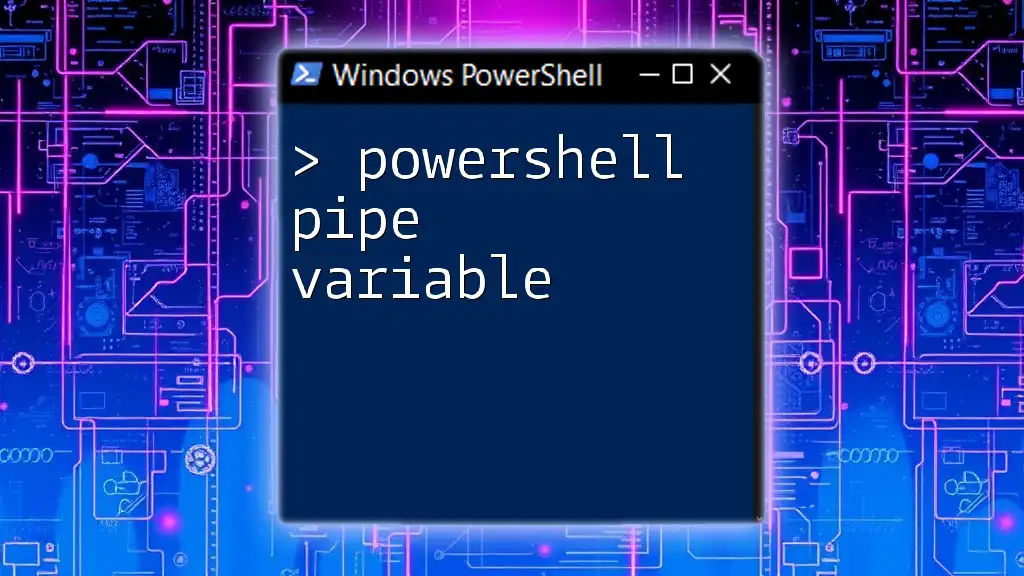
Utilizing PowerShell Pipe with Foreach
What is PowerShell Pipe Foreach?
The phrase "PowerShell pipe foreach" refers to the combination of using the pipe operator with the `Foreach-Object` cmdlet, which allows you to process each item in a pipeline. This functionality is powerful for modifying, filtering, or transforming data directly within the pipeline.
How to Combine Pipe and Foreach
To utilize the `Foreach-Object` cmdlet in a pipeline, simply append it after a command that produces output. For example:
Get-Process | ForEach-Object { $_.Name }
In this example, `Get-Process` retrieves all running processes, and `ForEach-Object` iterates over each process to output just its name. The `$_` variable represents the current object in the pipeline, ensuring you can access its properties.
Common Use Cases
Processing and Filtering Objects
You can filter objects before processing them using the pipe with `Where-Object`. Here’s an example:
Get-Service | Where-Object { $_.Status -eq 'Running' } | ForEach-Object { $_.Name }
This command first retrieves all services, filters the results to only include those that are Running, and then outputs their names.
Automating Administrative Tasks
Using `PowerShell pipe foreach`, you can automate repetitive tasks efficiently. For instance, to restart any stopped services:
Get-Service | Where-Object { $_.Status -eq 'Stopped' } | ForEach-Object { Start-Service $_.Name }
This command identifies all stopped services and automatically restarts them, showcasing how powerful automation can be with just a few lines of code.
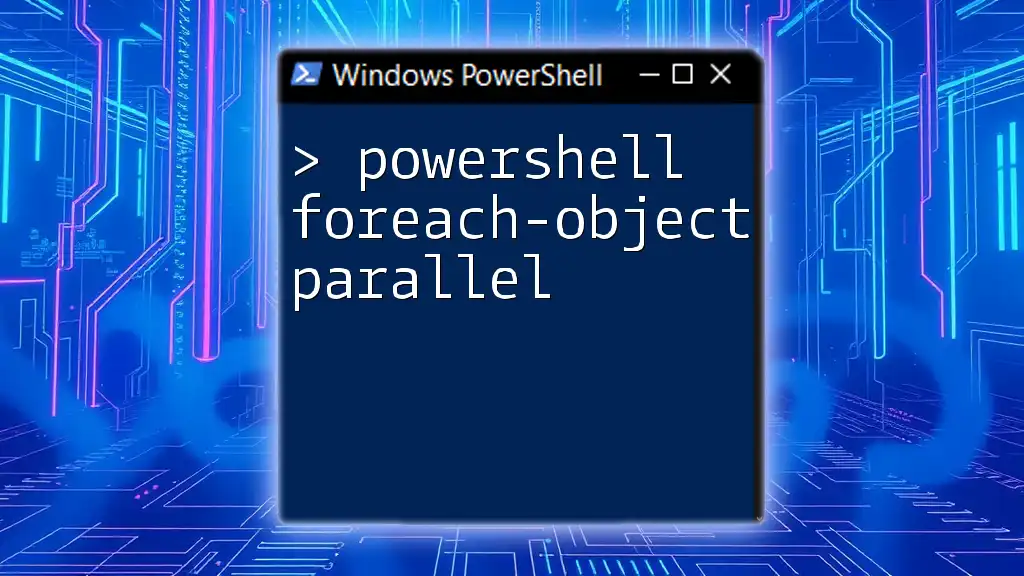
Advanced Techniques with PowerShell Pipe Foreach
Using Foreach-Object to Access Object Properties
Inside the `ForEach-Object` cmdlet, you can access various properties of the objects being processed using `$_`. For example:
Get-Process | ForEach-Object { "$($_.Name) is using $($_.WorkingSet / 1MB) MB" }
In this snippet, we output the name of each process along with its memory usage in megabytes. This illustrates how to effectively combine multiple properties within the foreach process.
Creating Custom Functions with Pipe Foreach
You can encapsulate the logic of your processing into a reusable function. Here’s an example of a function that retrieves running services based on status:
function Process-Services {
param ($Status)
Get-Service | Where-Object {$_.Status -eq $Status} | ForEach-Object { $_.Name }
}
This function accepts a parameter for service status, making it flexible and reusable in other scripts.
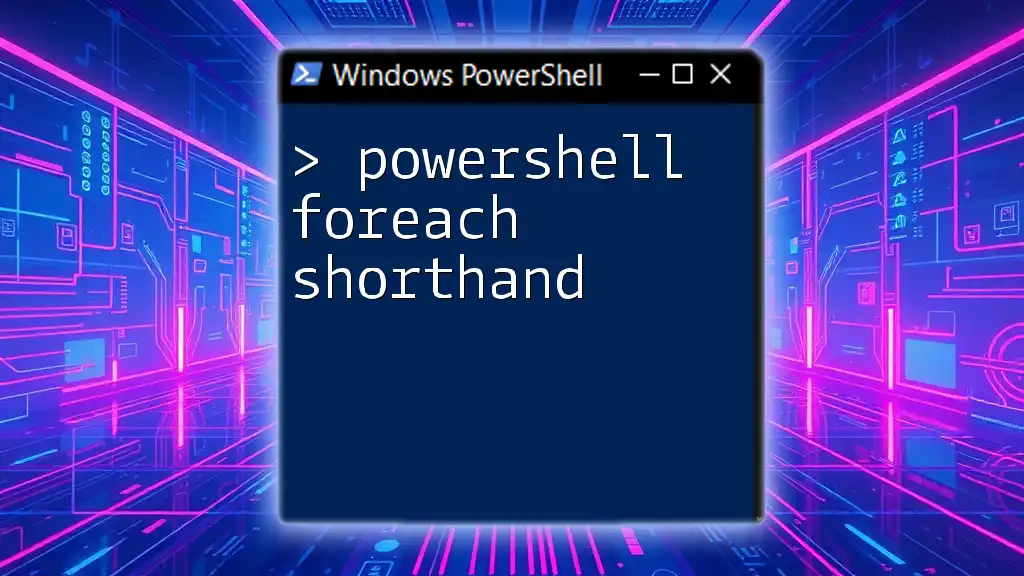
Best Practices When Using Pipe Foreach
Performance Considerations
To maximize performance, avoid excessive pipelining that could lead to unnecessary bottlenecks. Analyze your code to ensure that piped commands produce results efficiently and consider using cmdlets designed to handle large datasets without excessive use of memory.
Ensuring Readability
Maintain clean, understandable code by using descriptive variable names, employing comments, and structuring your pipelines neatly. This becomes essential, especially in larger scripts, to ensure maintainability.
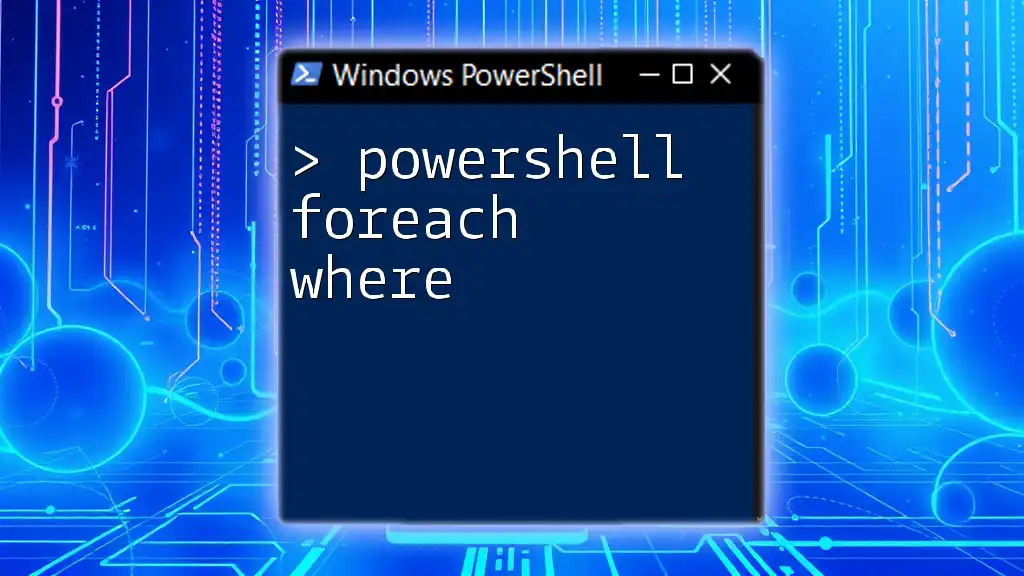
Common Errors and Troubleshooting
Common Mistakes to Avoid
One common error is misplacing the pipe symbol or incorrectly terminating a pipeline. This can lead to unexpected behavior or errors in execution. Always ensure your pipeline flow is logical and that proper cmdlets are used in sequence.
Debugging Techniques
To troubleshoot and debug your pipeline, you can insert simple output statements using `Write-Host` to display the current processing context. For example:
Get-Process | ForEach-Object { Write-Host "Processing $_" }
This allows you to see what’s currently being processed, helping identify where issues might arise.
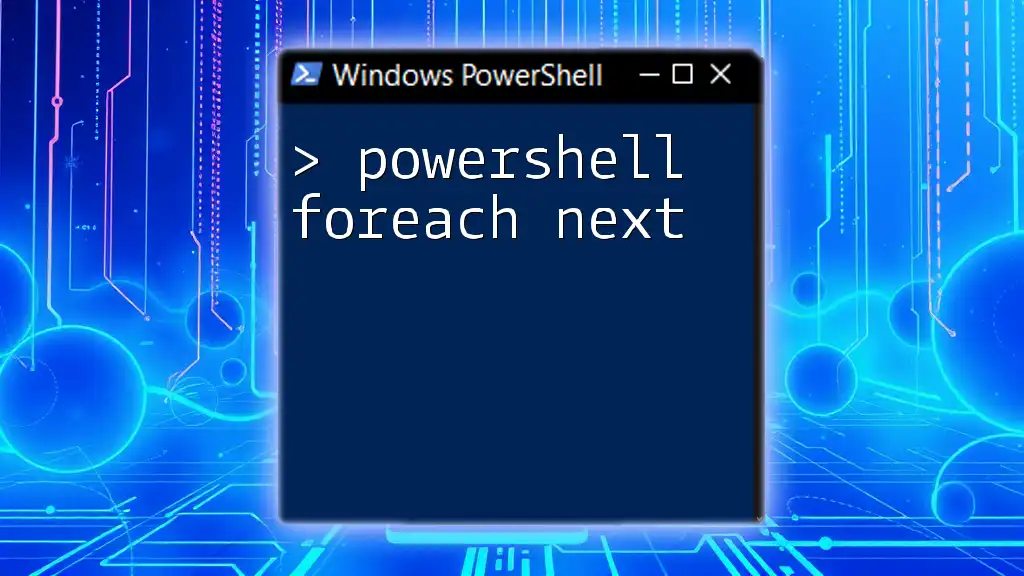
Conclusion
Incorporating `PowerShell pipe foreach` into your scripting toolkit opens up a wealth of possibilities for processing data efficiently and effectively. By understanding how to leverage these features together, you enhance your PowerShell scripting capabilities, making your scripts more concise and powerful. With practice, you'll find that these techniques are invaluable for both day-to-day automation tasks and larger scripting projects.
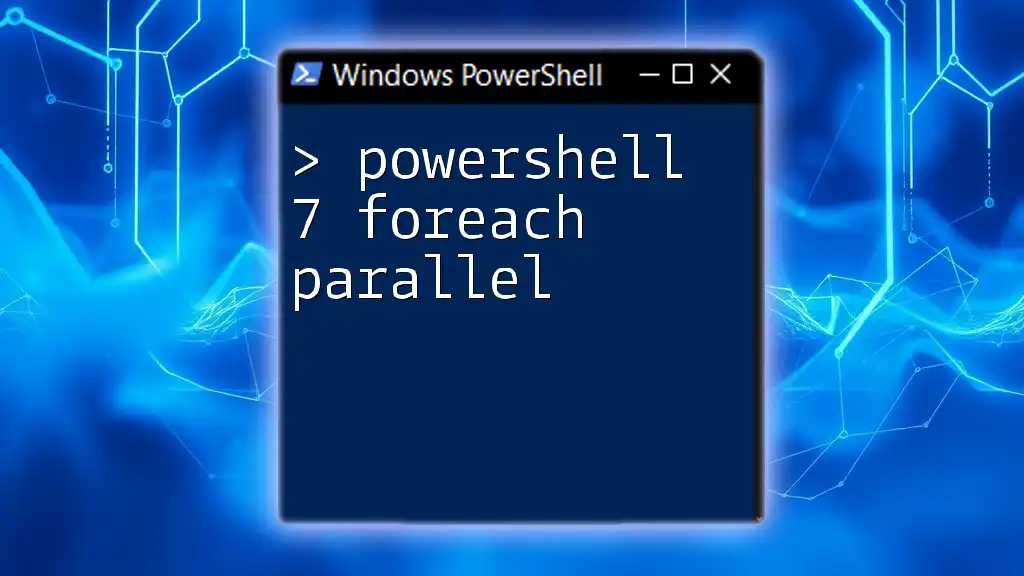
Additional Resources
- Official PowerShell Documentation
- Online Communities and Forums
- Recommended Books on PowerShell Scripting
Explore these resources to continue enhancing your skills and mastering the art of PowerShell scripting.