The `ForEach-Object -Parallel` cmdlet in PowerShell allows you to run commands in parallel, improving performance when processing large datasets. Here's a simple code snippet to demonstrate its usage:
1..10 | ForEach-Object -Parallel { Write-Host "Processing $_" }
Understanding ForEach-Object
What is ForEach-Object?
The `ForEach-Object` cmdlet is a fundamental part of PowerShell, allowing you to process items from a collection. It enables you to perform an operation on each item in a pipeline. For instance, if you have a list of files, you can easily loop through them and perform actions like copying or deleting. Its basic syntax is as follows:
InputObject | ForEach-Object {
# Your script block
}
Benefits of Using ForEach-Object
Using `ForEach-Object` streamlines your code, making it more readable and easier to maintain. It allows for efficient data handling by letting you perform operations directly on items as they flow through the pipeline. This enhances the performance of scripts, especially when utilizing parallel processing capabilities.

ForEach-Object -Parallel: An Overview
What Does -Parallel Mean?
The `-Parallel` parameter introduced in PowerShell 7 allows you to process multiple input items concurrently, significantly improving the execution time for scripts that handle large datasets or time-consuming tasks. The main advantage of parallel execution lies in its ability to harness multiple CPU cores, thereby reducing the overall runtime.
How ForEach-Object -Parallel Works
The `ForEach-Object -Parallel` cmdlet accepts a `-ScriptBlock` that defines the operations to be executed for each item. Each script block runs in isolation, which means you need to be mindful of variable scopes, as shared variables may lead to unexpected behaviors. However, items are passed to the script block seamlessly, allowing for efficient and powerful processing.

Setting Up Your Environment
Requirements for Using -Parallel
To utilize `ForEach-Object -Parallel`, you must be running at least PowerShell version 7.0 or higher. If you are working with older versions of PowerShell, you won’t have access to this feature.
Installing Windows Management Framework (if needed)
If your system does not support PowerShell 7, you can download and install the Windows Management Framework. Here's a quick guide:
- Visit the official Microsoft website.
- Download the appropriate version for your system.
- Follow the installation instructions provided.
After installation, check your PowerShell version by executing:
$PSVersionTable.PSVersion
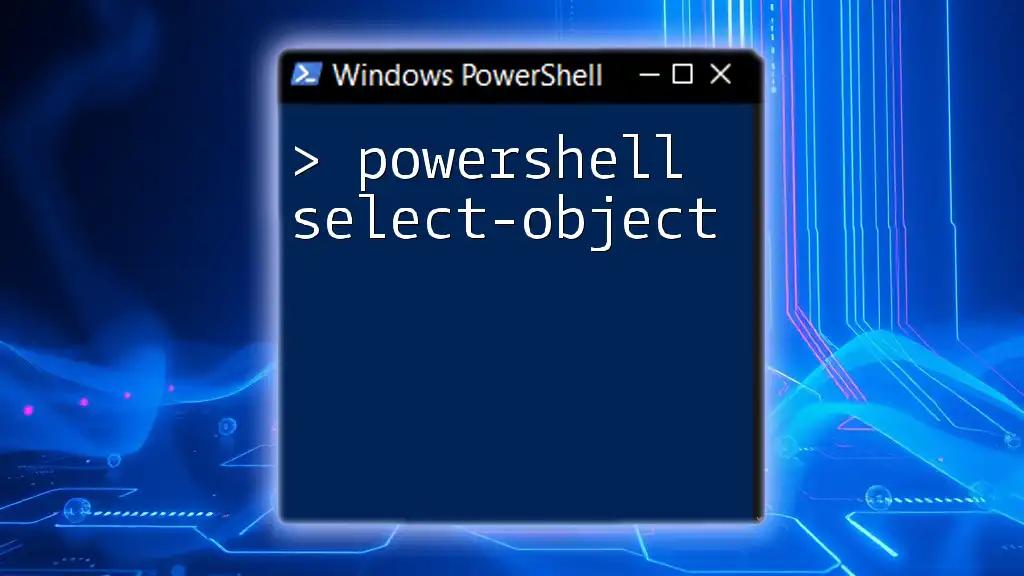
Syntax and Options for ForEach-Object -Parallel
Basic Syntax Breakdown
The basic syntax for `ForEach-Object -Parallel` looks like this:
InputObject | ForEach-Object -Parallel {
# Your script block
} -ThrottleLimit <int>
Parameters and Their Meanings
-InputObject
The `-InputObject` parameter specifies the input items that you want to process. This can be a range, array, or an output from another command.
-ThrottleLimit
The `-ThrottleLimit` parameter allows you to define the maximum number of threads that can run concurrently. By adjusting this, you can manage CPU load. Too many threads can lead to performance issues, while too few might not fully utilize your system’s resources.
-AsJob
Using the `-AsJob` parameter allows you to run the `ForEach-Object -Parallel` command in background jobs. This is particularly useful for long-running tasks that you want to start and forget, freeing up your console for other commands.
-ScriptBlock
This parameter is essential for defining the operations that you wish to execute on each item. This can include a broad range of actions, from simple calculations to intricate data manipulations.

Practical Examples of ForEach-Object -Parallel
Example 1: Basic Parallel Processing
In this initial example, we will run a simple command in parallel:
1..10 | ForEach-Object -Parallel {
Start-Sleep -Seconds 1;
Write-Output "Processed $_"
} -ThrottleLimit 4
In this code snippet, we are creating a range from 1 to 10 and processing each number with a delay of one second. The `-ThrottleLimit 4` ensures that only four threads run at the same time, balancing performance with system resource management.
Example 2: Working with Files
Here, we will demonstrate how to perform file operations in parallel, which can drastically enhance the efficiency of file management tasks:
Get-ChildItem -Path "C:\MyFiles" | ForEach-Object -Parallel {
Copy-Item -Path $_.FullName -Destination "C:\Backup"
} -ThrottleLimit 5
In this example, we retrieve all files from a specified directory and copy each one to a backup location. Utilizing `-ThrottleLimit` helps ensure that the copy operation remains efficient without overwhelming system resources.
Example 3: Data Processing with API
Lastly, let’s explore how to fetch data from an API concurrently, which can significantly speed up operations that require network requests:
$urls = @("http://example.com/api1", "http://example.com/api2")
$urls | ForEach-Object -Parallel {
Invoke-RestMethod -Uri $_
} -ThrottleLimit 2
This snippet arranges to make HTTP requests to two API endpoints. By setting the `-ThrottleLimit` to 2, we allow two requests to be processed simultaneously, optimizing network usage while avoiding potential throttling from the API.

Common Issues and Troubleshooting
Error Handling in ForEach-Object -Parallel
When using `ForEach-Object -Parallel`, you may encounter various errors. Common issues include scope-related errors or problems identifying variables. Implementing error handling with `Try/Catch` blocks within your script block can effectively manage exceptions:
$urls | ForEach-Object -Parallel {
Try {
Invoke-RestMethod -Uri $_
} Catch {
Write-Output "Failed to access $_: $_"
}
} -ThrottleLimit 2
Performance Troubleshooting
If you notice slow performance, consider the following:
- Evaluate your `ThrottleLimit` settings to strike the right balance between speed and resource usage.
- Review your script block for any bottlenecks, such as synchronous calls that may hinder performance.
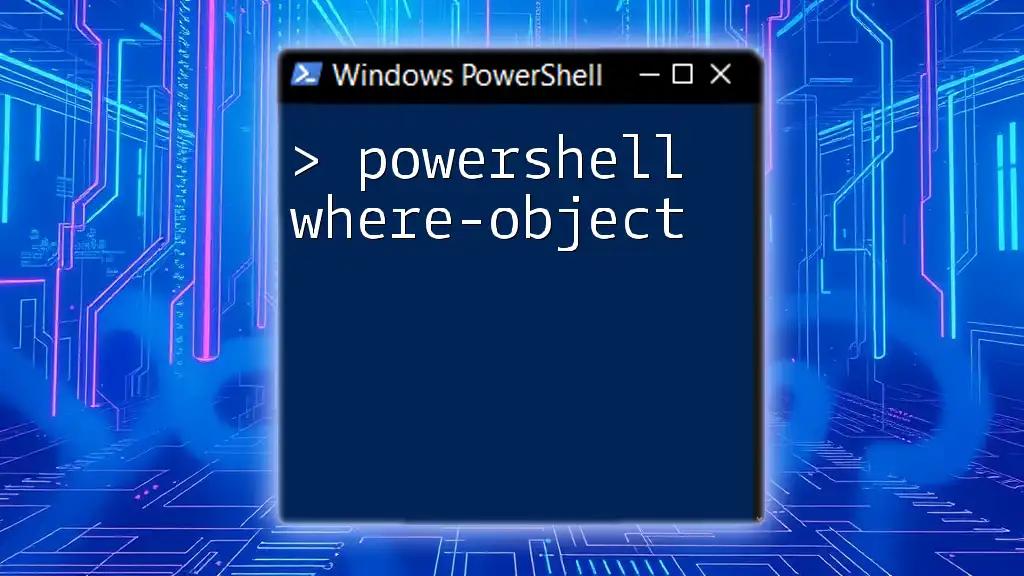
Best Practices for Using ForEach-Object -Parallel
Tips for Writing Efficient Code
To achieve maximum efficiency with `ForEach-Object -Parallel`, remember:
- Keep your script blocks concise to minimize execution time.
- Be cautious with shared state; variables declared outside the script block are not shared by default. Use `$Using:` to pass variables into the parallel execution context if needed.
When to Avoid Using -Parallel
While `ForEach-Object -Parallel` can be highly beneficial, there are scenarios where it may not be the best choice. Avoid using it when:
- Each operation relies heavily on shared state or local variables.
- The tasks being executed do not benefit from parallel execution, as added complexity can outweigh performance gains.
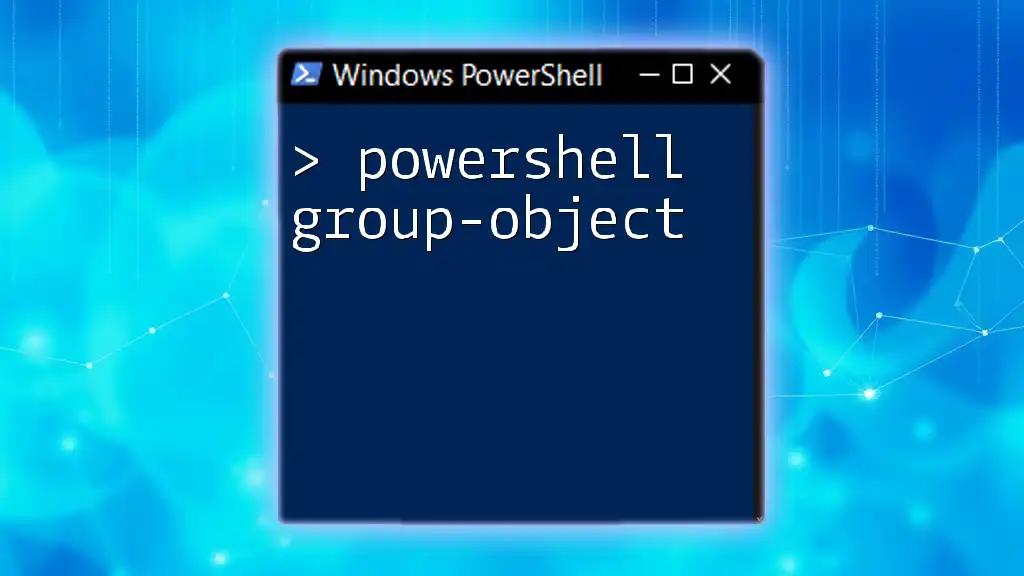
Conclusion
In conclusion, the PowerShell `ForEach-Object -Parallel` cmdlet is a powerful tool for enhancing script performance through concurrent processing. It equips you with the ability to run multiple tasks simultaneously, effectively utilizing your system's resources. By exploring the examples and best practices outlined in this guide, you are now well-equipped to harness the capabilities of PowerShell for your automation tasks. Experiment with the provided examples and consider enrolling in our training sessions for a deeper dive into mastering PowerShell scripting.

Additional Resources
Recommended Reading
For further reading, consider exploring the official Microsoft documentation on `ForEach-Object` and PowerShell workflows. You may also find specialized books and online courses valuable for deepening your understanding of PowerShell.
Community and Support
Join PowerShell community forums and groups to discuss tips, share scripts, and seek advice from fellow PowerShell enthusiasts. Don't forget to follow us on our social media channels for the latest updates and PowerShell tips!