The `Where-Object -Like` cmdlet in PowerShell is used to filter objects from a collection based on a wildcard pattern match.
Here’s a code snippet demonstrating its use:
Get-Process | Where-Object { $_.Name -like '*chrome*' }
Basic Syntax of `Where-Object`
The `Where-Object` cmdlet is foundational in PowerShell for filtering objects based on specific criteria. Its general structure can be summarized as follows:
Where-Object { <scriptblock> }
When using the `-Like` operator with `Where-Object`, the syntax becomes:
Where-Object { $_.Property -like 'Pattern' }
Here, `$_` represents the current object in the pipeline, and `Property` denotes the attribute of that object you want to evaluate. The `-like` operator allows for pattern matching, making it a versatile tool in your PowerShell arsenal.
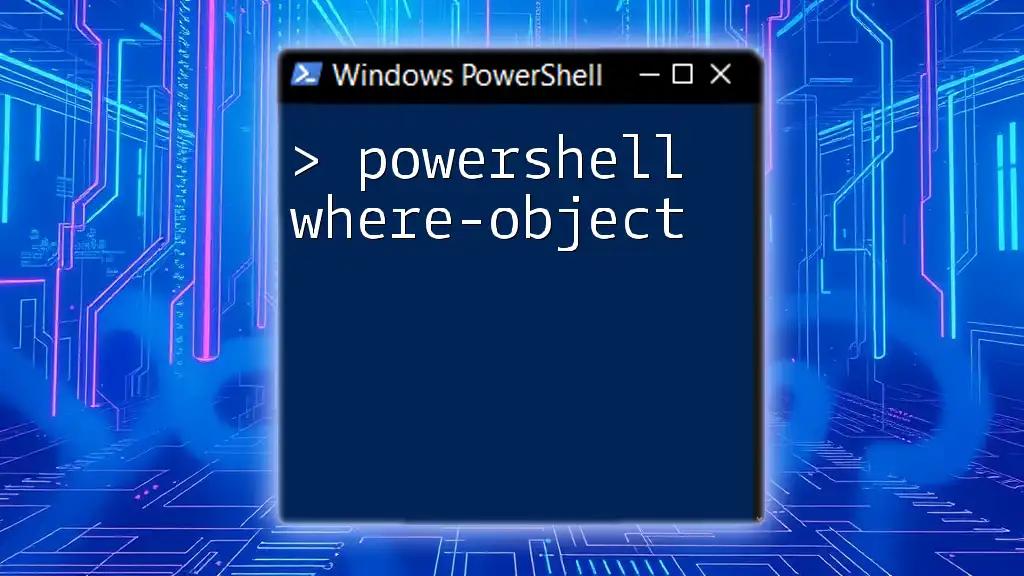
How `-Like` Works
One of the key features of `-Like` is its ability to perform wildcard pattern matching. The two wildcard characters you will frequently use are:
- `*`: Represents zero or more characters.
- `?`: Represents a single character.
For instance, if you want to find all processes that start with the letter "s", you can execute:
Get-Process | Where-Object { $_.ProcessName -like 's*' }
In this case, the command retrieves all processes whose names begin with "s".
You can also use the `?` wildcard for more specific matching. For example, to find processes where the name consists of an "s", followed by any character, and then "t", you could run:
Get-Process | Where-Object { $_.ProcessName -like 's?t' }
This command will match process names such as "sat" or "sbt", demonstrating the flexibility of the `-Like` operator for complex matching scenarios.

Practical Applications
Filtering User Accounts in Active Directory
In a more complex environment like Active Directory (AD), `Where-Object -Like` proves invaluable in filtering user accounts based on specific criteria. For example, if you want to list all users whose names start with the letter "A", you would run:
Get-ADUser -Filter * | Where-Object { $_.Name -like 'A*' }
This command fetches all AD users and then filters the result to show only those starting with "A".
Searching Log Files
When it comes to searching through log files for specific entries, `Where-Object -Like` can be a powerful ally. If you have a log file and want to filter for entries that contain the word "Error", you might use the following command:
Get-Content 'C:\Logs\application.log' | Where-Object { $_ -like '*Error*' }
This command scours the log file and outputs only lines that include the term “Error”, making it easier to identify issues quickly.

Combining `Where-Object -Like` with Other Cmdlets
Using with `Get-ChildItem`
The `Where-Object -Like` combination is also useful for filtering file types when working with file systems. For instance, to list all text files in a directory, you can use:
Get-ChildItem -Path 'C:\Temp' | Where-Object { $_.Name -like '*.txt' }
This command retrieves listing of files in the `C:\Temp` directory and filters the output to display only `.txt` files.
Chaining Cmdlets for More Advanced Filtering
One of the strengths of PowerShell is its ability to chain cmdlets together. For instance, when you want to filter processes based on CPU usage and name, you could do:
Get-Process | Where-Object { $_.CPU -gt 100 } | Where-Object { $_.ProcessName -like 's*' }
This command retrieves processes whose CPU usage is greater than 100 and further filters them to show only those that start with "s". This demonstrates how to create powerful command chains for data retrieval.

Common Mistakes and Troubleshooting
Case Sensitivity Issues
While using PowerShell, it's crucial to note that the `-Like` operator is case-insensitive by default. However, if you need a case-sensitive comparison, you can use the `-clike` operator. For example:
Get-Process | Where-Object { $_.ProcessName -clike 'S*' }
This command will only match processes whose names start with an uppercase "S".
Wildcards Misuse
Another common mistake when using `-Like` is improper wildcard usage. Ensure you understand when to use `*` and `?`, as misuse can lead to unexpected results. Testing and tweaking your patterns is vital, especially when you're not getting the results you expect.

Performance Considerations
Efficiency of `Where-Object`
When working with large datasets, performance can be an issue. Using `Where-Object` effectively means understanding that it will evaluate each object in the pipeline, which might slow down your script if the dataset is large.
Best Practices for Optimization
To optimize your scripts, aim to filter as early in the pipeline as possible. For example, try to limit the items being processed before applying `Where-Object`. Instead of:
Get-Process | Where-Object { $_.CPU -gt 100 }
You might use:
Get-Process -IncludeUserName | Where-Object { $_.CPU -gt 100 }
This helps reduce memory usage and speeds up your scripts.
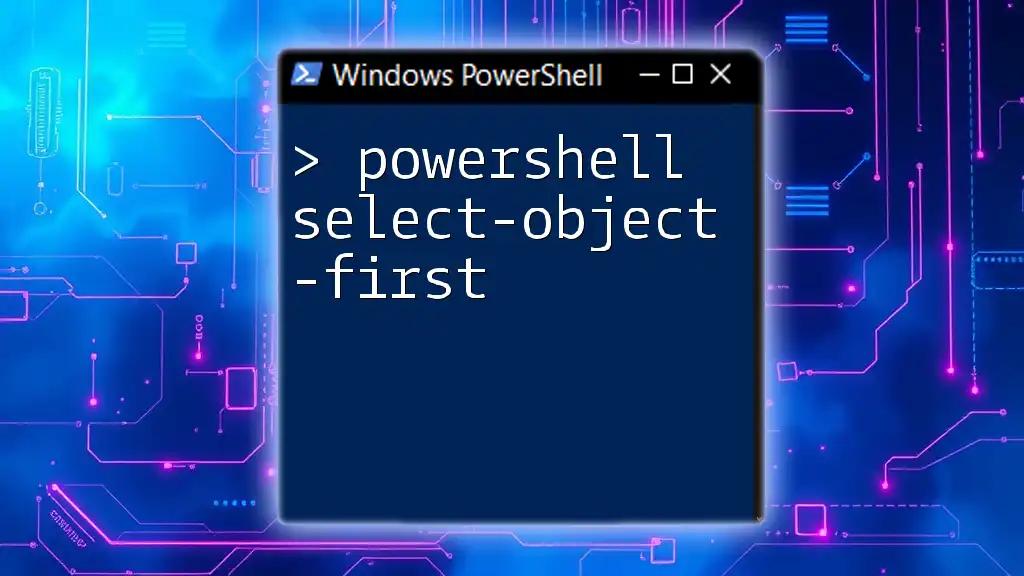
Conclusion
In summary, `Where-Object -Like` is a powerful tool within PowerShell that enhances your ability to filter and manipulate data efficiently. By mastering this cmdlet and its syntax, you will be able to refine your scripts and automate tasks more effectively.
Don’t hesitate to experiment with these commands in your PowerShell environment. The practice will go a long way in making you adept at using `Where-Object -Like`, and you'll discover countless applications to streamline your workflow.

Additional Resources
For further reading, the official PowerShell documentation serves as an excellent resource for understanding the intricacies of cmdlets and operators. Additionally, exploring recommended books and tutorials can significantly enhance your PowerShell skills and empower you to tackle more complex scripting challenges.