The `Where-Object` cmdlet in PowerShell allows you to filter objects based on specific criteria, and using the `-like` operator enables pattern matching with wildcards.
Here’s a code snippet demonstrating its use:
Get-Process | Where-Object { $_.Name -like '*notepad*' }
Understanding the `Where-Object` Cmdlet
What is `Where-Object`?
The `Where-Object` cmdlet is a fundamental component of PowerShell that serves the purpose of filtering objects in a pipeline. It enables users to process and filter collections of data based on specific criteria. By using `Where-Object`, you can refine results to only include items that meet your defined conditions, greatly enhancing the efficiency of your scripts.
Syntax of `Where-Object`
The basic structure of the `Where-Object` command is as follows:
Where-Object { <ScriptBlock> }
Here, `<ScriptBlock>` is where you define the conditions for the filtering process. For instance, to filter a list of services and only return those services that are currently running, you could use:
Get-Service | Where-Object { $_.Status -eq 'Running' }
In this example, `$_` represents the current object in the pipeline. The command retrieves all services and filters them based on their `Status`.
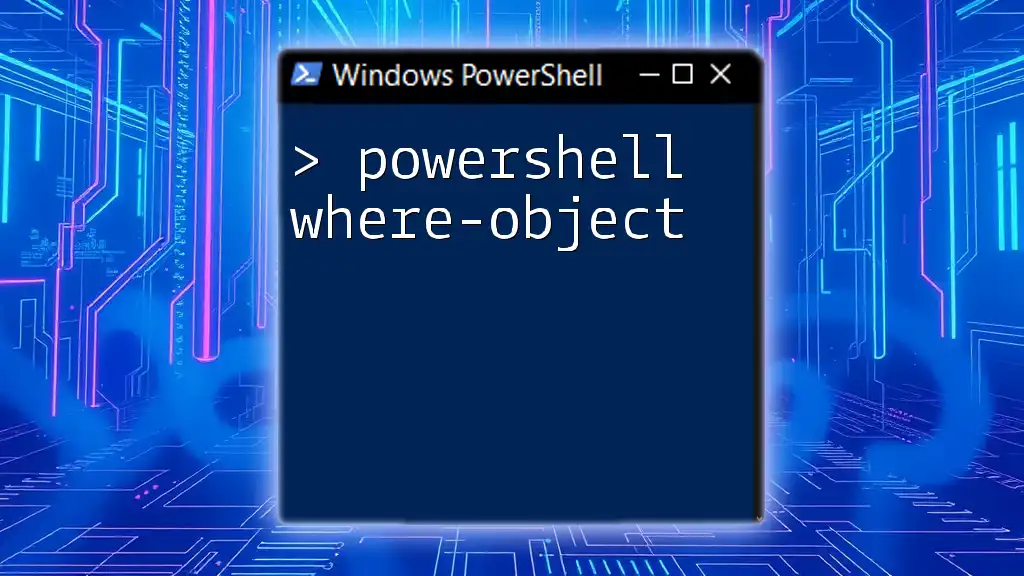
The `-like` Operator
What is the `-like` Operator?
The `-like` operator is specifically designed for pattern matching and allows for the use of wildcards. Unlike `-eq`, which checks for exact matches, `-like` empowers you to search for a pattern in string values. Additionally, `-match`, which is another comparison operator, uses regular expressions for more complex pattern matching.
Wildcards and Patterns
In PowerShell, the wildcards used with the `-like` operator are:
- `*`: Represents zero or more characters.
- `?`: Represents a single character.
For example, if you want to search for all services that have the word "Service" in their names, you would use:
Get-Service | Where-Object { $_.Name -like '*Service*' }
This retrieves any service whose name contains the string "Service."
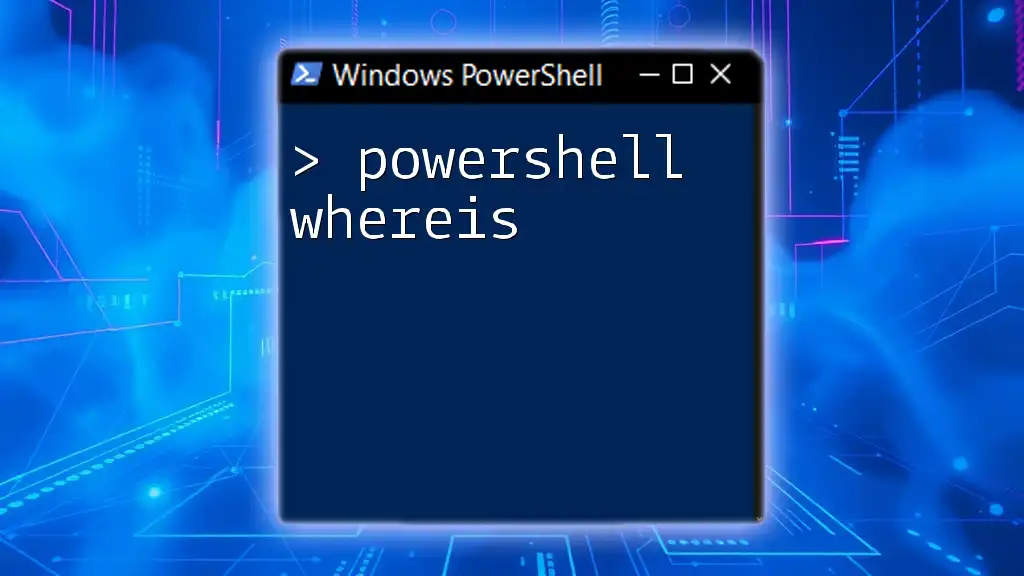
Using `Where-Object` with `-like`
Basic Usage Example
The fundamental use of `Where-Object` with `-like` demonstrates how effective filtering can be in managing data. Here's how to filter the running processes to find any that include "chrome":
Get-Process | Where-Object { $_.Name -like '*chrome*' }
This command will return all processes that include "chrome" in their name, showcasing a practical application of using the `-like` operator effectively.
Advanced Filtering Scenarios
Multiple Criteria
Filtering can be further refined using multiple criteria. For example, if you want to retrieve both Chrome and Firefox processes simultaneously, you can do so by combining conditions with `-or`:
Get-Process | Where-Object { $_.Name -like '*chrome*' -or $_.Name -like '*firefox*' }
This will return processes that meet either condition, providing a broader view of the data.
Combining with Other Cmdlets
In many instances, it may be beneficial to combine `Where-Object` with other cmdlets to enhance the data output. For example, you can sort the filtered services as follows:
Get-Service | Where-Object { $_.Name -like '*Service*' } | Sort-Object -Property Status
Here, services that match the `-like` condition are sorted by their status, allowing for easy identification of their running state.
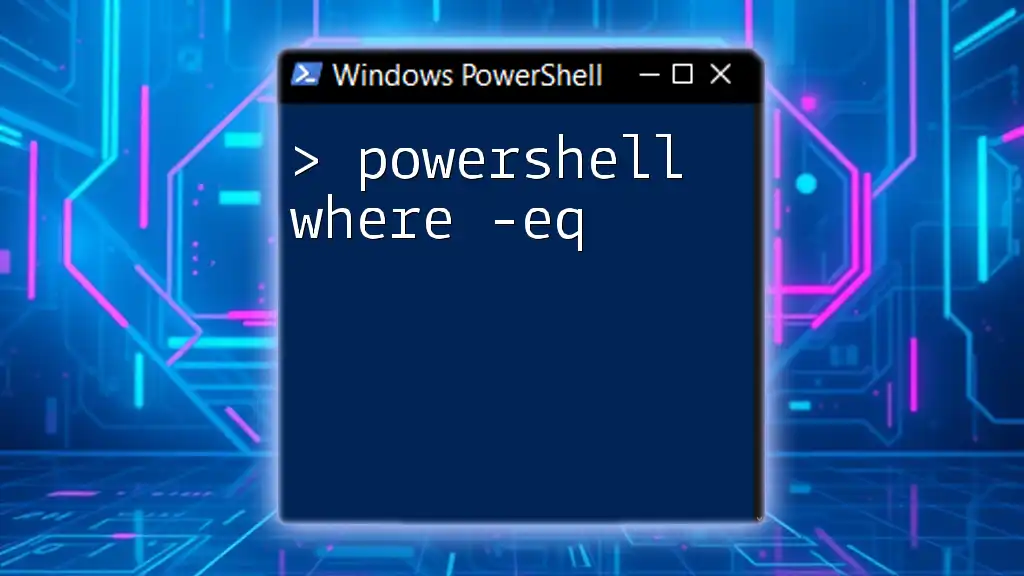
PowerShell Name Like
Using `Name` with `-like`
When you want to specifically filter by the `Name` attribute of files or objects, the `-like` operator can be exceptionally powerful. For instance, if you're looking for all text files in a directory, use:
Get-ChildItem | Where-Object { $_.Name -like '*.txt' }
This snippet retrieves all files that have a `.txt` extension, effectively filtering your output to only show relevant results.
Robust Filtering Techniques
Case-Insensitive Filtering
PowerShell's comparison operators are not case-sensitive by default. However, if you need to ensure case insensitivity explicitly, you can convert the string to lower case:
Get-Process | Where-Object { $_.Name.ToLower() -like '*explorer*' }
In this example, no matter how "explorer" appears (e.g., "Explorer", "EXPLORER"), it will be successfully matched.
Handling Multiple Extensions
To enhance your file filtering capabilities, you might want to find files across several extensions. You can achieve this using logical operators:
Get-ChildItem | Where-Object { $_.Name -like '*.jpg' -or $_.Name -like '*.png' }
This command retrieves all JPEG and PNG image files, reflecting the flexibility and power of using `Where-Object` in combination with the `-like` operator.
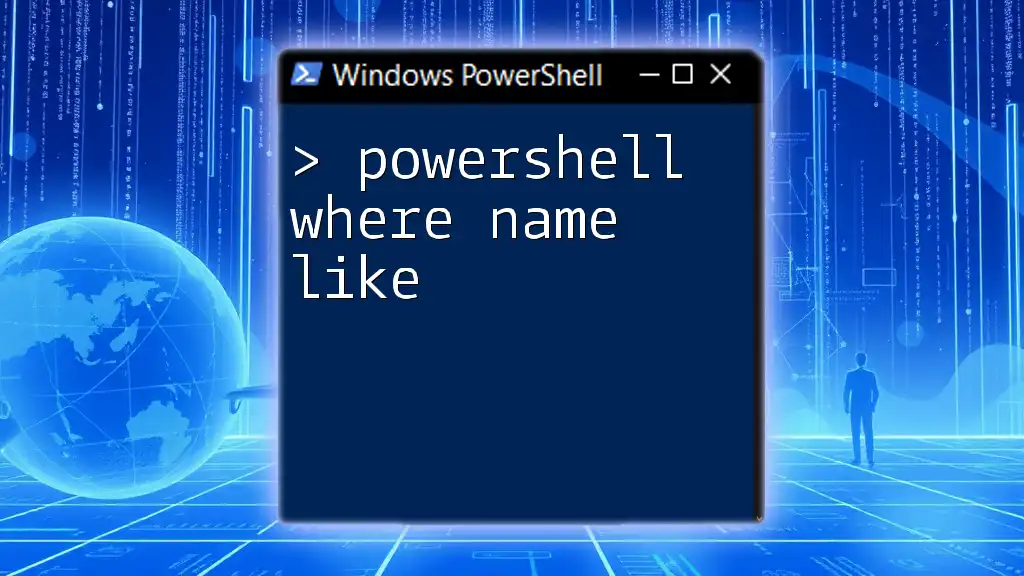
Best Practices in Using `Where-Object` and `-like`
Performance Considerations
While `Where-Object` is a powerful tool, its use can impact performance, especially with large datasets. To improve efficiency, it’s generally advisable to filter as early as possible in your command pipeline. This approach reduces the amount of data being processed at later stages.
Encouraging Readability
For maintainable and understandable scripts, follow best practices such as:
- Using meaningful variable names and clear comments.
- Breaking down complex filtering criteria into simpler, understandable statements.
For example, you could use comments to clarify your intentions:
# Filter for running services and sort by name
Get-Service | Where-Object { $_.Status -eq 'Running' } | Sort-Object -Property Name
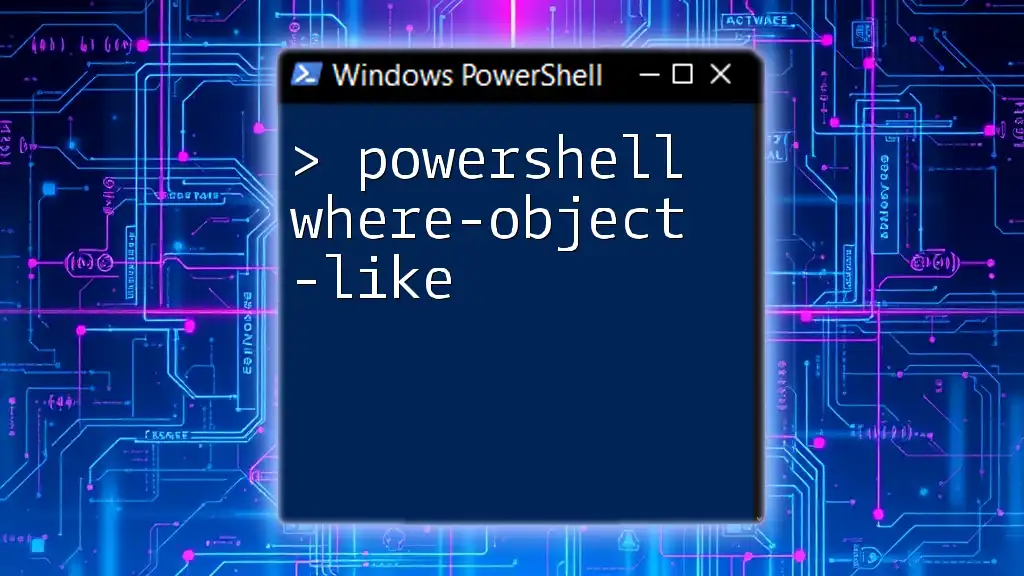
Common Mistakes and Troubleshooting
Frequent Errors with `Where-Object`
Common issues when using `Where-Object` include:
- Incorrect syntax: Be mindful of the use of curly braces and parentheses.
- Misinterpretation of `-like`; ensure that the string being searched is appropriate for the wildcard usage.
If you encounter an error, review your syntax closely and confirm that your criteria accurately reflect your intentions.
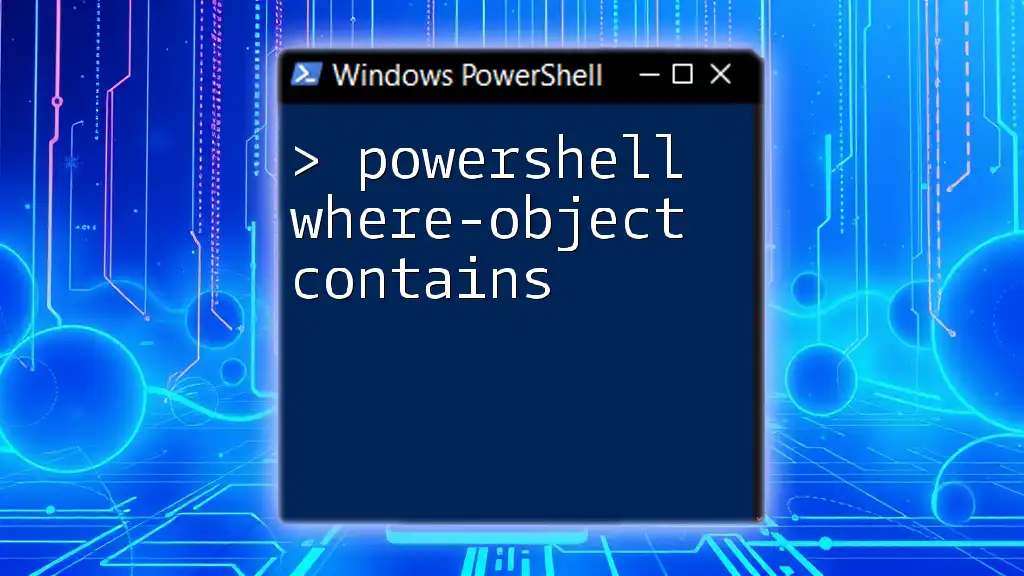
Conclusion
The combination of `Where-Object` and `-like` in PowerShell is paramount for effective data manipulation and analysis. By mastering these tools, you can develop scripts that filter data succinctly and accurately, making your PowerShell experience richer and more productive.
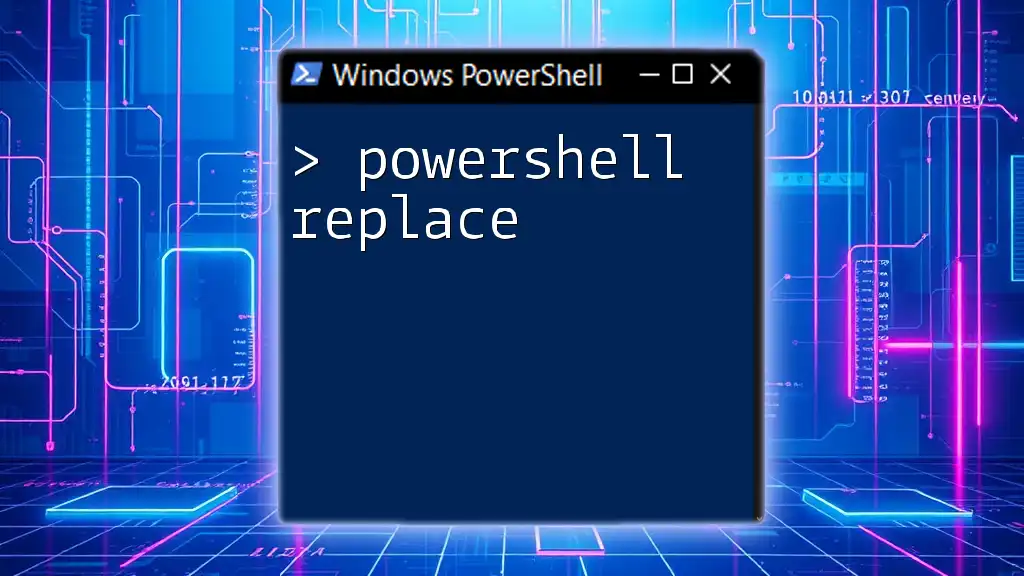
Additional Resources
To deepen your knowledge, consider exploring additional reading materials, tutorials, and courses on PowerShell and its extensive capabilities.
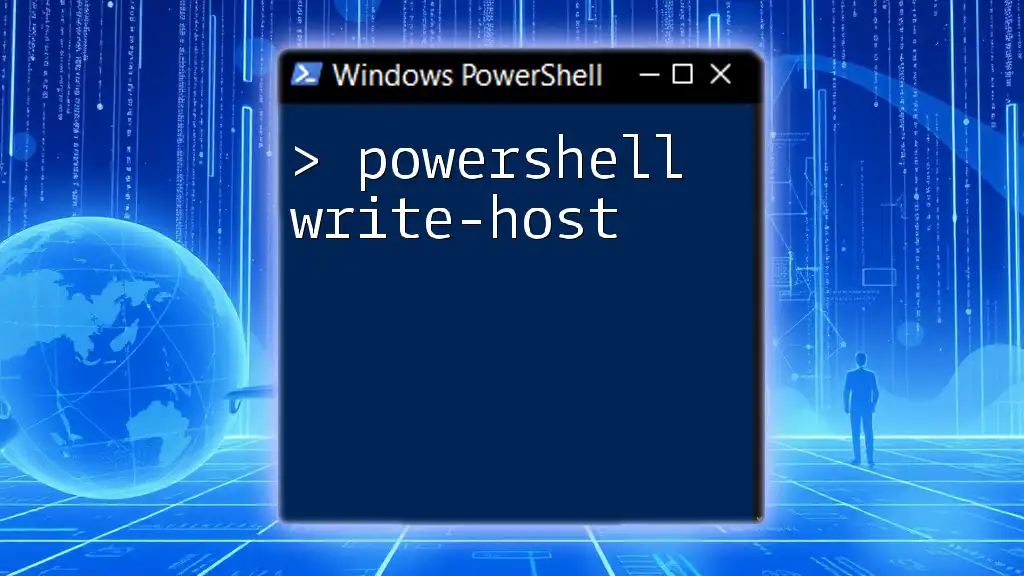
Call to Action
Try your hand at writing your own scripts using `Where-Object` and `-like` to filter for specific data sets! Embrace the versatility of PowerShell and see how it can streamline your workflows.