A PowerShell Helper is a tool or resource designed to assist users in efficiently executing commands and automating tasks within the PowerShell environment.
Here’s a simple code snippet to get you started:
Write-Host 'Hello, World!'
Understanding PowerShell Helpers
Definition of PowerShell Helpers
PowerShell helpers are tools and functionalities designed to assist users in effectively executing PowerShell commands and scripts. They simplify the process of learning and utilizing a wide range of commands by offering guidance, shortcuts, and optimized workflows.
Types of PowerShell Helpers
Built-in Cmdlets are essential building blocks in PowerShell that perform specific functions without needing extensive scripting. They allow users to perform tasks rapidly using simple commands.
Functions and Scripts are pieces of reusable code that can encapsulate complex operations into manageable sections. Functions can take parameters, perform actions, and return results, making scripts easier to run repeatedly without rewriting the same code.
Modules and Snapins expand PowerShell's capabilities. Modules are collections of cmdlets, providers, functions, and other tools, while snapins are similar but designed for older versions of PowerShell. Both allow users to access a diverse range of functionalities tailored to specific needs.
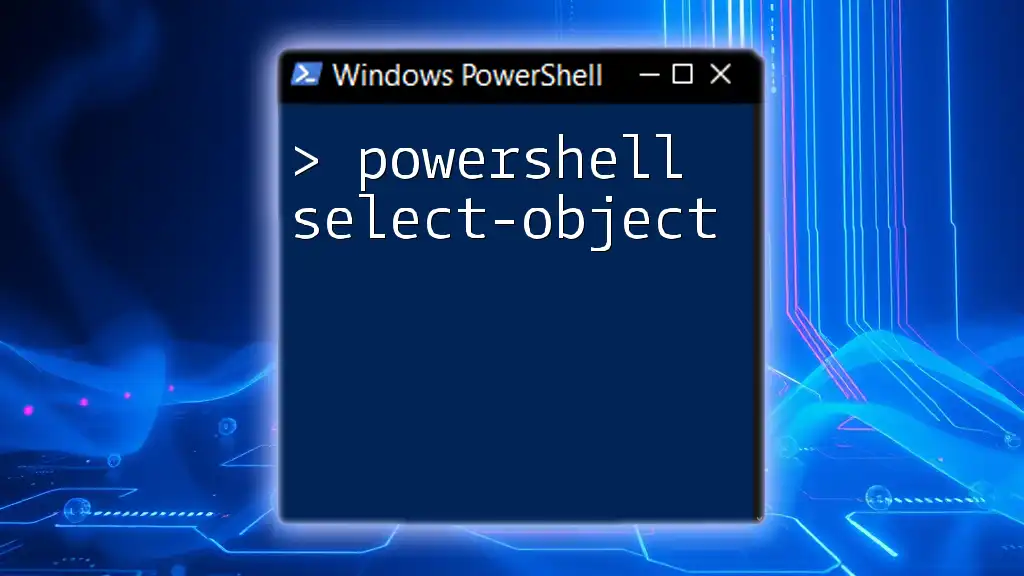
Using PowerShell Help Command
Overview of the Help Command
The `Get-Help` command is one of the most powerful features in PowerShell, providing essential guidance on commands, syntax, and examples. It acts as an on-demand encyclopedia, equipping users with detailed insights into how to effectively utilize the command line.
Examples of Using `Get-Help`
Basic Syntax: To get a brief overview of any command, simply use:
Get-Help <command-name>
Getting Detailed Information: For comprehensive details about a command, including parameters and usage examples, you can execute:
Get-Help <command-name> -Full
Finding Examples: To see practical examples that demonstrate command usage, the command is:
Get-Help <command-name> -Examples
Utilizing `Update-Help`
To ensure that your help content is up-to-date, you can run:
Update-Help
This command downloads the latest help files from the internet, ensuring you have access to the most recent information.
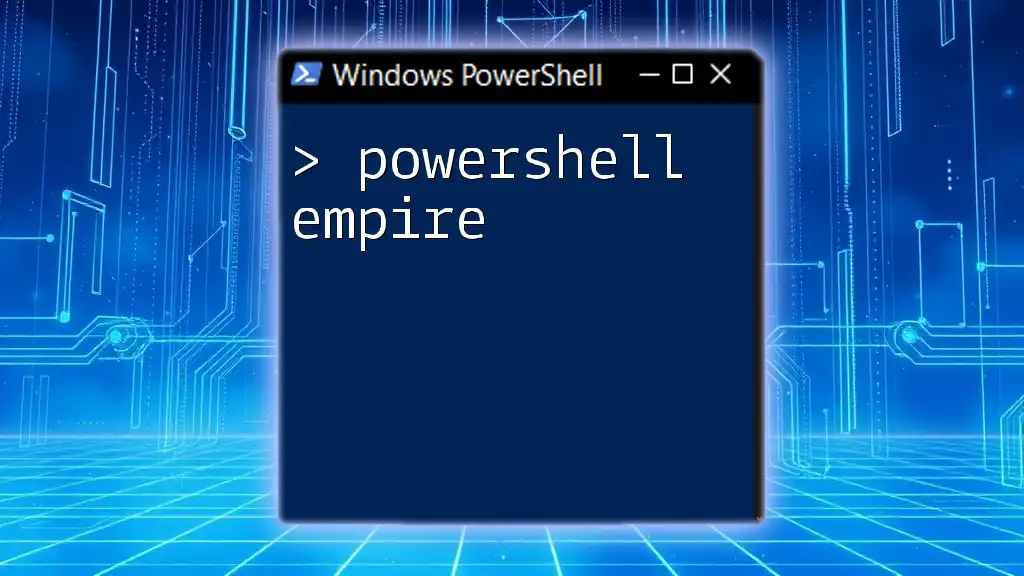
Leveraging PowerShell ISE
Introduction to PowerShell ISE
PowerShell Integrated Scripting Environment (ISE) is a versatile tool for writing, testing, and debugging PowerShell scripts. It offers an intuitive interface to help users streamline their scripting experience.
Features of PowerShell ISE
-
Syntax Highlighting improves the readability of scripts by visually differentiating elements of code, making debugging easier.
-
IntelliSense offers auto-completion suggestions for cmdLets, parameters, and variable names, speeding up the coding process.
-
Built-in Help Pane allows users to view help documentation alongside the script they’re working on, providing instant support without leaving the environment.
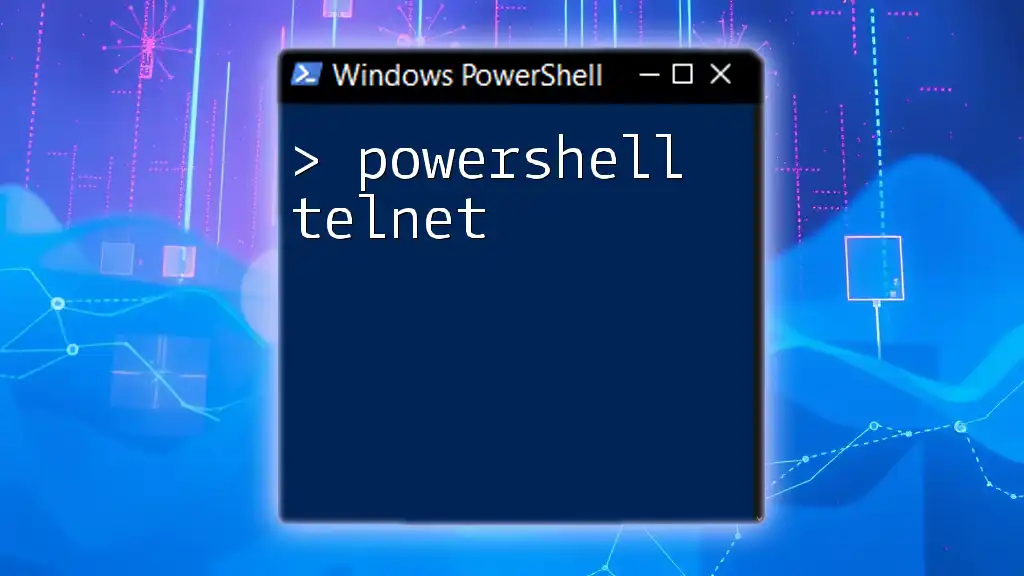
Common PowerShell Helpers
Using Aliases
Aliases in PowerShell are shortcuts for cmdlets, providing a simplified way to run commands, especially for frequent tasks.
Examples of Common Aliases
One of the most useful aliases is:
ls # This is an alias for Get-ChildItem
This allows users to quickly list files and directories without typing the full command.
Customizing Aliases
You can create your own aliases to suit your workflow. For example, if you often check the status of processes, you could do:
Set-Alias myalias Get-Process
This creates a shortcut for `Get-Process`, making it easy to execute.
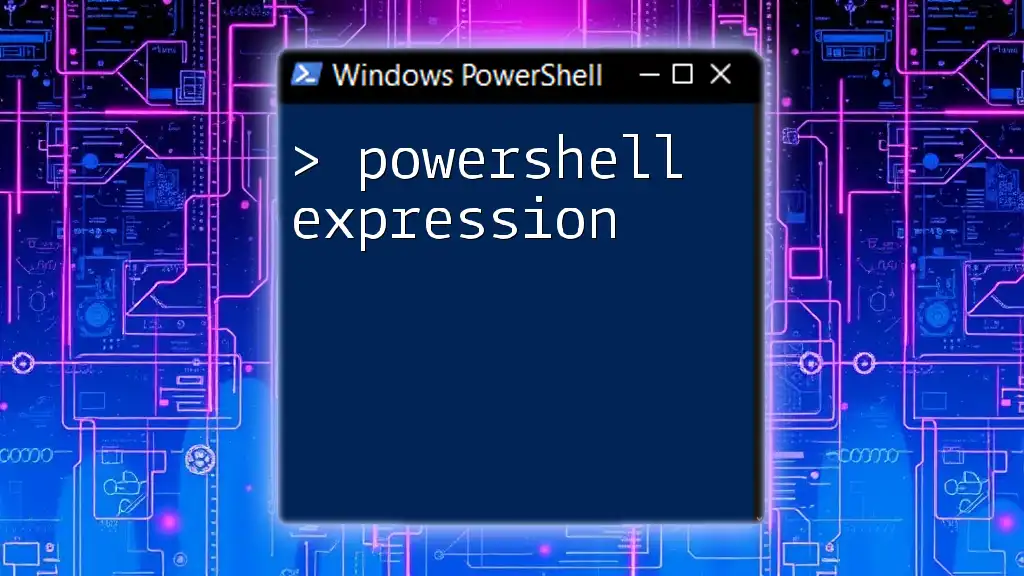
PowerShell Snippets
What Are PowerShell Snippets?
PowerShell snippets are small blocks of reusable code that can be integrated into larger scripts or commands. They help eliminate redundancy and speed up the coding process.
Building and Using Snippets
Creating reusable code snippets is straightforward. For instance, if you frequently need to format dates, you could write a snippet like this:
function Format-Date {
param (
[datetime]$date
)
return $date.ToString("yyyy-MM-dd")
}
This snippet formats a date in a specific way, and you can call it anytime throughout your scripts.
Using Snippets in ISE and VS Code
Snippets can be accessed and inserted effectively in both ISE and Visual Studio Code, making it easy to incorporate standard code into any project.
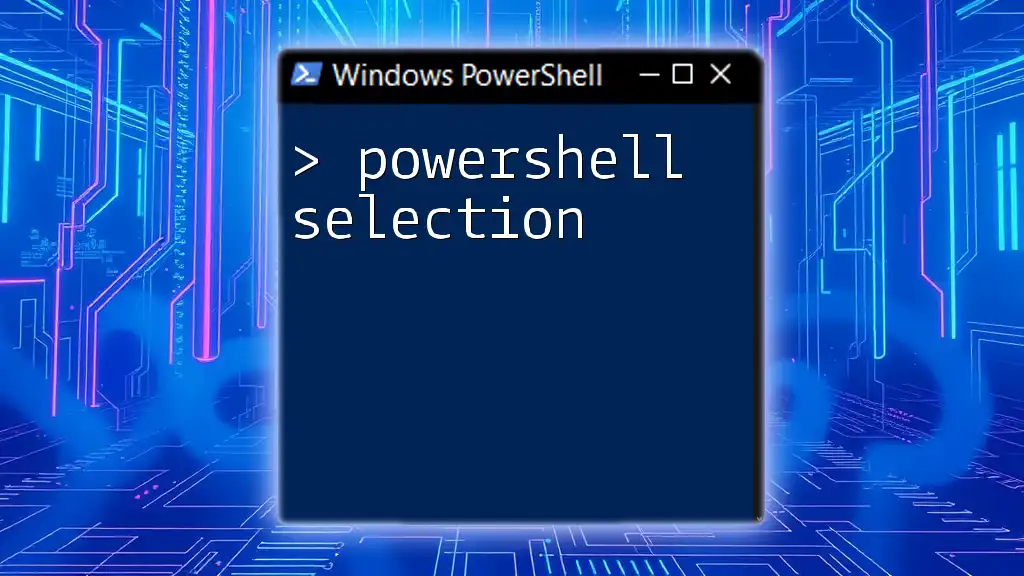
Automating Tasks with PowerShell Helpers
Creating Functions
Functions are essential for automating repetitive tasks, encapsulating code for better organization and reusability.
Basic Function Structure
A simple function follows this structure:
function Get-UserInfo {
param (
[string]$username
)
# Function body here, e.g., fetching user details
}
This structure allows you to define parameters, providing flexibility for users to input different values each time the function is invoked.
Advanced Function Techniques
In advanced functions, you can employ parameters and return values for enhanced functionality, allowing for greater versatility. For instance, functions can return complex objects or multiple values.
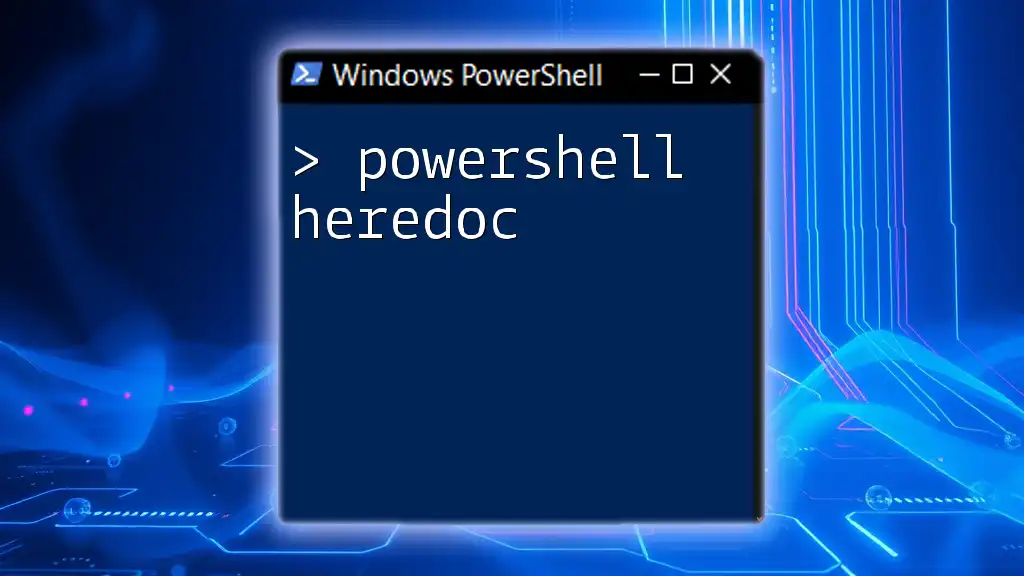
Best Practices for Using PowerShell Helpers
Writing Readable Code
Comments are vital in ensuring that your code is understandable, both for you and others who may maintain it in the future. Comments can clarify complex logic and provide context on specific sections of code.
Error Handling
Using `Try/Catch` blocks is essential for managing errors gracefully. This is how you can implement it:
try {
# Code that may fail
} catch {
# Code to handle failures
Write-Host "An error occurred: $_"
}
This ensures your script continues to run smoothly, even when unexpected errors occur.
Optimizing Performance
Using the Pipeline effectively is crucial for performance. Instead of storing data in variables and then processing them, the pipeline allows you to send the output of one cmdlet directly into another, reducing resource consumption and execution time.
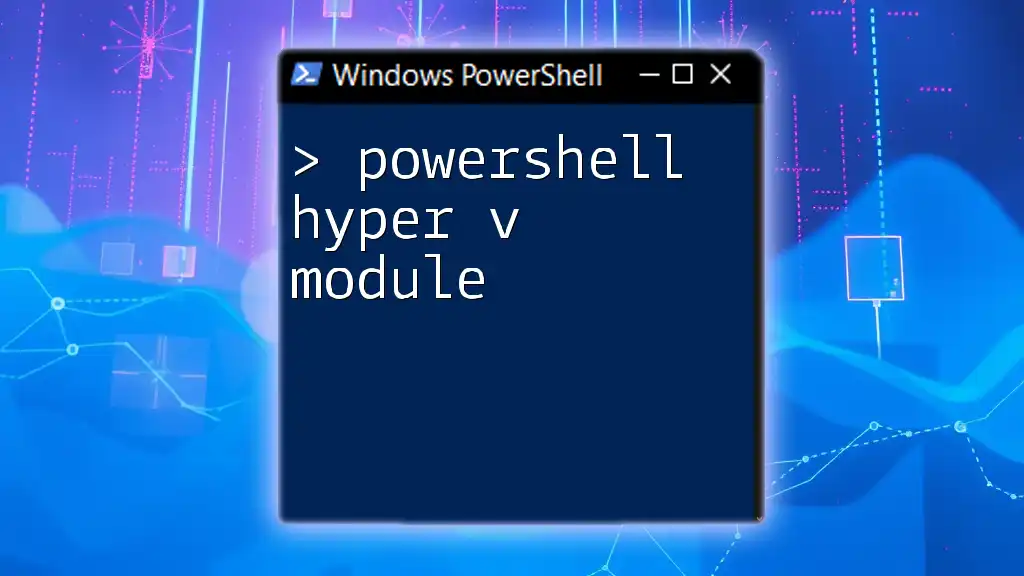
PowerShell Community Tools and Resources
Online Documentation
The Microsoft PowerShell Documentation provides comprehensive coverage of all cmdlets, syntax, and examples, invaluable for users of any skill level.
Community Forums
Numerous forums and blogs like the PowerShell Community Forum, Stack Overflow, and tech blogs dedicated to PowerShell offer peer support and additional resources, fostering a collaborative learning environment.
Useful PowerShell Modules
Several popular modules, such as `PSSQL` for SQL Server management and `PSTools` for advanced process management, greatly enhance PowerShell's capabilities, allowing users to undertake specialized tasks seamlessly.
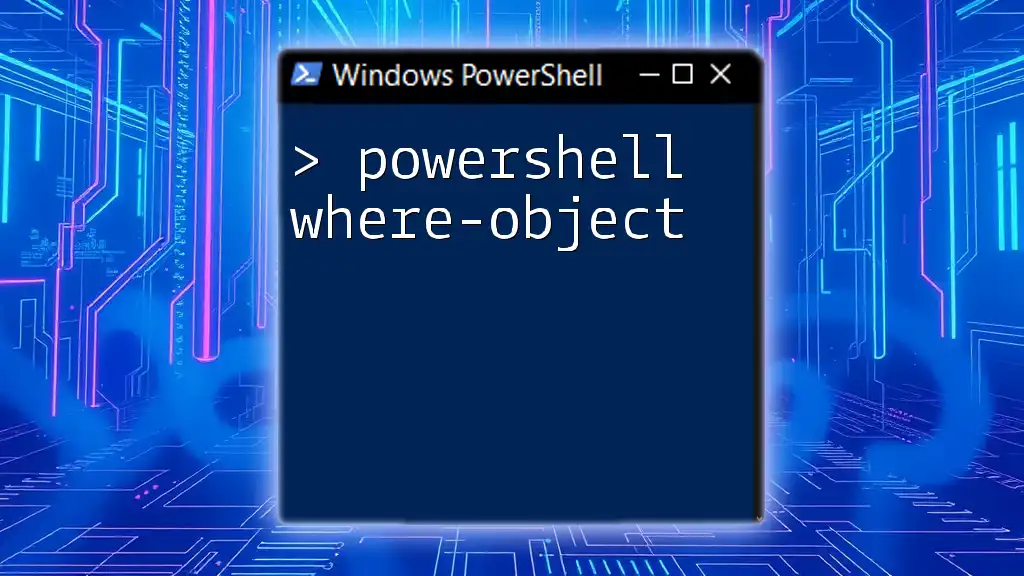
Conclusion
PowerShell helpers are crucial components for anyone looking to master PowerShell commands efficiently. From built-in cmdlets and modules to community resources and best practices, utilizing these helpers can significantly boost productivity and ease of scripting. Embrace the power of PowerShell helpers, practice frequently, and don't hesitate to leverage the vast community resources for your growth and understanding.