The PowerShell pipe (`|`) is a powerful feature that allows you to pass the output of one command directly as input to another command, enabling efficient data processing and manipulation.
Here’s a simple example:
Get-Process | Where-Object { $_.CPU -gt 100 }
This command retrieves all running processes and filters them to display only those that are using more than 100 CPU seconds.
What is a Pipe in PowerShell?
In PowerShell, a pipe is a powerful tool that allows the output of one command (or cmdlet) to be used as the input for another command. This creates a chain of commands that can perform more complex operations without the need for intermediate files. Using pipes helps streamline scripts, enhancing the efficiency of data processing and manipulation.
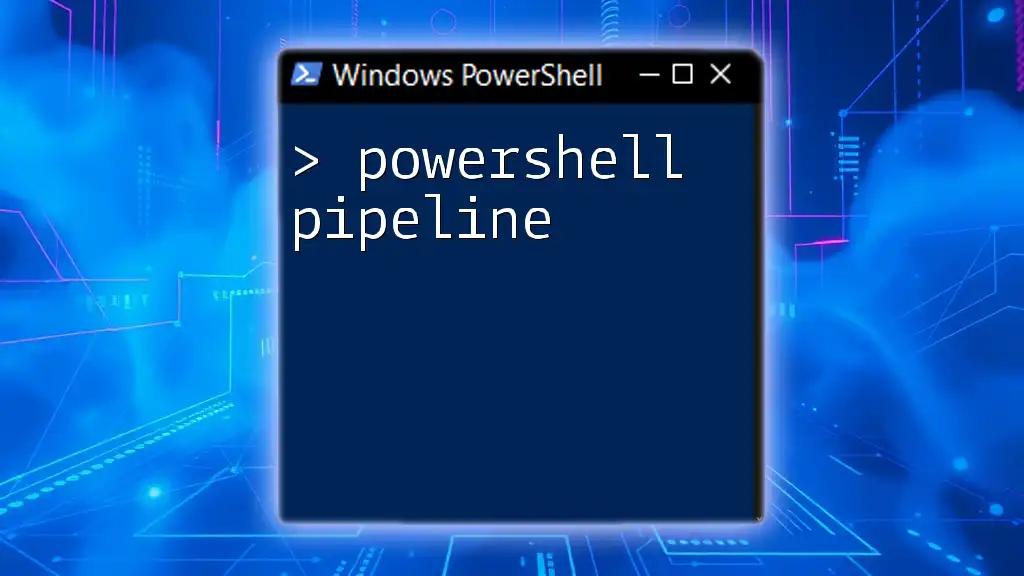
How Pipes Work
Data Flow Through Pipes
When you use a pipe, you're enabling a continuous flow of data from one command to the next. Each command in the pipeline processes the data and passes its output to the subsequent command. This data streaming nature allows for a seamless integration of functionalities. To visualize this, imagine a factory assembly line where each worker passes their finished product to the next person for further processing.
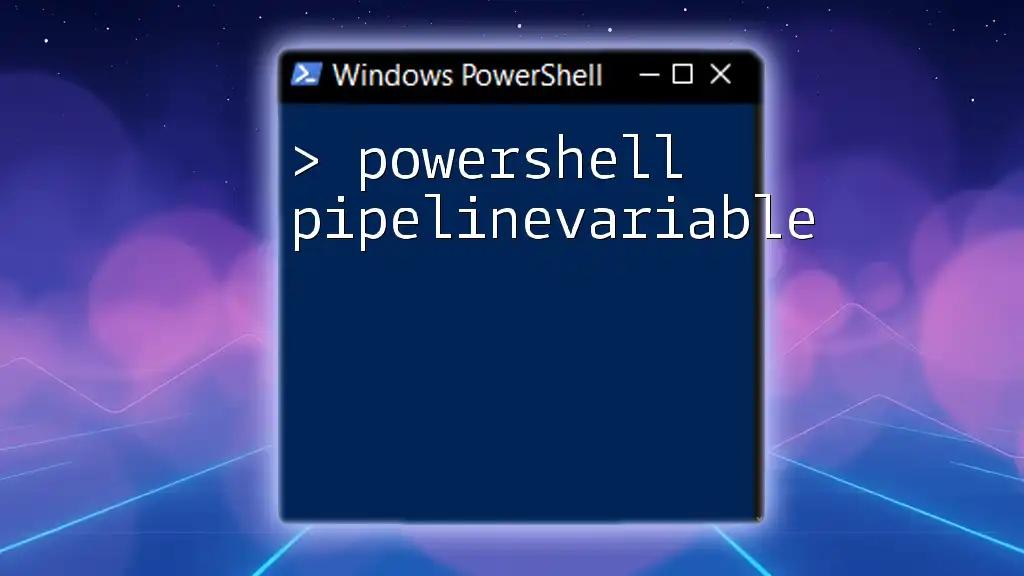
Understanding Cmdlets and Objects
What are Cmdlets?
Cmdlets are the native PowerShell functions designed to perform specific tasks. Each cmdlet follows a verb-noun naming convention, which makes them intuitive and easy to understand. Examples include `Get-Process`, `Set-Location`, and `Remove-Item`. Cmdlets form the backbone of PowerShell's functionality.
Understanding .NET Objects
In PowerShell, everything revolves around objects. PowerShell cmdlets typically output .NET objects, which have properties and methods that determine their behavior and characteristics. Familiarizing yourself with these objects is essential for effectively working with pipes, as this knowledge allows you to manipulate and filter data as needed.
Working with Objects
Consider the following example:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this command, `Get-Process` retrieves all running processes (returned as objects), and `Where-Object` filters those objects where the CPU time is greater than 100, showcasing the ease of manipulating objects through pipes.
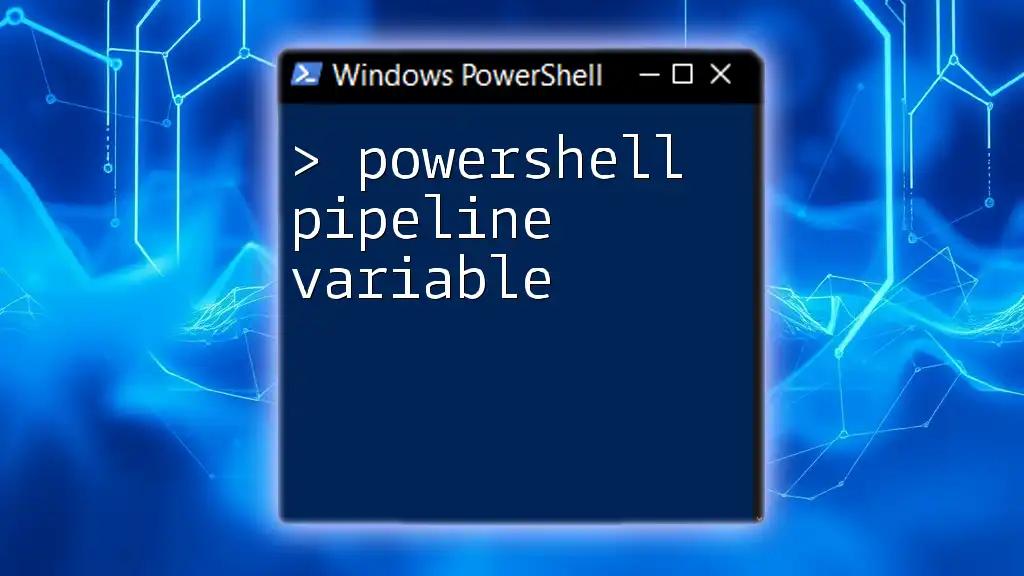
Basics of Using Pipes
Basic Syntax of a Pipe
The standard syntax for using a pipe in PowerShell is to place the pipe operator (`|`) between two commands. The command on the left processes data and pipes it to the command on the right. A simple example of this is:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this case, the output from `Get-Process` becomes the input for `Where-Object`.
Chaining Multiple Commands
One of the most powerful aspects of pipes is the ability to chain multiple commands together. This process allows for complex operations and data manipulation to occur in a streamlined and efficient manner. For example:
Get-Service | Where-Object { $_.Status -eq 'Running' } | Sort-Object DisplayName
In this code snippet, we're retrieving all services, filtering to show only running services, and then sorting those results by their display names—demonstrating how versatile command chaining can be.
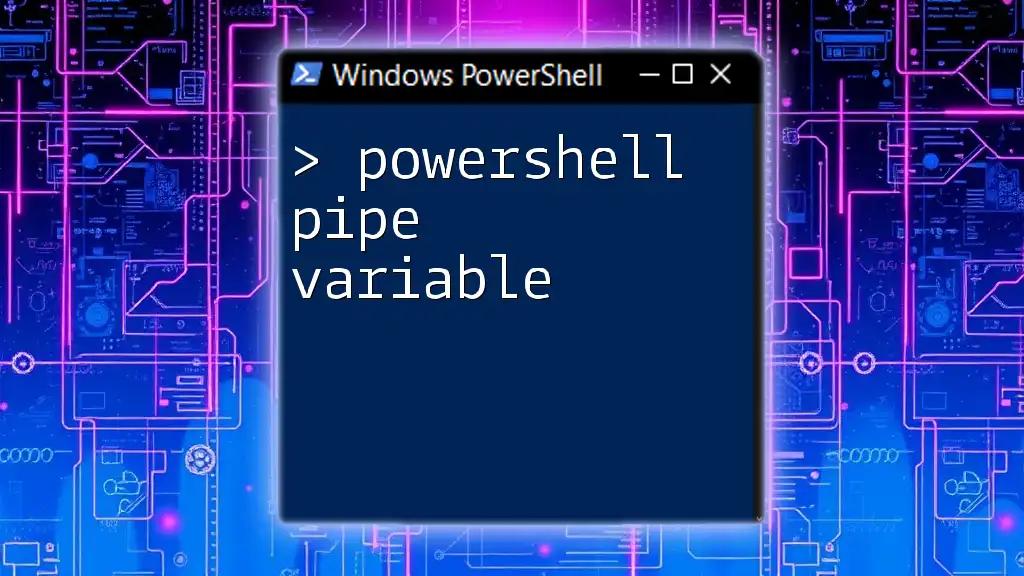
Practical Examples of Pipes
Filtering Data with Pipes
Filtering data is one of the most common uses of pipes in PowerShell. For example, the command:
Get-EventLog | Where-Object { $_.EntryType -eq 'Error' }
Let's break it down: `Get-EventLog` retrieves the event log entries, and `Where-Object` filters those to show only entries classified as 'Error'. This showcases how effective pipes are in extracting usable data from larger datasets.
Sorting and Formatting Output
Formatting output neatly can also be accomplished with pipes. For instance:
Get-Process | Sort-Object CPU | Format-Table -Property Name, CPU
This command retrieves a list of processes, sorts them by CPU usage, and formats the output into a table displaying only the Name and CPU properties, making the information easier to read and analyze.
Exporting Piped Data
Another useful application of pipes is when you need to export data. Using pipes, you can easily save processed data into formats like CSV or text files. Consider the following command:
Get-Content file.txt | Select-String 'Error' | Export-Csv errors.csv
In this example, `Get-Content` reads a text file, `Select-String` searches for occurrences of the word "Error," and `Export-Csv` saves the results in a new CSV file. This shows how pipes enable both manipulation and storage in a streamlined manner.

Advanced Pipe Techniques
Using Select-Object
The `Select-Object` cmdlet can be utilized within a pipeline to select specific properties from objects. This is particularly useful when you only need a subset of data. For example:
Get-ChildItem | Select-Object Name, Length
Here, `Get-ChildItem` retrieves files and directories, and `Select-Object` filters the results to show only the Name and Length properties, enhancing clarity and focus.
Using Group-Object
You can also use `Group-Object` in your pipelines to organize data into groups based on a common property. For instance:
Get-Process | Group-Object -Property Name
This command groups processes by their names, allowing you to see how many processes belong to each name category, which aids in summarizing data effectively.
Using ForEach-Object
For more advanced scenarios, `ForEach-Object` can be very powerful for iterative operations. Here’s an illustration:
Get-Process | ForEach-Object { $_.Name + " uses " + $_.CPU + " CPU time" }
In this example, each process is processed, and a custom string displaying the process name along with its CPU time is generated. This versatility allows for tailored outputs based on the data received.

Best Practices for Using Pipes
Keep it Concise
When using pipes, simplicity is key. Keep your commands concise and to the point, as overly complex pipelines can become difficult to debug and maintain.
Error Handling in Pipelines
Effective error handling is crucial when working with pipelines. Use techniques such as `Try/Catch` blocks or the `-ErrorAction` parameter to catch potential errors gracefully.
Performance Considerations
Utilizing pipes can actually enhance script performance. By passing objects directly from one command to the next, you reduce memory load compared to storing intermediate results in variables or files.

Troubleshooting Pipelines
Common Errors and Solutions
As with any tool, errors will occasionally occur when using pipes. Common issues might include type mismatches or properties that are not available in specific objects. Always check the output type of objects to ensure compatibility between commands.
Debugging Pipeline Commands
Debugging piped commands can be done effectively using the `-Debug` and `-Verbose` options to provide more information about the command execution, helping you identify where things may have gone awry.

The Power of Pipes in PowerShell
In conclusion, mastering the PowerShell pipe is essential for anyone looking to leverage PowerShell effectively. By understanding how to chain commands, filter and manipulate data, and take advantage of advanced techniques, you can significantly improve your scripting efficiency. Pipes not only enhance readability but also enable powerful data manipulation capabilities.

Further Reading and Resources
To deepen your understanding of PowerShell and pipes, consider exploring additional resources such as documentation, online courses, and community forums. Engaging with these materials will further enhance your skills and knowledge in this versatile scripting environment.

Useful Cmdlets for Piping
Familiarize yourself with cmdlets that work well within pipelines, such as `Get-Process`, `Select-Object`, `Where-Object`, `Sort-Object`, and many others. Knowing these commands will allow you to create effective and efficient pipelines.
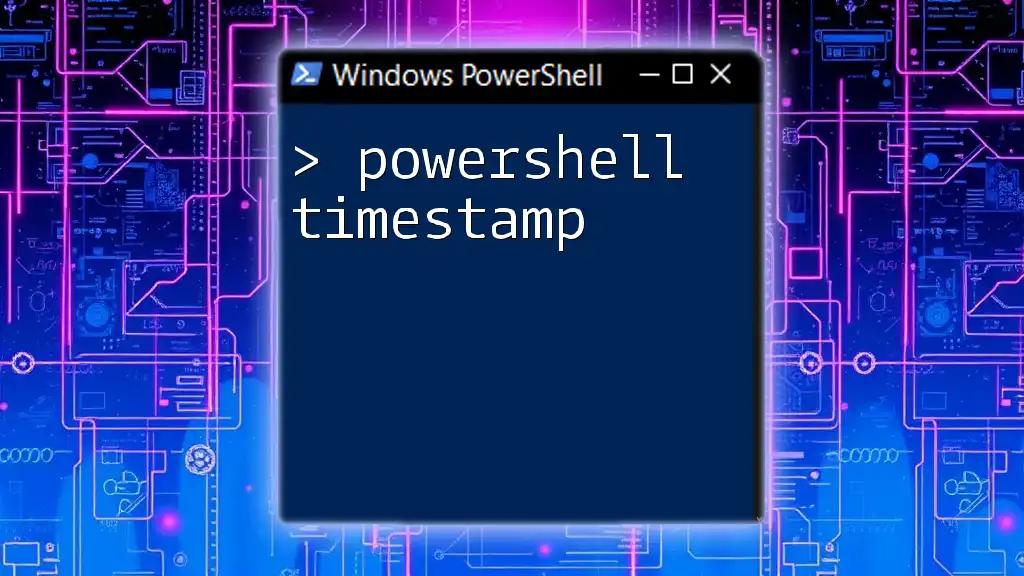
Sample Scripts
Creating sample scripts that utilize various piping techniques can be an excellent way to practice and reinforce your learning. Hands-on experience will solidify your understanding and enable you to troubleshoot issues as they arise in your PowerShell workflows.