In PowerShell, you can pipe the output of a command to a variable using the following syntax:
$myVariable = Get-Process | Where-Object { $_.CPU -gt 100 }
In this example, the variable `$myVariable` will store the processes that consume more than 100 CPU resources.
Understanding Variables in PowerShell
What Are Variables?
In PowerShell, variables are essential components that store data values for use throughout your scripts. They act as containers that hold information, which can be modified or retrieved later in your code. By understanding how to utilize variables effectively, you can enhance the efficiency and readability of your scripts.
Types of Variables in PowerShell
PowerShell features different types of variables:
-
Automatic Variables: These are pre-defined by PowerShell and provide context about your session. Examples include:
- `$PSVersionTable`: Provides information about the version of PowerShell in use.
- `$env`: Accesses environment variables.
-
User-Defined Variables: These are created by users and are essential for storing custom values. You define them using the `$` symbol followed by a name of your choice, e.g., `$myVariable = "My Value"`.
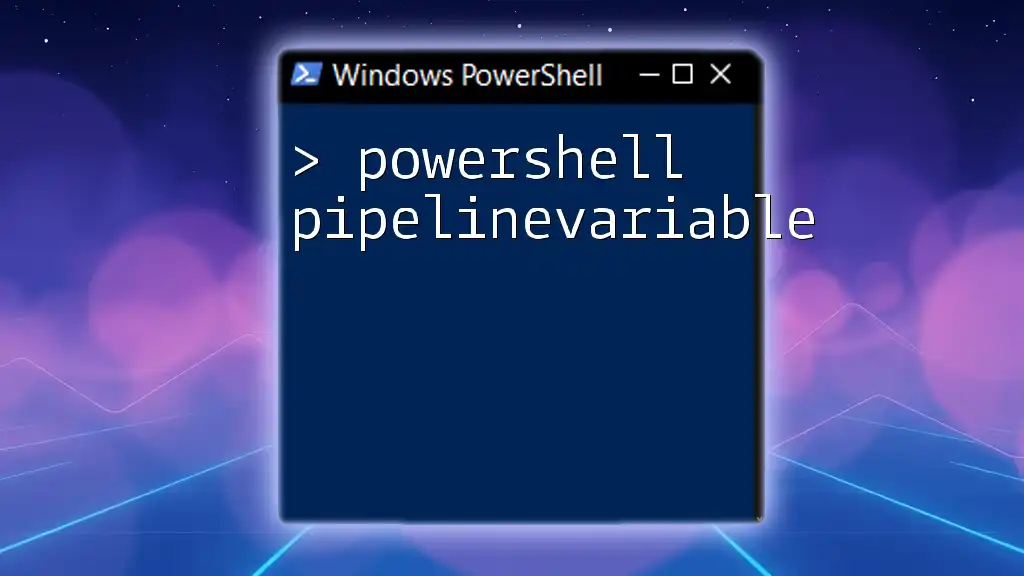
The Concept of Piping in PowerShell
What is Piping?
Piping is a powerful feature in PowerShell that allows you to send the output of one command directly into another command. This creates a seamless workflow where the results of a command become the input for the next, enabling efficient data manipulation without the need for intermediate files.
Basic Syntax of Pipe (`|`)
The pipe operator (`|`) is used to connect commands. For instance, consider the following example:
Get-Process | Sort-Object CPU
In this command, the output from `Get-Process` is sent through the pipe to `Sort-Object`, which rearranges the data based on CPU usage.
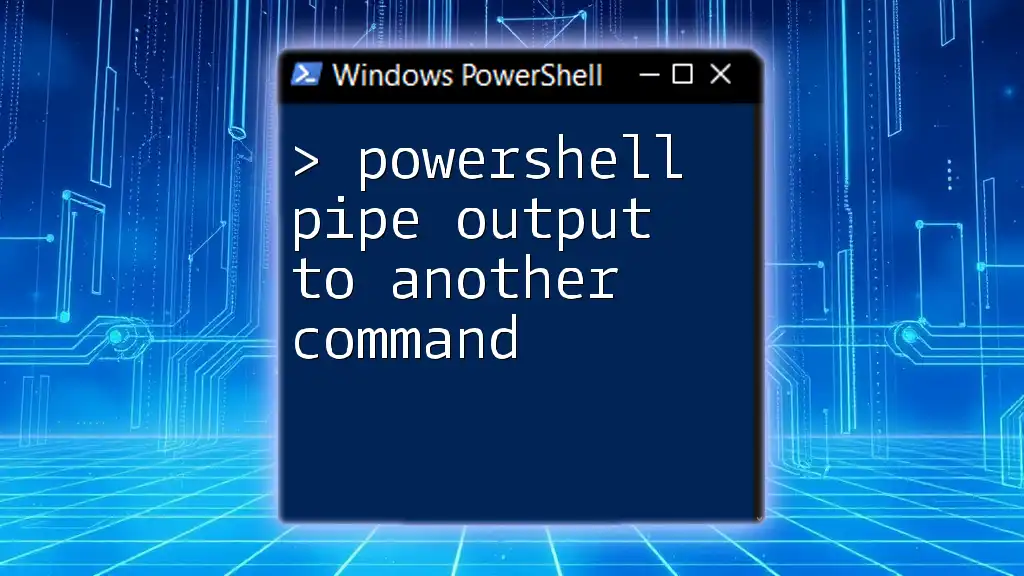
How to Pipe Output to Variables in PowerShell
Using the Pipe Operator to Assign Output
You can effectively capture the output from commands and assign it to a variable using piping. This allows you to operate on that output later in your scripts. For example:
$processList = Get-Process | Select-Object -First 5
In this example, the first five processes running on your system are stored in the variable `$processList`. This makes it easy to reference or manipulate this data later.
Storing Different Types of Outputs
Storing Text Outputs
You can pipe commands that return text output into variables. Here’s how you can capture the computer's name:
$hostname = Get-Content env:COMPUTERNAME
The variable `$hostname` now holds the name of the computer, which can be used for system identification or logging purposes.
Storing Object Outputs
Commands may return more complex objects rather than just text. For instance:
$processes = Get-Process | Where-Object { $_.CPU -gt 0 }
In this case, `$processes` will contain all processes that are utilizing CPU resources, returning a collection of process objects for further analysis.
Storing Multiple Outputs
When you expect multiple items from a command, variables can behave like arrays. For example:
$groupMembers = Get-ADGroupMember -Identity "GroupName"
Here, `$groupMembers` stores all members of a specific Active Directory group, ready to be processed in your script.
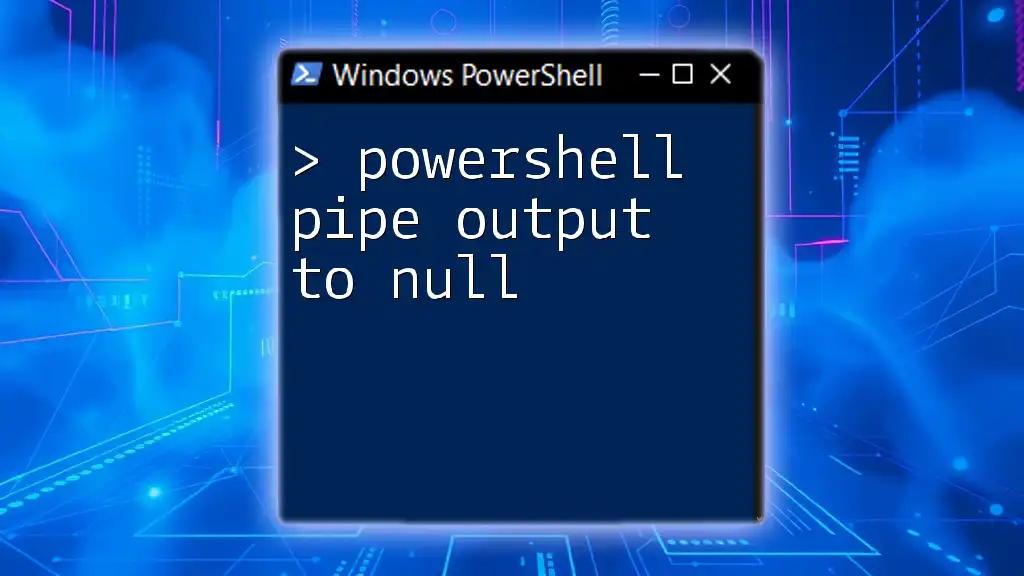
Best Practices for Piping Output to Variables
Readability and Maintainability
When working with variables, it’s crucial to maintain clarity. Choose descriptive variable names that reflect their contents, such as `$runningServices` instead of `$a`. This ensures anyone reviewing your code can quickly understand its purpose. Additionally, adding comments can help clarify complex command chains for future reference.
Error Handling
Use proper error handling when piping output to variables to ensure your script runs smoothly without interruption. Implement error management using `try` and `catch`, as illustrated below:
try {
$user = Get-ADUser -Identity "username" -ErrorAction Stop | Select-Object Name
} catch {
Write-Host "Error occurred: $_"
}
This example captures errors that might occur if `Get-ADUser` cannot find the user, providing feedback instead of abruptly stopping the script.
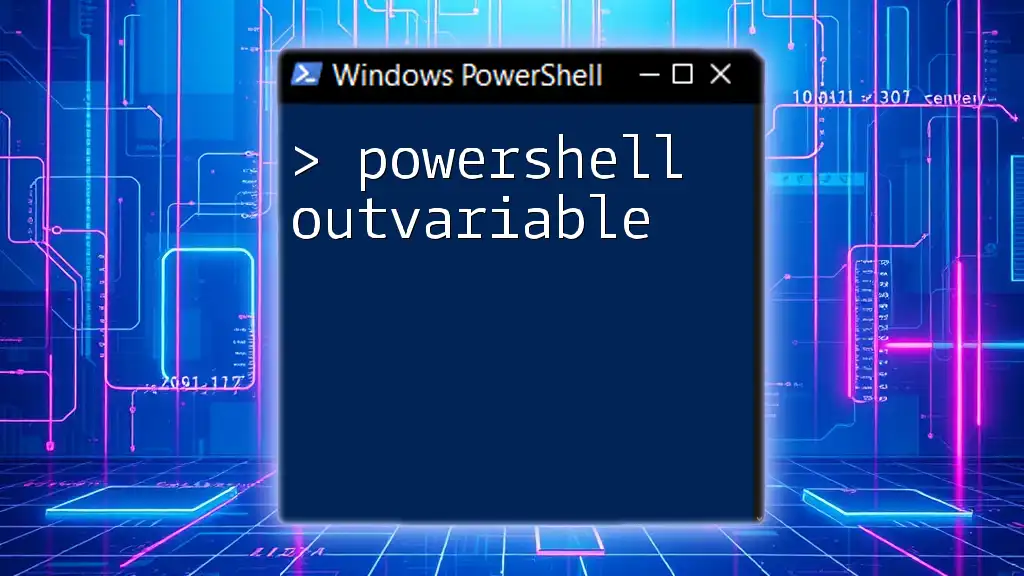
Practical Use Cases for Piping Output to Variables
System Administration
In system administration tasks, piping can significantly enhance your productivity. For example:
$services = Get-Service | Where-Object { $_.Status -eq 'Running' }
The `$services` variable now holds all running services, which can be analyzed or manipulated as needed.
File Management
When managing files, it's useful to filter and process only the relevant data. A common example:
$files = Get-ChildItem -Path C:\Temp | Where-Object { $_.Length -gt 1MB }
This command captures all files greater than 1MB in size in the specified directory, storing them in the `$files` variable for subsequent actions like deletion or archiving.
Data Reporting
Generating reports can be automated with PowerShell, making it a breeze. For instance:
$reportData = Get-EventLog -LogName Application | Group-Object Source | Sort-Object Count -Descending
The `$reportData` variable contains a grouped list of event sources sorted by their occurrence. This can be essential for system diagnostics or audits.
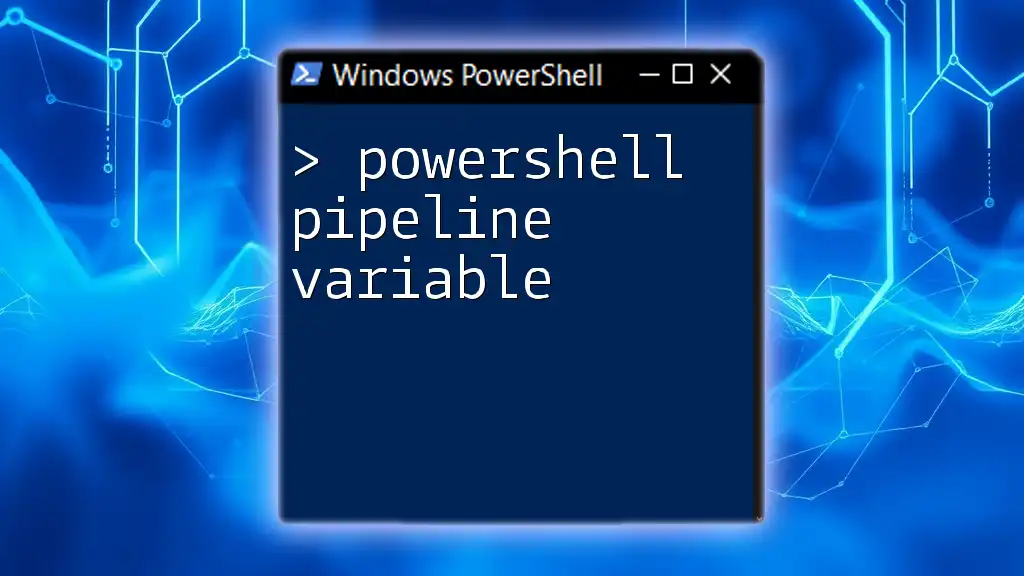
Common Pitfalls When Piping Output to Variables
Misunderstanding Object Types
One common pitfall is misunderstanding the types of objects returned by commands. For example, commands may return single objects or collections of objects which can affect how you manipulate and reference them later. Always review the data type when piping output to ensure you're handling the data correctly.
Overwriting Variables
When working with multiple pieces of information, be mindful of variable naming. When you reuse a variable name, it can lead to unexpected behavior and data loss:
$services = Get-Service
# Later in the script
$services = Get-Process # This overwrites the previous data
Using unique variable names or ensuring that codes are structured to avoid conflicts is crucial for maintaining data integrity.
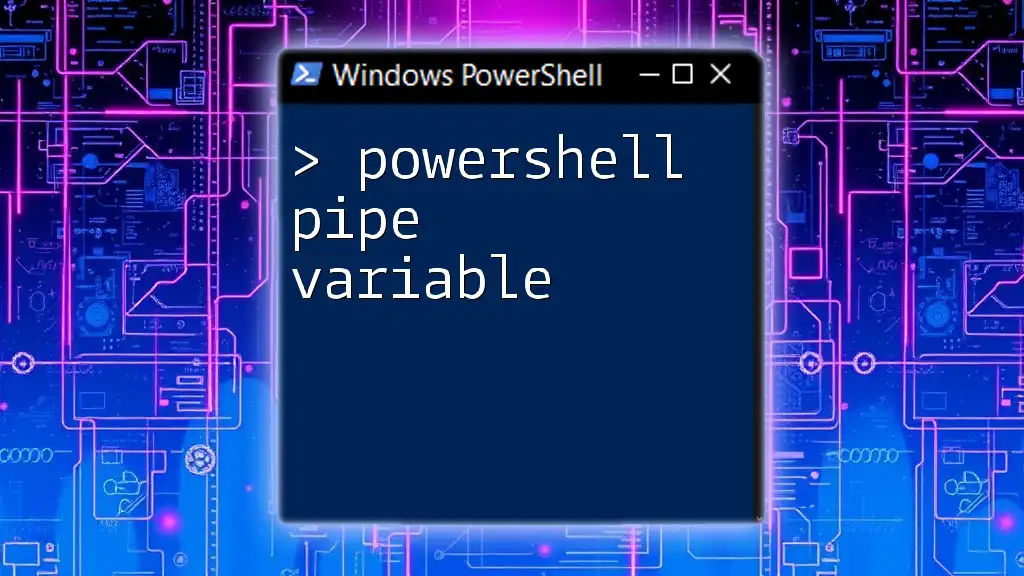
Conclusion
Piping output to variables in PowerShell is an effective technique that can transform your scripts from cumbersome and inefficient to streamlined and powerful. With a strong grasp of variables, piping, and best practices, you can maximize the capabilities of PowerShell, leading to more organized, faster, and effective automation solutions. Experiment with piping in your scripts and share your findings for collaborative learning and growth in the PowerShell community.
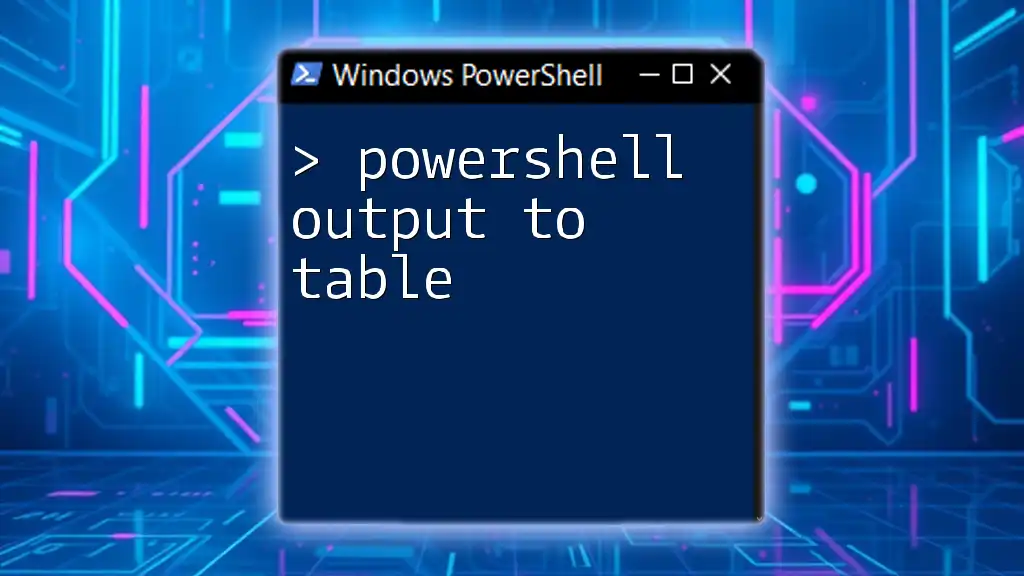
Additional Resources
For those eager to deepen their understanding, consider referring to the [official PowerShell documentation](https://docs.microsoft.com/en-us/powershell/), exploring recommended books, and engaging with community forums where you can share insights and learn from fellow enthusiasts.