In PowerShell, a pipeline variable allows you to store data flowing through the pipeline for further use in your commands, enabling more efficient scripting.
Here's a code snippet demonstrating the use of a pipeline variable:
$items = Get-Process | Where-Object { $_.CPU -gt 100 } | ForEach-Object { $_.Name }
Write-Host "Processes using more than 100 CPU seconds: $items"
Understanding the PowerShell Pipeline
What is a Pipeline?
The PowerShell pipeline is a powerful feature that allows you to pass the output of one command directly into another command. This chaining of commands simplifies complex processes and enhances overall efficiency by enabling the output of one command to be used as input for the next. The power of the pipeline lies in its ability to allow data to flow seamlessly between commands, thus creating a streamlined approach to processing and manipulating data.
Purpose of the Pipeline
The pipeline serves various purposes, including:
- Efficiency: Reduces the need for intermediate variables by connecting commands directly.
- Modularity: Enables you to break down complex tasks into smaller, manageable functions.
- Readability: When used properly, it can lead to scripts that are easier to read and understand.
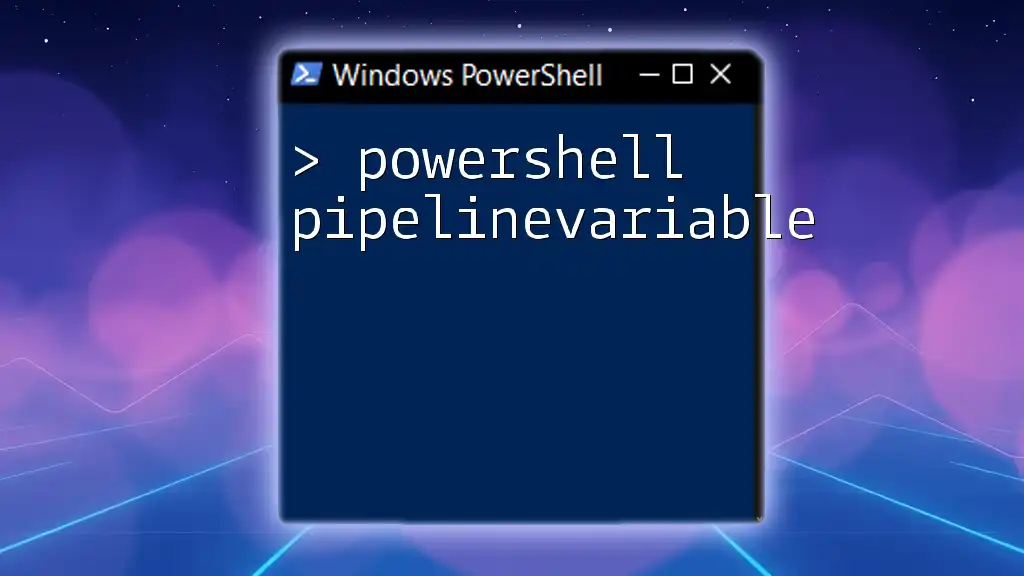
Introducing Pipeline Variables
What are Pipeline Variables?
Pipeline variables are variables that are specifically created to hold the output of individual pipeline objects as they are processed. Unlike regular PowerShell variables, which can hold a single value or object, pipeline variables are designed to work within the context of the pipeline's operations.
Importance of Pipeline Variables
Pipeline variables are beneficial in various scenarios:
- They provide a means to reference the current object being processed within a loop.
- They simplify the understanding of what data is currently being manipulated, particularly in larger scripts.
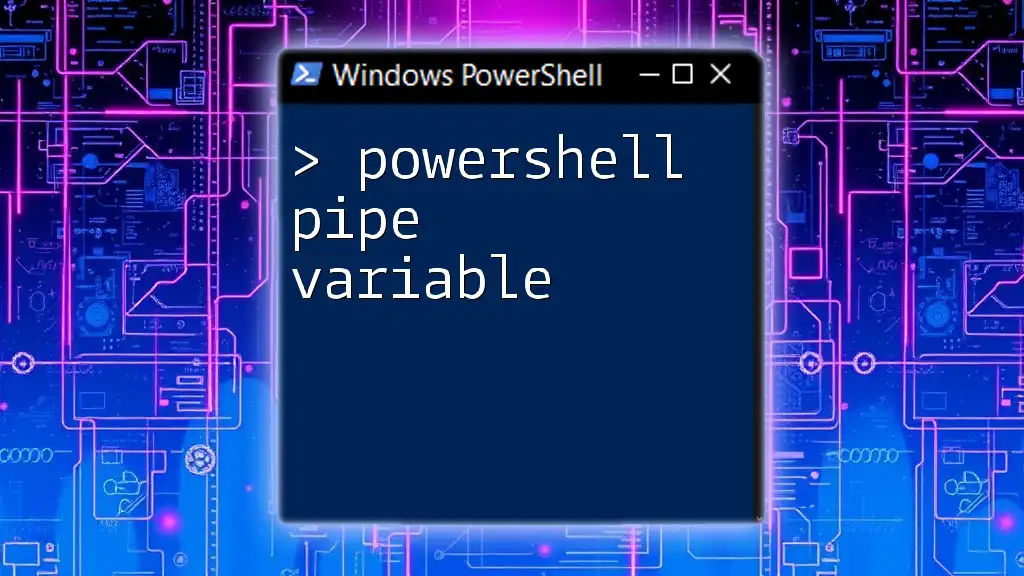
Creating and Using Pipeline Variables
Syntax of Pipeline Variables
To declare and use pipeline variables, you generally assign them within script blocks. The syntax revolves around the special variable `$_`, which represents the current object in the pipeline. However, you can also create your custom variables for clarity in more complex operations.
Example 1: Simple Use of a Pipeline Variable
In this example, we will inspect running processes and display their names and IDs:
Get-Process | ForEach-Object { $proc = $_; "Process: $($proc.Name), ID: $($proc.Id)" }
This script uses the `Get-Process` cmdlet to retrieve a list of running processes. Inside the `ForEach-Object` cmdlet, `$proc` is assigned the value of the current object `$_`, enabling us to access its properties (Name and Id) for display.
Example 2: Using Pipeline Variables in Complex Situations
Let's consider filtering services based on their status. The script below identifies running services:
Get-Service | Where-Object { $svc = $_; $svc.Status -eq 'Running' } | Select-Object Name, Status
Here, `$svc` is utilized to store the output of each service object. The `Where-Object` cmdlet checks if the service status equals 'Running' and passes this filtered selection to `Select-Object` to display the relevant properties.
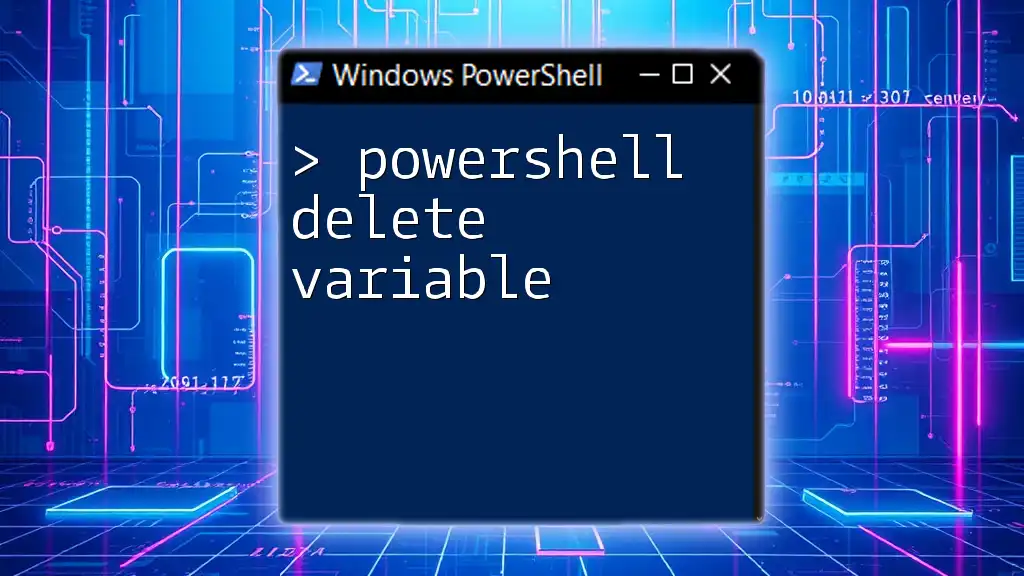
Best Practices for Using Pipeline Variables
Naming Conventions
When creating pipeline variables, it’s crucial to adhere to clear naming conventions. Use meaningful names that describe the data they hold. For instance, prefer `$service` over `$s` or `$proc` over `$p` for better clarity.
Avoiding Common Pitfalls
Using pipeline variables can lead to confusion if mismanaged. Common mistakes include:
- Overusing `$_` when you could define a clearer variable.
- Forgetting to reference properties correctly, leading to errors in output.
To help debug, you can insert `Write-Host` within your loops to display the current variable values.
Maintaining Readability within Code
Strive to maintain readability in your code by:
- Breaking complex logic into smaller functions.
- Adding comments that clarify the purpose of your pipeline variables.
- Structuring your scripts in a way that logically separates different operations.
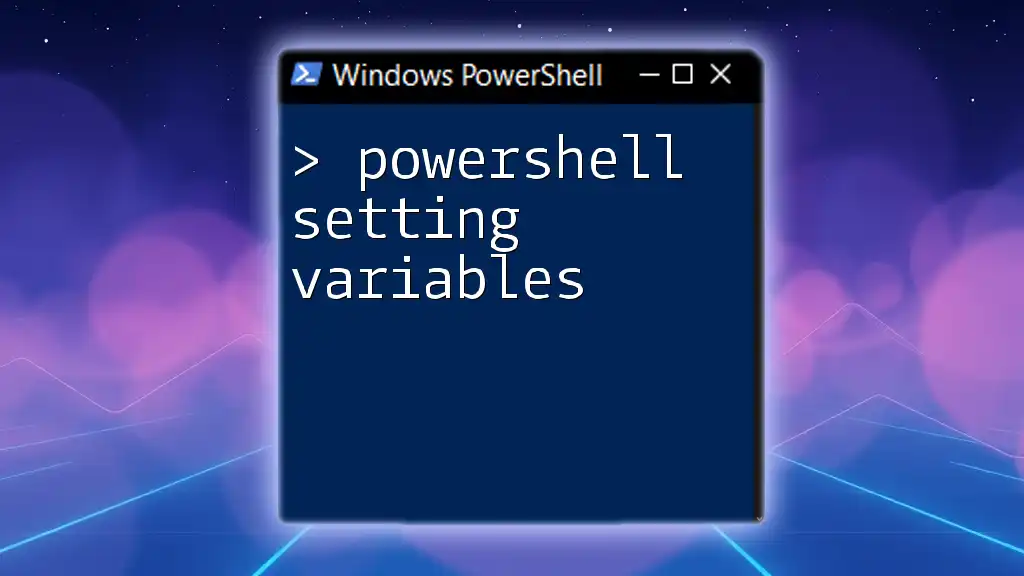
Advanced Usage of Pipeline Variables
Chaining Multiple Pipeline Commands
You can chain multiple commands in a way that employs several pipeline variables for more complex tasks. For instance, filtering processes, then piping them into another command:
Get-Process | Where-Object { $proc = $_; $proc.WorkingSet -gt 1MB } | Select-Object Name, Id
Pipeline Variables in Functions
PowerShell pipeline variables can also be used within functions. Consider the following function that retrieves running processes:
function Get-RunningProcesses {
param()
Get-Process | ForEach-Object { $proc = $_; $proc.Name }
}
In this script, the `Get-RunningProcesses` function retrieves processes and outputs their names, using `$proc` to hold each process object throughout the loop.
Utilizing `$_` with Pipeline Variables
While you can use custom pipeline variables, utilizing `$_` is equally valid. For example:
Get-EventLog -LogName Application | Where-Object { $_.EventID -eq 1000 }
This retrieves events from the Application log where the event ID equals 1000, showcasing the straightforward yet powerful use of `$_`.
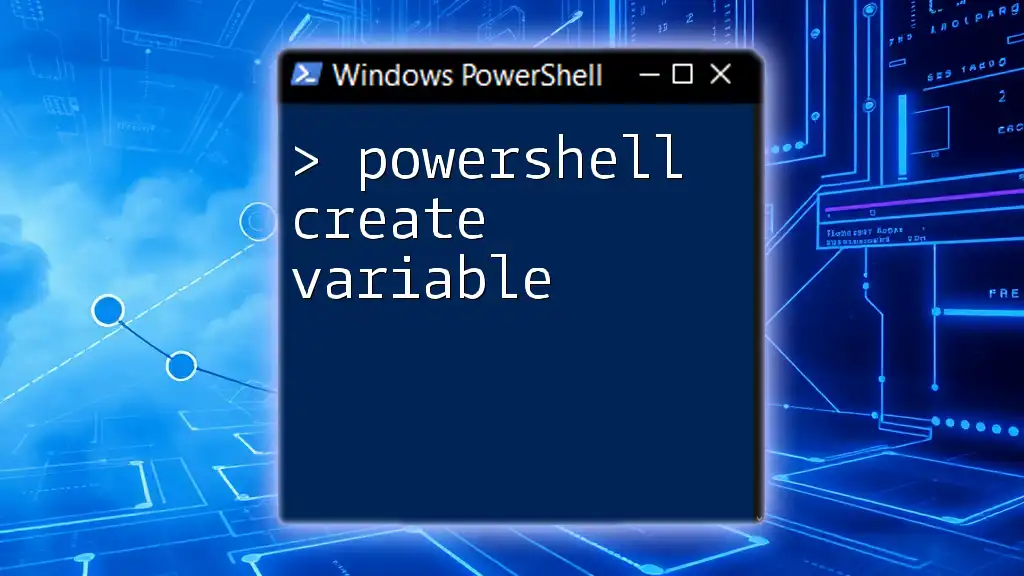
Real-world Applications of Pipeline Variables
Automating Administrative Tasks
PowerShell pipeline variables shine in automating repetitive administrative tasks, like batch user creation or health checks across multiple servers. For instance:
Get-ADUser -Filter * | ForEach-Object { Disable-ADAccount -Identity $_.SamAccountName }
This command retrieves all Active Directory users and, using a pipeline variable, disables their accounts.
Data Processing and Reporting
With pipeline variables, you can also process data efficiently. For example, you might want to filter logs and export results to a CSV file:
Get-EventLog -LogName System | Where-Object { $log = $_; $log.EventID -eq 6005 } | Export-Csv -Path "SystemEvents.csv" -NoTypeInformation
This script filters system events based on their ID and exports the results for later analysis.
Monitoring Systems and Performance
Pipeline variables are helpful for real-time monitoring. You can quickly evaluate system performance metrics:
Get-Process | Measure-Object | ForEach-Object { "Total Processes: $($_.Count)" }
In this example, you're measuring the total number of processes currently running on the system.
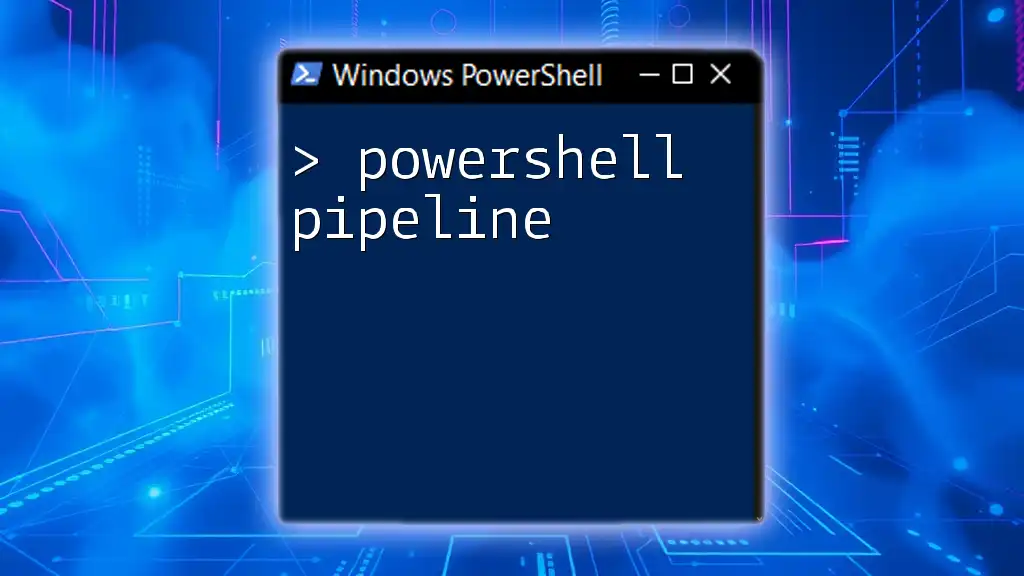
Conclusion
Understanding and applying PowerShell pipeline variables can significantly improve your scripting efficiency and effectiveness. By leveraging pipeline variables, you can create more modular, readable, and powerful scripts that can handle a variety of tasks—from automating administrative duties to processing data effectively. Mastering this concept opens the door to more advanced scripting techniques, enabling you to harness the full potential of PowerShell.
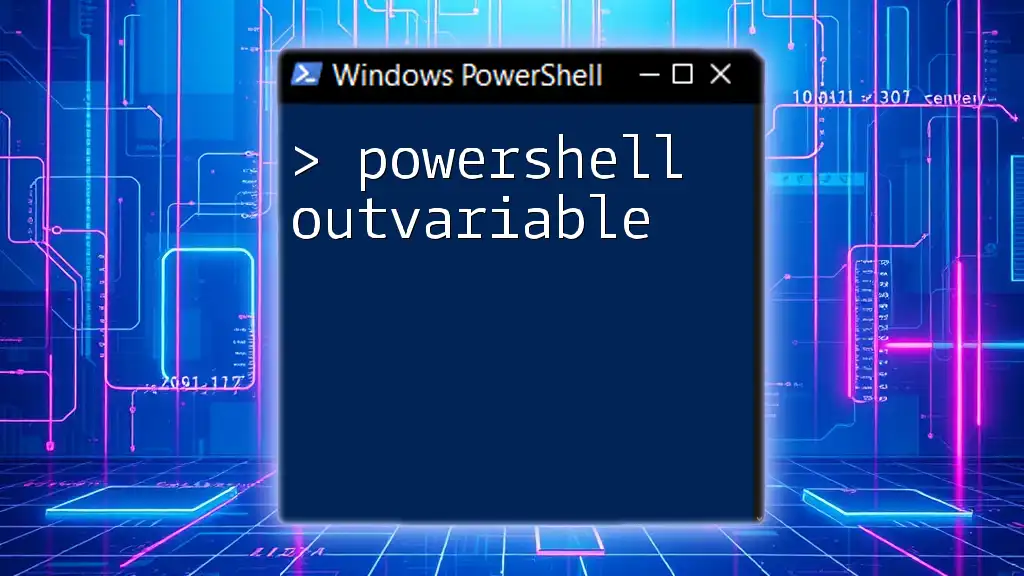
Additional Resources
For further learning, refer to the [official PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) and explore books dedicated to PowerShell scripting. Consider signing up for services that delve deeper into PowerShell commands and techniques to enhance your skills.