In PowerShell, you can append to a variable by using the `+=` operator, which adds new content to the existing value of the variable.
$myVariable = "Hello"
$myVariable += ", World!" # Appending to the variable
Write-Host $myVariable # Output: Hello, World!
Understanding Variables in PowerShell
Definition of Variables
In PowerShell, variables are elements that store data values that you can manipulate, process, and reference later in your scripts. They act as containers for holding data, and their flexibility allows you to adjust values based on the script's execution context.
Types of Variables
PowerShell supports several variable types, among which the most common are:
Scalar Variables: These are single-value variables that store one item at a time.
Array Variables: These variables can hold multiple values within a single variable, making them powerful for collection management.
Hash Tables: A combination of key-value pairs, hash tables are useful for storing data related to one another contextually.
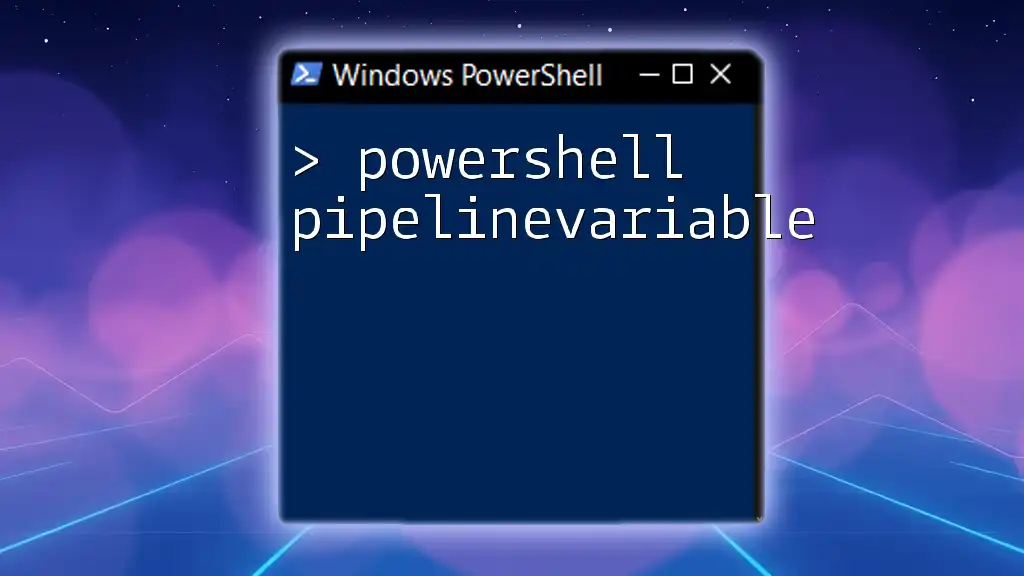
Appending Data to Variables
What Does "Appending" Mean?
Appending data to a variable refers to the process of adding information to an existing variable's content without overwriting it. This is particularly useful in scenarios where data accumulates over time, such as collecting responses, log entries, or processing multiple items dynamically.
Appending to Scalar Variables
Scalar variables are relatively straightforward. You can append data using the `+=` operator.
Example:
$greeting = "Hello"
$greeting += ", World!"
In this example, the original value of `$greeting` is "Hello". When we append ", World!", the final output will be "Hello, World!" This method efficiently concatenates strings without losing the original content.
Appending to Array Variables
Creating an Array
PowerShell allows you to create arrays easily. You can define an array by listing items separated by commas.
Example:
$numbers = 1, 2, 3
In this case, `$numbers` holds the values 1, 2, and 3.
Appending to an Array
When appending a single element, the same `+=` operator can be used, which effectively redefines the original array.
Example:
$numbers += 4
In this case, after appending, `$numbers` becomes 1, 2, 3, 4. It's critical to note that arrays in PowerShell are immutable; thus, appending creates a new array rather than modifying the existing one directly.
Appending Multiple Items to an Array
If you need to append multiple elements at once, you can do that as well.
Example:
$numbers += 5, 6, 7
After executing this line, `$numbers` will now contain 1, 2, 3, 4, 5, 6, 7. The method remains efficient and concise for expanding their contents.
Appending to Hash Tables
Hash tables in PowerShell provide a more structured way to handle paired data values. You can append new key-value pairs easily.
Example:
$person = @{"Name"="John"; "Age"=30}
$person["Occupation"] = "Developer"
In the example above, a hash table named `$person` initially includes Name and Age. By appending the Occupation, it effectively becomes Name: John, Age: 30, Occupation: Developer. This flexibility allows you to efficiently categorize related data together.
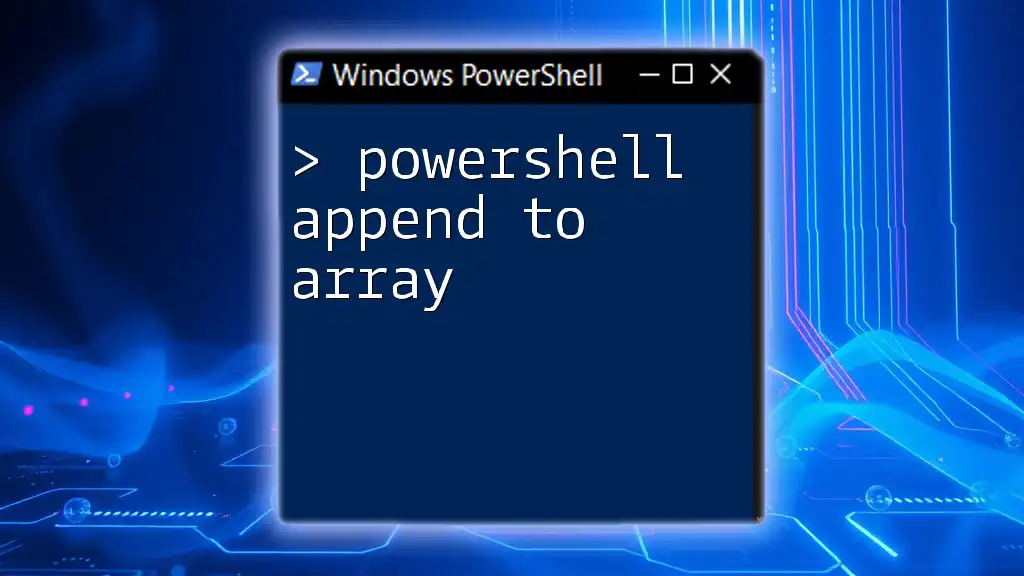
Best Practices for Appending to Variables
Avoiding Common Pitfalls
One common mistake in PowerShell is accidentally overwriting variables when appending data. Utilizing the `+=` operator allows the original content to persist. Furthermore, be cautious about the scope of your variables—local versus global variables can lead to unexpected behavior within scripts.
Performance Considerations
While appending to arrays is a straightforward task, continually appending can make scripts less efficient, particularly with large datasets. To enhance performance, consider using collections, such as `System.Collections.ArrayList`, which allow for more efficient resizing when adding items.
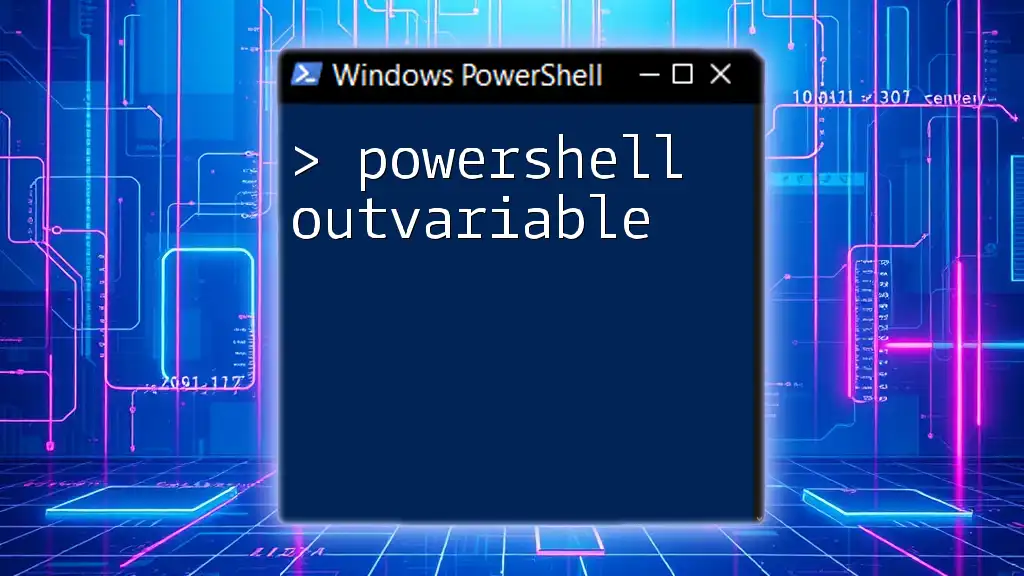
Real-World Use Cases
Automation Scripts
In practical automation scenarios, you might collect log entries or error messages throughout a script's execution. By appending these entries into an array, you can output a comprehensive log at the end of the script.
Example:
$logEntries = @()
$logEntries += "Beginning process..."
# Simulating a process
$logEntries += "Process completed successfully."
Report Generation
When generating reports, a common approach is to collect data iteratively within a loop and append it for final reporting. This allows you to maintain a coherent summary of activities or data outputs in a structured manner.
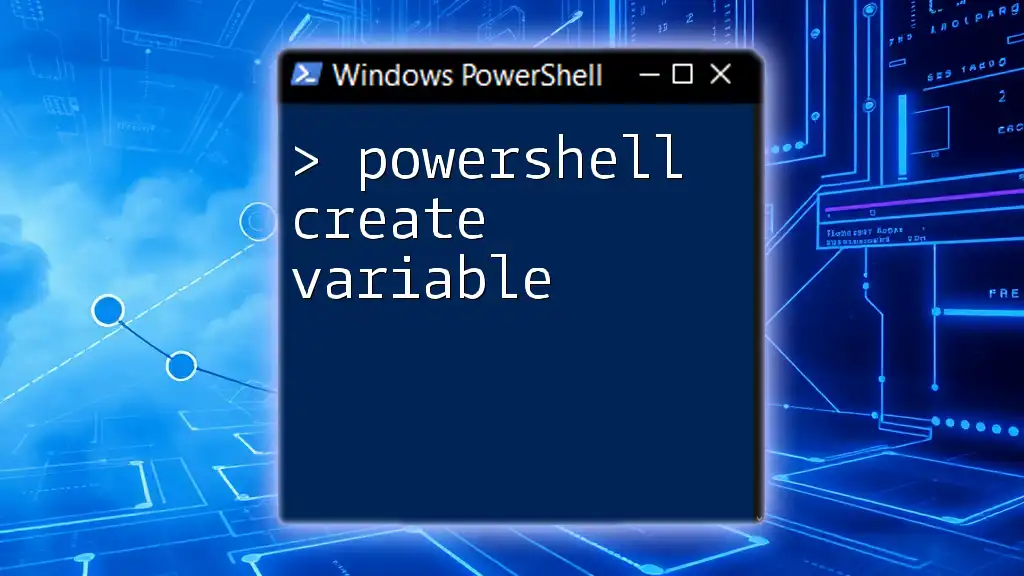
Conclusion
Understanding how to efficiently append to variables in PowerShell enhances your scripting capabilities significantly. Through the use of scalar variables, arrays, and hash tables, you can manipulate and manage data with ease. Experiment with the examples provided, and don't hesitate to dive deeper into PowerShell scripting to harness its full potential.
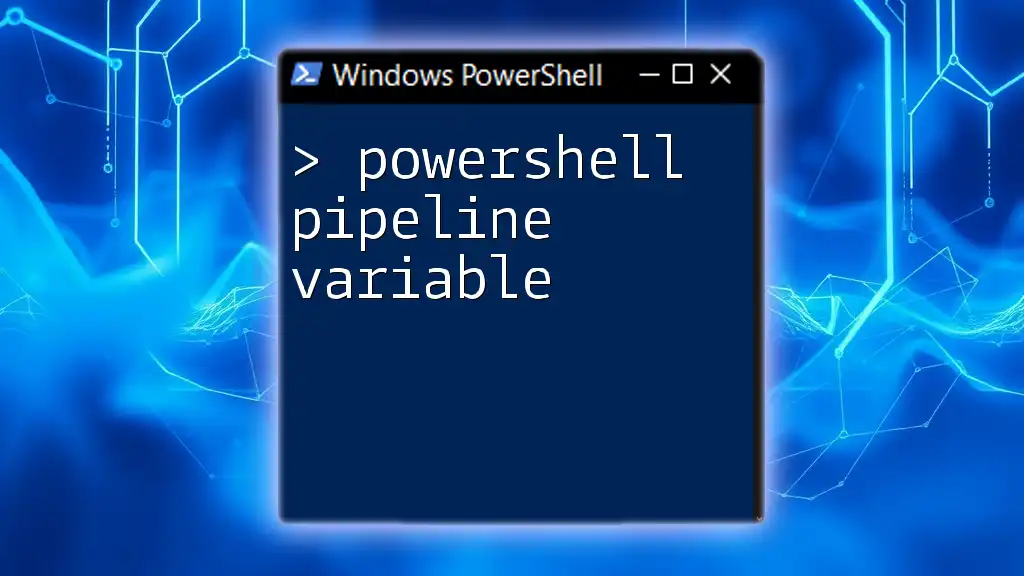
Additional Resources
For those eager to learn more, consider exploring the official PowerShell documentation or enrolling in a structured course to extend your knowledge. Stay updated by subscribing to relevant blogs or forums that focus on PowerShell commands and techniques.
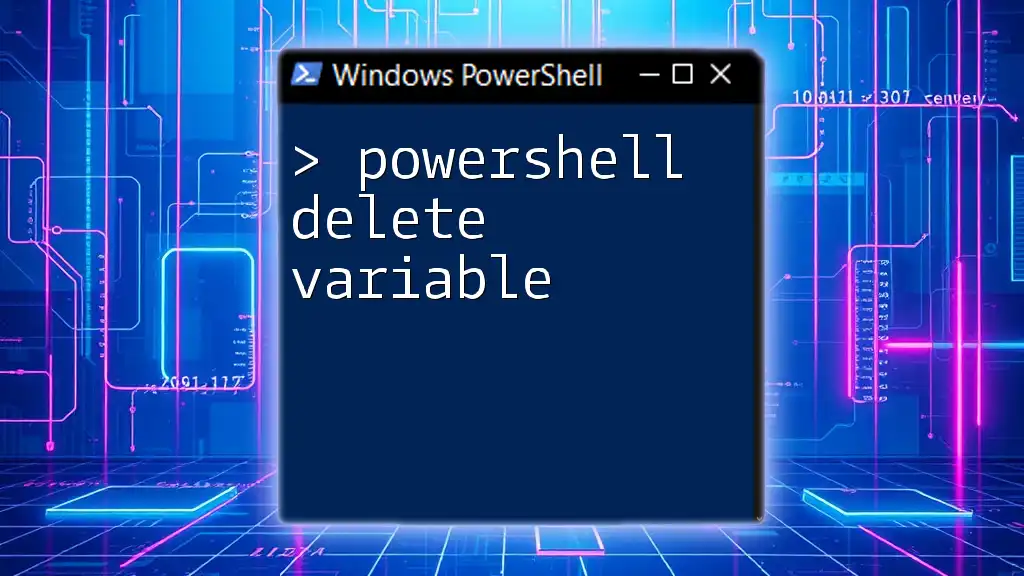
FAQs
Can I append to a read-only variable?
Read-only variables cannot be modified once set; thus, these variables don’t support appending. Understanding the difference in variable behavior helps avoid unintended errors while scripting.
Are there alternatives to appending?
Instead of appending to an array, consider using collections that inherently handle dynamic resizing, such as `ArrayList`, which offers better performance for frequent insertions.
How do I handle large datasets efficiently?
For large datasets, consider leveraging efficient data structures like `HashSet` for unique items or leveraging databases for more extensive data management. Optimizing your script with these structures can dramatically improve its speed and performance.