The `AppendChild` method in PowerShell is used to add a new child node to an existing XML node, enabling dynamic manipulation of XML data structures.
Here's a code snippet to illustrate its usage:
# Load the XML document
[xml]$xmlDoc = @"
<root>
<item>Original Item</item>
</root>
"@
# Create a new child node
$newNode = $xmlDoc.CreateElement('item')
$newNode.InnerText = 'Newly Added Item'
# Append the new child node to the root
$xmlDoc.root.AppendChild($newNode)
# Display the updated XML
$xmlDoc.OuterXml
Understanding the AppendChild Method
What is AppendChild?
The `AppendChild` method is a fundamental part of working with XML and HTML in PowerShell. It allows you to insert a new child node into an existing parent node, thereby maintaining a hierarchical data structure. This method is particularly useful when programmatically constructing XML documents or manipulating HTML content dynamically.
Key Concepts to Know
Before diving into the applications of `AppendChild`, it's essential to grasp some foundational concepts related to XML and the Document Object Model (DOM):
-
XML (eXtensible Markup Language): A markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable. PowerShell simplifies XML manipulation using built-in capabilities.
-
Nodes and Elements: In XML, an element is a basic building block that can contain text, attributes, other elements, and more. Each element is represented as a node within the XML DOM.
-
Document Object Model (DOM): This structure models the elements of an HTML or XML document as a tree, allowing for dynamic content manipulation. The `AppendChild` method directly interacts with the DOM, making it indispensable for developers.
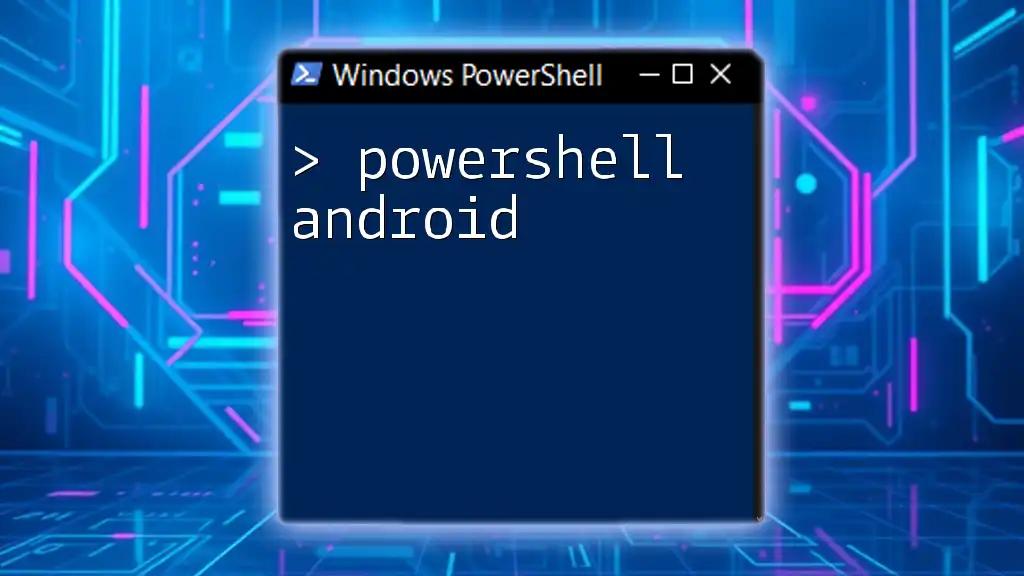
Practical Applications of AppendChild in PowerShell
Creating XML Documents
To effectively use the `AppendChild` method, you first need to create an XML document. PowerShell provides an easy way to do this. Here's how:
[xml]$xml = "<root></root>"
This line initializes a new XML document with a root node called `<root>`. By using `[xml]`, you instruct PowerShell to treat the string as an XML document.
Adding Child Nodes with AppendChild
Once you've established your base XML structure, you can start appending child nodes. Here's a simple example of how to do this using the `AppendChild` method:
$rootNode = $xml.DocumentElement
$childNode = $xml.CreateElement("child")
$rootNode.AppendChild($childNode)
In this code:
- `$rootNode` captures the root element of your XML document.
- `$childNode` creates a new element called `<child>`.
- Finally, `AppendChild` attaches the child node to the root.
This approach allows you to develop a structured XML document dynamically, which can be crucial for applications like configuration files or data repositories.
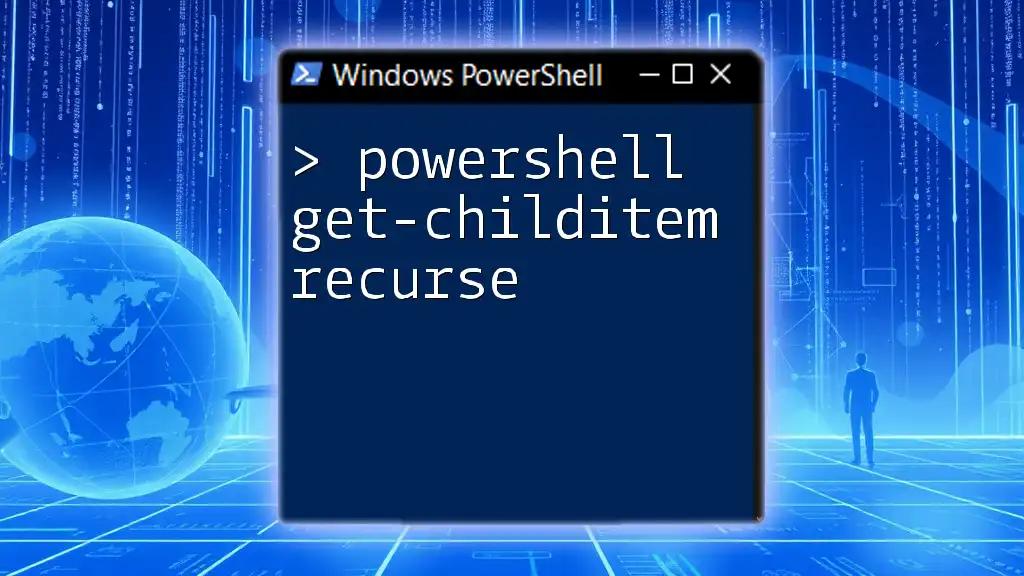
Advanced Usage of AppendChild
Appending Multiple Child Nodes
In scenarios where you need to add multiple nodes, using a loop can streamline the process. Consider the following example:
1..5 | ForEach-Object {
$childNode = $xml.CreateElement("child$_")
$rootNode.AppendChild($childNode)
}
This code iterates from 1 to 5, creating a new `<child>` node for each iteration, named `<child1>`, `<child2>`, and so on. The flexibility of loops allows for scalable XML document creation, making it ideal for bulk data manipulation.
Working with Attributes
Adding attributes to nodes enhances the information contained within your XML structure. Here’s an example of how to append an attribute to a node:
$childNode.SetAttribute("id", "1")
$rootNode.AppendChild($childNode)
In this example, the `SetAttribute` method assigns an `id` attribute to the child node. This capability allows you to store additional metadata about each element, enabling richer data structures.
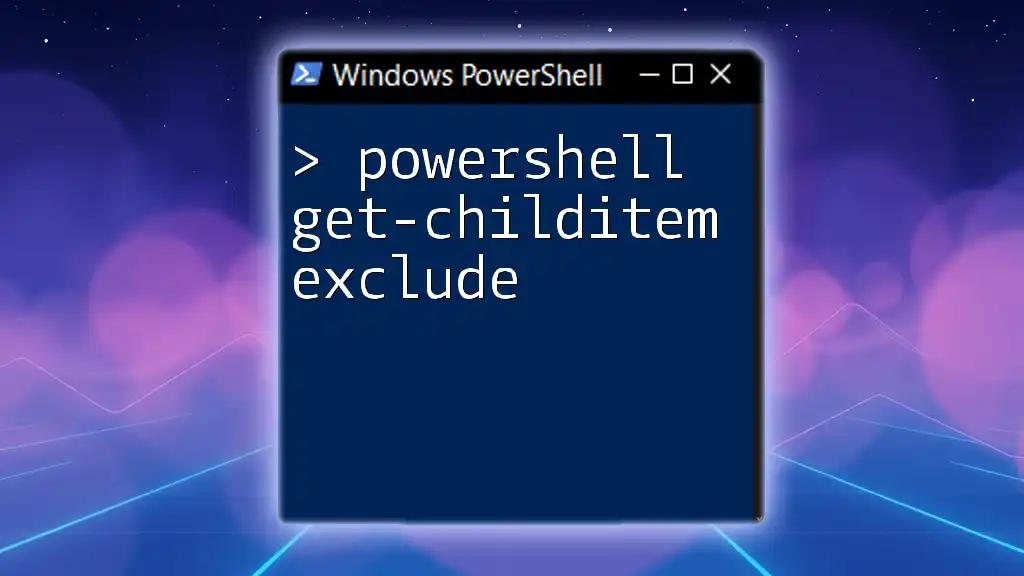
Real-World Scenarios and Use-Cases
Automating Reports with XML
A practical application of the `AppendChild` method is generating automated XML reports. For instance, if you're creating a report summarizing user data, you might structure your XML with nodes for each user, containing attributes like name, ID, and role. Using `AppendChild`, you can build the report dynamically, effectively populating it with real-time data.
Configuring Bulk Operations
Another common scenario is configuring multiple settings at once in an application. You can use `AppendChild` to append various configuration elements in your XML document, each containing the necessary parameters. This method minimizes manual edits and increases automation efficiency.
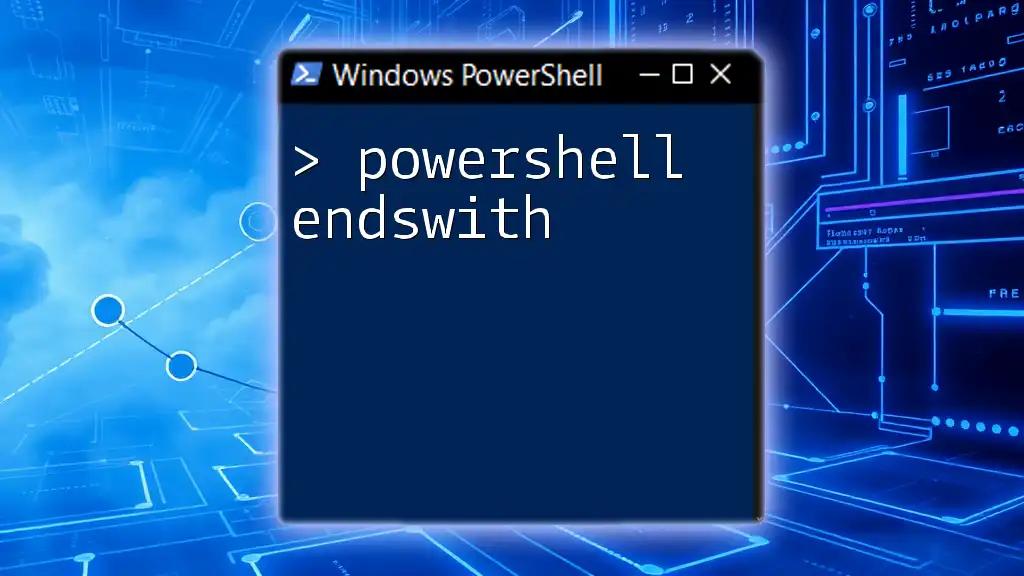
Troubleshooting Common Issues
Debugging XML with PowerShell
When working with XML elements and the `AppendChild` method, errors can occur. Learning to identify and debug these issues is crucial. Some common mistakes are:
- Attempting to append a node that has no parent.
- Creating nodes with invalid characters in their names.
Utilizing PowerShell's debugging tools can help you trace these issues and capitalize on PowerShell's built-in error reporting features to identify the root problem.
Performance Considerations
While `AppendChild` is powerful, performance can degrade with very large XML structures. If you encounter performance issues, consider strategies such as:
- Batch node creation to minimize repetitive method calls.
- Evaluating the structure of your XML to ensure it is as efficient as possible.
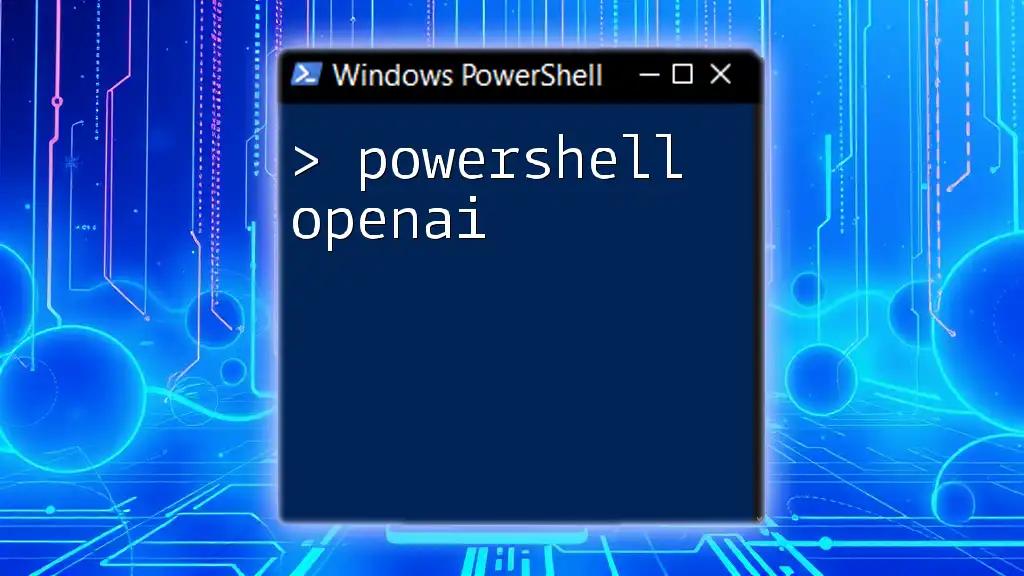
Conclusion
The `AppendChild` method in PowerShell is a powerful tool for managing XML structures. By understanding how to create XML documents, add child nodes, and work with attributes, you can effectively leverage this method to automate tasks and enhance data organization. With hands-on practice and exploration of the concepts discussed, you'll be well-prepared to implement `AppendChild` in your own PowerShell scripts.
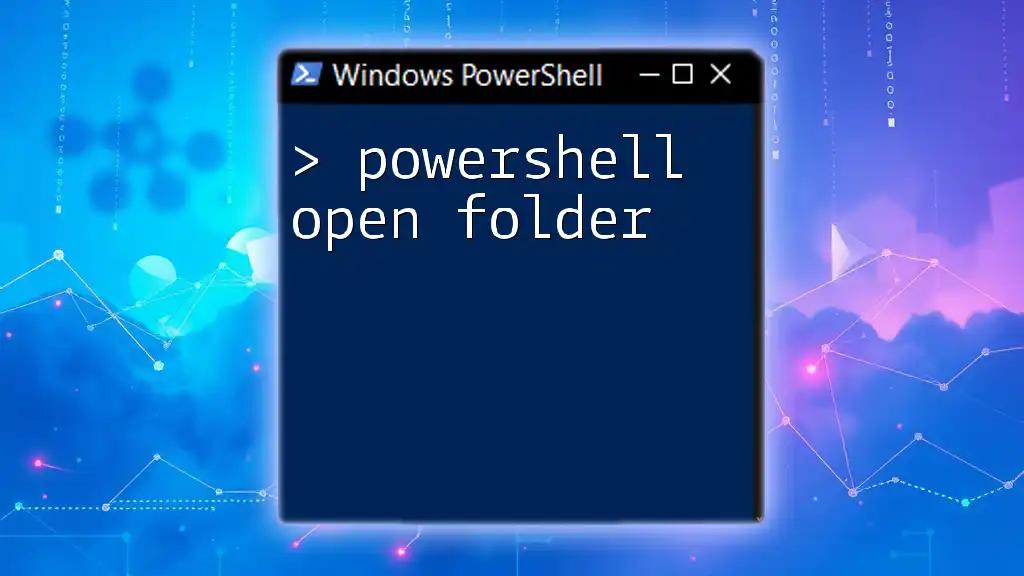
Additional Resources
For further exploration, consider diving into more advanced references on XML manipulation, DOM techniques in PowerShell, and other related scripting methods.

Call to Action
We invite you to share your questions or experiences regarding the `AppendChild` method. Let us know how you use PowerShell in your daily tasks or what topics you'd like to see covered next! Consider signing up for our newsletters or courses for more in-depth training in PowerShell scripting techniques.