The `Get-ChildItem` cmdlet in PowerShell retrieves the files and folders in a specified directory or the current directory if none is specified, allowing for easy exploration and management of file system items.
Here’s a simple code snippet to use `Get-ChildItem`:
Get-ChildItem -Path 'C:\Your\Directory\Path'
What is Get-ChildItem?
The `Get-ChildItem` cmdlet in PowerShell is essential for navigating the filesystem. It retrieves the items (files and directories) from a specified location, similar to what you might see while browsing through Windows Explorer. Understanding this command is vital for any PowerShell user who needs to manage files and folders effectively.
Comparison with other cmdlets
While `Get-ChildItem` serves to list items, other cmdlets like `Get-Item` retrieve a specific item, and `Remove-Item` allows you to delete items. Knowing how `Get-ChildItem` fits into the PowerShell environment is critical for efficient scripting and file management.
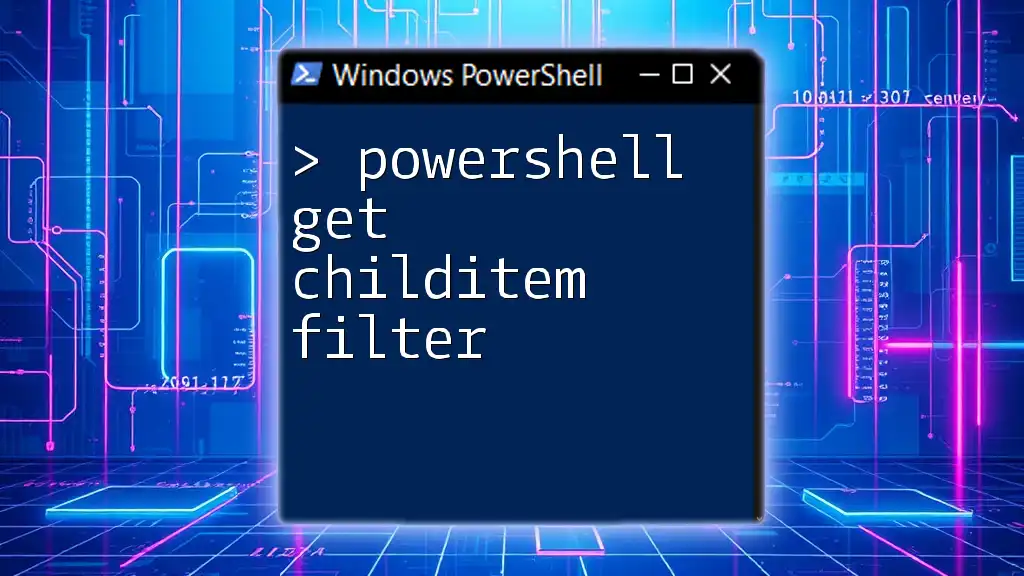
Basic Syntax of Get-ChildItem
The syntax of the `Get-ChildItem` cmdlet is straightforward and can be adapted based on needs:
Get-ChildItem -Path <path> -Options
In this structure, `-Path` specifies where you want to look, and `-Options` stands for various parameters that can be customized to filter results.
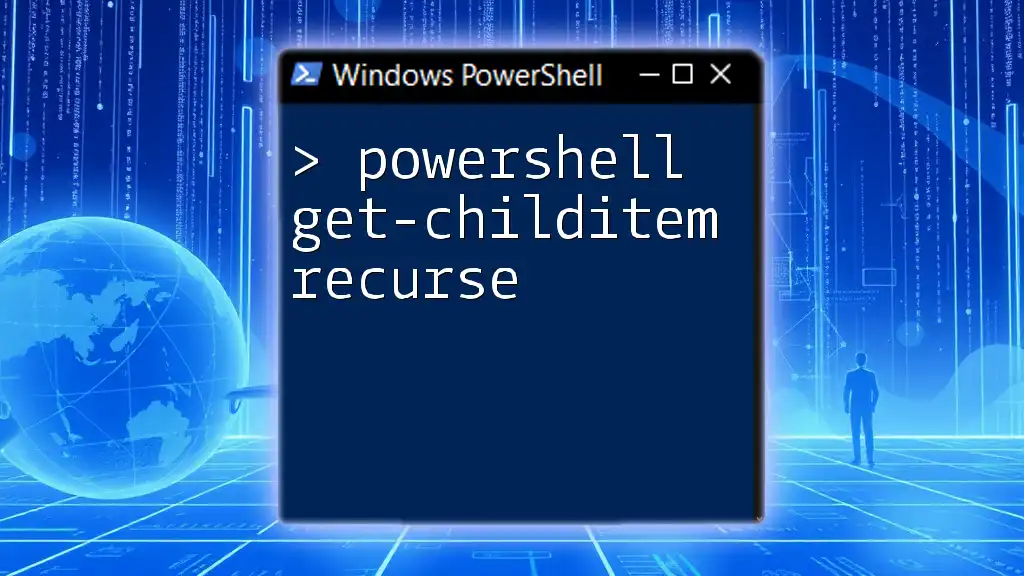
Common Parameters of Get-ChildItem
Path
The `-Path` parameter specifies the directory to search. You can use absolute or relative paths. For example:
Get-ChildItem -Path C:\Users
In this example, PowerShell outputs all files and folders within the `C:\Users` directory.
Filter
The `-Filter` parameter lets you define specific criteria to narrow down results. It supports wildcards to give you flexibility. For instance, to find all text files:
Get-ChildItem -Path C:\Documents -Filter *.txt
This command retrieves all `.txt` files within the `C:\Documents` folder.
Recurse
When you use the `-Recurse` parameter, PowerShell searches through all subdirectories as well, making it a powerful tool for deep dives into the filesystem. Here's an example:
Get-ChildItem -Path C:\ -Recurse
Although beneficial, be cautious, as this can produce extensive output and may take time depending on the directory's depth.
Force
Sometimes you need to access hidden files and folders, and the `-Force` parameter helps in that regard. It reveals items that are usually concealed. For example:
Get-ChildItem -Path C:\ -Force
This command lists all files, including hidden ones, in the `C:\` directory.
Include and Exclude
These parameters further refine your search, allowing you to specify which items to include or exclude based on file types. Here's how you can use them:
Get-ChildItem -Path C:\ -Include *.txt, *.docx -Exclude *.log
In this command, all `.txt` and `.docx` files are included, while any `.log` files are excluded from the results.
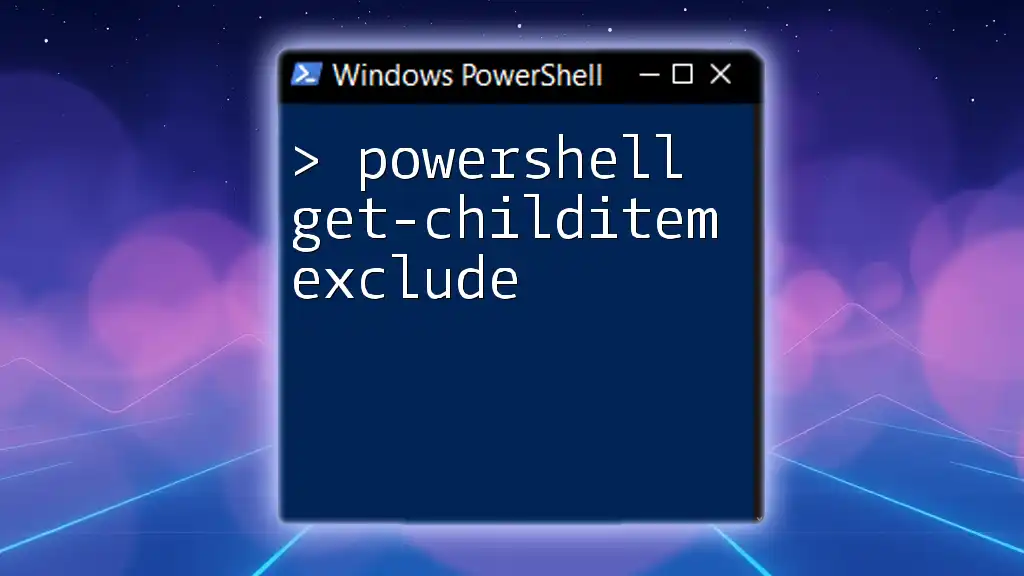
Practical Examples of Get-ChildItem
Listing Files in a Directory
Getting started is easy. To list files in a directory, you can use:
Get-ChildItem -Path C:\Reports
This command will provide you with a simple list of all files and directories under `C:\Reports`.
Searching for Specific File Types
In situations where you need certain file types, you can leverage the `-Filter` parameter effectively. For example:
Get-ChildItem -Path C:\Projects -Filter *.ps1
This retrieves all PowerShell scripts (`.ps1`) from the `C:\Projects` directory.
Retrieving Information About Files
If you want more than just names, you can filter the output with `Select-Object` to show properties such as file size and last modified date:
Get-ChildItem -Path C:\ -Recurse | Select-Object Name, Length, LastWriteTime
This command returns a detailed list that includes the name, size, and last modified timestamp for each file in the specified directory.
Using Get-ChildItem with Other Cmdlets
PowerShell scripting often involves chaining commands together. Using `Get-ChildItem` with pipes allows for refined outputs. For instance:
Get-ChildItem -Path C:\Logs | Where-Object { $_.Length -gt 1MB }
This command filters the output to only show log files larger than 1MB.
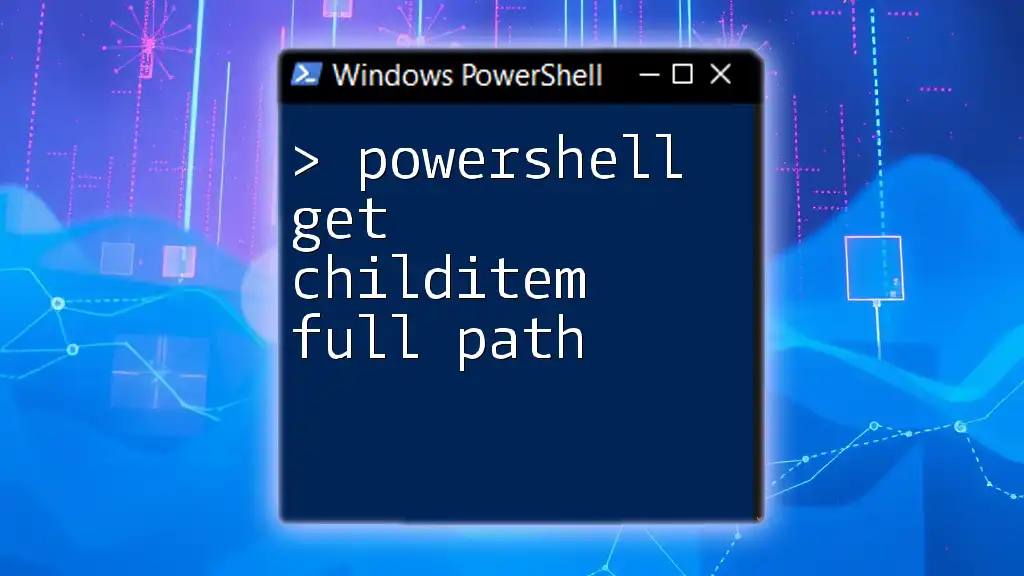
Handling Errors and Exceptions
Running scripts often invites unexpected errors. Familiarizing yourself with common mistakes can save time. For instance, specifying an invalid path may result in an error message. Implementing Try-Catch blocks can help manage these scenarios gracefully:
Try {
Get-ChildItem -Path Z:\InvalidPath
} Catch {
Write-Host "Error: $_"
}
This structure catches errors and provides clear feedback without halting the script.
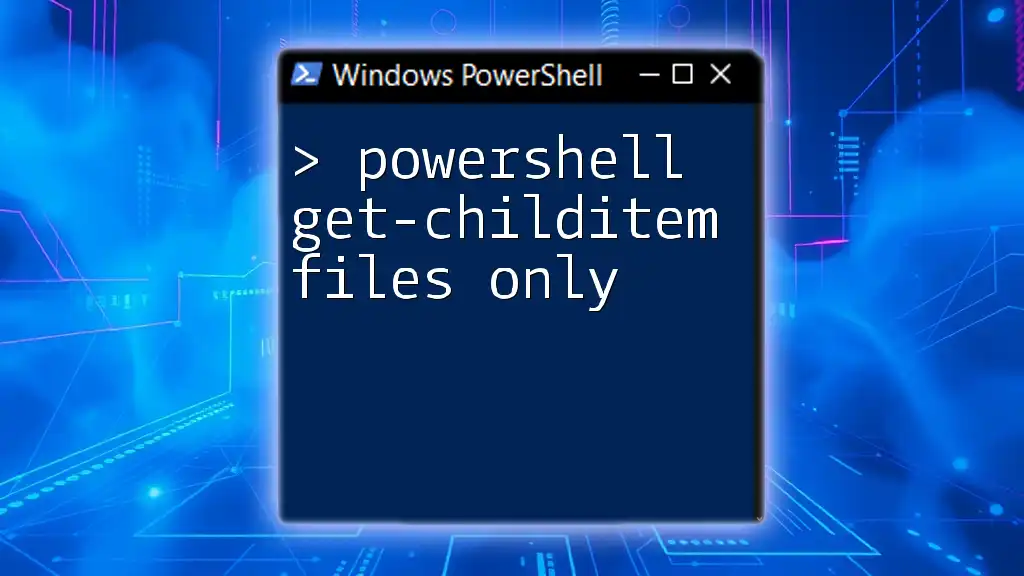
Best Practices for Using Get-ChildItem
Tips for maximizing efficiency
- Limit recursion: If deep searches aren't necessary, avoid using `-Recurse`, which can slow down execution.
- Pair with filters: Using `-Filter` and `-Include` or `-Exclude` will help narrow your focus, returning results faster.
Avoiding performance issues
Too broad a search can lead to performance degradation. Always specify the path as precisely as possible to maintain efficiency.
Using Get-ChildItem in scripts effectively
Integrate `Get-ChildItem` into larger automation scripts carefully, ensuring that subsequent cmdlets can process the output as intended.
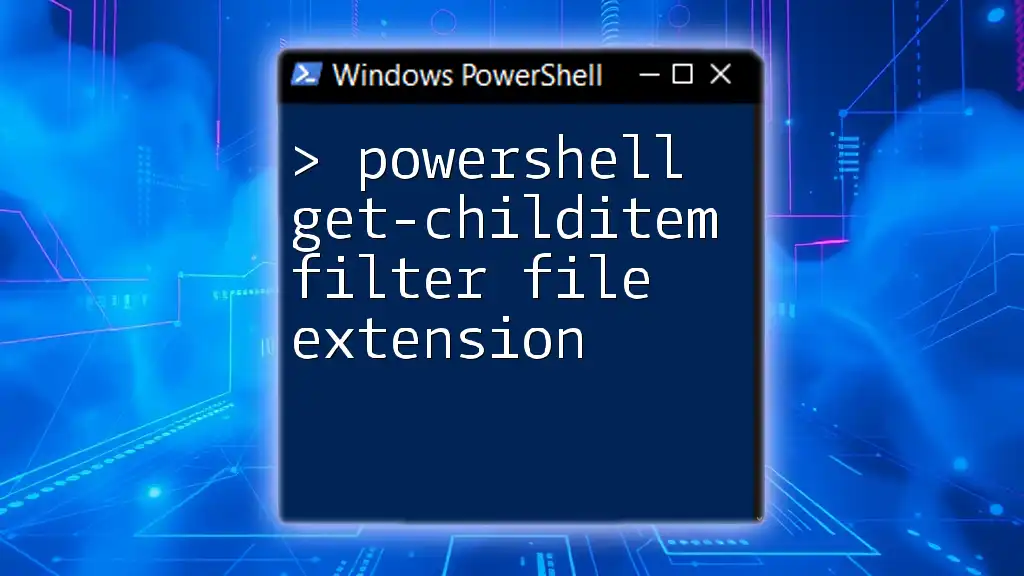
Conclusion
By now, you should understand how to utilize the `Get-ChildItem` cmdlet effectively for managing directories and files in PowerShell. Experimenting with different parameters will deepen your understanding and refine your scripting skills. As you continue learning, remember to incorporate `Get-ChildItem` into your toolkit for a robust file management strategy.
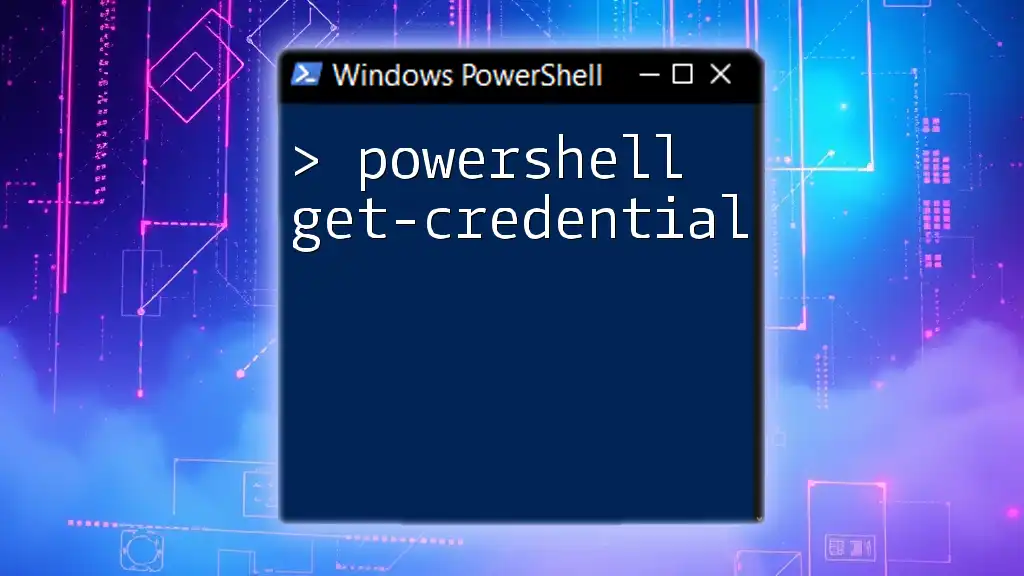
Additional Resources
For those eager to learn more, consider checking the official Microsoft documentation on PowerShell, exploring recommended books, or engaging with community forums for deeper insights into PowerShell usage.