In PowerShell, you can retrieve the current timestamp using the `Get-Date` cmdlet, which provides the date and time in a variety of formats.
Here's a code snippet to get the current timestamp:
Get-Date
Understanding Timestamps in PowerShell
A timestamp is a data representation that tracks when a particular event occurred, often specifying the date and time down to the second. In the realm of scripting and automation, timestamps are crucial for logging events, monitoring changes, and scheduling tasks. They allow users to keep a chronological record of activities, making it easier to troubleshoot issues and trace back steps if necessary.
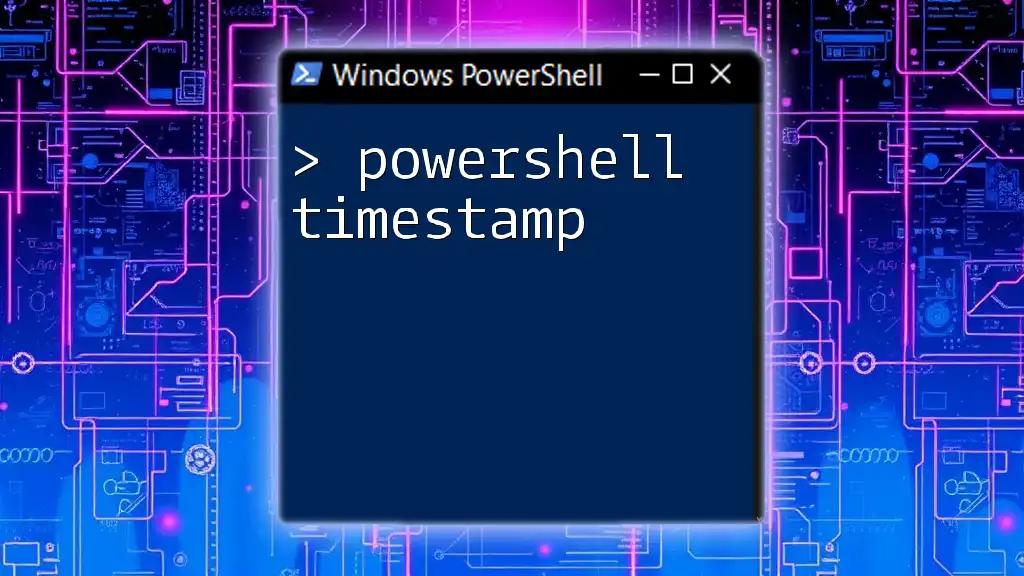
Using Get-Date Cmdlet
Introduction to Get-Date
The `Get-Date` cmdlet in PowerShell is the primary tool for retrieving the current date and time, along with custom formatting options. It efficiently generates timestamps that you can manipulate based on your specific requirements.
Retrieving Current Timestamp
To get the current timestamp, you can simply use the following command:
Get-Date
When you execute this command, you’ll receive an output that reflects the current date and time. This information is not only useful for scripting but also comes in handy for logging events, presenting data, or analyzing time-sensitive information.
Formatting Timestamps
Default Formatting
By default, `Get-Date` returns the date and time in a standard format. Nevertheless, you can customize how you see the output using the `-Format` parameter.
Get-Date -Format "G"
This command yields the current date and time in a general date/time pattern, which is both human-readable and straightforward.
Custom Formatting Options
PowerShell offers a range of format specifiers, allowing for precise control over how timestamps appear. Here are a few essential formats:
- ISO 8601 format: This standard format is often used for data interchange and logging.
Get-Date -Format "yyyy-MM-ddTHH:mm:ss"
- Short date format: Ideal for displaying dates concisely.
Get-Date -Format "d"
- Long date format: Displays a more detailed representation of the date.
Get-Date -Format "D"
These formatting options are particularly useful when you need timestamps to be in specific formats for further processing or for display in reports.
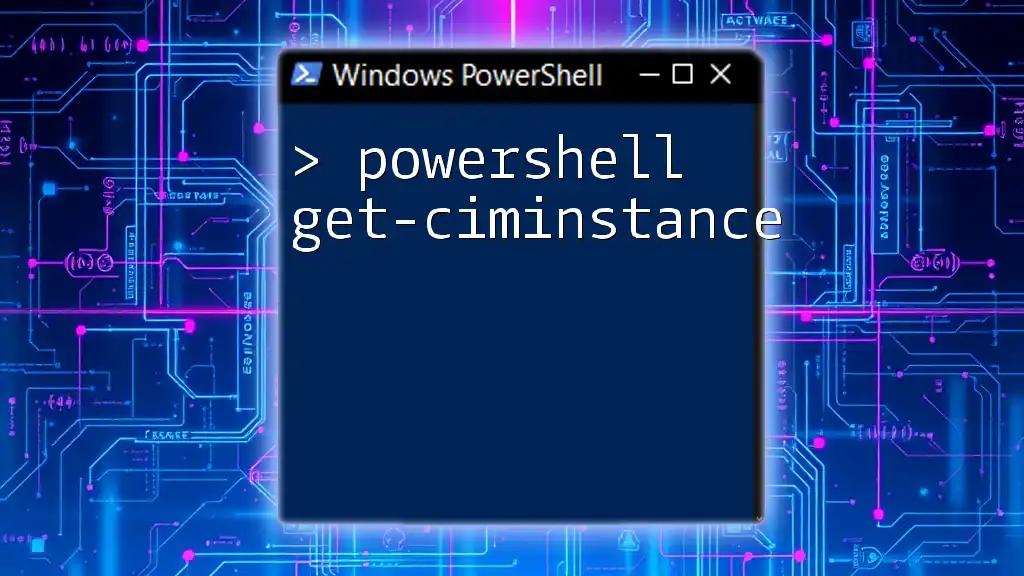
Getting Future and Past Timestamps
In many scenarios, you may need to work with timestamps that aren't just the current moment, but rather a point in the future or the past.
Retrieving a Future Timestamp
You can easily create future timestamps by employing either the `AddDays()` or `AddMonths()` methods. For instance, to get the timestamp for one week from today:
Get-Date -Date (Get-Date).AddDays(7)
This command reliably calculates a date seven days into the future, which can be handy for scheduling tasks or reminders.
Retrieving a Past Timestamp
Conversely, if you need a timestamp from the past, you can simply call:
Get-Date -Date (Get-Date).AddMonths(-1)
This command gives you the date that was one month prior to the current date. Such functionality may serve you well in analyzing patterns, historical data, or version comparisons.

Using Timestamps in PowerShell Scripts
Logging Events with Timestamps
Timestamps play a fundamental role in logging events within your scripts. Including the date and time in logs enhances their utility, making it easier to trace operations. Here’s how you could implement logging with accurate timestamps:
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"[$timestamp] Event occurred" | Out-File -Append -FilePath "log.txt"
In the example above, every time the logging script runs, it appends a new line to `log.txt` with the current timestamp, providing a clear and chronological data trail.
Timestamps in File Operations
Another common usage of timestamps is in manipulating file properties. You may want to set the creation date of a file to a specific timestamp. Here’s how you can do this:
$timestamp = Get-Date "2023-10-01"
$filename = "example.txt"
$(New-Item -ItemType File -Name $filename)
(Get-Item $filename).CreationTime = $timestamp
This script creates a new file named `example.txt` and sets its creation time to October 1st, 2023. Such operations may prove valuable for data integrity or historical tracking.
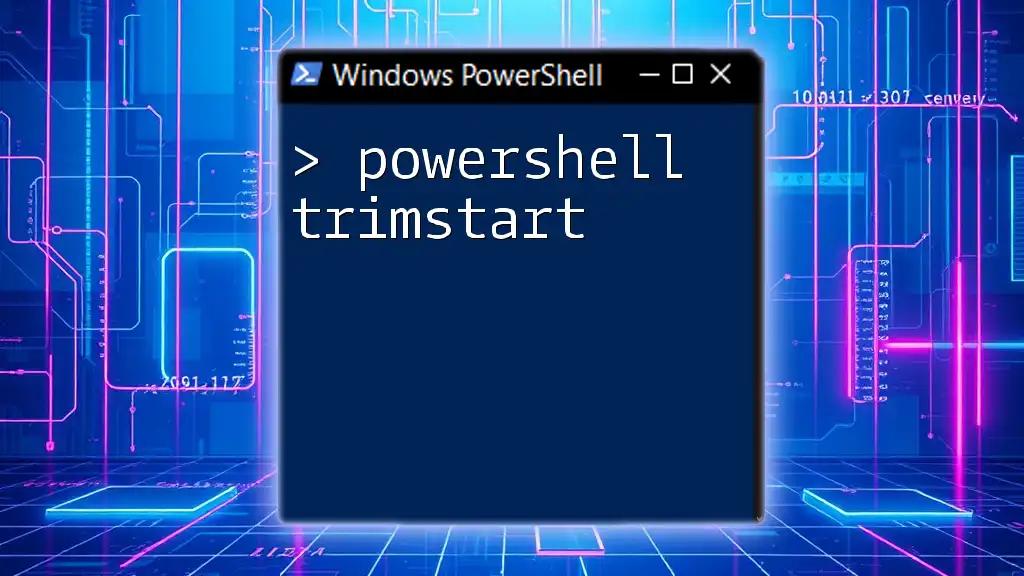
Modifying Timestamp Properties
Changing File Timestamps
Beyond creating timestamps, you may also find yourself needing to modify the timestamp properties of existing files. Manipulating file properties like the last modified time can be essential for file management and version control.
To update a file's `LastWriteTime`, you can use the following command:
(Get-Item "example.txt").LastWriteTime = Get-Date
This command updates the last modified timestamp of `example.txt` to the current date and time, ensuring your file reflects the latest changes.
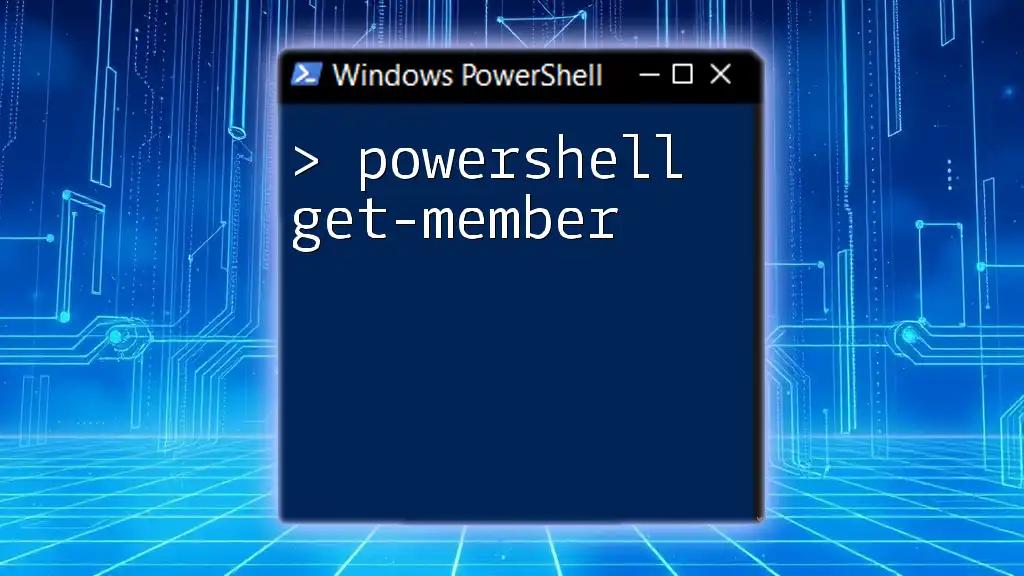
Conclusion
In the world of PowerShell automation and scripting, managing timestamps is indispensable. From retrieving and formatting the current date and time with `Get-Date` to manipulating timestamps for logging and file operations, mastering timestamp handling elevates the functionality of your scripts significantly. We encourage you to practice using these commands to become proficient in managing timestamps with PowerShell.
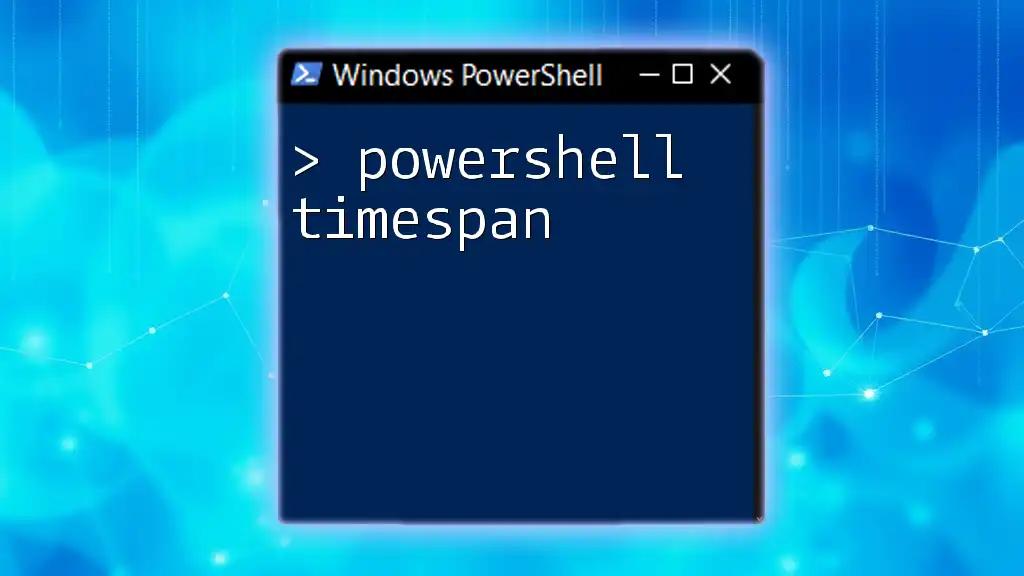
Additional Resources
For more information on PowerShell commands and usage, consider visiting the official PowerShell documentation, where you can find detailed explanations and best practices for advanced functionality. Happy scripting!