In PowerShell, you can set the system time using the `Set-Date` cmdlet followed by the desired date and time in quotes.
Set-Date -Date "2023-10-10 14:30:00"
Understanding the System Time
What is System Time?
System time refers to the current time a computer records based on its internal clock. It is crucial for various processes within an operating system, affecting everything from file timestamping to scheduling tasks and logging events. Setting the correct system time ensures accurate operation of applications and prevents potential issues with time-sensitive operations.
Why Set Time in PowerShell?
Using PowerShell to manage system time offers several advantages:
- Automation: You can easily automate time adjustments through scripts, reducing the chances of human error.
- Integration with Other Tasks: Setting the time as part of larger scripts allows for better synchronization of processes.
- Ease of Use: PowerShell provides a simple interface to execute commands frequently and adjust settings without navigating through GUI options.

Common Scenarios for Setting Time with PowerShell
Aligning Time with Network Time Protocol (NTP)
Network Time Protocol (NTP) allows computers to synchronize their clocks with a reliable time source, ensuring accurate time across a network. Misalignment can lead to problems like failed scheduled tasks or inconsistent logging. Setting up NTP is crucial for environments where timing matters.
Adjusting Time for Scheduled Tasks
In many cases, tasks scheduled to run at specific times may fail if the system clock is set incorrectly. Using PowerShell to adjust the time ensures that these automated processes run as intended, avoiding discrepancies in execution time.

Preparing Your Environment
Ensuring PowerShell is Installed
Before working with PowerShell commands, verify that PowerShell is installed on your system. Most modern Windows versions have it pre-installed, but older operating systems might require installation.
Required Permissions
Setting the system time typically requires administrator permissions. To run PowerShell with these elevated privileges, right-click the Start menu and select "Windows PowerShell (Admin)". This ensures that your commands execute without permissions-related errors.
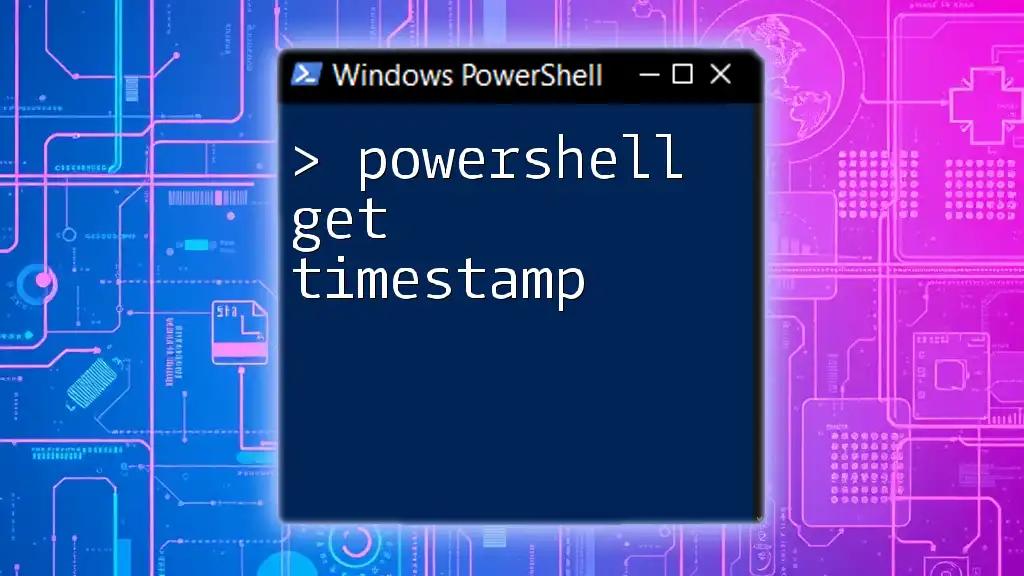
How to Set Time in PowerShell
Using Set-Date Cmdlet
The `Set-Date` cmdlet is the primary tool for changing the system date and time in PowerShell. It allows for versatile adjustments and can use a simple date string for setting.
Syntax of Set-Date
The syntax for the `Set-Date` cmdlet is straightforward:
Set-Date -Date <DateTime>
Basic Example of Setting Time
To set the system time to a specific date and time, you can use the following command:
Set-Date -Date "09/30/2023 13:45:00"
This command will set the system time to 1:45 PM on September 30, 2023. Upon execution, check the current time using `Get-Date` to confirm the change.
Setting Time with Specific Parameters
Setting Time Zone
It's just as important to ensure your time zone is correct. Using the incorrect time zone can lead to miscalculations in time-sensitive tasks.
To set the time zone, you can use the `tzutil.exe` command-line tool alongside PowerShell:
tzutil.exe /s "Pacific Standard Time"
This sets your time zone to Pacific Standard Time. To confirm the change, you can check the time zone with:
Get-TimeZone
Combining Date and Time Adjustments
You can also set the date and make adjustments to the current time simultaneously. For example, if you want to set the time one hour in the future, you can run:
Set-Date -Date (Get-Date).AddHours(1)
This command takes the current date and time, then adds one hour to it before setting the system clock. It’s a flexible way to ensure your computer aligns with required time parameters instinctively.

Verifying the Time Set in PowerShell
Displaying Current Time
After making adjustments, it’s important to confirm that the time has been set correctly. You can use the `Get-Date` cmdlet for this:
Get-Date
This command will display the current system date and time, allowing you to verify the accuracy of your previous commands.
Checking Time Zone Settings
To ensure the time zone is correctly set, you can use:
Get-TimeZone
This command provides the current system's time zone details, confirming if you have adjusted it appropriately.
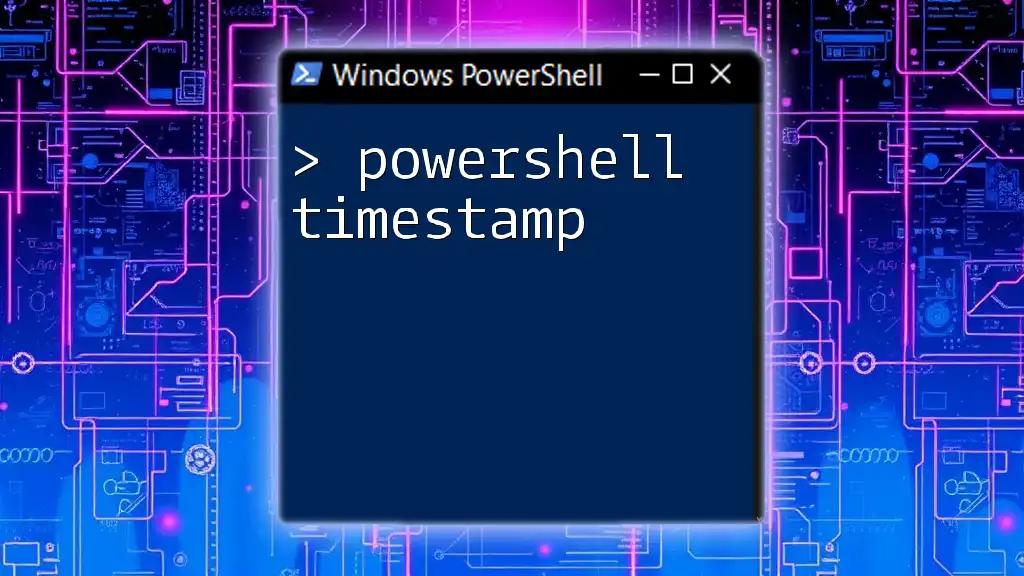
Troubleshooting Common Issues
Permissions Issues
If you encounter permission-related error messages such as "Access Denied" when trying to set the time, it often indicates you need elevated privileges. Always ensure that you are running PowerShell as an administrator to avoid these issues.
Syntax Errors
Common syntax errors may arise, such as incorrect date formatting or typos in cmdlets. Pay close attention to the syntax and use error messages to troubleshoot the issues. Familiarize yourself with the format needed to ensure your commands execute properly.
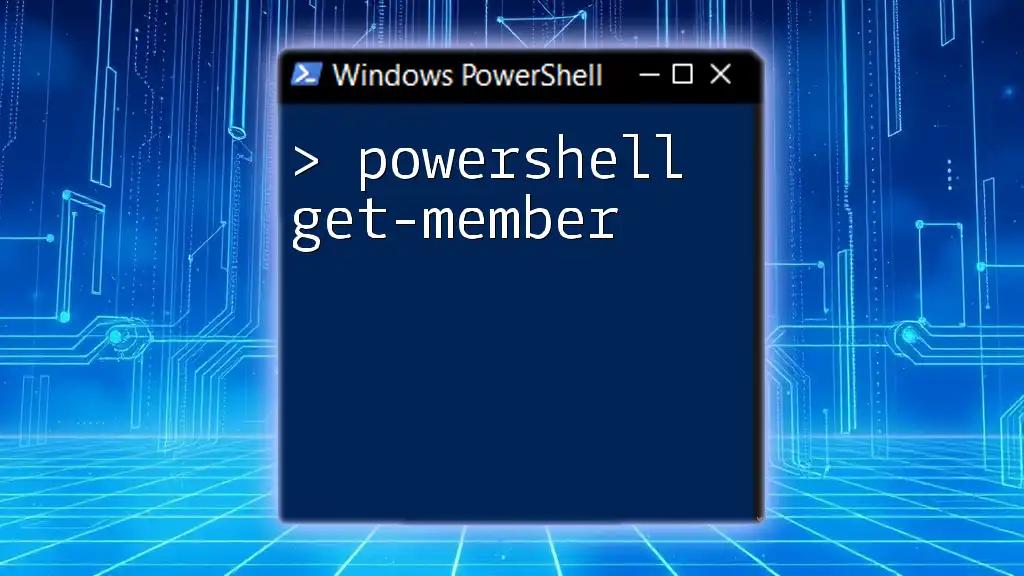
Best Practices for Setting Time with PowerShell
Automating Time Synchronization
For those managing multiple systems, consider automating time synchronization. Scheduling a PowerShell script that periodically checks and adjusts the time ensures that each system remains synchronized, minimizing the risk of discrepancies.
Logging Changes
It's important to keep a log of time changes for security and audit purposes. A simple way to log changes in PowerShell is to output changes to a text file:
Set-Date -Date "09/30/2023 13:45:00" | Out-File -Append -FilePath "C:\Logs\TimeChangeLog.txt"
This command appends the time change along with a timestamp, maintaining a record for future reference.

Conclusion
In summary, using PowerShell to manage system time is not only powerful but essential for ensuring accurate operations. It simplifies the process of setting and verifying time, crucial for system integrity and scheduled operations. By becoming familiar with the various commands and best practices highlighted here, you can leverage PowerShell for effective time management, enhancing your systems' performance and reliability.