In PowerShell, setting variables allows you to store and manipulate data, enabling easier script writing and efficient data handling.
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding Variables in PowerShell
What are Variables?
In programming and scripting, variables are placeholders that hold data values. They enable developers to store and manipulate data dynamically throughout their scripts. In PowerShell, like many other programming languages, variables allow you to create more versatile and reusable scripts, making your automation tasks easier and more efficient.
PowerShell Variable Naming Conventions
When working with PowerShell, it's essential to adhere to naming conventions for your variables. Here are some important rules:
- Variable names must start with a dollar sign ($).
- The name can include letters, numbers, and underscores, but it cannot start with a number.
- Use case sensitivity to differentiate between variables, though PowerShell itself is not case-sensitive.
Valid examples: `$myVariable`, `$variable1`, `$my_variable` Invalid examples: `$1variable`, `$my-variable`
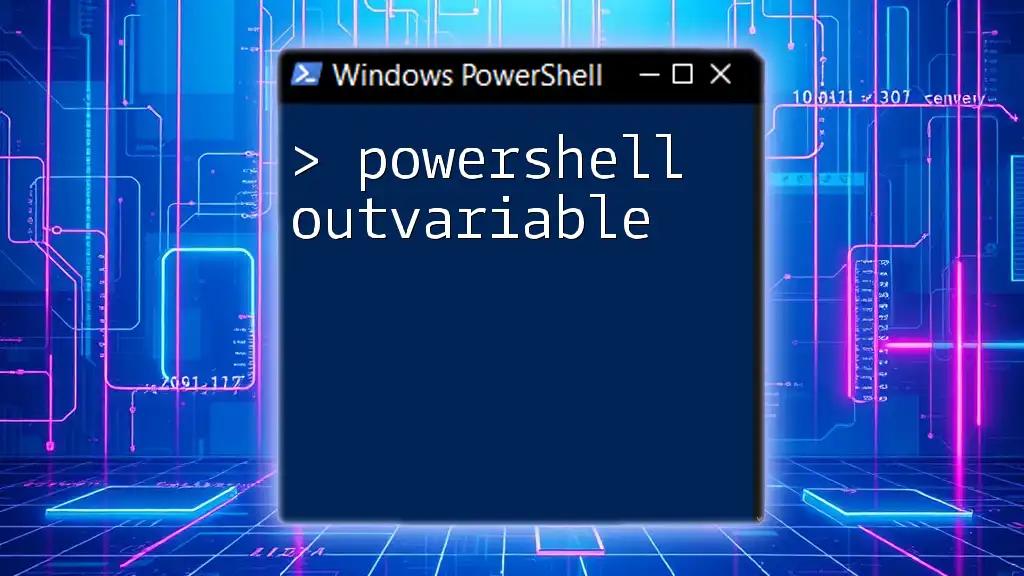
How to Assign Variables in PowerShell
Assigning a Variable in PowerShell
To assign a variable in PowerShell, use the equal sign (=) operator. This operator sets the variable on the left to the value on the right. The basic syntax looks like this:
$myVariable = "Hello, World!"
In this example, we created a variable named `$myVariable` and assigned it the string value "Hello, World!". This string can now be manipulated or accessed throughout the rest of your script.
Types of Variables
Scalar Variables
Scalar variables hold a single value. In PowerShell, you typically assign a scalar variable as follows:
$scalarVariable = 42
Here, `$scalarVariable` holds the integer 42.
Array Variables
An array variable can hold multiple values. You create an array by enclosing the values in parentheses and separating them with commas. Here’s an example:
$arrayVariable = @(1, 2, 3, 4, 5)
The variable `$arrayVariable` now contains the numbers 1, 2, 3, 4, and 5. You can access individual items using their index, starting from 0.
HashTable Variables
A hashtable variable (or dictionary) consists of key-value pairs, allowing you to store data in a structured format. You can declare a hashtable like this:
$hashTableVariable = @{"Key1" = "Value1"; "Key2" = "Value2"}
In this case, `$hashTableVariable` contains two keys, Key1 and Key2, with their corresponding values. Hashtables are particularly useful for organizing related information.
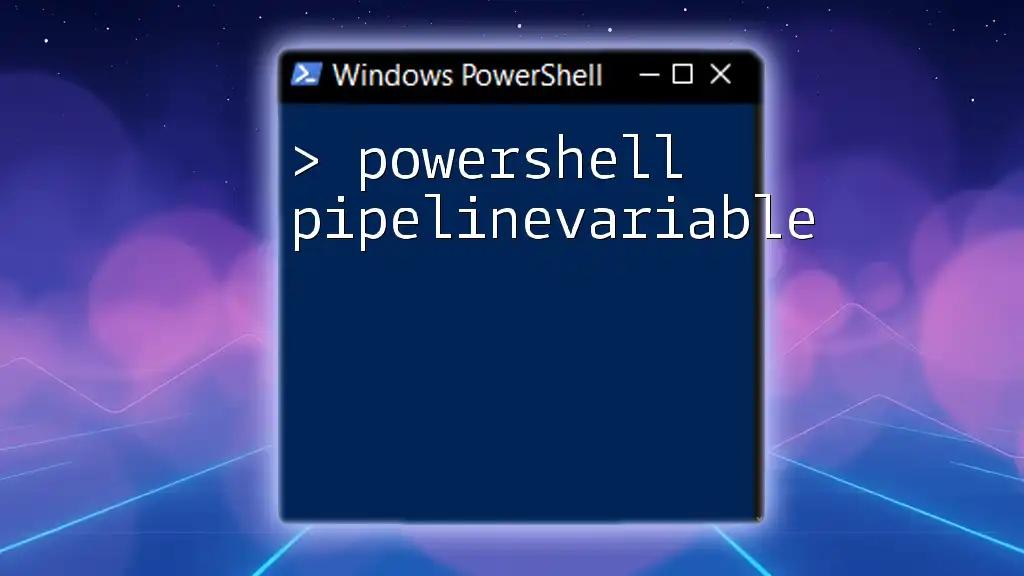
Retrieving Variable Values
Accessing and Displaying Variable Values
To access the value stored in a variable, simply refer to it by name. For instance:
Write-Output $myVariable
This command will output "Hello, World!" to the console. The Write-Output cmdlet is commonly used to display variable values.
Reassigning Variable Values
You can easily change the value assigned to a variable. For example:
$myVariable = "New Value"
After executing this line, `$myVariable` now holds "New Value" instead of the original string. This ability to reassign values is crucial for dynamic scripting.
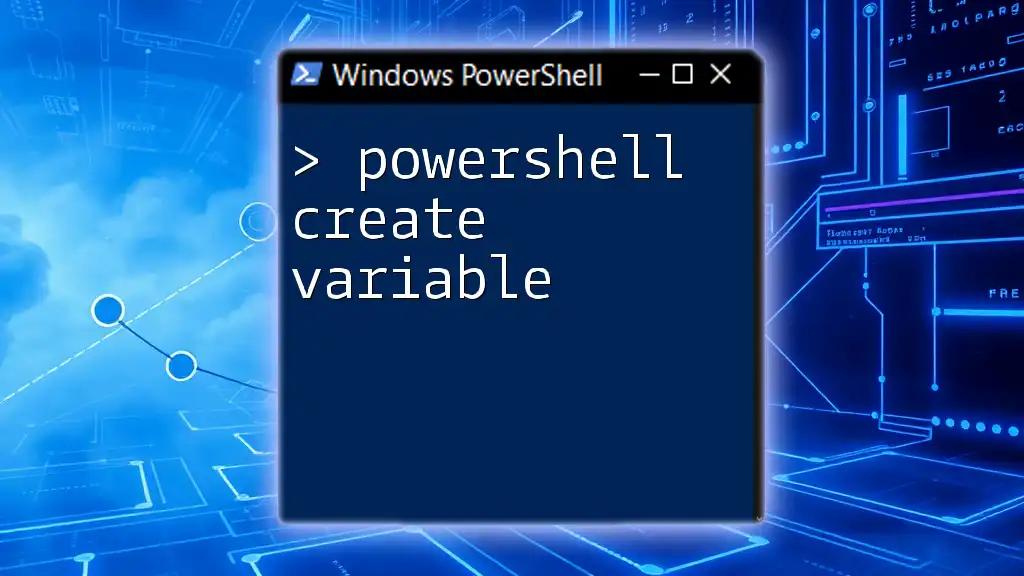
Best Practices for Using Variables in PowerShell
Scope of Variables
Understanding variable scope is critical in PowerShell. Variables can exist in different scopes: Global, Local, and Script.
- Global: Available throughout the entire session.
- Local: Available only within the function or block where it’s declared.
- Script: Accessible only within the script file.
Using appropriate scopes helps prevent naming conflicts and makes your scripts more maintainable.
Variable Types and Memory Management
Different variable types in PowerShell consume varying amounts of memory. Scalar variables typically use less memory than arrays or hashtables. When writing scripts, consider using the most efficient variable type for your data needs, as this can improve performance and reduce resource usage.
Naming Best Practices
Choose meaningful names for your variables to enhance readability. Avoid using generic names like `$a` or `$temp`; instead, use descriptive names like `$userName` or `$filePath`. This practice encourages maintainability, especially for larger scripts.
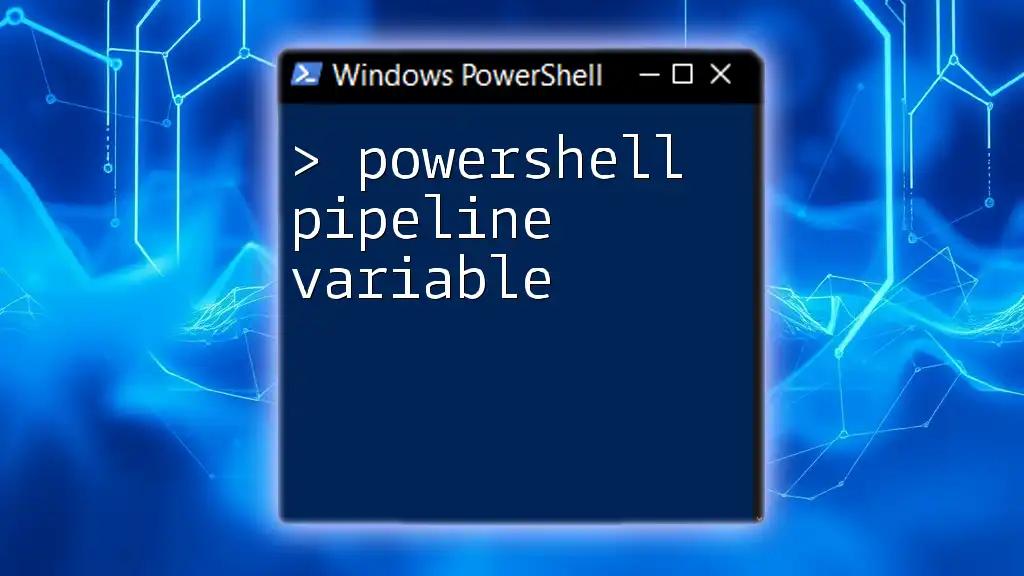
Troubleshooting Common Variable Issues
Common Mistakes
While working with PowerShell variables, you may encounter issues such as:
- Using an undeclared variable (which causes an error).
- Forgetting to use the $ sign when referencing a variable.
- Mismanaging scopes, resulting in variable values being inaccessible.
Debugging Variable Issues
Debugging variable-related issues can be done by:
- Using the Get-Variable cmdlet to inspect variables.
- Printing variable values at different points in your script to trace changes.
- Employing error handling to manage unexpected outcomes.

Advanced Variable Options
Working with Dynamic Variables
PowerShell allows you to create dynamic variables where the name itself can be set during script execution. Here’s how you do it:
$dynamicVarName = "dynamicVariable"
New-Variable -Name $dynamicVarName -Value "Dynamic Value"
This code creates a new variable named dynamicVariable that holds the value "Dynamic Value". This capability is useful for creating variables based on external inputs or configurations dynamically.
Using Variables in Functions
Variables can also be passed as parameters to functions, enabling code reuse. Here’s an example function that uses a variable:
function Get-Greeting {
param($name)
return "Hello, $name"
}
When you call `Get-Greeting "Alice"`, it will return "Hello, Alice". This feature makes your scripts modular and easier to manage.
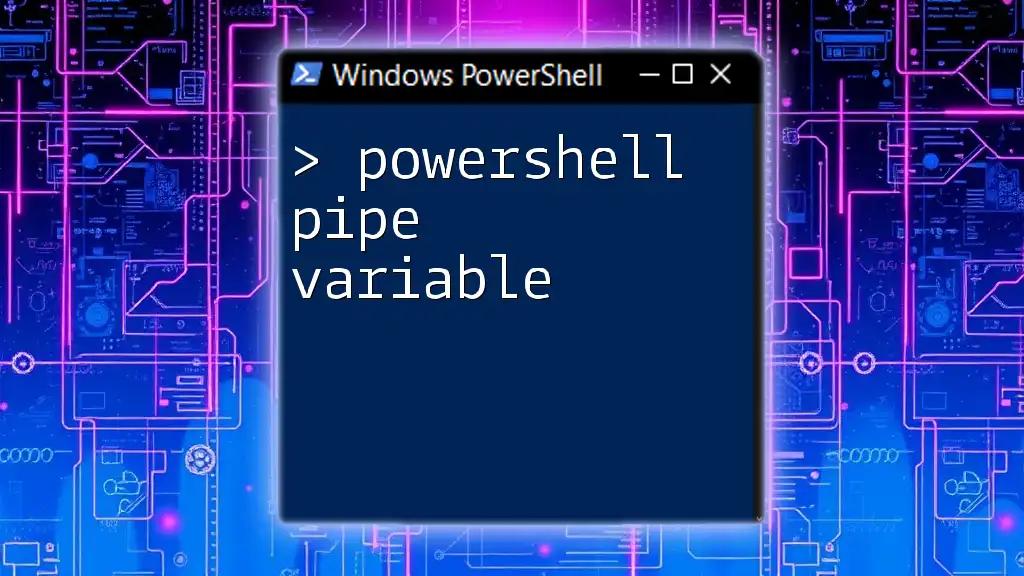
Conclusion
In this guide, we've explored the fundamentals of PowerShell setting variables, including how to assign, retrieve, and manage variables effectively. By following best practices and understanding the various variable types and scopes, you will be well-equipped to write cleaner, more efficient PowerShell scripts. Practice these concepts in your own scripts to build your confidence and proficiency in PowerShell.