In PowerShell, you can increment a variable by using the `+=` operator, which adds a specified value to the current value of the variable. Here's a simple example:
$counter = 1
$counter += 1
Write-Host $counter # Output: 2
Introduction to PowerShell Variables
Understanding PowerShell variables is essential for anyone looking to enhance their scripting capabilities. Variables serve as storage locations for data, and they allow you to manipulate that data throughout your script, from numbers and strings to object types.
What is a Variable?
In programming, a variable acts as a named storage container for data. In PowerShell, variables are prefixed with a dollar sign (`$`), and they can hold various kinds of types, including strings, integers, arrays, and more. For instance, you might have:
$greeting = "Hello, World!"
Why Use Variables in PowerShell?
Using variables streamlines your code and enhances automation. Variables allow you to:
- Store intermediate results that can be referred back to later.
- Write cleaner, more maintainable scripts by avoiding redundancy.
- Modify configurations easily by changing values in one place.
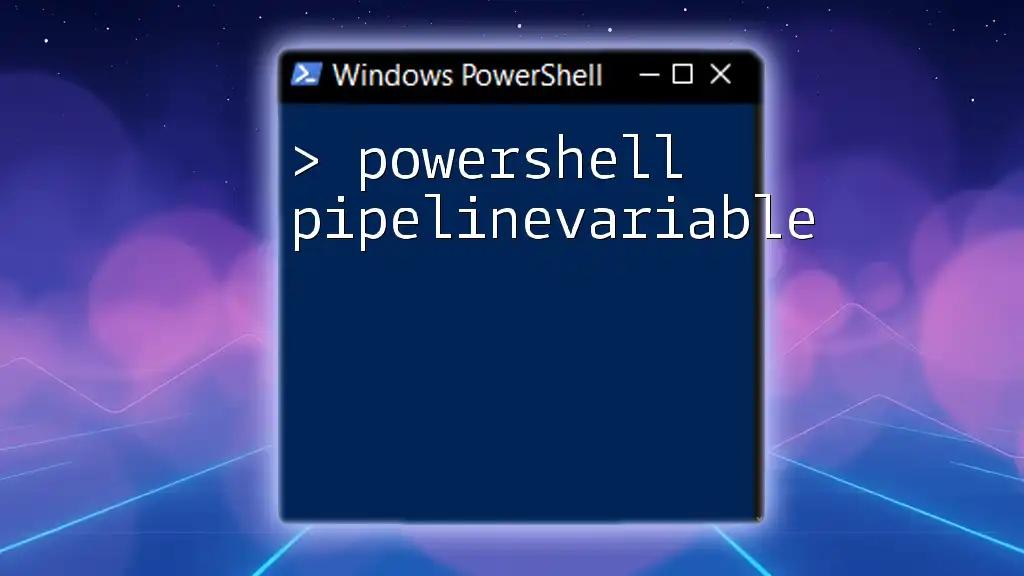
The Mechanics of Variable Incrementing
To effectively manage and manipulate data in scripts, understanding how to increment variables is crucial.
What Does Incrementing a Variable Mean?
To increment a variable means to increase its value by a specific amount, typically by one. This is particularly useful for creating counters—for instance, keeping track of iterations in a loop or counting occurrences in a dataset.
Basic Syntax of PowerShell Variable Incrementing
Incrementing a variable in PowerShell can be done with various operators, one of which is the increment operator (`++`). The syntax for this is simple and intuitive. Here’s a quick demonstration:
$counter = 0
$counter++
Write-Output $counter # Output: 1
In this example, `$counter` starts at zero and increments by one when the `++` operator is applied.
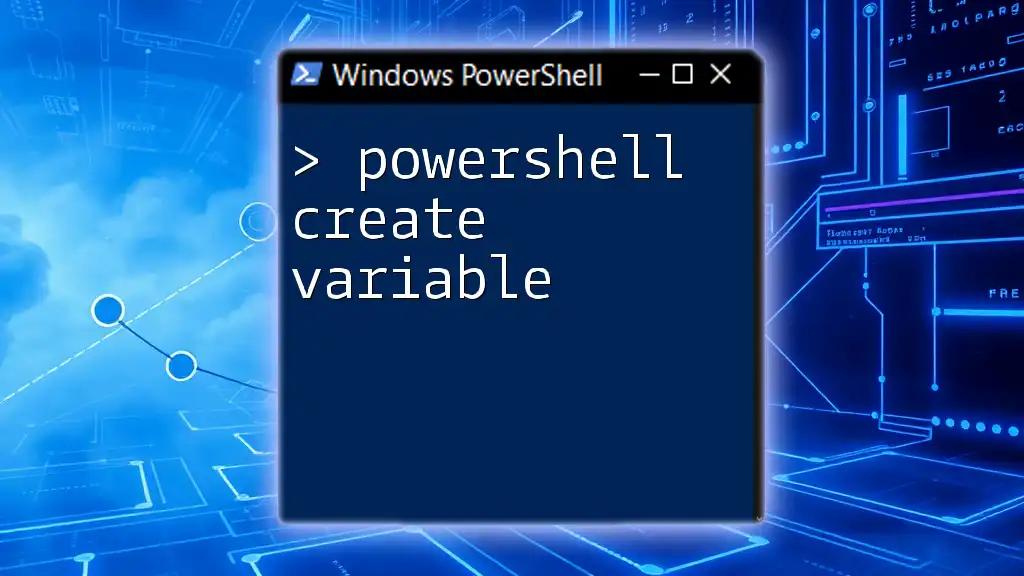
Methods to Increment a Variable
PowerShell offers several ways to increment a variable effectively, each suited to different scenarios in your scripts.
Using the Increment Operator
The increment operator (`++`) is one of the most common methods to increment counter-style variables. It’s straightforward and intuitive. Here is how it works:
$counter = 0
$counter++
Write-Output "Counter: $counter" # Counter: 1
In this code, `$counter` begins at `0`, and using `$counter++` increases its value to `1`.
Incrementing with Addition Assignment
Another method is to use the addition assignment operator (`+=`). This allows you to specify the amount by which to increment the variable.
$counter = 5
$counter += 2
Write-Output "Counter: $counter" # Counter: 7
Here, we start with `$counter` at `5` and then add `2`, resulting in `7`.
Incrementing in Loops
Incrementing variables within loops is a common practice to perform repeated actions. Here’s an example using a `for` loop where the variable increments automatically based on the loop's structure:
for ($i = 0; $i -lt 5; $i++) {
Write-Output "Current value of i: $i"
}
In this loop, `$i` counts from `0` to `4`, incrementing with each iteration.
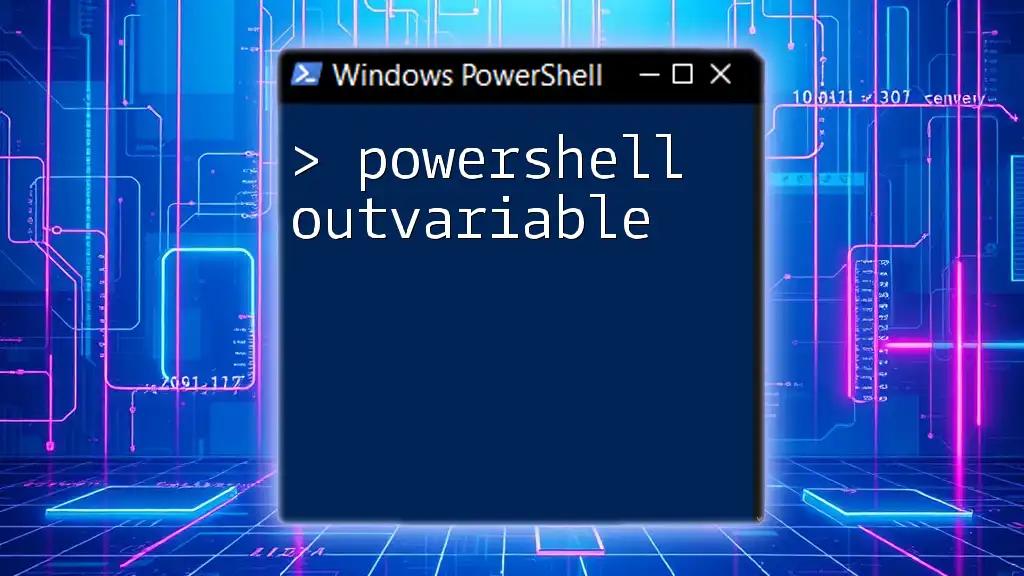
PowerShell Increment Counter Examples
Practical examples highlight the versatility and utility of incrementing variables in PowerShell.
Simple Counter Example
Consider a scenario where you want to count items in a collection. A straightforward implementation might look like this:
$counter = 0
foreach ($item in 1..5) {
$counter++
}
Write-Output "Total items counted: $counter" # Total items counted: 5
In this case, the `foreach` loop iterates through the range of numbers `1` to `5`, incrementing the `$counter` with each iteration.
Advanced Counter Increment with Conditions
For scenarios requiring more control over what gets counted, implementing conditions can be useful. Here’s an example where the counter increments only for even numbers:
$counter = 0
for ($i = 1; $i -le 10; $i++) {
if ($i % 2 -eq 0) {
$counter++
}
}
Write-Output "Even numbers count: $counter" # Even numbers count: 5
In this structure, the `$counter` only increments when `$i` is even, demonstrating conditional incrementing.
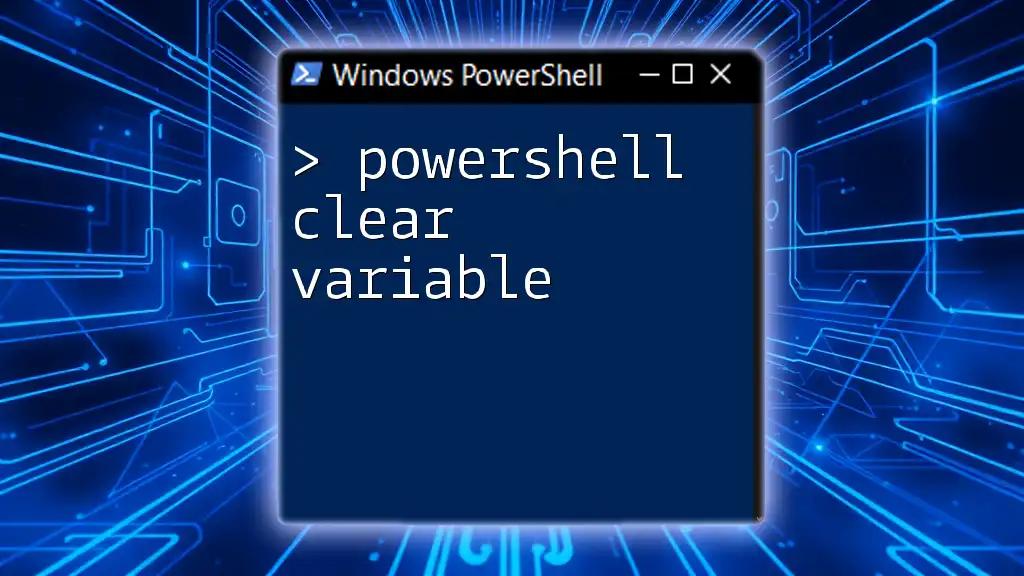
Common Pitfalls When Incrementing Variables
While incrementing variables is straightforward, there are common mistakes that can lead to unexpected results.
Forgetting to Initialize the Counter
Always ensure that your counter is initialized before incrementing it. Failing to do so may produce errors or unexpected behavior because PowerShell will interpret the variable as `null`.
Incrementing in the Wrong Scope
Understanding variable scope is crucial. Incrementing a variable outside of its defined scope can lead to logical errors in your script.
Using Wrong Operator Syntax
Incorrect operator usage can lead to confusion. Ensure you’re using `++` for incrementing rather than `+`, which merely performs addition but does not assign the incremented value back to the variable.
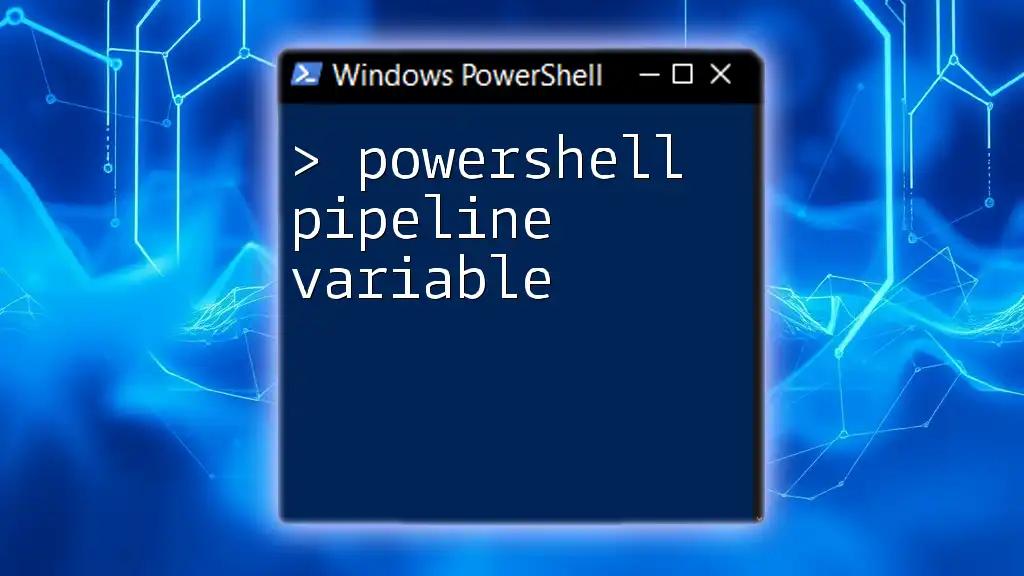
Best Practices for Working with Increment Variables
For effective PowerShell scripting involving increments, consider these best practices:
Keep Your Code Clean
Strive for clarity. Use meaningful variable names that convey their purpose, making your scripts easier to read and maintain.
Commenting Your Code
Comments can be invaluable. Document your logic, especially when using increments, to clarify your intentions. For example:
# Incrementing counter for successful login attempts
$loginCounter++
Testing and Validation
Don’t forget to test your scripts. Thorough testing helps ensure that all increments are working as intended, reducing the risk of errors during execution.

Conclusion
Understanding how to increment variables in PowerShell is fundamental to effective scripting. Mastering methods such as using the increment operator (`++`), addition assignment (`+=`), and applying these concepts in loops gives you a robust toolkit for automation and data manipulation.
To effectively apply these techniques, practice incrementing variables in your scripts, incorporate various methods, and pay attention to common pitfalls. The more you practice, the more intuitive variable manipulation will become.
For more practical tips and tutorials on PowerShell, don’t miss out on additional resources available on our website!