In PowerShell, you can create a date variable by using the `Get-Date` cmdlet, which retrieves the current date and time, as shown in the following example:
$dateVariable = Get-Date
Write-Host "The current date and time is: $dateVariable"
Understanding DateTime in PowerShell
Definition of DateTime
In PowerShell, a DateTime variable represents a specific point in time and is crucial for various scripting tasks. Unlike simple string representations of dates, the DateTime object allows for more complex operations such as arithmetic, formatting, and timezone management. Understanding how to effectively use DateTime objects can enhance your PowerShell scripts significantly.
Creating DateTime Variables
You can create DateTime variables flexibly using the `Get-Date` cmdlet. This cmdlet retrieves the current date and time. For instance, to create a DateTime variable for the current time, one might use:
$CurrentDateTime = Get-Date
You can also specify a custom DateTime by constructing it directly through `[datetime]` or using `New-Object`. Here’s how to get today’s date:
$Today = Get-Date
To create a specific date, you can construct a DateTime object using `New-Object` like so:
$SpecificDate = New-Object -TypeName DateTime -ArgumentList 2023, 10, 3
Specifying Date and Time
You can create DateTime objects with exact year, month, day, hour, minute, and second values. The syntax for this is:
[datetime]::new(year, month, day, hour, minute, second)
For example, if you want to create a DateTime object for January 1, 2024, at Noon, you would write:
$NewYear = [datetime]::new(2024, 1, 1, 12, 0, 0)
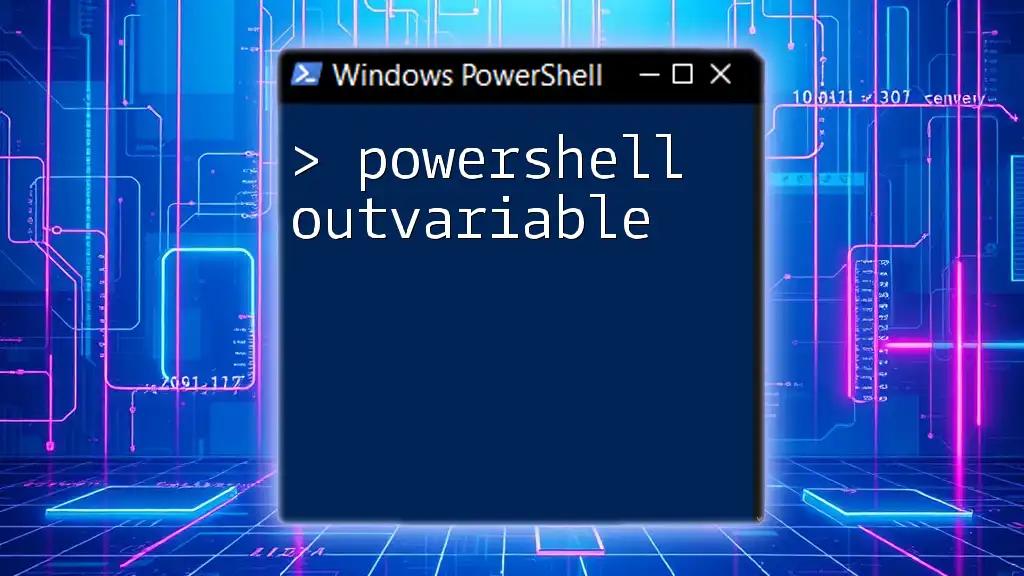
Manipulating DateTime Variables
Adding and Subtracting Time
PowerShell permits simple arithmetic on DateTime variables, enabling you to calculate future or past dates easily.
Using Add Methods
To add time to a DateTime object, you can use methods like `AddDays()`, `AddMonths()`, and `AddYears()`. Here’s how you can add 10 days to your current date:
$FutureDate = $CurrentDateTime.AddDays(10)
Similarly, if you want to see what date it will be three months from now, use:
$ThreeMonthsLater = $CurrentDateTime.AddMonths(3)
Using Subtraction
You can also subtract two dates to find the difference. For example, if you have two DateTime objects and you want to find how many days are between them:
$DaysDifference = ($FutureDate - $CurrentDateTime).Days
Formatting DateTime Output
To display DateTime variables in human-readable formats, you can utilize formatting options.
Using Format Strings
PowerShell offers built-in options like `-Format` for creating string representations of the date. For instance:
Get-Date -Format "yyyy-MM-dd HH:mm:ss"
This would yield the current date in a structured format.
Custom Formatting
You can refine how your DateTime appears by using the `.ToString()` method, providing custom format strings. For example, to see a fully descriptive date, you may use:
$CurrentDateTime.ToString("MMMM dd, yyyy")
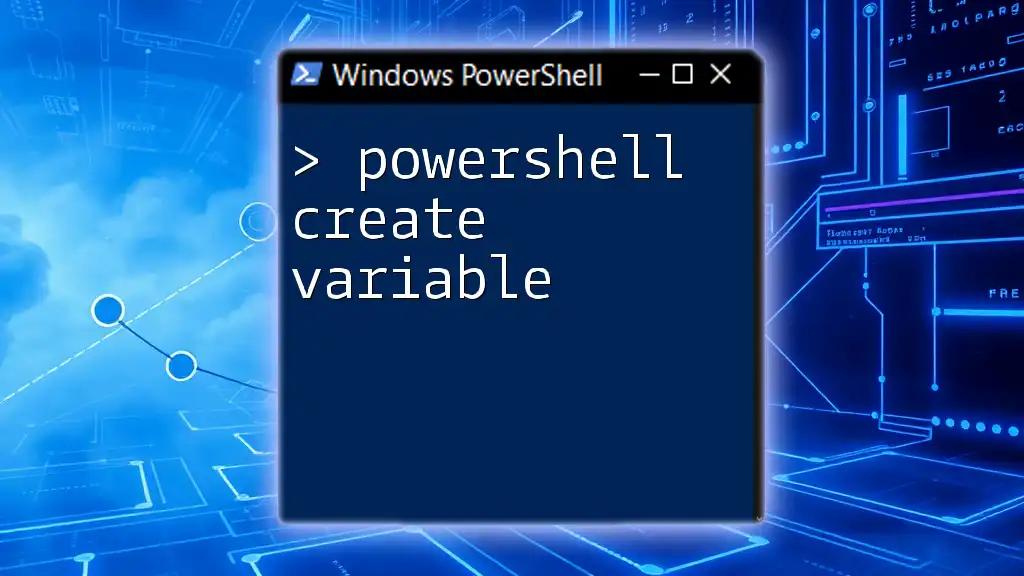
Common Use Cases for Date Variables
Comparing Dates
Sometimes, it's essential to compare two DateTime variables. PowerShell allows you to employ comparison operators. For instance, checking if one date is greater than another could look like this:
if ($SpecificDate -gt $Today) {
Write-Host "The specific date is in the future."
}
This could be pivotal when you wish to determine if deadlines are met or deadlines are upcoming.
Working with Time Zones
Understanding timezone differences is crucial when working with DateTime variables.
Getting Time Zone Information
You can obtain information about the local timezone using:
$LocalTimeZone = [System.TimeZoneInfo]::Local
And if you're interested in a specific timezone, you can find it using:
$UtcTimeZone = [System.TimeZoneInfo]::FindSystemTimeZoneById("UTC")
Converting Time Zones
Converting between time zones is straightforward with PowerShell. For instance, to convert from UTC to your local time, you would do:
$ConvertedTime = [System.TimeZoneInfo]::ConvertTime($UtcDateTime, $UtcTimeZone, $LocalTimeZone)
Date Arithmetic in Scripts
Date arithmetic can be handy in numerous scripting scenarios.
Scheduling Tasks
You can leverage DateTime variables to automate tasks based on specific dates. For example, scheduling a task to execute seven days from the current date can look like this:
$ScheduleDate = (Get-Date).AddDays(7)
Then, use `$ScheduleDate` for further task configurations.
Logging Events with Timestamps
Including timestamps in your logs can clarify when events occur. Here’s how you might log an event:
$LogEntry = "Event logged at: " + (Get-Date -Format "yyyy-MM-dd HH:mm:ss")
Write-Host $LogEntry

Practical Examples
Example 1: Create a Script to Display Current Date and Time
An everyday requirement is to display the current date and time. This simple script will do just that:
$CurrentDateTime = Get-Date
Write-Host "Current Date and Time: $CurrentDateTime"
Example 2: Send Reminder Emails Based on Date
You may want to send reminder emails when specific dates approach. Here’s how you could structure the logic:
$EventDate = Get-Date "2023-10-15"
if ($EventDate -lt (Get-Date).AddDays(7)) {
# Logic to send reminder email
}
This script checks if the event is set for less than a week away and triggers sending a reminder if it is.
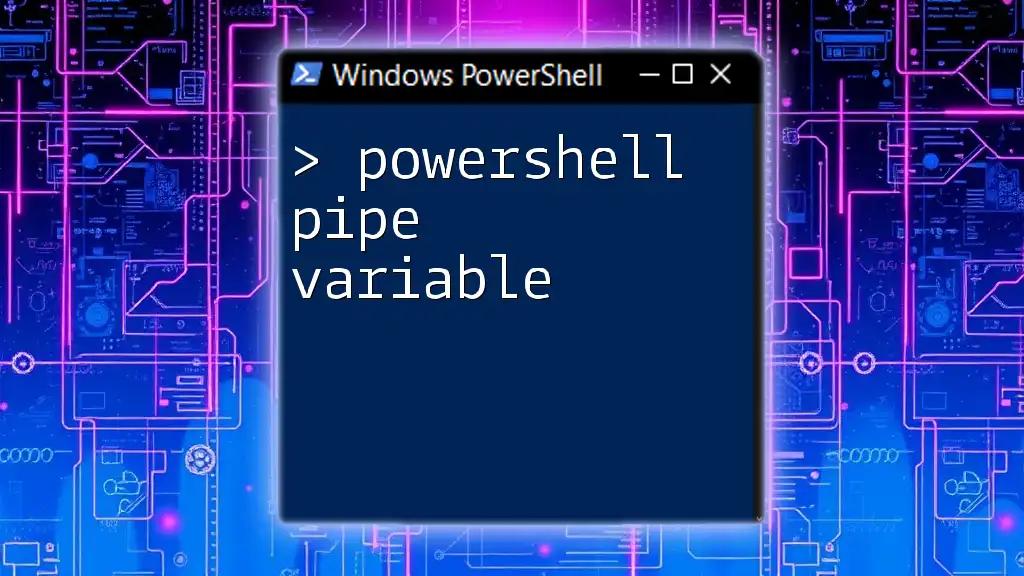
Debugging Common Issues with Dates
Time Zone Discrepancies
Time zone issues often arise when manipulating date variables, particularly when servers or user environments operate in different zones. Ensure your DateTime variables account for the correct time zones, using the conversion methods outlined above.
Formatting Errors
When converting dates to strings or vice versa, formatting errors can occur. Always ensure the format string matches your intended usage, and test snippets thoroughly to catch any potential formatting mismatches.
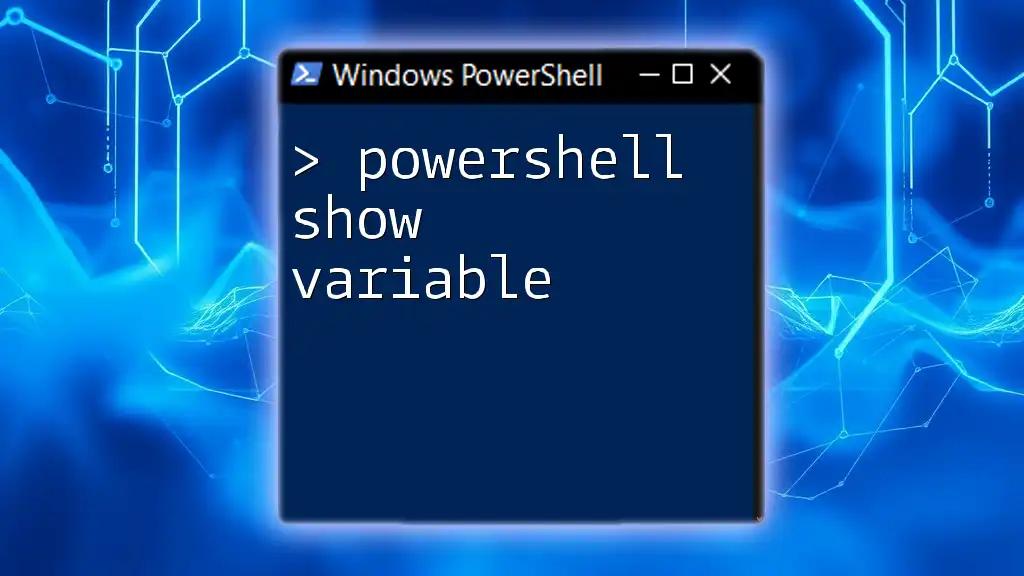
Conclusion
Mastering PowerShell date variables can greatly enhance your scripting capability, facilitating a variety of tasks ranging from scheduling to logging. By understanding how to create, manipulate, and format DateTime objects, you can produce powerful scripts tailored to your needs.
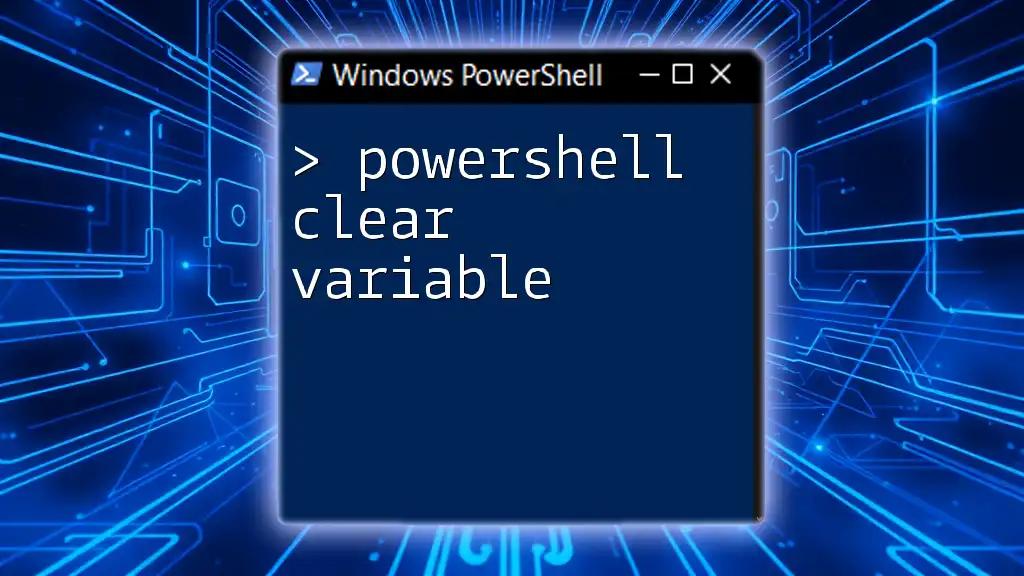
Additional Resources
Looking to deepen your knowledge? Explore [Microsoft PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/) for official guidelines, and join online forums and courses dedicated to PowerShell learning!