A PowerShell data table is a structured collection of data that allows you to organize and manipulate information in an efficient format. Here’s a simple example of how to create and display a data table:
# Create a new DataTable
$dataTable = New-Object System.Data.DataTable
# Define the columns
$dataTable.Columns.Add("Name", [string])
$dataTable.Columns.Add("Age", [int])
# Add rows to the DataTable
$dataTable.Rows.Add("Alice", 30)
$dataTable.Rows.Add("Bob", 25)
# Display the DataTable
$dataTable | Format-Table -AutoSize
Understanding Data Tables in PowerShell
What is a Data Table?
A Data Table is a crucial component of PowerShell that comes from the .NET Framework. It serves as a versatile way to manage structured data, similar to how databases do. Unlike simple arrays or hash tables, a Data Table can handle multiple data types and relationships among them, making it a preferred choice for complex data manipulation.
Understanding a Data Table allows for enhanced data management, primarily when handling larger datasets where organization and accessibility are crucial.
Key Features of PowerShell Data Tables
PowerShell Data Tables boast several notable features:
- Support for Complex Data: They can manage various types of data, including integers, strings, and dates, allowing for intricate data structures.
- Data Types and Structure: Each column in a Data Table can have a different data type, granting flexibility in how data is stored and accessed.
- Ease of Manipulation and Querying: Data Tables provide built-in methods for adding, modifying, and querying data, significantly streamlining the process of data handling.
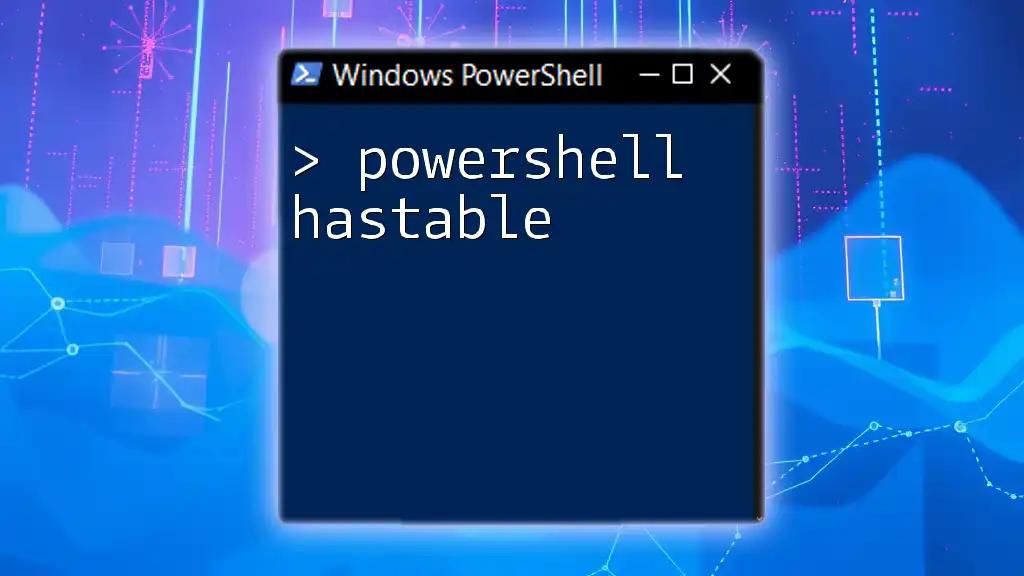
Creating a PowerShell Data Table
The Basic Syntax
To start working with a Data Table in PowerShell, you first need to create an instance of it. This is achieved with the following command:
$dataTable = New-Object System.Data.DataTable
This line initializes a new Data Table, setting the groundwork for adding data to it.
Adding Columns
Once you have a Data Table, the next step is to define its structure by adding columns. You can do this easily with the `Columns.Add` method. For example:
$dataTable.Columns.Add("Name", [string])
$dataTable.Columns.Add("Age", [int])
This code creates two columns: one for names as strings and another for ages as integers.
Adding Rows
To populate your Data Table with data, you need to add rows. This can be done by creating a new DataRow and filling it with values. Here's a simple way to achieve this:
$dataRow = $dataTable.NewRow()
$dataRow["Name"] = "John"
$dataRow["Age"] = 30
$dataTable.Rows.Add($dataRow)
This example introduces a new row with the name "John" and age 30.
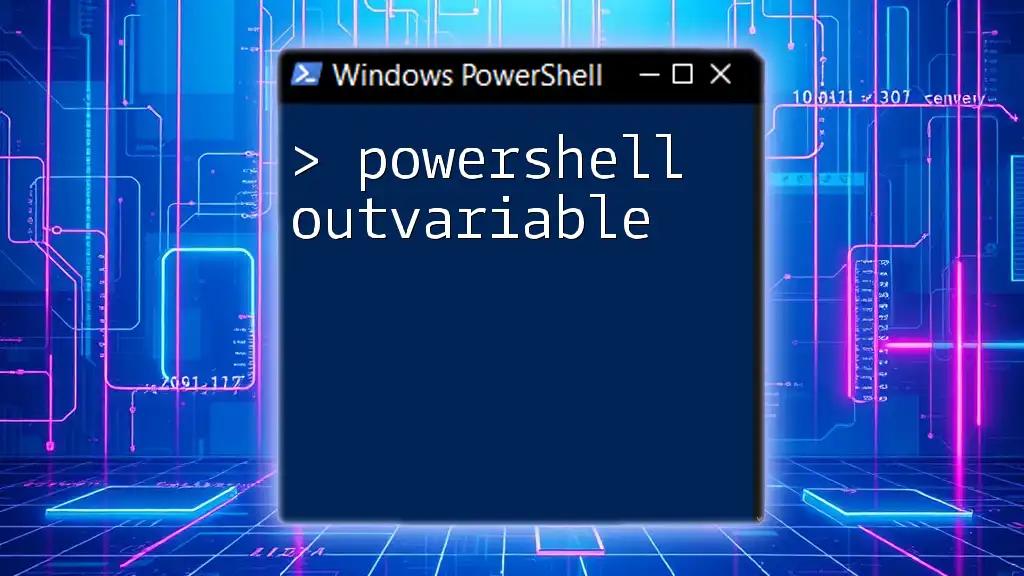
Manipulating Data Tables
Reading Data from Data Tables
Once your Data Table is populated, reading data from it is straightforward. You can loop through each row using:
foreach ($row in $dataTable.Rows) {
"$($row.Name) is $($row.Age) years old."
}
This code snippet neatly outputs the names and ages stored in the Data Table.
Modifying Data in Data Tables
Modifying values in a Data Table is equally easy. To update a value, access the specific row and column, as shown below:
$dataRow = $dataTable.Rows[0]
$dataRow["Age"] = 31
This piece of code finds the first row in the Data Table and updates John's age to 31.
Deleting Rows
Should you wish to remove entries from your Data Table, you can do so by using the `Remove` method. Here's how you can delete a row:
$dataTable.Rows.Remove($dataRow)
This command effectively removes the row you previously defined, thereby keeping your Data Table organized.
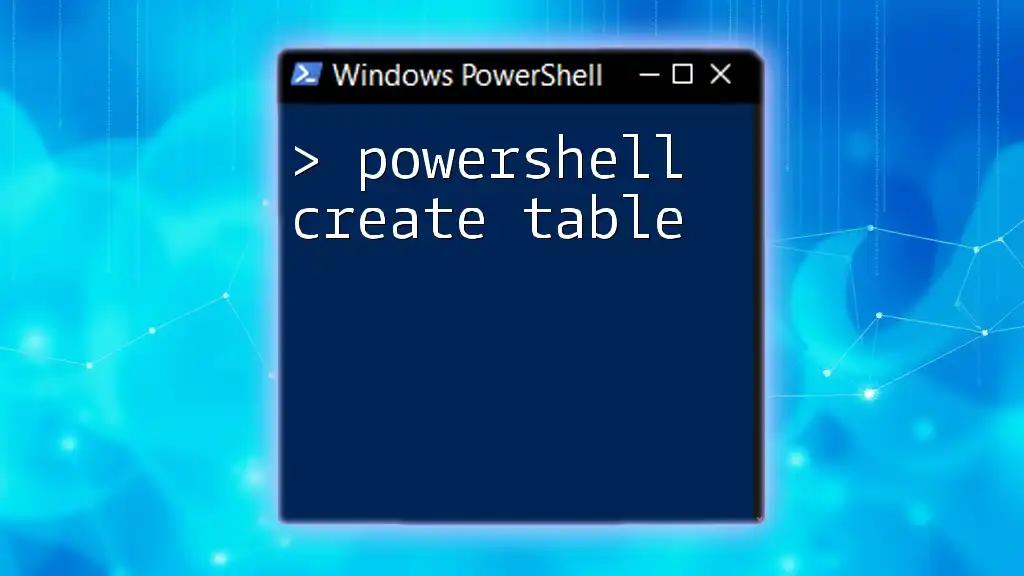
Advanced Features
Querying Data Tables with LINQ
PowerShell allows for more sophisticated querying through LINQ (Language Integrated Query). LINQ can filter and manipulate data directly within your Data Tables. Here’s how you can filter rows with a specific condition:
$filteredRows = $dataTable.AsEnumerable() |
Where-Object { $_.Age -gt 25 }
This example filters the Data Table to only include rows where the age is greater than 25.
Sorting Data in Data Tables
Sorting your data makes it easier to analyze and present. You can sort the entries in a Data Table by a particular column, such as:
$sortedRows = $dataTable.Select() | Sort-Object Age
In this instance, the rows would be sorted in ascending order based on the age column.
Filtering Data in Data Tables
Filtering allows you to narrow down results based on specific criteria. You can use a filter string like this:
$filter = "Name LIKE '%John%'"
$filteredData = $dataTable.Select($filter)
This command would select all rows where the Name column contains "John".
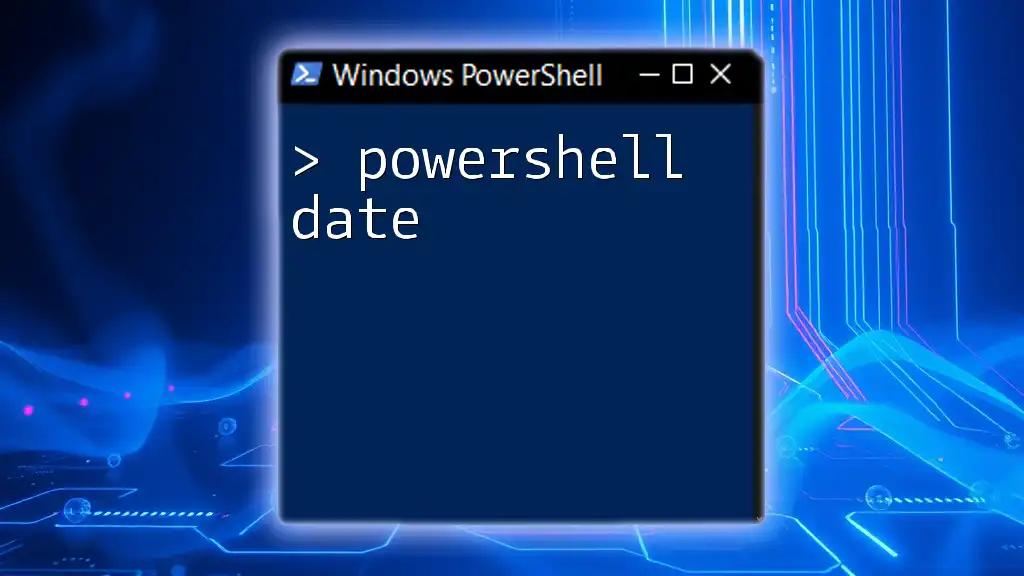
Practical Use Cases for PowerShell Data Tables
Data Import and Export
PowerShell Data Tables are excellent for importing and exporting data, especially with formats like CSV. Here’s a way to import a CSV file into a Data Table:
$importedData = Import-Csv "data.csv"
$dataTable = New-Object System.Data.DataTable
# Logic to fill the DataTable from imported data
This code sets up for further handling and analysis of data from an external source.
Data Transformation
Transformation of data formats can be accomplished easily within a Data Table. For example, you might want to convert names to uppercase while calculating future ages:
$newFormat = $dataTable | ForEach-Object {
[PSCustomObject]@{
FullName = $_.Name.ToUpper()
AgeInFiveYears = $_.Age + 5
}
}
This snippet transforms the data into a new format focusing on the desired outputs.
Reporting and Analysis
Data Tables enable effective reporting and analysis of information. To generate simple summaries, you can use:
$summary = $dataTable.Compute("SUM(Age)", "")
This would provide the total sum of ages contained in your Data Table, useful for quick reporting metrics.
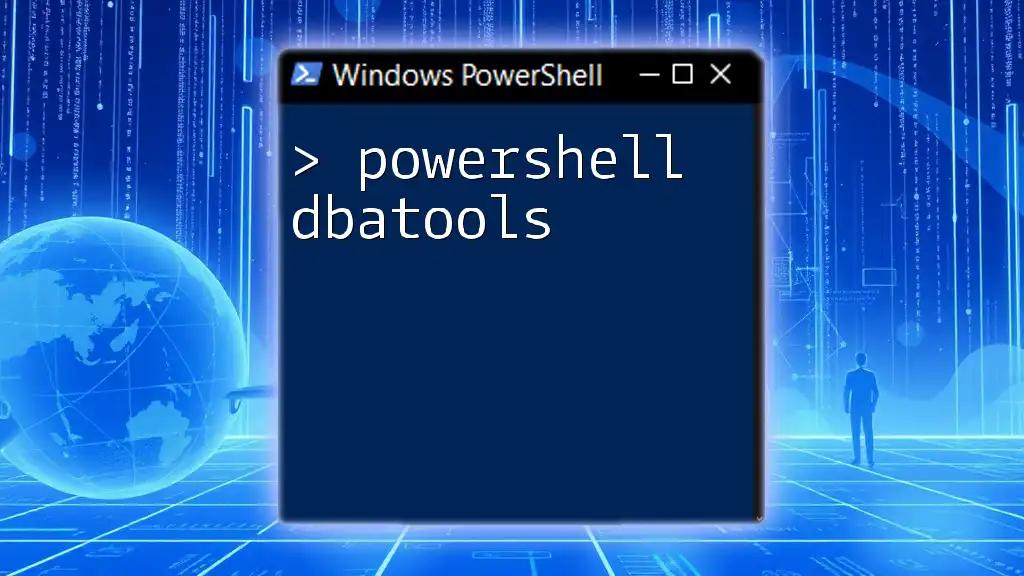
Common Pitfalls and Troubleshooting
Common Errors and Warning Messages
When working with PowerShell Data Tables, it’s important to understand that errors may arise, particularly concerning index out of bounds or incorrect data types. It pays to double-check if a column exists before attempting to retrieve its data.
Performance Considerations
Handling large datasets can introduce performance issues. To optimize performance, avoid unnecessary loops over rows. Instead, leverage built-in query methods and minimize data manipulation steps.
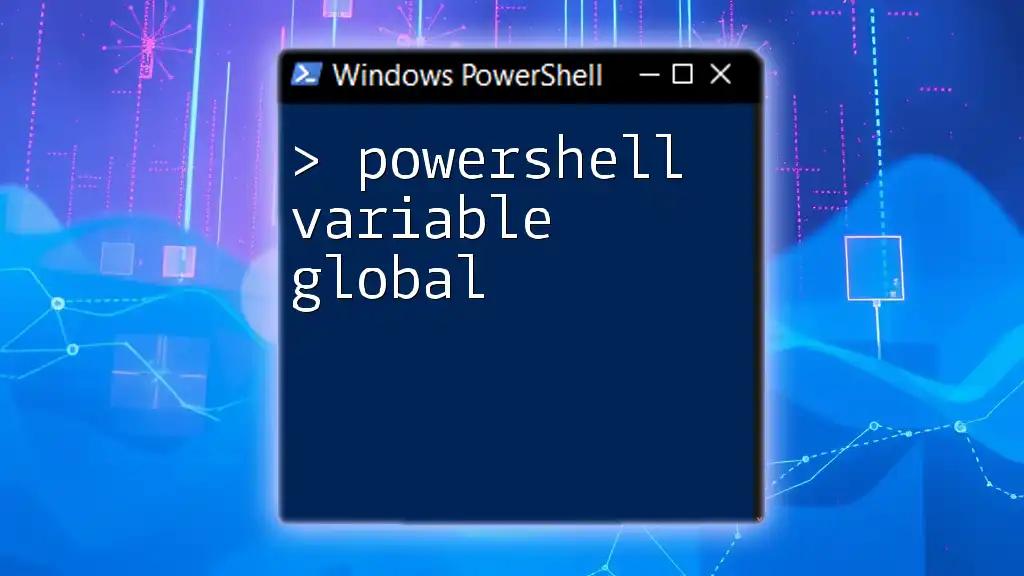
Conclusion
PowerShell Data Tables are a powerful tool for managing complex datasets efficiently. Understanding their structure, manipulation, and advanced features allows for robust data handling, whether for simple scripts or more elaborate automation tasks. By practicing these techniques, you’ll enhance your scripting skills and streamline your workflows.
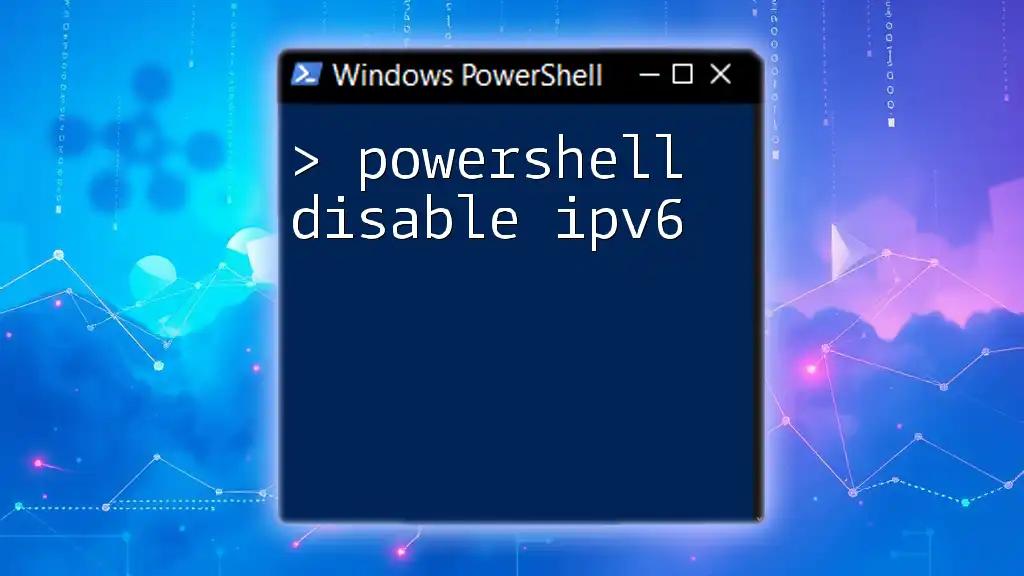
Resources for Further Learning
For more in-depth learning resources, consider exploring the official Microsoft documentation and reputable PowerShell courses that dive deeper into Data Tables and their practical applications.
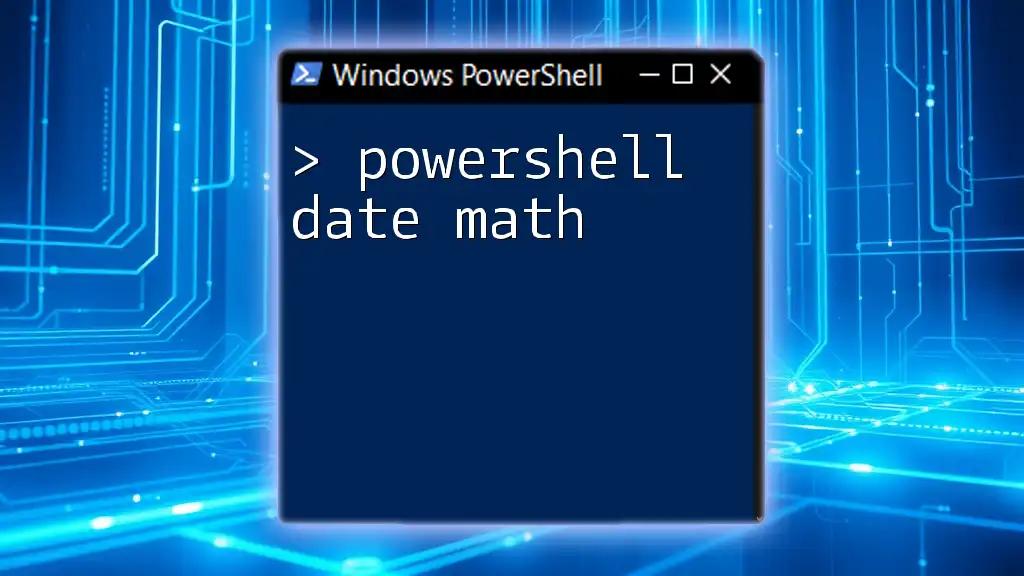
Call to Action
Don’t hesitate to share your experiences with PowerShell Data Tables in the comments! Exploring this versatile tool can significantly enhance your scripting productivity. Dive deeper into additional PowerShell content to expand your skill set further!