In PowerShell, you can create a table using the `New-Object` cmdlet to define a data table and then add columns and rows as needed. Here's a simple example:
# Create a new Data Table
$table = New-Object System.Data.DataTable
# Define columns
$column1 = New-Object System.Data.DataColumn("ID", [int])
$column2 = New-Object System.Data.DataColumn("Name", [string])
$table.Columns.Add($column1)
$table.Columns.Add($column2)
# Add a row
$row = $table.NewRow()
$row.ID = 1
$row.Name = "John Doe"
$table.Rows.Add($row)
# Display the table
$table | Format-Table
Understanding Tables in PowerShell
What is a Table in PowerShell?
In PowerShell, a table acts as a structured way to organize and manipulate data. Tables are often utilized to represent rows and columns, similar to database tables or spreadsheets. This makes them incredibly useful for storing data you may want to query, display, or manipulate during your PowerShell sessions.
While tables offer an organized structure for data, they differ from formats like CSV or JSON. Tables are primarily used within PowerShell for immediate use in scripts and pipelines, while CSV and JSON formats are commonly used for data exchange and storage.
Use Cases for Tables
Tables are especially beneficial in several scenarios, such as:
- Maintaining records where each entry has multiple attributes (e.g., user information).
- Selecting and filtering large datasets quickly.
- Presenting data in a more structured, readable format.
Using tables can greatly enhance your data handling capabilities in PowerShell, aligning with best practices in data organization.
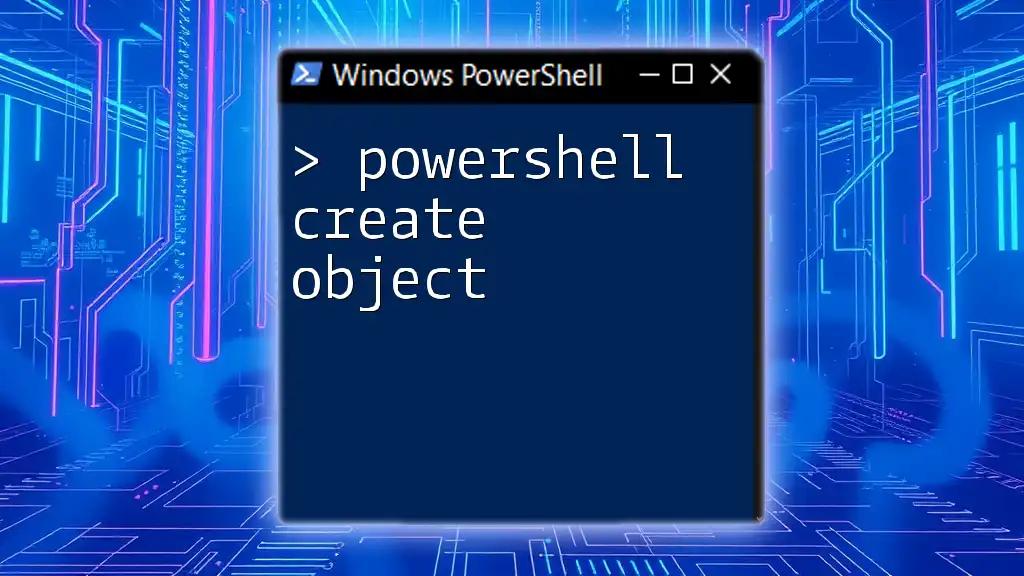
Creating a Table in PowerShell
Basic Command to Create a Table
To kick off our journey of PowerShell create table, we begin by using the `New-Object` cmdlet to generate a new table. The command is simple yet powerful:
$table = New-Object System.Data.DataTable
By executing this line, you are essentially declaring a new instance of a `DataTable` object. This serves as your foundation on which you can build your data structure.
Defining Columns for the Table
Every table requires a well-defined structure. We accomplish this by adding columns to our table, which ultimately dictates what data can be inserted.
To establish the columns, you can execute:
$table.Columns.Add("Id", [int])
$table.Columns.Add("Name", [string])
In this code snippet:
- The first line adds a column titled `Id` of type `int`, intended to hold integer values.
- The second line adds a column titled `Name` of type `string`, expected to store text values.
By clearly defining column types, you ensure that the data stored in your table maintains integrity and fits your intended structure.
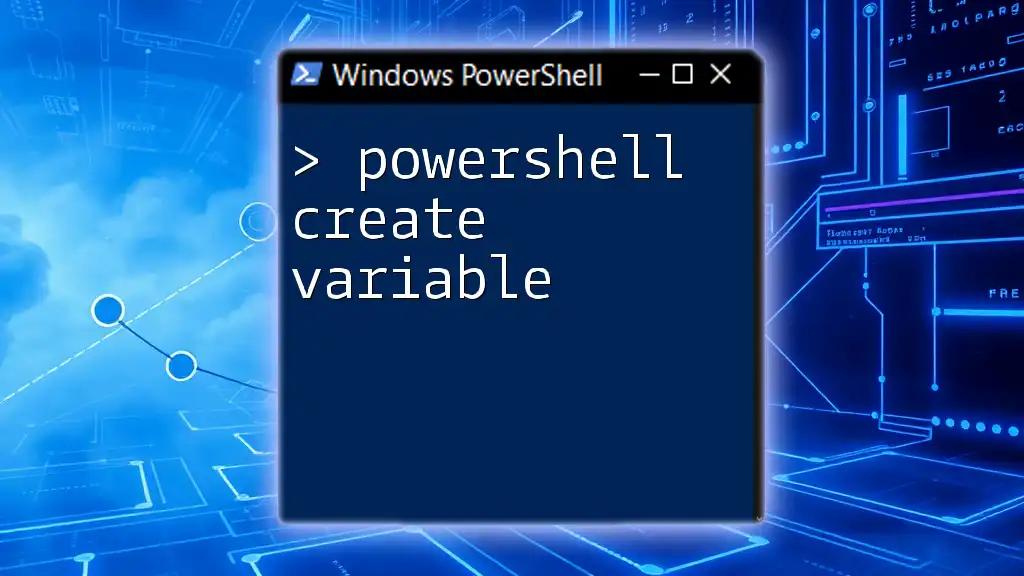
Populating the Table with Data
Adding Rows to the Table
Once the structure is in place, it’s crucial to populate your table with actual data. This is easily accomplished by creating a new row and assigning values to the respective columns.
Example:
$row = $table.NewRow()
$row["Id"] = 1
$row["Name"] = "John Doe"
$table.Rows.Add($row)
In this snippet:
- A new row is created by invoking `NewRow()` on the table, allowing you to dynamically add new entries.
- You assign the `Id` value of 1 and the `Name` value of "John Doe".
- Finally, you append this row to the table with `$table.Rows.Add($row)`.
This process gives your table meaningful data entries.
Looping to Add Multiple Rows
To expedite data entry, especially when dealing with larger datasets, you can employ a loop to generate multiple rows at once.
Consider the following loop:
for ($i = 1; $i -le 5; $i++) {
$row = $table.NewRow()
$row["Id"] = $i
$row["Name"] = "User $i"
$table.Rows.Add($row)
}
Here, you’re iterating from 1 to 5, creating a new row for each iteration. The `Id` is set to the current loop index, while the `Name` dynamically incorporates the index into its string (e.g., "User 1", "User 2", etc.). This loop significantly simplifies data population, especially when handling substantial datasets.
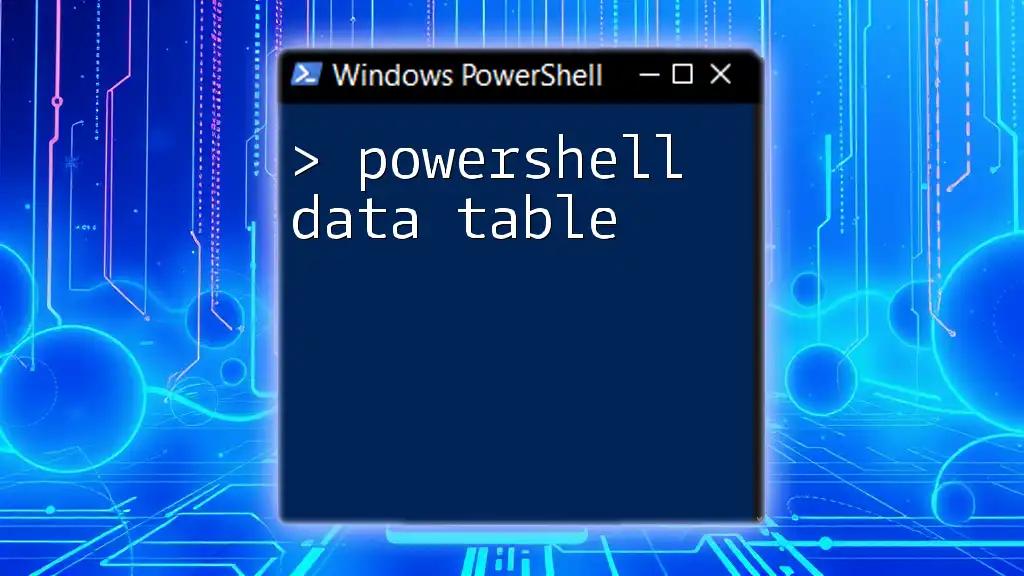
Displaying the Table
Outputting the Table Data
Once filled with data, you may want to display it effectively. Format-Table is a powerful cmdlet that provides a clean, readable way to showcase your table data.
To display your table's contents, use:
$table | Format-Table
This line will output the data in a tabular format directly in the PowerShell console, enhancing readability and usability.
Exporting Table Data
In some cases, you might want to save or share your table data. PowerShell offers various options for exporting data into different formats like CSV or XML.
For example, to save your table as a CSV file, you can execute:
$table | Export-Csv -Path "tableOutput.csv" -NoTypeInformation
This command takes your table, piping it to Export-Csv while specifying a file path. The `-NoTypeInformation` parameter denotes that you do not want to include the type information in the output file. This makes the resulting CSV cleaner and more straightforward for use in other applications.
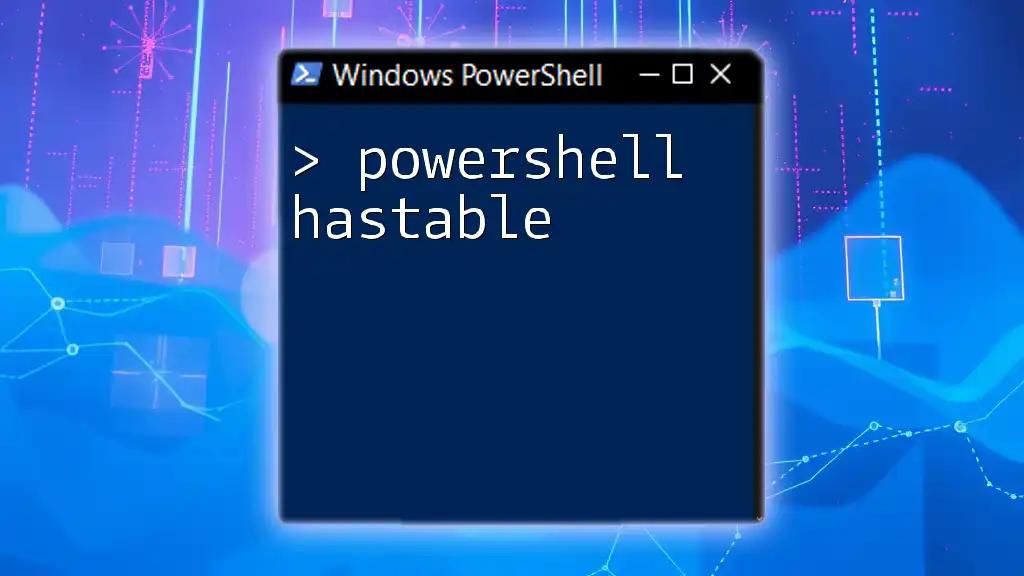
Advanced Table Manipulations
Adding Constraints to Table Columns
To ensure data integrity within your table, you may want to impose certain constraints. For instance, if you want the `Id` column to have unique values, you can set a constraint as follows:
$table.Columns["Id"].Unique = $true
This single line prevents any duplicate entries in the `Id` column, safeguarding against data entry errors that could complicate further data analysis.
Filtering and Sorting Table Data
PowerShell also allows querying table data using LINQ-like syntax. For instance, if you need to filter entries where `Id` exceeds 2, you can simply run:
$filteredRows = $table.Select("Id > 2")
$filteredRows | Format-Table
The Select method retrieves rows that meet specific criteria, storing them in `$filteredRows`. You can then display this subset of data neatly with Format-Table. Such querying capabilities make it easy to analyze and observe trends within your dataset.
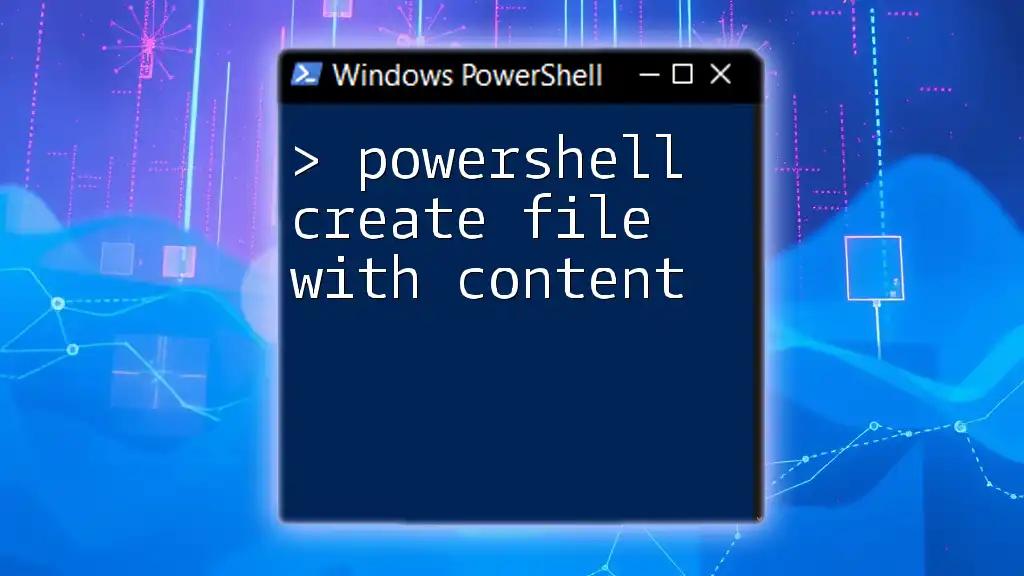
Common Errors and Troubleshooting
Common Issues When Creating Tables
Many beginners encounter common pitfalls, such as:
- Forgetting to define column types, leading to errors during data entry.
- Attempting to insert rows without first creating the necessary structure.
Troubleshooting Tips
Being aware of possible issues can facilitate a smoother experience:
- Always ensure that your columns are defined before adding data.
- When encountering errors, double-check your syntax and the data types being assigned.
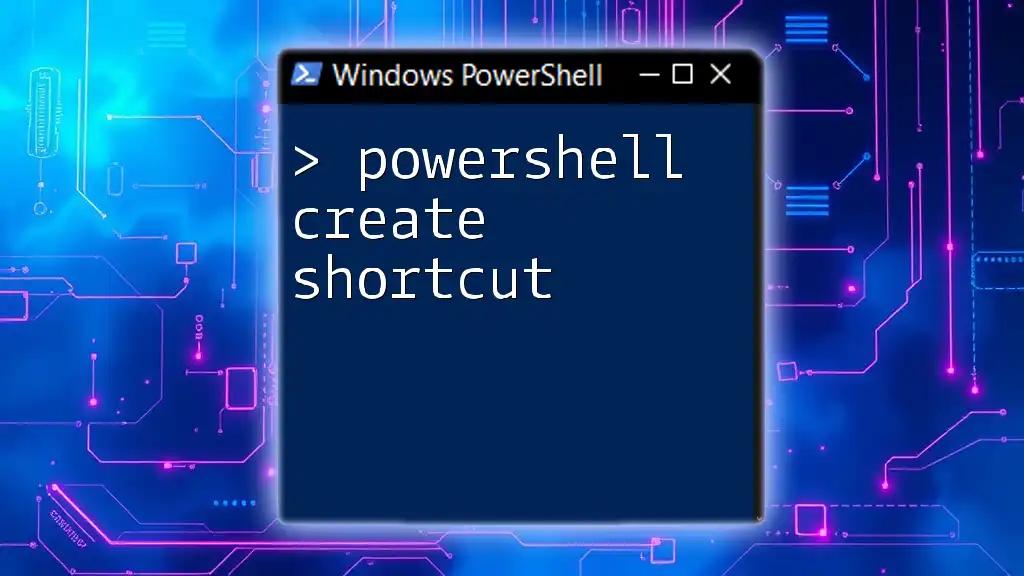
Conclusion
Throughout this guide, we explored how to PowerShell create table, from establishing a solid structure to populating it with relevant data. Understanding these concepts not only enriches your PowerShell skills but also enhances your effectiveness as a scripter or systems administrator.
To truly harness the power of PowerShell, continue experimenting and leverage the information presented here. There is always more to learn, and these foundational skills will serve you well in any PowerShell environment.
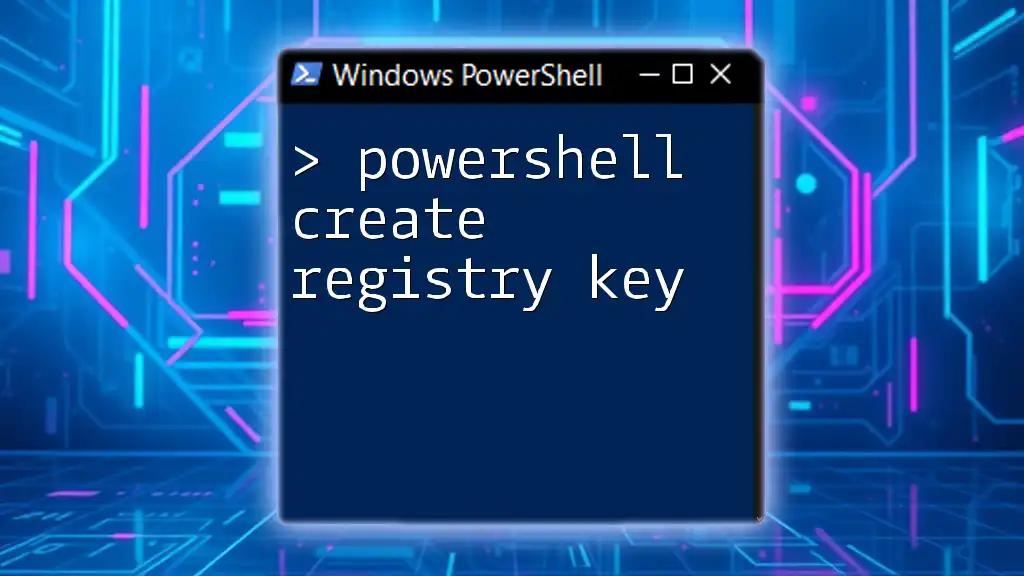
FAQs
Frequently Asked Questions
-
What are the advantages of using tables in PowerShell? Tables provide a structured way to handle data and enable easy manipulation, filtering, and exporting capabilities.
-
Can I use tables with data from other sources, like databases? Yes, PowerShell can interact with databases, and you can create tables based on queried data.
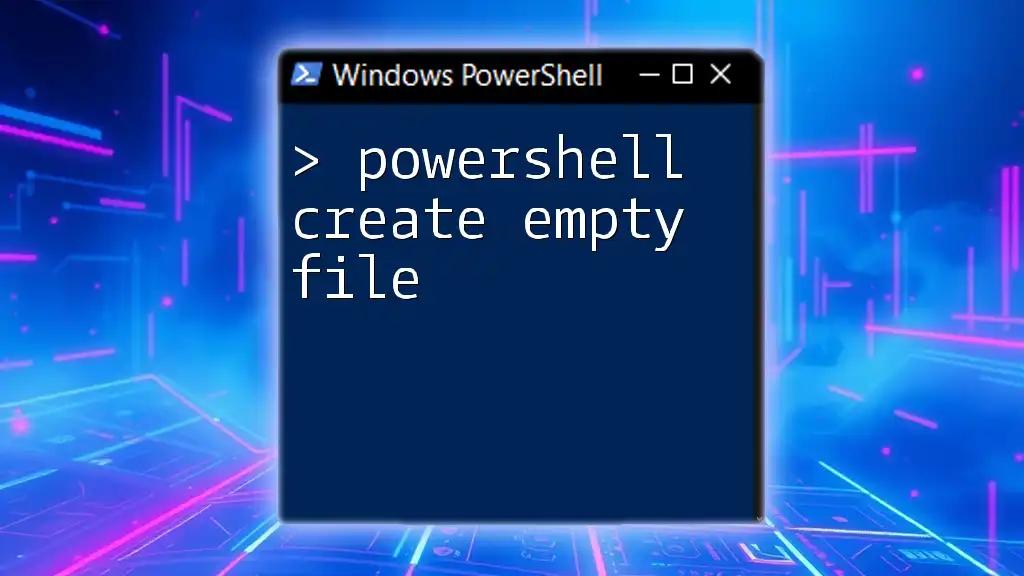
Additional Resources
- Official Microsoft Documentation on PowerShell and DataTables provides more in-depth information and examples for further learning.