To create an empty file in PowerShell, you can use the following command:
New-Item -Path 'C:\Path\To\Your\Folder\NewFile.txt' -ItemType File
This command generates an empty text file named `NewFile.txt` in the specified directory.
Understanding Empty Files in PowerShell
An empty file is a file that takes up space on disk but contains no data. These files can be useful in various scenarios, such as creating placeholders during development or scripting processes. While they hold no content initially, they can be altered later to include necessary data.
Using PowerShell to create empty files offers several advantages over traditional methods like Windows Explorer. With PowerShell, you can quickly automate the file creation process, especially when dealing with numerous files or integrating file management into scripts.
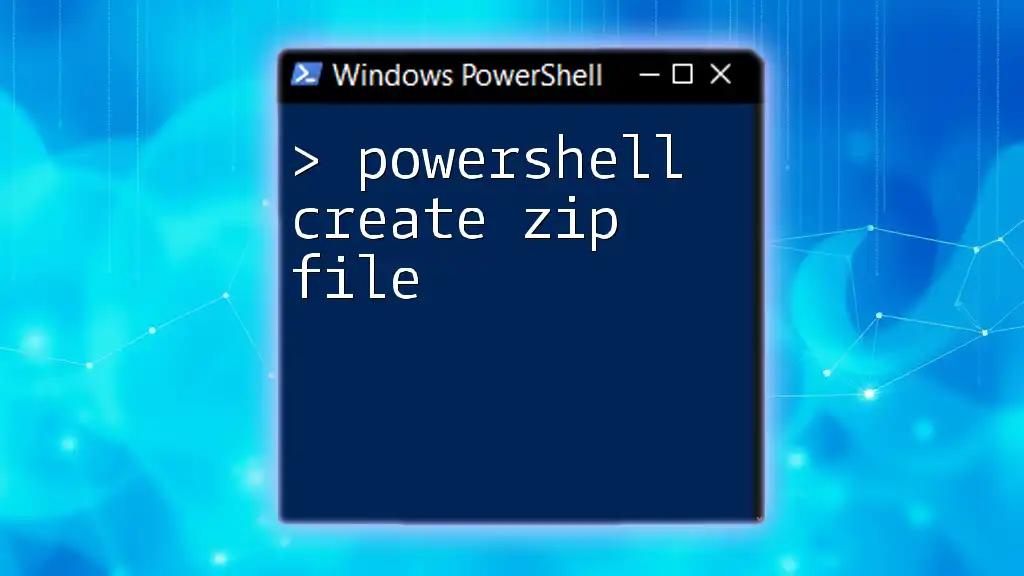
Methods to Create an Empty File in PowerShell
Using the `New-Item` Command
The `New-Item` cmdlet is a powerful way to create new items in PowerShell, including empty files. The basic syntax is straightforward and effective:
New-Item -Path "C:\path\to\your\file.txt" -ItemType "File"
For example, you can create a new empty text file in your Documents folder by replacing the path accordingly:
New-Item -Path "C:\Users\YourUser\Documents\emptyfile.txt" -ItemType "File"
This command understands that as the `-ItemType` is specified as "File," it will create an empty text file if it does not already exist.
Using the `Out-File` Command
Another way to create an empty file is by utilizing the `Out-File` cmdlet, a method mainly known for output redirection. The approach using redirection can be expressed as:
"" | Out-File -FilePath "C:\path\to\your\file.txt"
To create an empty file specifically, you would execute:
"" | Out-File -FilePath "C:\Users\YourUser\Documents\emptyviaout.txt"
This command effectively redirects an empty string to the specified file path, resulting in an empty file.
Using the `Set-Content` Command
The `Set-Content` cmdlet can also help create empty files by setting content to `$null`, ensuring that you generate a file without any data. The syntax for this method is:
Set-Content -Path "C:\path\to\your\file.txt" -Value $null
Here’s an example:
Set-Content -Path "C:\Users\YourUser\Documents\emptysetcontent.txt" -Value $null
This command instructs PowerShell to create a file at the designated path with no content, merging simplicity with efficiency.
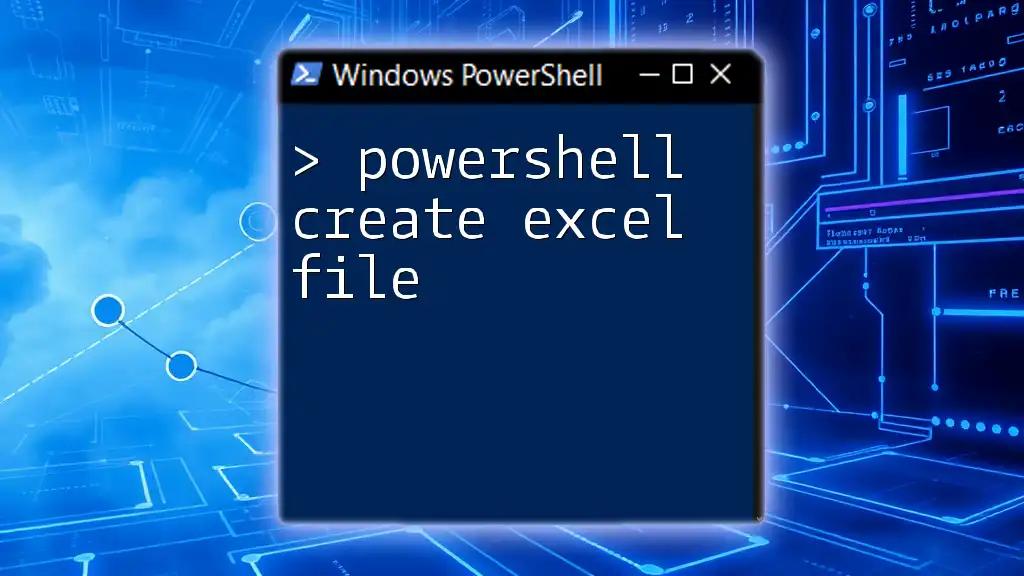
Frequently Asked Questions
Can I create multiple empty files at once?
Yes! You can create multiple empty files by using a loop construct in PowerShell. An example using the `ForEach-Object` cmdlet looks like this:
1..5 | ForEach-Object { New-Item -Path "C:\path\to\your\emptyfile$_" -ItemType "File" }
This will create five empty files with sequential naming, such as `emptyfile1`, `emptyfile2`, and so on.
What if the file already exists?
If the file already exists at the specified path, you may encounter an error. To overwrite the existing file, use the `-Force` parameter with the `New-Item` cmdlet:
New-Item -Path "C:\path\to\your\file.txt" -ItemType "File" -Force
Adding `-Force` allows you to replace any existing file at the path without prompting for confirmation.
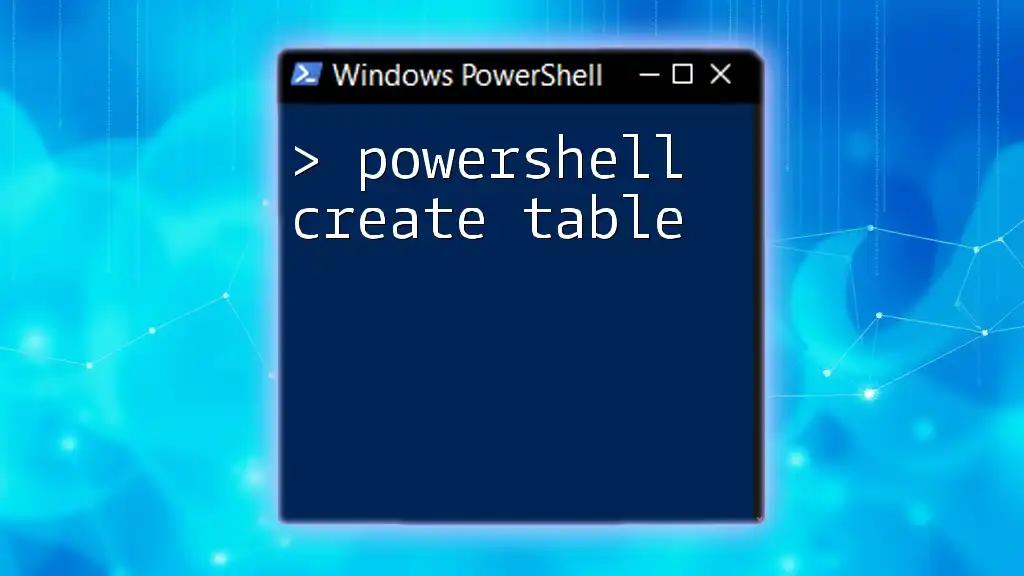
Best Practices for Creating Empty Files
Naming Conventions
When creating empty files, it's crucial to follow good naming conventions. Descriptive names help you remember the purpose of each file, especially when dealing with numerous files. Consider using meaningful prefixes or suffixes that indicate the intended use.
File Location Considerations
Organizing your files logically within directories enhances accessibility. Strive to keep similar files together in relevant folders, making it easier to remember where you’ve saved them. This practice is valuable, especially when automating tasks through scripts.
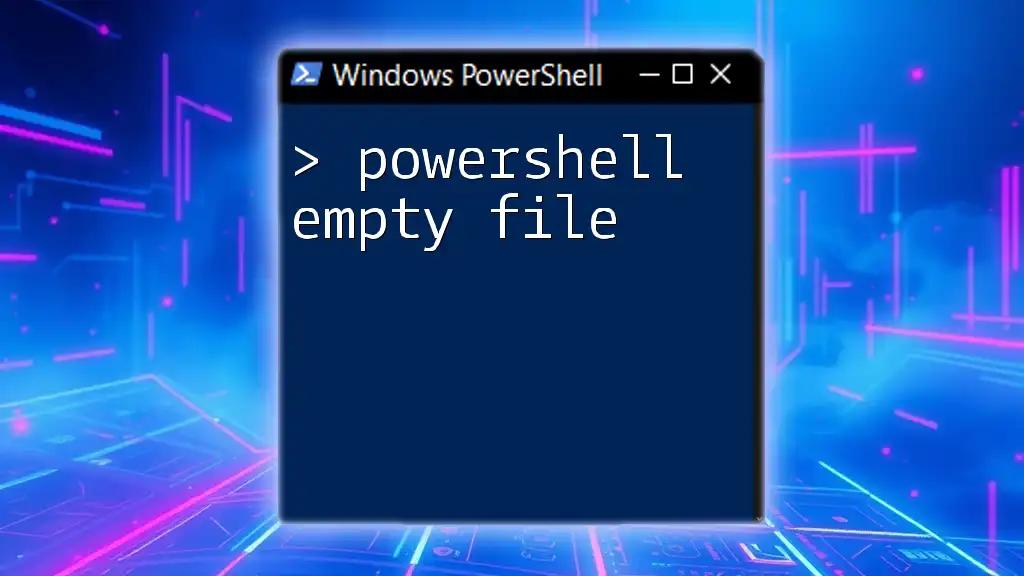
Conclusion
Having explored the methods to powerfully create empty files in PowerShell, you are now equipped with essential tools for effective file management. Whether you choose to use `New-Item`, `Out-File`, or `Set-Content`, each method provides unique advantages for your specific requirements.
As you continue to work with PowerShell, consider utilizing these techniques in your projects. The ability to automate file creation will save you time and streamline your efforts.
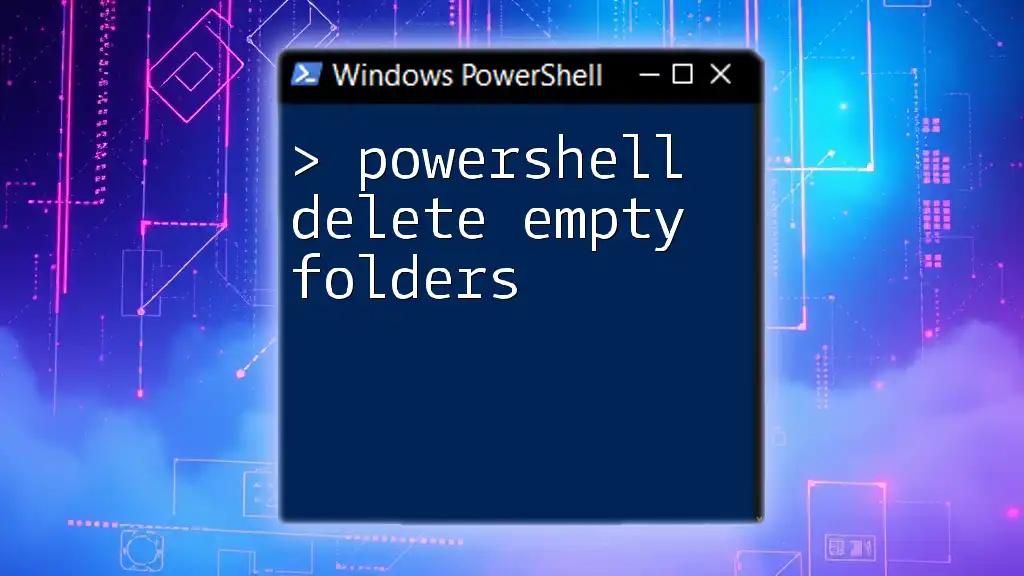
Further Reading
For those looking to expand their knowledge of PowerShell, consider delving into topics like file management, automation scripts, and even advanced cmdlet usage. These resources will aid you on your journey to mastering PowerShell.