To create a zip file using PowerShell, you can utilize the `Compress-Archive` cmdlet, which allows you to specify the source files and the destination zip file in a streamlined manner.
Here's a code snippet to demonstrate this:
Compress-Archive -Path 'C:\Path\To\Your\Files\*' -DestinationPath 'C:\Path\To\Your\Archive.zip'
Understanding Zip Files
Zip files are a convenient way to compress and package multiple files and directories into a single file. They reduce storage space and make it easier to transfer large amounts of data. In the realm of IT and everyday use, zip files serve various purposes, including backing up important documents, distributing software, and bundling resources for web projects. The benefits of using zip files include reduced file sizes, faster transfer speeds, and better organization.
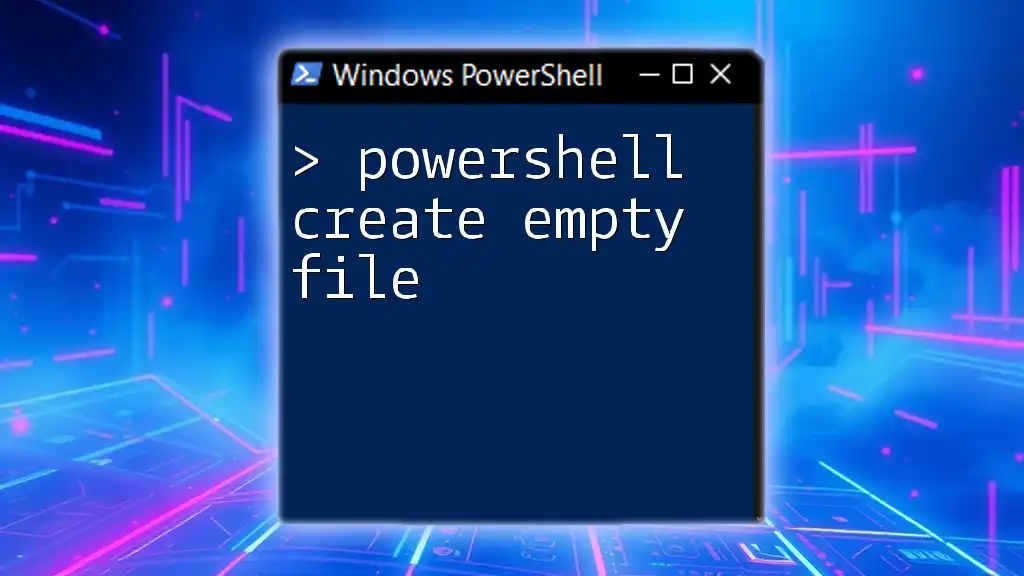
Why Use PowerShell to Create Zip Files?
Using PowerShell to create zip files offers numerous advantages over traditional graphical user interfaces. PowerShell allows automation, making it easier to create scripts that can generate zip files quickly and repeatedly without manual intervention. This scripting capability is particularly useful for tasks like backup processes, where you might need to zip a folder daily.
Moreover, PowerShell's integration with other scripts and tools means you can streamline your workflows and integrate file compression into larger processes effortlessly.
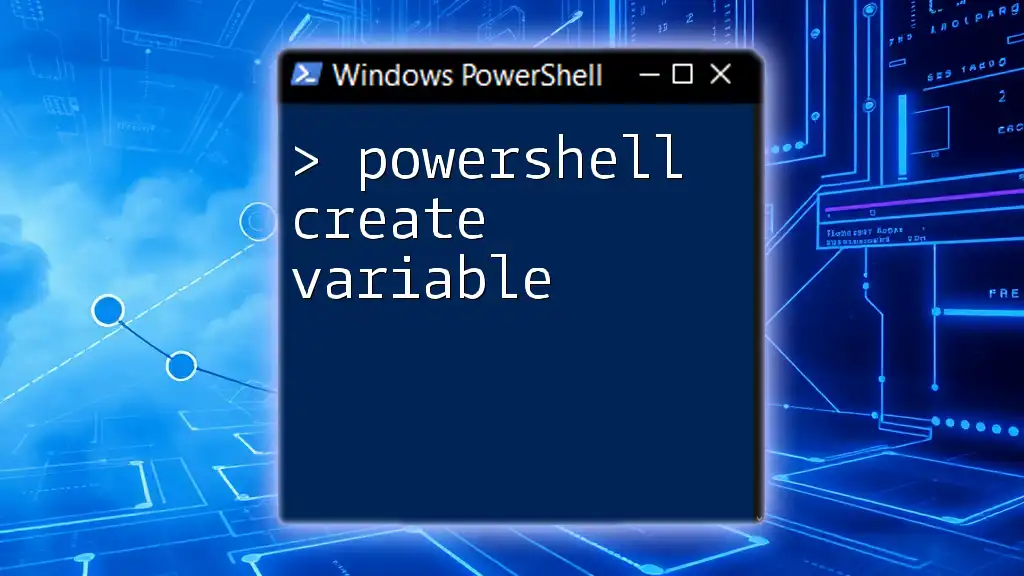
Getting Started with PowerShell
Installing PowerShell
PowerShell is available across various platforms, including Windows, macOS, and Linux. To ensure you have the latest features, it’s crucial to install or update to the latest version of PowerShell. You can find installation packages on the [official PowerShell GitHub releases page](https://github.com/PowerShell/PowerShell/releases).
Accessing PowerShell
Once installed, starting PowerShell depends on your operating system:
- Windows: Search for “PowerShell” in the Start menu or use `Win + R`, type `powershell`, and hit Enter.
- macOS: Open Terminal and type `pwsh` after installing PowerShell Core.
- Linux: Launch your terminal and type `pwsh`.
For effective usage, consider running PowerShell as an administrator, especially when working with system files or performing administrative tasks.
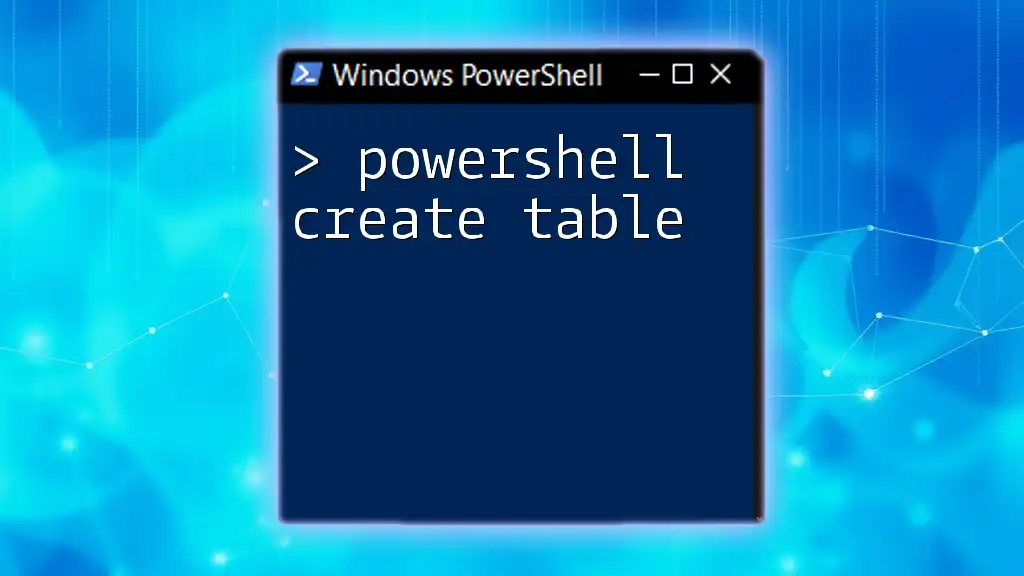
Basic Syntax for Creating a Zip File in PowerShell
In PowerShell, the `Compress-Archive` cmdlet is your primary tool for file compression. Its basic syntax is straightforward:
Compress-Archive -Path "<source_path>" -DestinationPath "<destination_path>"
Here, `-Path` specifies the files and folders you want to compress, while `-DestinationPath` denotes where the zip file will be created.
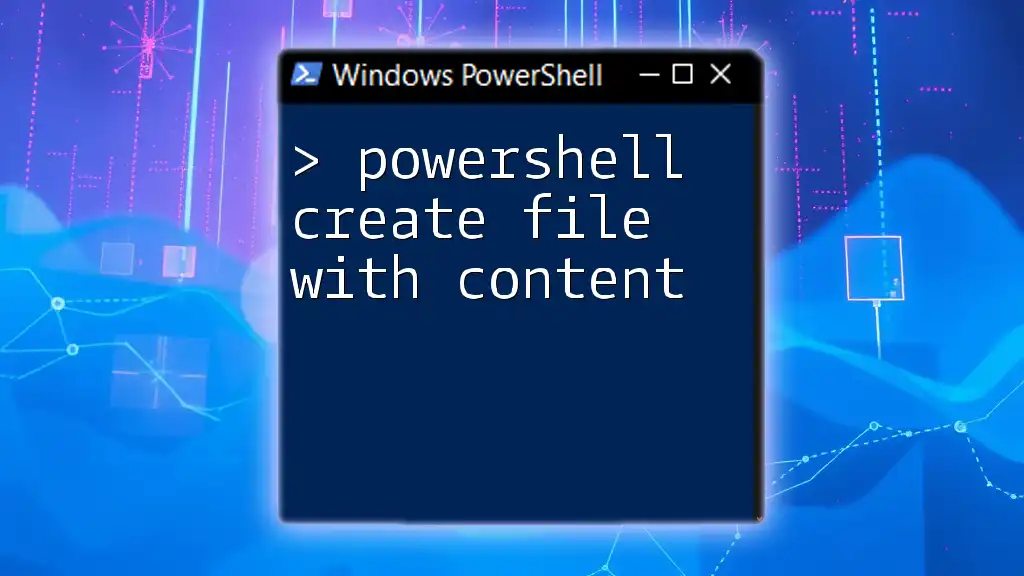
Creating a Zip File: Step-by-Step Guide
Preparing Your Files
Before creating a zip file, choose the files and folders you want to include. Organizing your files logically can make zipping easier and help streamline future retrieval.
Writing the PowerShell Command
The simplest command to create a zip file might look like this:
Compress-Archive -Path "C:\Path\To\Your\Files\*" -DestinationPath "C:\Path\To\Your\Zips\MyArchive.zip"
In this command:
- The asterisk (`*`) indicates that all files within the specified directory will be included in the zip.
- The destination path is where your new zip file will reside.
Using Variables for Dynamic Paths
To enhance readability and maintainability, you can set variables for your paths:
$sourcePath = "C:\Path\To\Your\Files\*"
$destinationPath = "C:\Path\To\Your\Zips\MyArchive.zip"
Compress-Archive -Path $sourcePath -DestinationPath $destinationPath
This approach makes it easier to modify your paths without combing through your script, and it clarifies what each path represents.
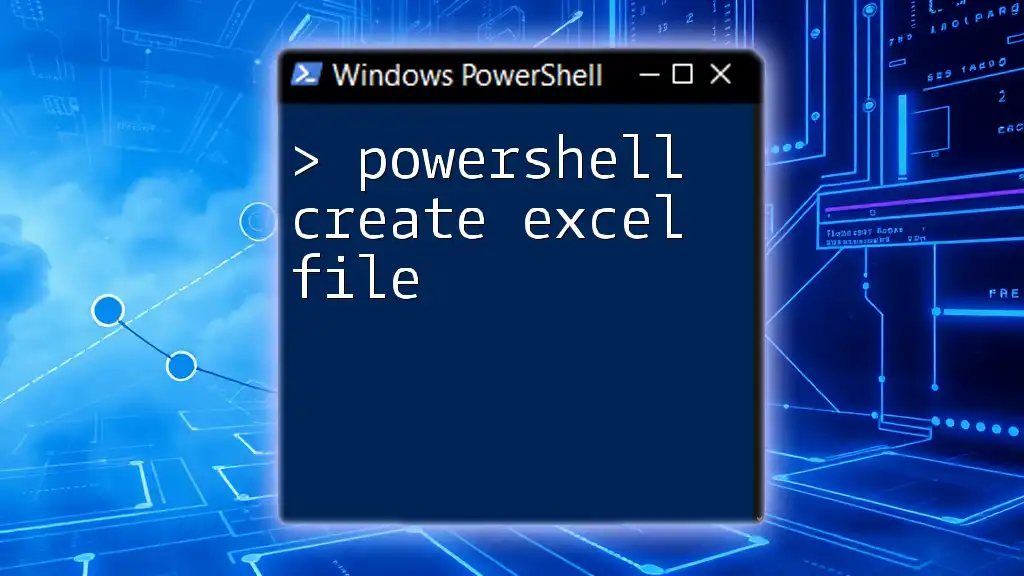
Advanced Techniques for Creating Zip Files
Zipping Multiple Files and Folders
When faced with zipping several files and folders, consider using an array:
$items = "C:\Path\To\File1.txt", "C:\Path\To\Folder1", "C:\Path\To\File2.txt"
Compress-Archive -Path $items -DestinationPath "C:\Path\To\Your\Zips\MyArchive.zip"
This method allows you to package a diverse set of files in one operation, ideal for multifaceted projects.
Excluding Files from Compression
Sometimes, you may want to exclude certain file types. The `-Exclude` parameter can help:
Compress-Archive -Path "C:\Path\To\Your\Files\*" -DestinationPath "C:\Path\To\Your\Zips\MyArchive.zip" -Exclude "*.tmp"
This example creates a zip file of all files excluding those ending in `.tmp`, which is useful for leaving out temporary or unnecessary files.
Overwriting Existing Zip Files
If you need to overwrite an existing zip file without prompts, the `-Force` parameter can be used:
Compress-Archive -Path $sourcePath -DestinationPath $destinationPath -Force
Ensure you understand the implications of overwriting files, as this action is irreversible.
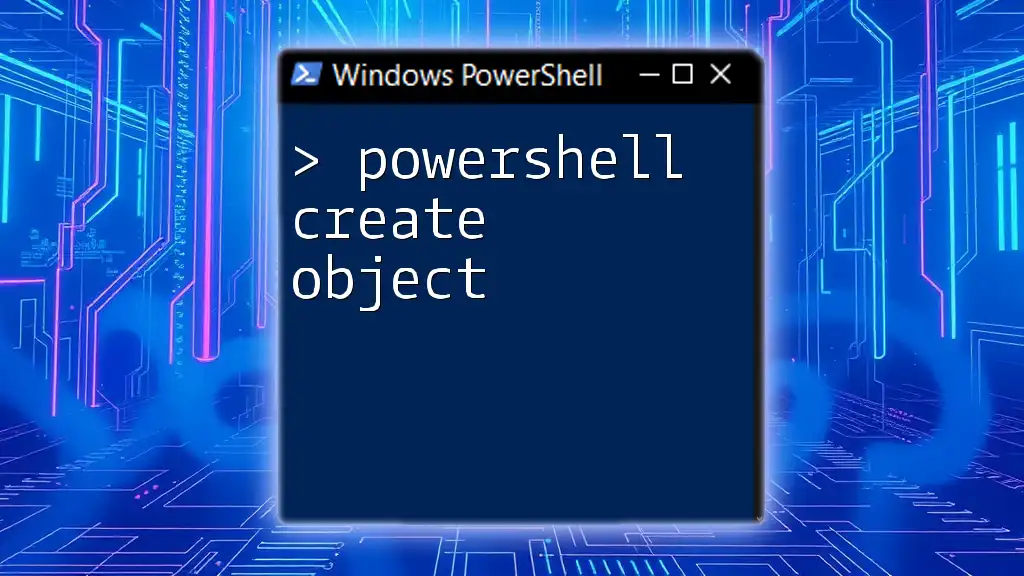
Automating Zip Creation with PowerShell Scripts
Saving Your Commands as Scripts
By saving your PowerShell commands in a `.ps1` file, you can reuse them easily:
# Example script for daily backups
$sourcePath = "C:\ImportantFiles\*"
$destinationPath = "C:\Backups\Backup_$(Get-Date -Format 'yyyyMMdd').zip"
Compress-Archive -Path $sourcePath -DestinationPath $destinationPath
This script allows you to create a backup zip that includes the date, making file management more straightforward.
Scheduling Zip Creation with Task Scheduler
To automate your script, use Windows Task Scheduler. Set it up by following these general steps:
- Open Task Scheduler and create a new task.
- Set the trigger schedule (daily, weekly, etc.).
- In the action, select “Start a program” and point it to `powershell.exe`.
- Use the argument `-File “C:\Path\To\Your\Script.ps1”`.
This automation can help maintain regular backups without manual effort.
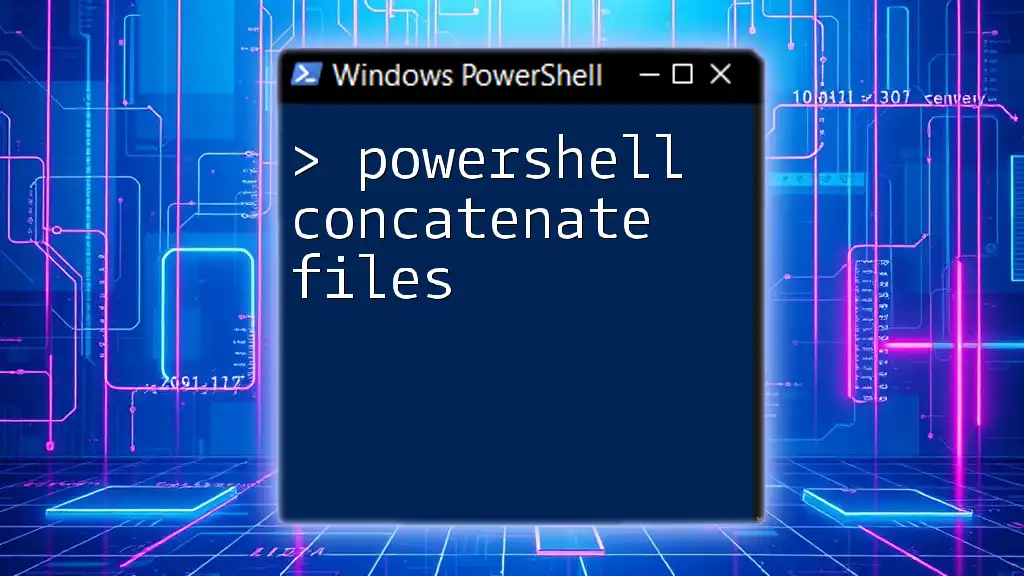
Troubleshooting Common Issues
Common Errors and Solutions
While creating zip files, you might encounter errors such as “File already exists.” In these cases, consider modifying your command by using the `-Force` parameter to overwrite or ensure that the paths you specify are correct.
Best Practices for Managing Zip Files
To keep your zipped files organized, establish a naming convention and system for archiving. Regular maintenance to remove outdated files from archives can also prevent storage bloating and make future retrieval straightforward.
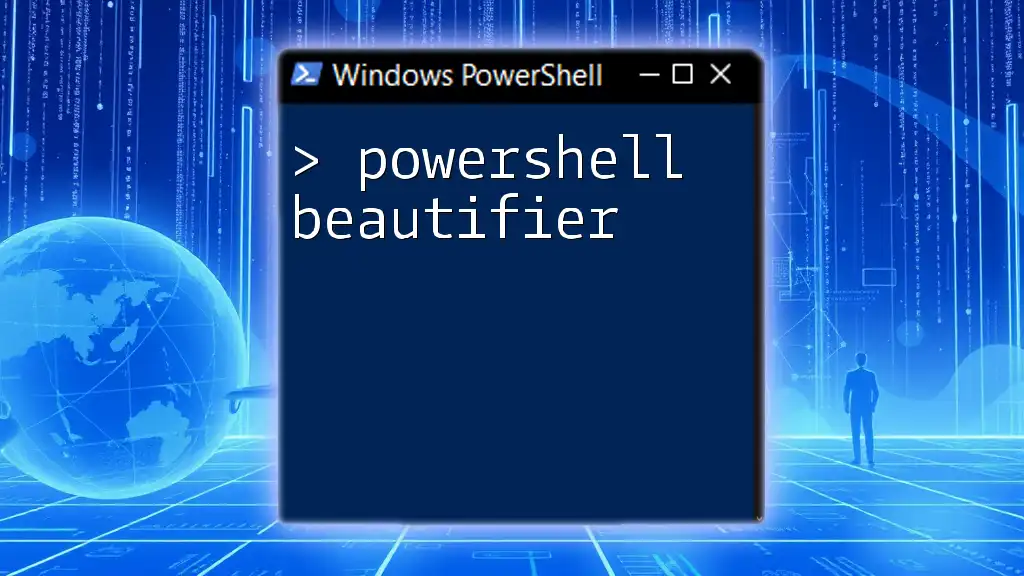
Conclusion
Utilizing PowerShell to create zip files streamlines file management and introduces automation into your workflows. By mastering cmdlets like `Compress-Archive`, you can harness the power of PowerShell for efficient data handling. Don't hesitate to explore and practice these commands—your productivity will thank you!
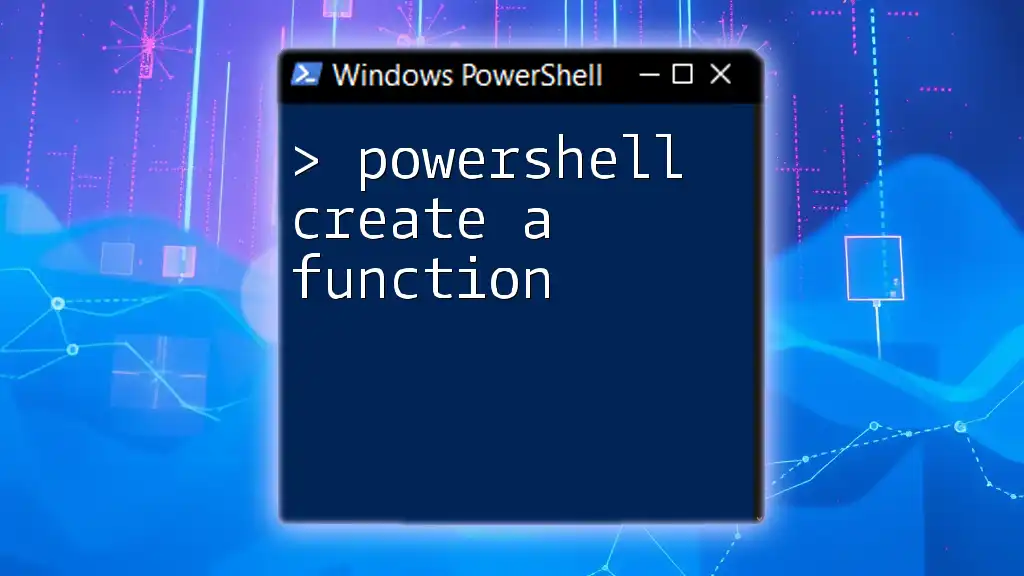
Additional Resources
For those looking to deepen their PowerShell knowledge, consider exploring the official [Microsoft PowerShell documentation](https://docs.microsoft.com/powershell/) as well as community forums and video tutorials that cover practical applications of PowerShell commands. Recommended books are also available for a more structured learning experience.