Certainly! Here's a concise explanation along with a code snippet for creating an Excel file using PowerShell:
To create an Excel file using PowerShell, you can utilize the `Excel.Application` COM object to automate the generation of an Excel spreadsheet.
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Add()
$worksheet = $workbook.Worksheets.Item(1)
$worksheet.Cells.Item(1,1) = "Hello, Excel!"
$workbook.SaveAs("C:\Path\To\Your\File.xlsx")
$excel.Quit()
Make sure to change `"C:\Path\To\Your\File.xlsx"` to your desired file path.
Understanding PowerShell and Excel Interactions
What is PowerShell?
PowerShell is a powerful scripting language developed by Microsoft that is designed for task automation and configuration management. It serves as both a command-line shell and an object-oriented scripting language that allows system administrators and power users to automate administrative tasks. With PowerShell, users can perform complex operations and manage system configurations more efficiently compared to traditional methods.
Overview of Excel Interactions
PowerShell can interact seamlessly with Excel through both its inherent capabilities and third-party modules, enabling tasks such as data manipulation, report generation, and dashboard creation. Common applications for this interaction include generating automated reports, analyzing data, and creating visualizations without the need for user input.
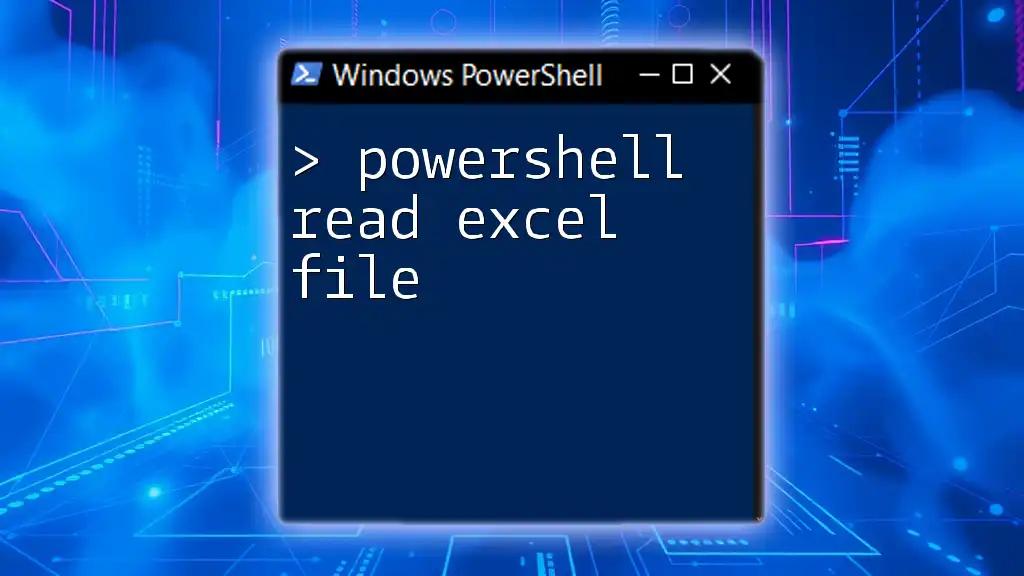
Prerequisites for Creating an Excel File with PowerShell
Required Software
Before diving into the technical aspects, ensure you have the necessary software:
- Microsoft Excel must be installed on your machine, as PowerShell will drive the Excel application to create and manipulate files.
- A compatible version of Windows PowerShell (ideally PowerShell 5.1 or higher) is recommended for script execution.
- To streamline Excel file creation, consider installing the ImportExcel Module, which simplifies the process.
Enabling PowerShell Scripting
By default, the execution of PowerShell scripts may be restricted. To check your current execution policy, run the following command:
Get-ExecutionPolicy
If the output is `Restricted`, you'll need to change it to `RemoteSigned` or `Unrestricted` to allow script execution. You can modify the execution policy using this command:
Set-ExecutionPolicy RemoteSigned
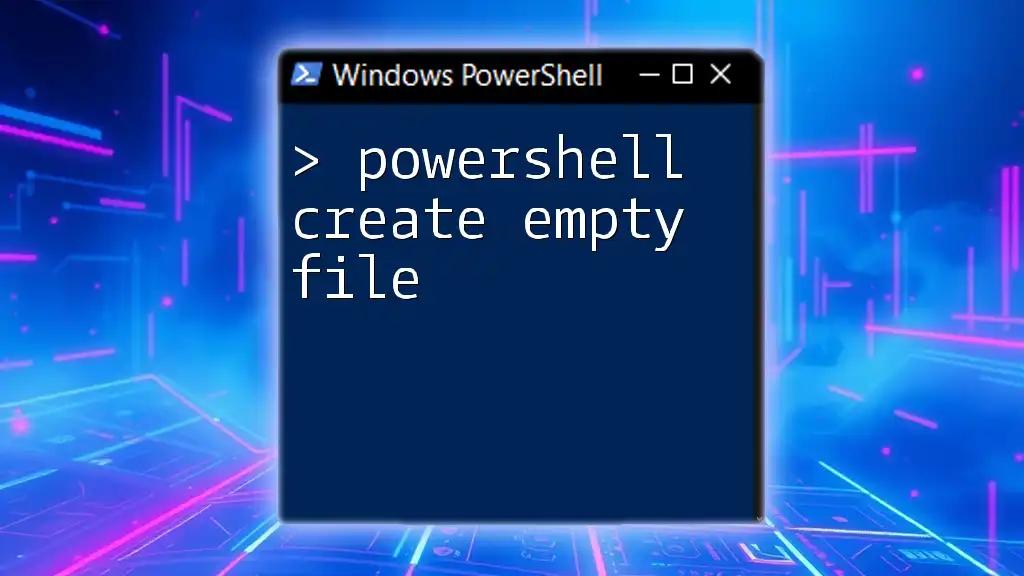
Setting Up Your PowerShell Environment
Installing the ImportExcel Module
The ImportExcel module significantly enhances the functionality of PowerShell when it comes to creating and manipulating Excel files. To install this module, run:
Install-Module -Name ImportExcel -Scope CurrentUser
Ensure you accept any prompts and wait for it to complete. This module is lightweight and should not impact system performance.
Importing the Module
Once the module is installed, you need to import it into your PowerShell session to access its functionalities. You can do so using:
Import-Module ImportExcel
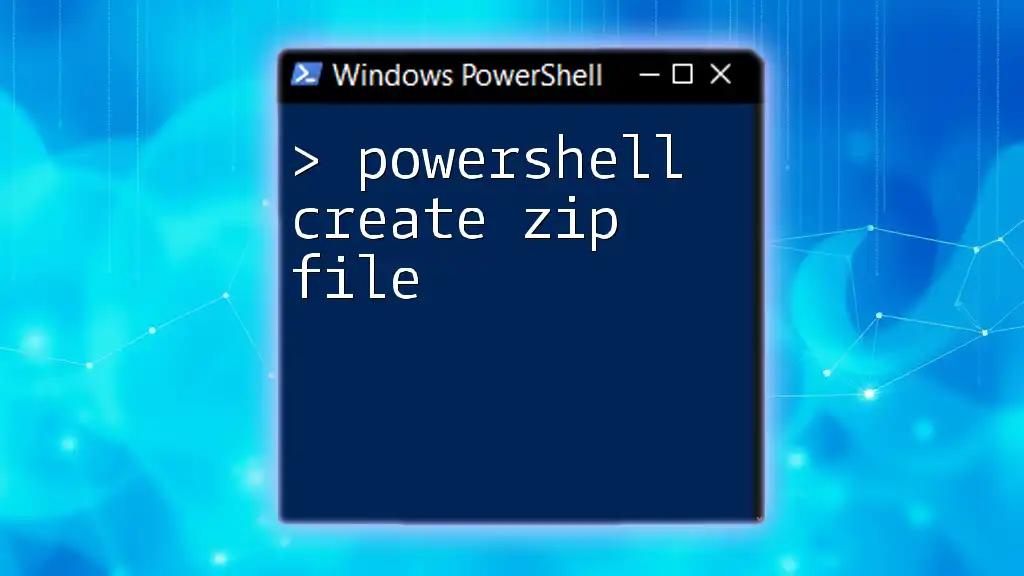
Creating an Excel File: Step-by-Step Guide
Basic Structure of a PowerShell Script
To create an Excel file, you'll need a basic script structure that initializes the workbook and adds data. Here's how to do that:
Creating a New Excel Workbook
You can create a new Excel file by specifying a path and initializing an Excel package:
$excelFile = "C:\Path\To\Your\File.xlsx"
$excelPackage = New-ExcelPackage -Path $excelFile
This line creates a new Excel file at the specified path.
Adding a Worksheet
After initializing your Excel package, the next step is to add a worksheet. You can name it anything you prefer:
$worksheet = $excelPackage.Workbook.Worksheets.Add("Sheet1")
This command adds a new worksheet titled “Sheet1” to your workbook.
Writing Data to the Excel Worksheet
Populating Cells with Data
Now that your worksheet is ready, it's time to populate it with data. You can easily write data into specific cells using the `.Cells` property:
$worksheet.Cells["A1"].Value = "Hello, World!"
$worksheet.Cells["A2"].Value = "PowerShell is Powerful!"
This example fills cell A1 with "Hello, World!" and A2 with "PowerShell is Powerful!".
Formatting Cells
To enhance the look of your Excel file, you can apply formatting. For example, to make the first cell bold, use:
$worksheet.Cells["A1"].Style.Font.Bold = $true
You can also explore other formatting options such as changing background colors, font sizes, and borders.
Saving the Excel File
After populating your worksheet and applying any desired formatting, it's crucial to save your changes. Use:
$excelPackage.Save()
This command saves all the modifications made to your Excel file.
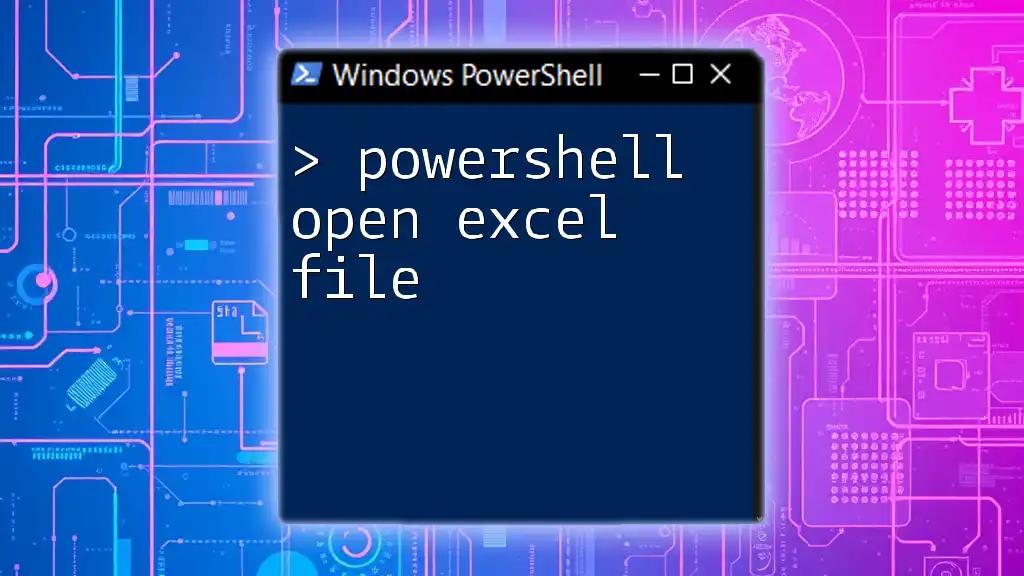
Advanced Excel Features with PowerShell
Adding Formulas to Excel Cells
Going beyond simple data entry, PowerShell can also insert formulas directly into cells, allowing for dynamic calculations. For instance, to input a SUM formula, use:
$worksheet.Cells["B1"].Formula = "SUM(A2:A10)"
This formula will automatically sum the values from cells A2 to A10.
Importing Data from CSV to Excel
PowerShell can be a powerful tool for transferring data from CSV files into Excel. Here’s how to do it:
Import-Csv "C:\Path\To\Your\Data.csv" | Export-Excel -Path $excelFile -WorksheetName "Data"
This command imports data from a CSV file and creates a new worksheet named "Data" in your Excel file.
Creating Charts from Data
Visualization is paramount in data analysis. With PowerShell, you can create charts programmatically. Here’s an example:
$chart = $worksheet.Drawings.AddChart("SalesChart", "ColumnClustered")
$chart.SetPosition(5, 0, 0, 0)
$chart.SetSize(600, 400)
This snippet adds a clustered column chart and sets its position and size on the worksheet.
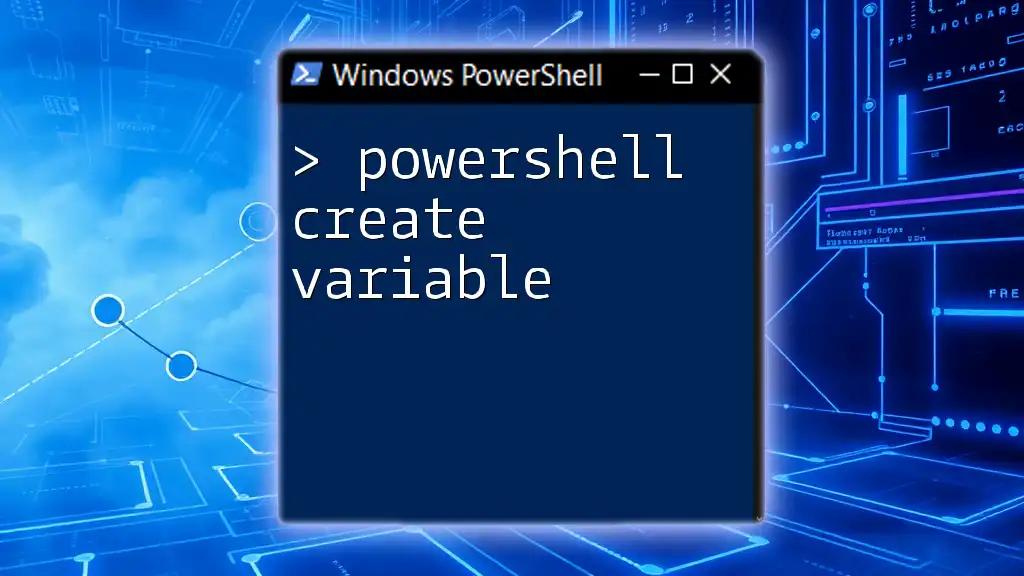
Troubleshooting Common Issues
Common Errors and Their Solutions
While working with PowerShell to create Excel files, you might encounter issues such as errors during data import or file path problems. It's essential to check the following:
- Ensure the file path is correct and accessible.
- Verify that your Excel installation is functioning properly.
Resources for Further Learning
For those interested in deepening their understanding of PowerShell and Excel, various resources are available:
- Microsoft Documentation: Comprehensive guides and examples.
- PowerShell Community Forums: Useful for troubleshooting and tips from other users.
- Online Courses: Look for platforms like Udemy or Pluralsight for structured learning opportunities.
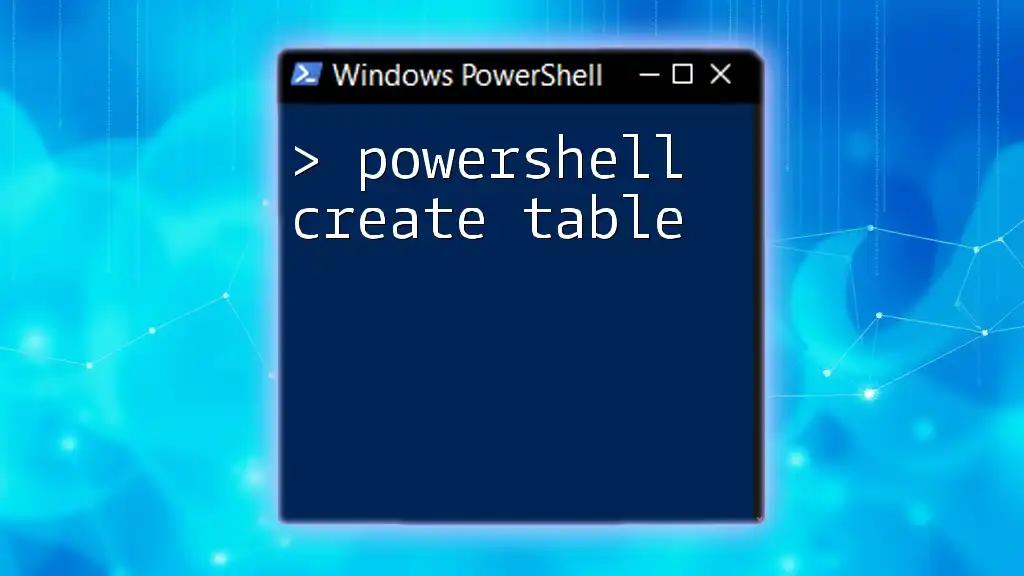
Conclusion
In summary, using PowerShell to create Excel files is a straightforward process that can greatly enhance productivity. By automating these tasks, users can focus on analysis rather than manual data entry. The combination of PowerShell's scripting capabilities and Excel's data manipulation features opens a world of automation possibilities.
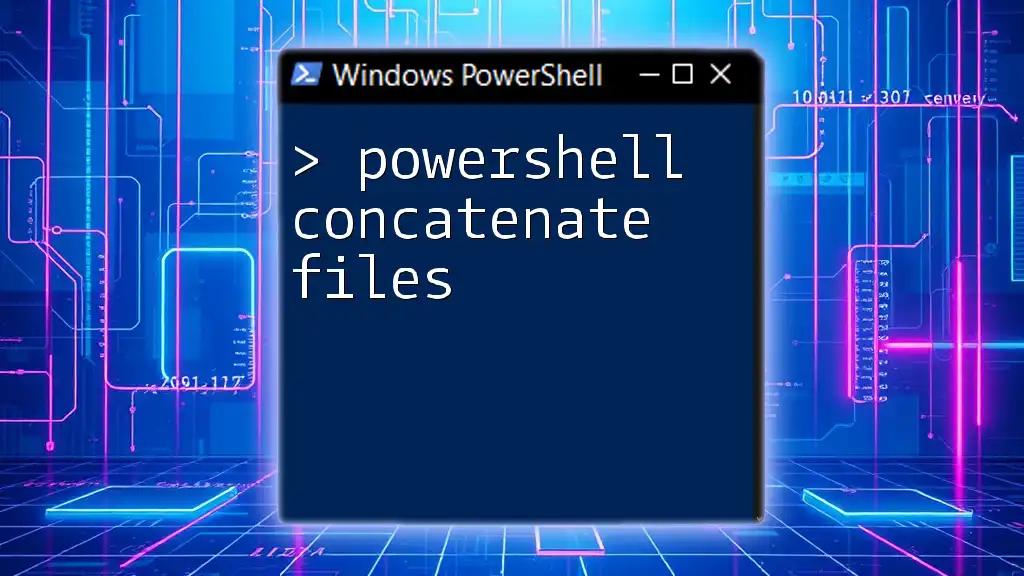
Call to Action
We encourage you to explore these techniques and share your experiences! If you have questions or tips, feel free to leave a comment. Subscribe to our newsletter for more PowerShell tips and tricks, and stay updated!
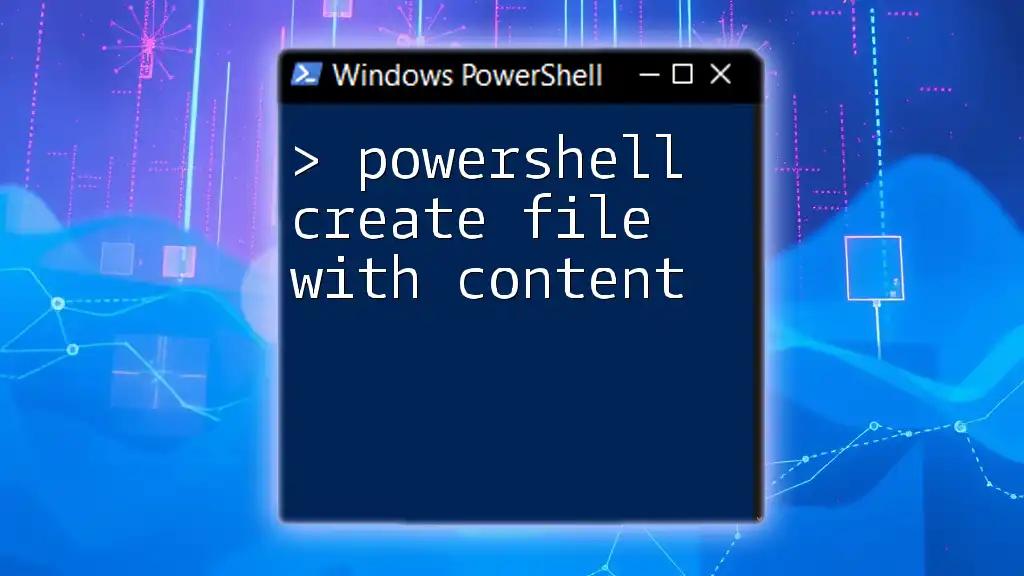
Frequently Asked Questions (FAQs)
Can PowerShell create Excel files without MS Excel installed?
PowerShell methods that leverage Excel require a local installation of Excel. However, alternatives like using CSV format can be used as a lightweight solution.
How do I automate running my PowerShell script?
You can schedule your PowerShell scripts using the Windows Task Scheduler, which allows scripts to run automatically at specified intervals or triggers.
Is it safe to enable PowerShell scripting?
While enabling scripting can offer powerful automation capabilities, it's essential to adhere to best practices, such as running scripts from trusted sources and maintaining updated security settings.