In PowerShell, the `New-Object` cmdlet is used to create an instance of a .NET object, allowing users to leverage the full power of the .NET framework within their scripts.
$newObject = New-Object -TypeName PSObject -Property @{ Name = "Example"; Value = 42 }
What is an Object in PowerShell?
In PowerShell, an object is a data structure that contains both data (properties) and actions (methods). Unlike basic data types—like integers and strings—objects offer more complexity and flexibility. They allow for rich data manipulation and are foundational to how PowerShell operates.
When working with PowerShell, understanding objects is crucial because most of the cmdlets you will encounter operate with objects as their primary data type. This forms the bedrock of PowerShell's programming paradigm, which is designed to manage environments and automate tasks efficiently.
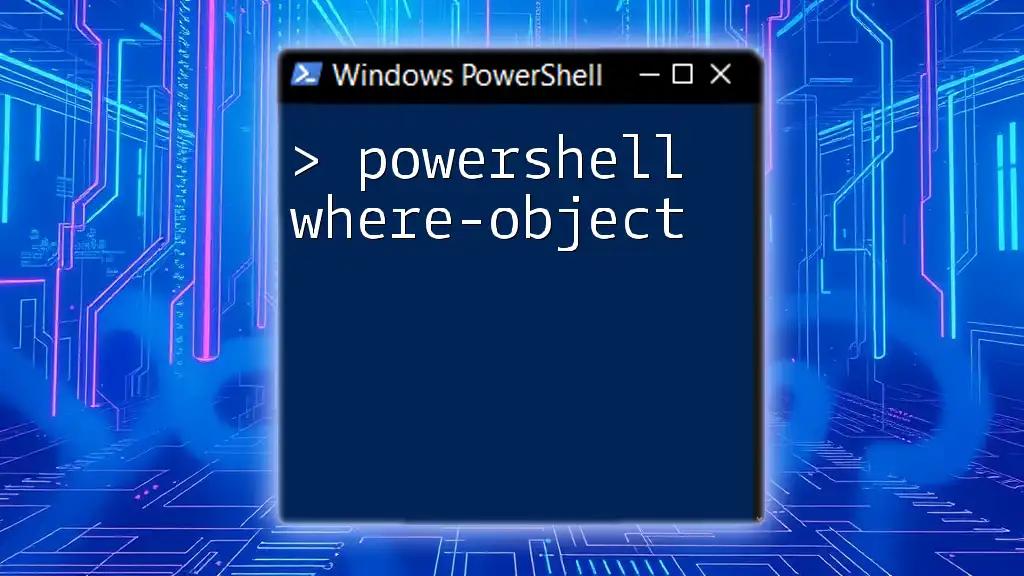
PowerShell Create Object: Understanding `New-Object`
What is `New-Object`?
The `New-Object` cmdlet is one of the main tools you can use to create new objects in PowerShell. It is versatile and can be utilized to instantiate both .NET objects and COM objects. This makes it a powerful tool for developers and system administrators alike.
Syntax of `New-Object`
The syntax for `New-Object` is fairly straightforward. It follows the structure:
New-Object -TypeName <TypeName> [-ArgumentList <ArgumentArray>]
Here, `-TypeName` specifies the type of the object to create, and `-ArgumentList` is optional and can be used to pass parameters to the constructor of the object being created.
Commonly Used Object Types
You can create various types of objects using `New-Object`, including:
- FileInfo: Represents a file in the filesystem.
- WebClient: Enables downloading data from the web.
For example, to create a FileInfo object, you would use:
$fileObj = New-Object System.IO.FileInfo "C:\path\to\file.txt"
This command creates a new instance of a FileInfo object, which can be used to work with the specified file programmatically.

PowerShell CreateObject vs New-Object
What is `CreateObject`?
`CreateObject` is a method primarily used for instantiating COM objects. Unlike `New-Object`, which is mainly for .NET objects, `CreateObject` enables interfacing with applications that expose their functionalities via Component Object Model.
Syntax of `CreateObject`
The typical syntax for using `CreateObject` is as follows:
$obj = New-Object -ComObject <ProgID>
In this case, `<ProgID>` is the Programmatic Identifier associated with the COM object you wish to create.
Commonly Used COM Objects
One common application of `CreateObject` is automating desktop applications such as Microsoft Office. For example, to create an Excel application instance, you can run:
$excelApp = New-Object -ComObject Excel.Application
This command initializes a new instance of Excel that you can then manipulate using PowerShell.

Creating Custom Objects in PowerShell
Using `New-Object` to Create Custom Objects
Creating custom objects is simple with `New-Object`. You can define properties for your object, which makes it easy to represent complex data structures. The following example illustrates how to create a custom object with several properties:
$customObj = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
Location = "USA"
}
This command creates a new object with the properties Name, Age, and Location, assigning values to each.
Using `PSCustomObject`
A more modern approach to creating custom objects is by using the `[PSCustomObject]` type. This method is lightweight and more efficient compared to `New-Object`. An example is:
$customPSObject = [PSCustomObject]@{
Name = "Jane Doe"
Occupation = "Developer"
}
Using `PSCustomObject` not only simplifies object creation but also offers improved performance.
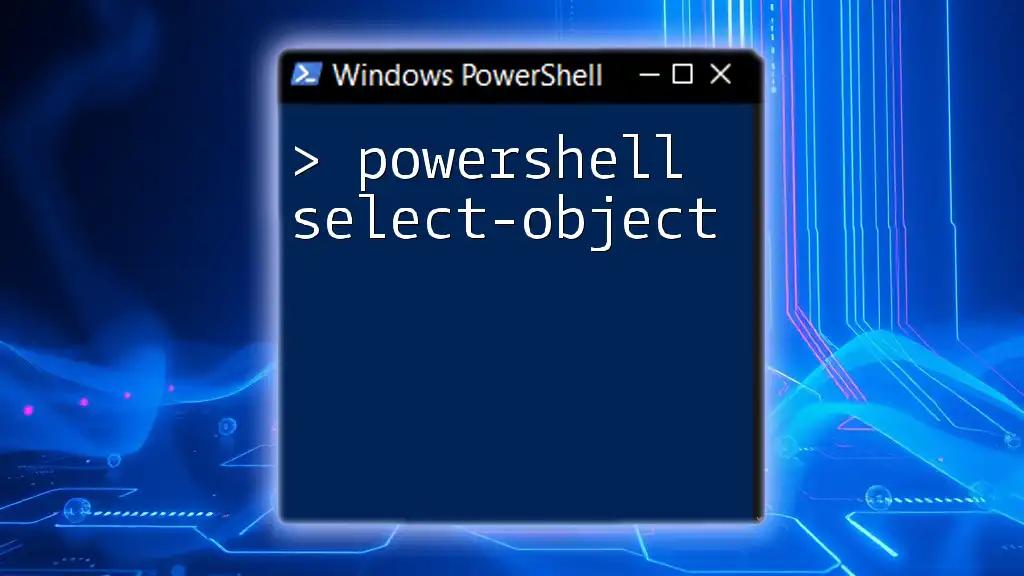
Practical Applications of Creating Objects
Automating Daily Tasks
One of the significant advantages of creating objects is the ability to automate repetitive administrative tasks. For example, you can create an array of custom user objects that represent employees, making it easier to manage employee information in scripts.
$employees = @()
$employees += [PSCustomObject]@{Name = "Alice"; Role = "Manager"}
$employees += [PSCustomObject]@{Name = "Bob"; Role = "Developer"}
This approach allows for quicker reference and maintenance of employees' information.
Data Storage and Retrieval
When working with data, created objects can serve as a convenient means of storage and manipulation. For instance, if you're developing a script to extract user data, you can store the information in custom objects for easier retrieval:
$userData = [PSCustomObject]@{
Username = "johndoe"
Email = "johndoe@example.com"
}
Such structures enable you to utilize the stored data in your scripts effectively.
Interacting with COM Objects
Creating COM objects allows for the automation of applications that expose functionalities via COM. For example, once you create an Excel application, you can manipulate its features, such as creating a new worksheet or writing data to cells through a script.
$excelApp.Workbooks.Add()
$excelApp.Cells.Item(1,1).Value = "Hello, Excel!"
$excelApp.Visible = $true
This snippet of code launches Excel, creates a new workbook, and writes "Hello, Excel!" into the first cell.
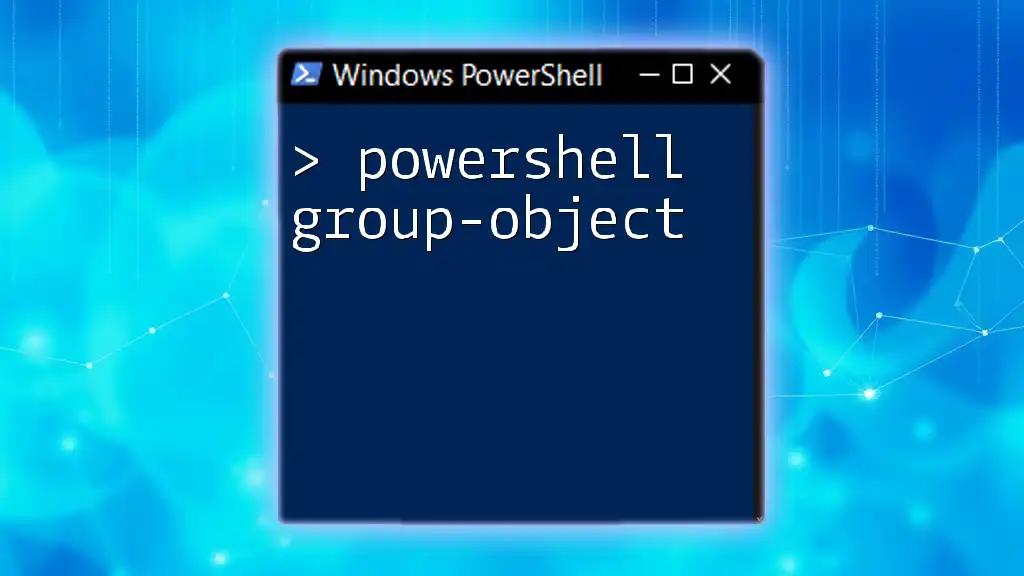
Best Practices for Creating Objects in PowerShell
Choosing When to Use `CreateObject` or `New-Object`
The decision to use `CreateObject` or `New-Object` largely depends on the type of object you need. Use `New-Object` for .NET objects and prefer `CreateObject` when dealing with COM components. Knowing the right method to use can greatly enhance your script's performance and reliability.
Structuring Custom Objects for Reusability
Design your custom objects for reusability by establishing clear properties and keeping object creation logic separate from core logic wherever possible. This modularity improves clarity and facilitates easier debugging and maintenance.
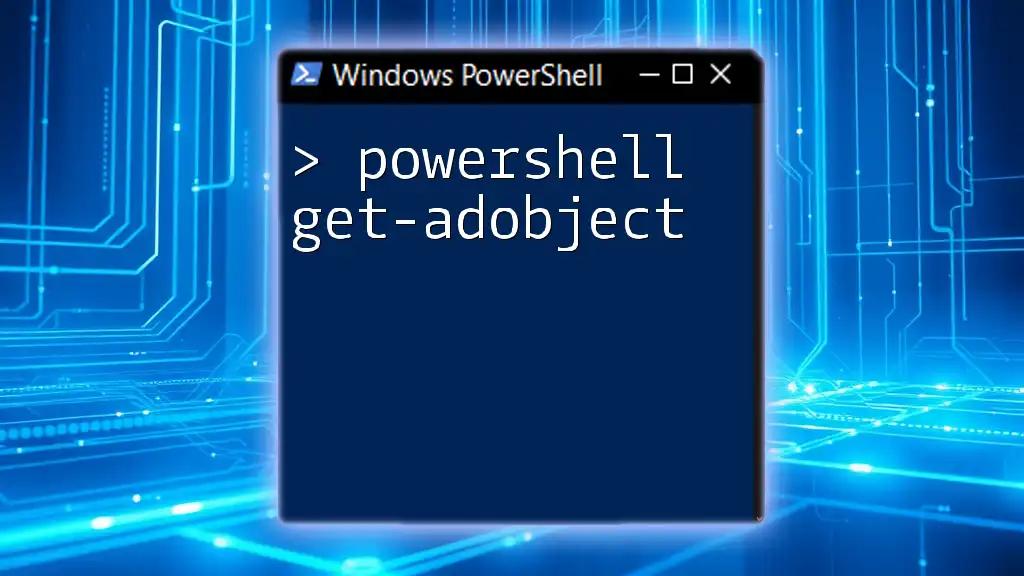
Troubleshooting Common Issues
Common Errors When Creating Objects
While creating objects, you might encounter errors such as "Type not found" or "COM object not registered." These messages typically indicate that you are either using an incorrect type name or trying to create an object that’s not properly registered on your system.
Debugging Object Creation
When facing issues with object creation, debugging techniques can help. Use the `Get-Member` cmdlet to inspect an object and ensure it has been created correctly:
$someObject | Get-Member
This command will display the properties and methods available for that object, helping you identify any problems quickly.

Conclusion
Creating objects in PowerShell is an essential skill for anyone looking to utilize the full potential of the platform. By mastering `New-Object` and `CreateObject`, along with understanding how to create custom and COM objects, you can significantly enhance your scripting capabilities.
Setting aside time to practice and explore these concepts will pay dividends as you develop your PowerShell skills. Start experimenting with creating objects today and unlock the powerful capabilities of PowerShell!

Additional Resources
For further reading and improved understanding, check out official PowerShell documentation, as well as tutorials dedicated to advanced object management and scripting techniques. These resources will provide a deeper insight into how to effectively create and manage objects within PowerShell, ultimately boosting your productivity and proficiency.