In PowerShell, unique objects are distinct items in a collection that can be retrieved using the `Select-Object` cmdlet with the `-Unique` parameter to filter out duplicates.
Here's a code snippet to demonstrate this:
Get-Process | Select-Object -Property Name -Unique
Understanding Objects in PowerShell
What Are Objects?
In PowerShell, objects are the fundamental building blocks of all operations. An object consists of data and the functionality to manipulate that data. Each object has properties (attributes) and methods (actions that can be performed). For instance, a file can be represented as an object with properties such as name, size, and creation date, and methods that allow you to open or delete the file.
To demonstrate object creation, you can easily create a simple object in PowerShell as follows:
$myObject = New-Object PSObject -Property @{
Name = "ExampleFile"
Size = 1024
}
How PowerShell Handles Objects
Understanding how PowerShell handles objects is essential, particularly regarding pass-by-reference and pass-by-value. When you pass an object to a function in PowerShell, you are actually passing a reference to the original object. Any changes made to that object within the function will affect the original object.
Here’s a small example to illustrate this:
Function Modify-Object {
param($obj)
$obj.Size = 2048
}
Modify-Object -obj $myObject
Write-Host "New Size: $($myObject.Size)" # Outputs 2048
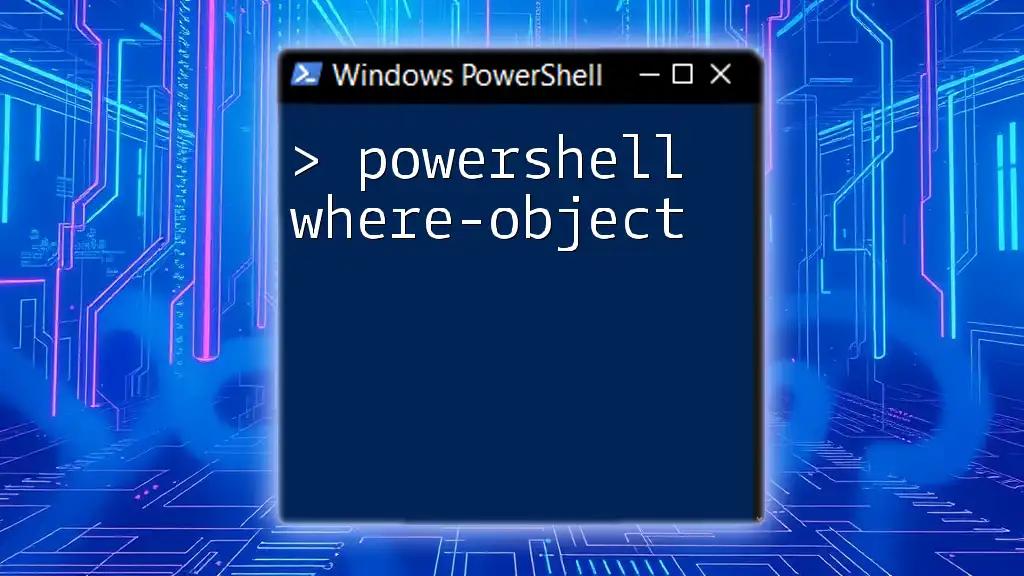
The Concept of Unique Objects
Definition of Unique Objects
Unique objects are those that possess distinct characteristics not shared with other objects in a collection. In PowerShell, uniqueness often refers to the properties of objects, where two objects are considered unique if their property values differ.
Why Unique Objects Matter
Understanding unique objects is crucial in various scenarios, from ensuring data integrity to optimizing scripts. For example, when querying a database or filtering results from a log file, you need to analyze unique entries to derive meaningful insights without redundancy.
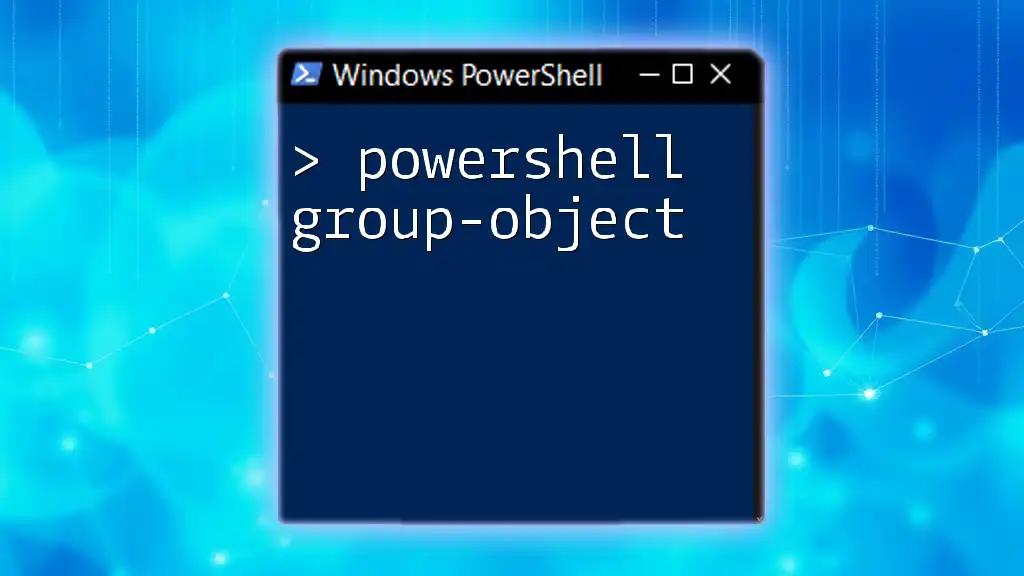
Creating Unique Objects
Basic Creation of Unique Objects
You can create unique objects manually using custom properties. By defining unique identifiers, you can guarantee that each object is distinctly recognized within your scripts.
$uniqueObject1 = New-Object PSObject -Property @{
ID = 1
Name = "John Doe"
}
$uniqueObject2 = New-Object PSObject -Property @{
ID = 2
Name = "Jane Smith"
}
Using Hash Tables to Create Unique Objects
Hash tables can help create collections of unique objects efficiently. You can leverage hash tables to store and retrieve unique items by their keys.
$uniqueObjects = @{}
$uniqueObjects["user1"] = New-Object PSObject -Property @{
ID = 1
Name = "Alice"
}
$uniqueObjects["user2"] = New-Object PSObject -Property @{
ID = 2
Name = "Bob"
}
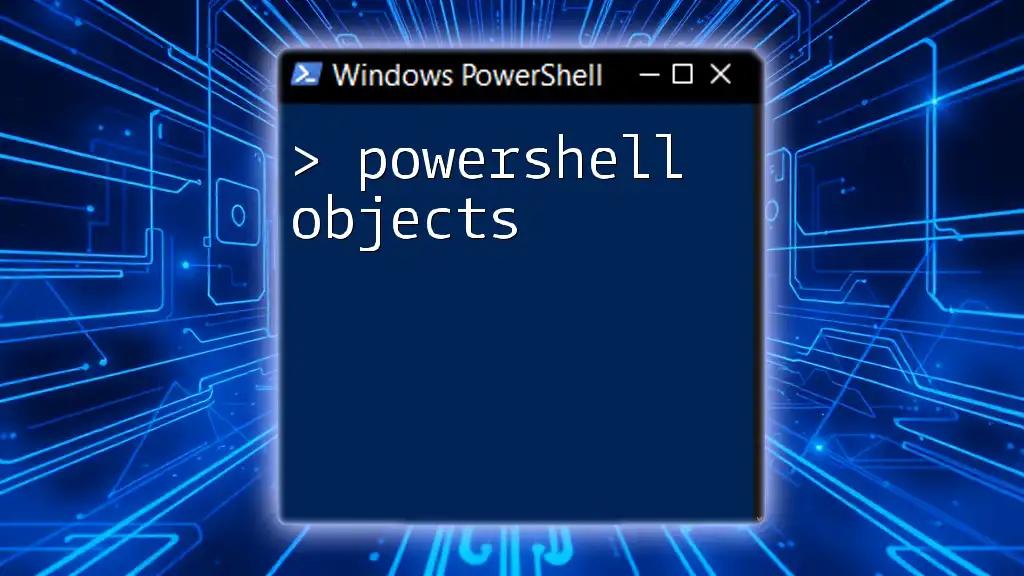
Identifying Unique Objects
Using `Get-Unique` Cmdlet
The `Get-Unique` cmdlet is useful for identifying unique values in a data stream. It compares the successive input objects and outputs only unique entries.
For example, consider a text file with duplicate lines:
Get-Content "C:\path\to\file.txt" | Get-Unique
Using `Select-Object -Unique`
The `Select-Object` cmdlet can also be employed to select unique items from a collection. This tool is especially beneficial when working with complex datasets.
Get-Content "C:\path\to\input.csv" | Select-Object -Unique

Comparing and Filtering Unique Objects
Comparing Objects for Uniqueness
To find unique entries between two sets of objects, you can use the `Compare-Object` cmdlet. This is particularly useful for comparing different lists or arrays.
$firstArray = @("Apple", "Banana", "Cherry")
$secondArray = @("Banana", "Cherry", "Dates")
Compare-Object -ReferenceObject $firstArray -DifferenceObject $secondArray
Filtering with `Where-Object`
The `Where-Object` cmdlet can filter collections to find unique instances based on specified criteria. This is handy when working with filtered data in complex scripts.
Get-Process | Where-Object { $_.Name -eq "powershell" }
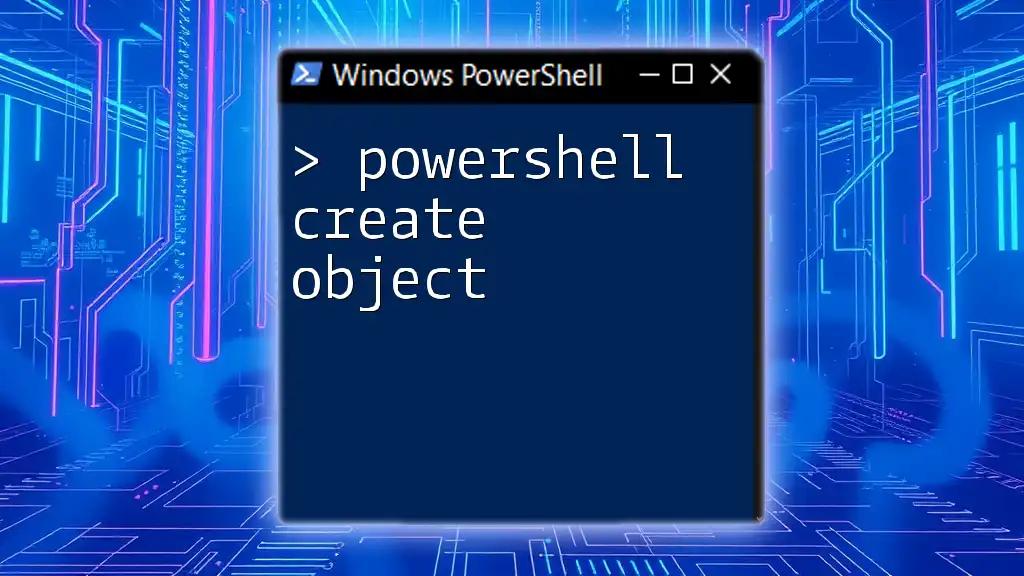
Manipulating Unique Objects
Sorting Unique Objects
Sorting collections of unique objects can make it easier to view and analyze data. You can use the `Sort-Object` cmdlet to achieve this.
$uniqueProcesses = Get-Process | Select-Object -Unique | Sort-Object Name
Exporting Unique Objects
PowerShell provides robust options for exporting unique objects to various formats like CSV or JSON. This allows for easy integration with other tools or data analysis platforms.
Get-Process | Select-Object -Unique | Export-Csv -Path "C:\path\to\unique_processes.csv" -NoTypeInformation

Common Pitfalls
Mistakes When Handling Unique Objects
When working with unique objects, it’s easy to misinterpret how PowerShell evaluates object uniqueness. Ensuring that you are comparing the correct properties and understanding the object structure is vital to avoid errors.
Performance Considerations
When filtering large datasets for unique items, performance issues can arise. Efficient scripting practices and utilizing native cmdlets designed for handling unique objects can help mitigate these concerns.

Real-World Applications of Unique Objects
Automation Scripts
In the world of automation, unique objects play a vital role. For instance, when managing user accounts in Active Directory, ensuring that you have unique user entries can prevent duplicate accounts and potential security issues.
Data Cleanup Tasks
Unique objects are applicable in data cleanup tasks, such as removing duplicate entries within databases or log files. Understanding how to identify and manipulate these objects can streamline administrative operations in IT environments.
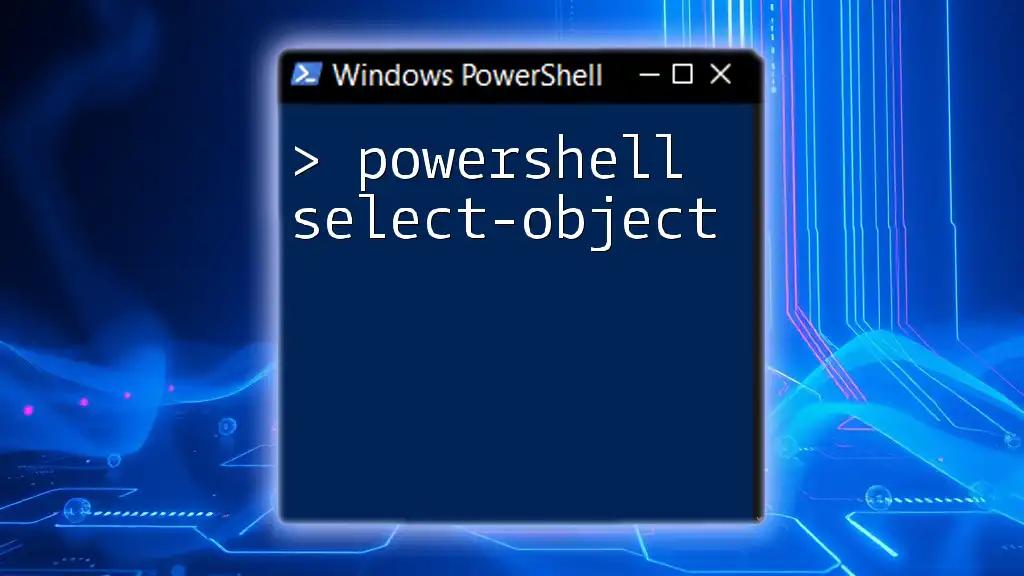
Conclusion
By mastering PowerShell unique objects, you can significantly enhance your scripting capabilities. Understanding how to create, identify, and manipulate unique instances will aid in efficient data handling, leading to more robust automation and management tasks. Embrace the possibilities that unique objects offer in PowerShell, and begin integrating these practices into your workflows today.
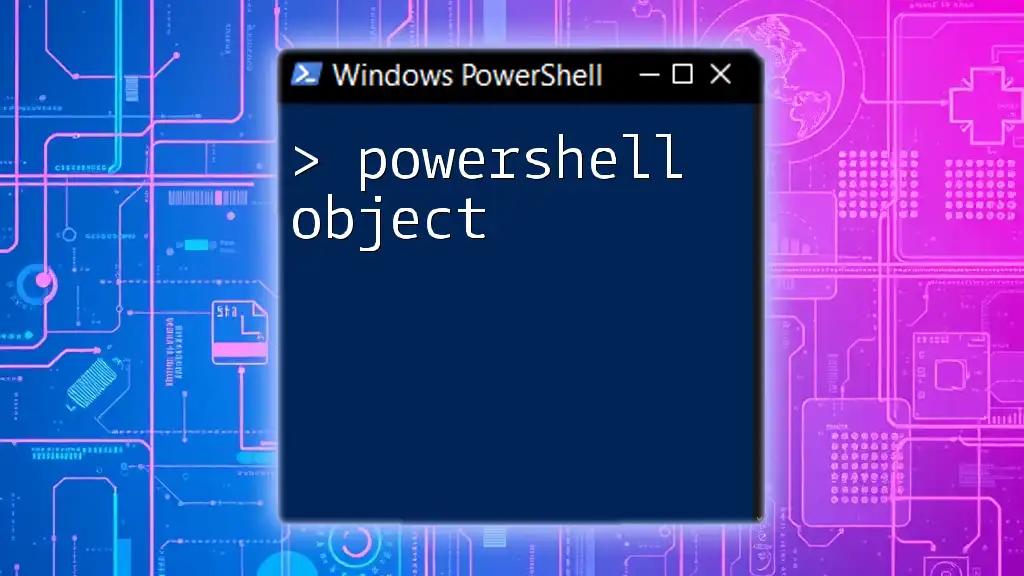
Additional Resources
For further learning, consult the official Microsoft PowerShell documentation, which offers extensive resources on cmdlets, object manipulation, and best practices. Additionally, consider exploring community blogs and forums where PowerShell enthusiasts share insights and scripts to enhance your skills and knowledge.