A PowerShell COM object allows you to interact programmatically with components from the Windows Component Object Model, enabling automation of applications like Excel or Word.
Here’s a simple code snippet to create a COM object for Excel and display a message box:
$excel = New-Object -ComObject Excel.Application
$excel.Visible = $true
[System.Windows.Forms.MessageBox]::Show("Excel has been opened!")
Understanding COM Objects
What is a COM Object?
A Component Object Model (COM) object is a technology that allows for software components to communicate with one another across different programming languages. As a foundational piece of Windows programming, COM provides a way to build reusable software components that can be invoked from various applications. It enables inter-process communication and dynamic object creation in a versatile manner.
Why Use COM Objects in PowerShell?
By leveraging PowerShell COM objects, you gain the ability to automate repetitive tasks and interact with other Windows applications like Microsoft Office products. This is particularly helpful for system administrators and developers who want to streamline their workflows. COM objects facilitate automation of various tasks, such as generating reports in Excel or managing files in Word.
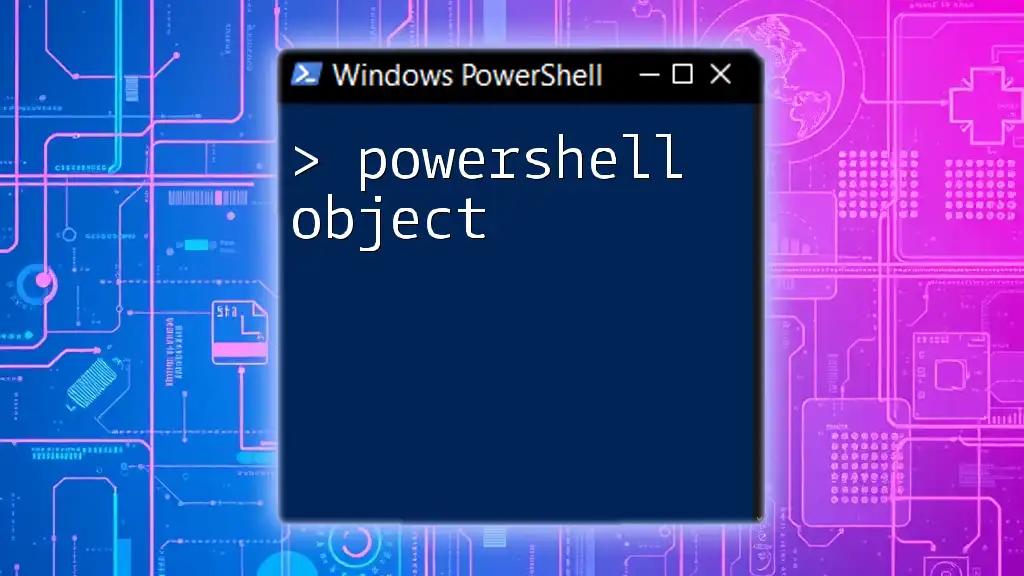
Accessing COM Objects in PowerShell
Creating a COM Object
To create an instance of a COM object within PowerShell, you can use the `New-Object` cmdlet. This cmdlet is the gateway to working with COM objects.
Example: Creating a Word Application COM Object
$word = New-Object -ComObject Word.Application
In this example, we create an instance of the Microsoft Word application, allowing us to interact with it programmatically.
Using Existing COM Objects
You can also use existing COM objects that are running on your system. This is useful for automation tasks that involve data or documents that are already open or present.
Example: Accessing Excel COM Object to Create a Workbook
$excel = New-Object -ComObject Excel.Application
$workbook = $excel.Workbooks.Add()
The above code snippet not only opens Excel but also creates a new workbook within it.

Commonly Used COM Objects
Microsoft Excel
The Excel COM Object is one of the most frequently used COM objects in PowerShell scripting due to its vast capabilities in data management.
Example: Automating Excel Tasks
$excel.Visible = $true
$sheet = $workbook.Worksheets.Item(1)
$sheet.Cells.Item(1, 1).Value2 = "Hello World"
This example makes Excel visible to the user and writes "Hello World" into the first cell of the first worksheet. Such automation can save significant time for data entry tasks.
Microsoft Word
The Word COM Object allows you to interact with Microsoft Word documents. You can automate the creation of reports, letters, and other documents seamlessly.
Example: Writing Text in a Word Document
$doc = $word.Documents.Add()
$selection = $word.Selection
$selection.TypeText("Hello from PowerShell")
In this code snippet, a new Word document is created, and we're typing "Hello from PowerShell" into it. The potential for document automation is immense with this capability.
Internet Explorer
You can automate web activities through the Internet Explorer COM Object, which can be particularly useful for tasks like automated testing and web scraping.
Example: Navigating to a Website
$ie = New-Object -ComObject InternetExplorer.Application
$ie.Visible = $true
$ie.Navigate("http://www.example.com")
In this example, Internet Explorer opens and navigates to the specified web page, which illustrates how easily we can automate browser functions.
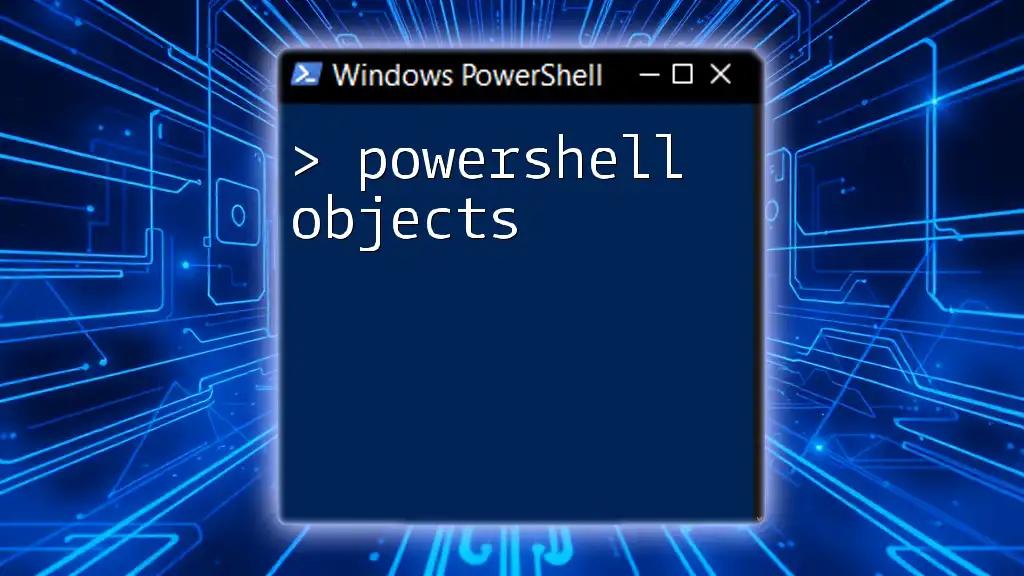
Working with COM Object Properties and Methods
Accessing Properties
Once you've created a COM object, you can manipulate its properties to configure it as needed.
Example: Changing Excel Application Properties
$excel.DisplayAlerts = $false
This code snippet turns off the display of alerts in Excel, allowing for smoother automation without interruptions from dialog windows.
Using Methods
Methods of COM objects can be called to perform actions within the program.
Example: Saving an Excel File
$workbook.SaveAs("C:\path\to\file.xlsx")
Here, we're using the `SaveAs` method to save the current workbook to a specified path. Understanding these methods is key to effective automation.

Error Handling with COM Objects
Common Errors
While working with COM objects, you may encounter errors relating to object access, invalid method invocations, or issues with file paths. Recognizing these common pitfalls can aid in smoother scripting.
Implementing Try/Catch Blocks
To enhance robustness in your PowerShell scripts, it's crucial to implement error handling using `try/catch` blocks.
Example: Safe Invocation of Methods
try {
$workbook.SaveAs("C:\invalid\path.xlsx")
} catch {
Write-Host "An error occurred: $_"
}
In this example, if an error occurs while saving the workbook (like an invalid path), the catch block will output the error message, allowing you to troubleshoot effectively.

Best Practices for Using COM Objects with PowerShell
Cleanup
To prevent memory leaks when working with COM objects, it's essential to properly clean up after usage. Always close the applications and release the COM objects after your tasks are complete.
Example: Properly Closing and Releasing COM Objects
$workbook.Close($false)
$excel.Quit()
[System.Runtime.Interopservices.Marshal]::ReleaseComObject($excel)
This snippet closes the workbook without saving changes, quits Excel, and releases the COM object, ensuring that system resources are freed.
Performance Considerations
When using COM objects, consider the performance implications. Opening multiple instances of applications or repeatedly creating new COM objects can slow down execution. Optimize your code to minimize resource usage and improve speed.
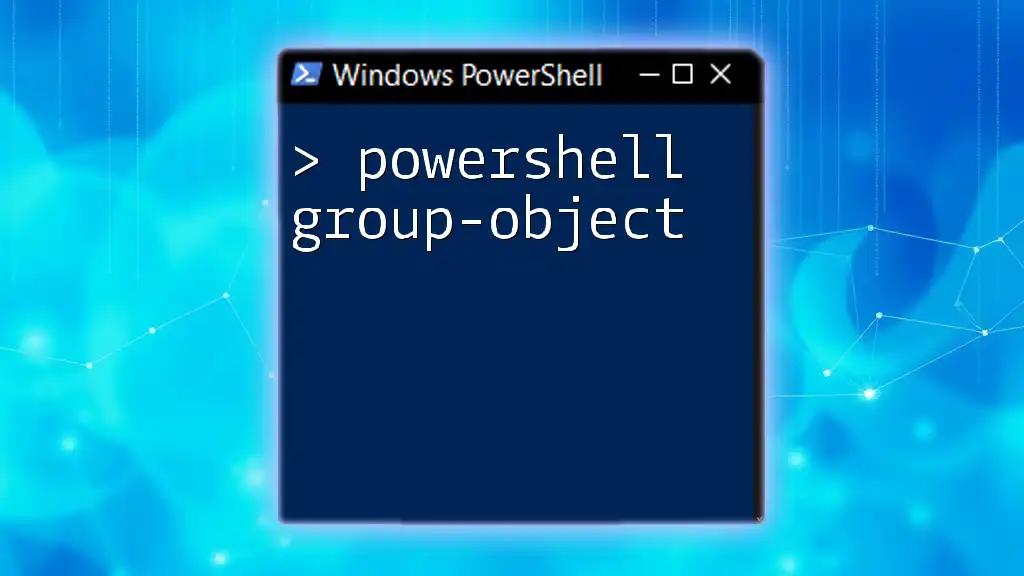
Use Cases and Real-World Applications
Automating Reports
Imagine automating a report generation process in Excel. Using PowerShell, you can pull data from various sources, format it, and produce a polished report without manual intervention. This can be especially beneficial in business environments where time is critical.
Desktop Automation
PowerShell can automate desktop tasks that involve document creation and management. For example, generating weekly status reports in Word can be scheduled to run automatically, saving time and improving efficiency.
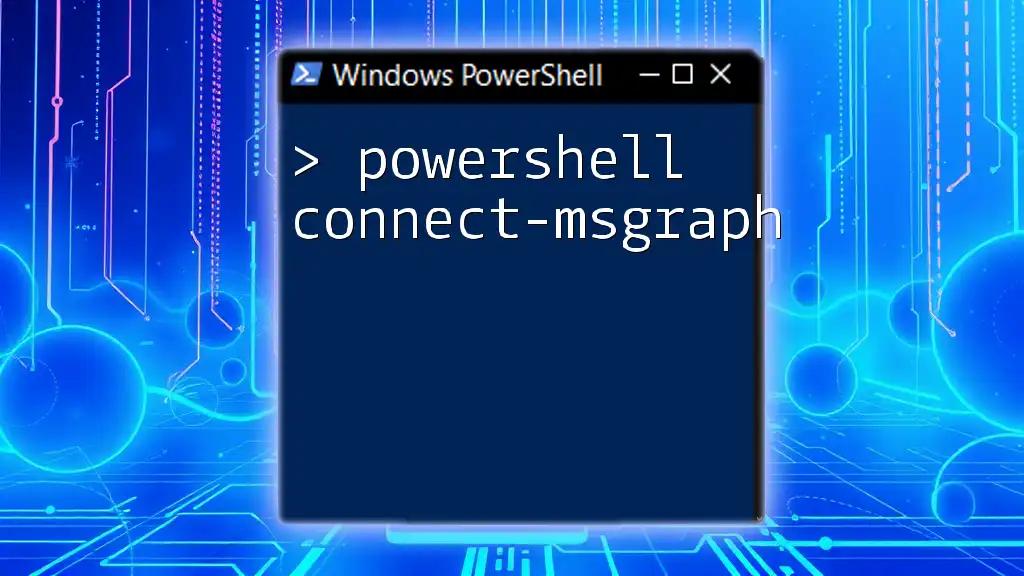
Conclusion
PowerShell COM Objects provide powerful capabilities for automation and scripting in Windows environments. Whether you're creating documents, managing spreadsheets, or navigating web pages, understanding how to work with COM objects can transform your workflow. By applying the techniques and best practices outlined in this guide, you’re now equipped to harness the power of PowerShell for your automation needs.
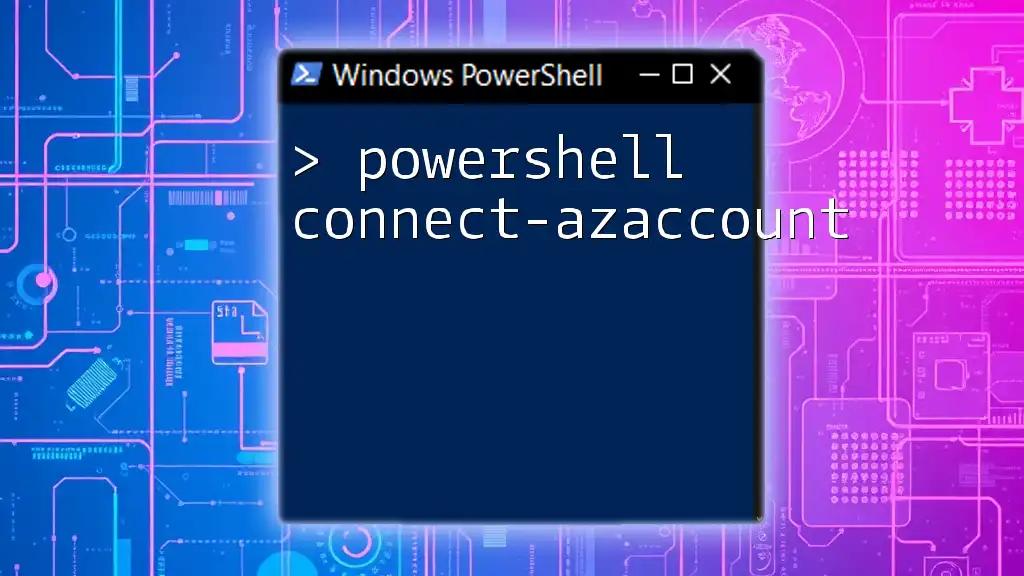
Additional Resources
For further exploration, consider checking out the official PowerShell documentation, which offers extensive resources and community support. Engage with forums dedicated to PowerShell to gain insights and assistance from fellow users.

FAQs
It's helpful to address frequently asked questions about PowerShell COM objects. Common queries might include troubleshooting common errors, understanding performance issues, and best practices for cleanup and resource management. Addressing these will enrich the learning experience for your readers.