A PowerShell custom object allows you to create a structured data representation with specific properties and methods, making your scripts more organized and easier to manage.
Here's a simple example of creating a custom object in PowerShell:
$person = New-Object PSObject -Property @{
Name = 'John Doe'
Age = 30
Occupation = 'Software Developer'
}
$person
Understanding PowerShell Custom Objects
What is a PowerShell Custom Object?
A PowerShell custom object is an object that you create specifically tailored to your needs, allowing you to define properties and methods that suit your requirements. Unlike standard PowerShell objects, which are predefined and rigid, custom objects provide flexibility and customization in your scripting.
Why Use Custom Objects?
Using custom objects enhances the capability of your scripts by allowing you to:
- Represent data in a more intuitive way, making your scripts easier to read and maintain.
- Organize information effectively, especially when dealing with complex datasets.
- Simplify the passing of data between different functions and scripts, improving code modularity.

Creating Custom Objects in PowerShell
Basic Syntax for Creating Custom Objects
Creating a custom object in PowerShell is straightforward. You can use the `New-Object` cmdlet or `[PSCustomObject]` to define your object. Here's an essential example:
$customObject = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
Occupation = "Engineer"
}
This creates a custom object with three properties: Name, Age, and Occupation. Each property has a defined value, forming the foundation of your custom structure.
Creating Custom Objects with Properties
Another convenient way to create a custom object is by utilizing the `[PSCustomObject]` syntax directly, which may be more efficient for rapid object creation. Here’s how you can do it:
$customObject = [PSCustomObject]@{
Name = "Jane Smith"
Age = 28
Occupation = "Designer"
}
This method not only creates the object but also assigns its properties in a concise format.
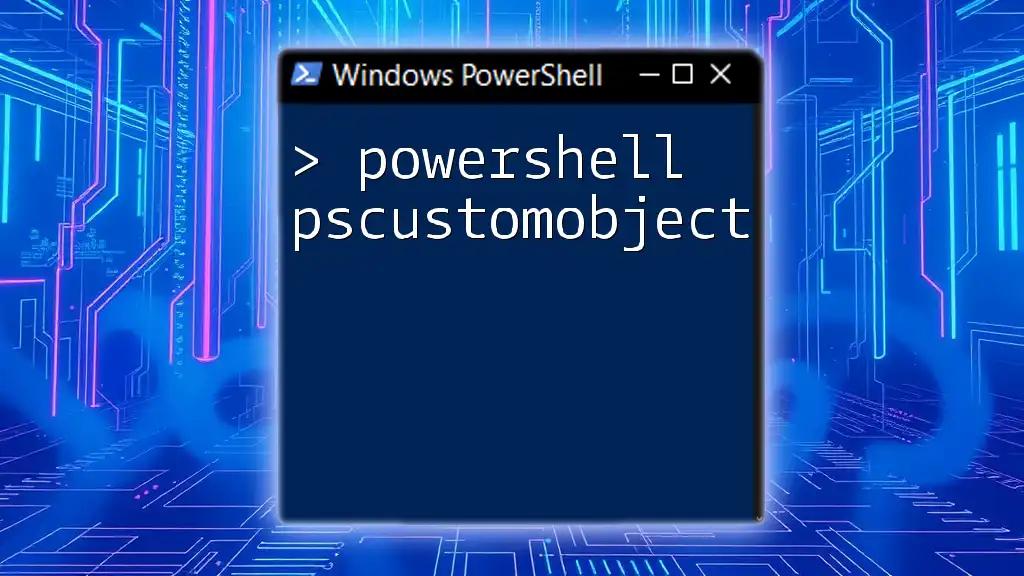
Adding Properties to Custom Objects
Using Add-Member Cmdlet
You can enhance your custom objects by adding additional properties after creation using the `Add-Member` cmdlet. This is particularly useful if you decide to enrich your object during runtime. Here’s an example:
$customObject | Add-Member -MemberType NoteProperty -Name "Salary" -Value 60000
This code snippet adds a new property called Salary to the existing custom object.
Dynamic Property Management
You can add properties conditionally based on certain criteria. This can be useful when you’re producing objects based on dynamic data sources. For instance:
if (-not $customObject.PSObject.Properties['Experience']) {
$customObject | Add-Member -MemberType NoteProperty -Name "Experience" -Value 5
}
In this example, if the Experience property doesn’t exist, the script adds it with a value of `5`.

Accessing and Modifying Custom Object Properties
Retrieving Property Values
Accessing and displaying property values in a custom object is straightforward. You can use the dot notation. Here’s how:
Write-Host "Name: $($customObject.Name), Age: $($customObject.Age)"
This command retrieves the Name and Age properties and prints them to the console.
Modifying Existing Properties
Modifying properties in your custom object can be done using the same dot notation:
$customObject.Age = 31
This command updates the Age property to `31`, demonstrating how easily you can manage your objects.

Working with Arrays of Custom Objects
Creating an Array of Custom Objects
When dealing with multiple custom objects, you can store them in an array. Here’s how you can do this:
$people = @()
$person1 = [PSCustomObject]@{ Name = "Alice"; Age = 25 }
$person2 = [PSCustomObject]@{ Name = "Bob"; Age = 35 }
$people += $person1
$people += $person2
This code initializes an array called $people and adds two custom objects representing different individuals.
Looping Through Arrays of Custom Objects
Iterating over each element in the array is simple and effective. You can easily loop through and access properties:
foreach ($person in $people) {
Write-Host "Name: $($person.Name), Age: $($person.Age)"
}
This loop prints the Name and Age for each person in the $people array, demonstrating how you can dynamically work with collections of custom objects.

Exporting Custom Objects
Exporting to CSV
For reporting and analysis, exporting custom objects to CSV format is a common task. Here’s how to do it:
$people | Export-Csv -Path "people.csv" -NoTypeInformation
This command will write the $people array into a CSV file named people.csv, making it easy to share and analyze in other applications.
Importing Custom Objects from CSV
You can also import your custom objects back into PowerShell from a CSV file. This is useful for restoring data. Here’s an example:
$importedPeople = Import-Csv -Path "people.csv"
This command reads the CSV file and converts it back into an array of custom objects, preserving the data structure.
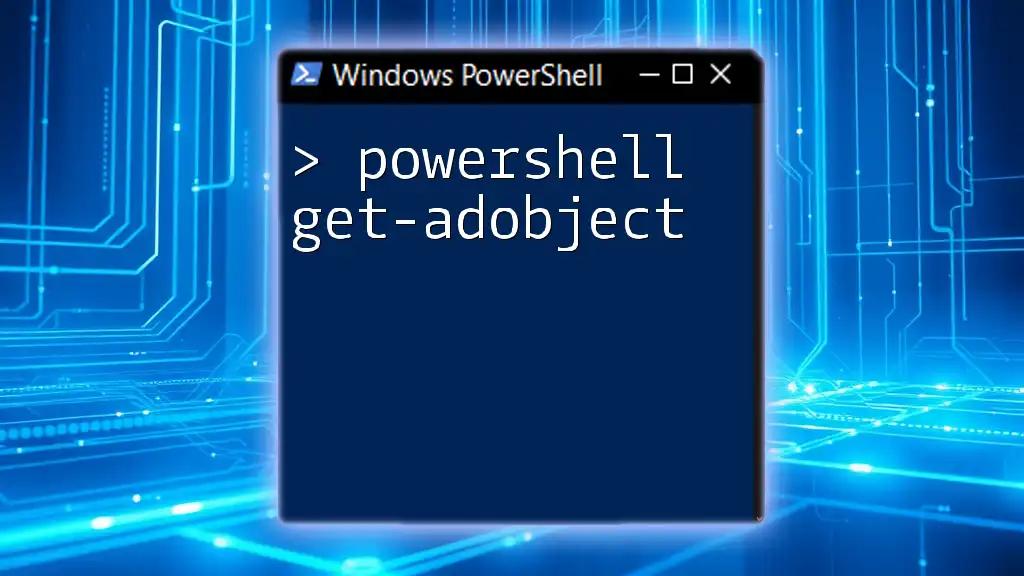
Best Practices for Using PowerShell Custom Objects
Naming Conventions and Structure
When creating custom objects, it is essential to use clear and descriptive names for properties. This clarity enhances code readability and maintainability. Ensure consistency in naming to facilitate understanding and collaboration among script developers.
Debugging Custom Objects
When working with complex custom objects, effective debugging techniques are crucial. Use commands like `Get-Member` to inspect the properties and methods of your objects, ensuring that they are behaving as expected.
Performance Considerations
While custom objects are highly versatile, it's important to recognize that they may not always be the fastest option in terms of performance. When dealing with large datasets, consider whether you need the full flexibility of a custom object or if a simpler data structure like a hashtable or an array would suffice.
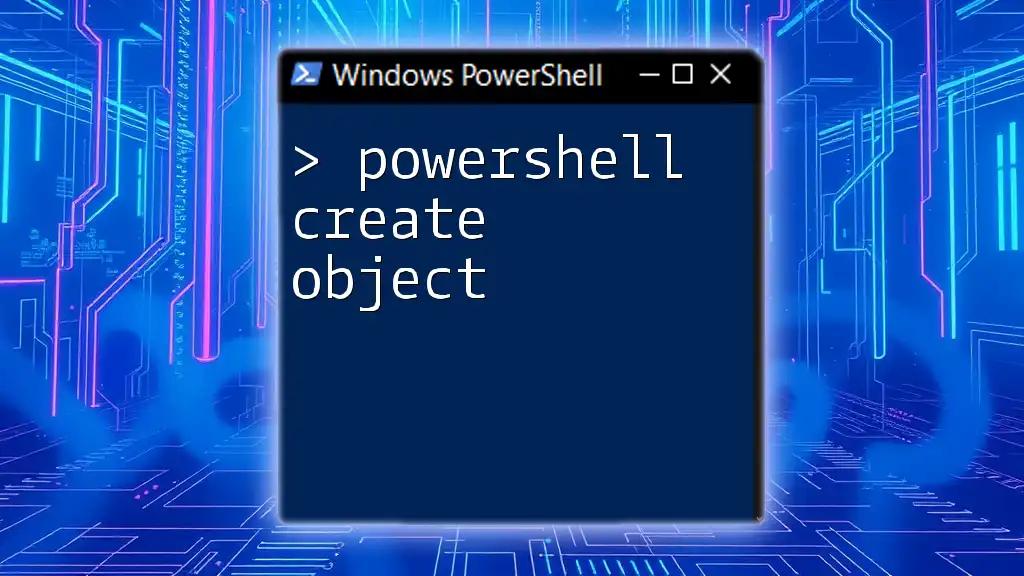
Conclusion
In summary, understanding and effectively using PowerShell custom objects can significantly enhance your scripting capabilities. They provide the flexibility to manage complex data, create organized structures, and interact intuitively with your datasets. As you become familiar with creating, modifying, and utilizing custom objects, you'll find that they are an invaluable tool in your PowerShell toolkit. Experiment with creating your custom objects and leveraging their full potential in your scripting endeavors.