In PowerShell, you can count the number of objects in an array using the `Count` property or the `Measure-Object` cmdlet.
Here’s a code snippet demonstrating both methods:
# Method 1: Using the Count property
$array = @(1, 2, 3, 4, 5)
$count1 = $array.Count
Write-Host "Count using Count property: $count1"
# Method 2: Using Measure-Object cmdlet
$count2 = $array | Measure-Object | Select-Object -ExpandProperty Count
Write-Host "Count using Measure-Object: $count2"
Understanding Arrays in PowerShell
What is an Array?
In PowerShell, an array is a collection of items stored in a single variable. Arrays are versatile data structures that can hold multiple values (strings, numbers, objects, etc.) of the same type or different types. They provide the capacity to manage and manipulate groups of data efficiently, making them a key feature of effective PowerShell scripting.
Creating Arrays in PowerShell
Creating an array in PowerShell is straightforward. You can use the `@()` syntax to instantiate an array:
$myArray = @("Apple", "Banana", "Cherry")
In the above example, `$myArray` is an array that contains three string elements. You can also create arrays by specifying the type:
[int[]]$numbers = @(1, 2, 3, 4, 5)
In this case, `$numbers` is declared as an integer array, ensuring that only integer values can be stored.
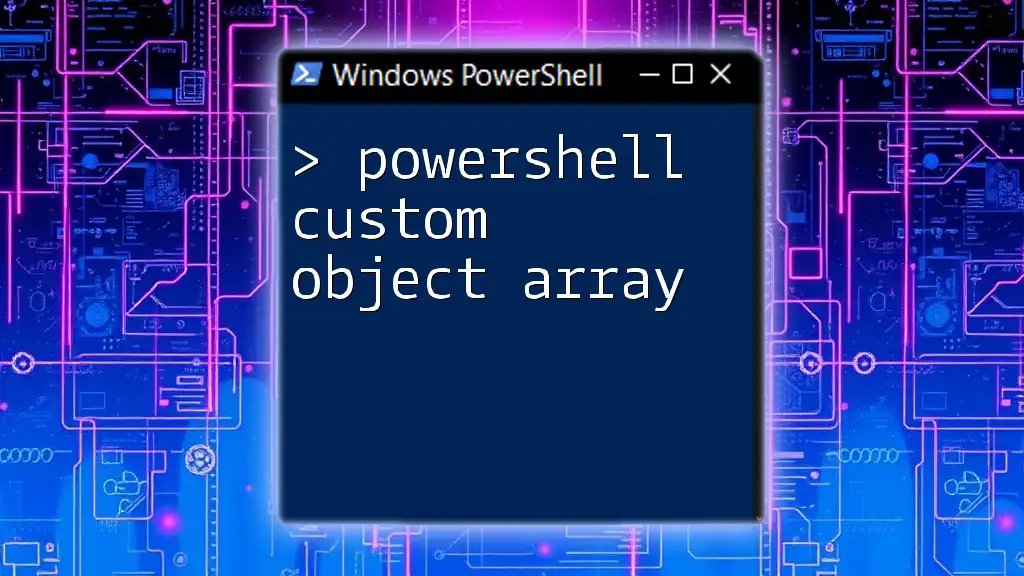
Counting Objects in an Array
The Importance of Counting Objects
Counting objects in an array is a critical skill in PowerShell scripting. It is especially useful for determining the size of an array, validating input data, debugging scripts, and analyzing performance metrics. Whether you're processing lists, handling configuration settings, or managing outputs from functions, knowing how to count objects in an array allows for better decision-making and enhances script functionality.
Using the `.Count` Property
One of the simplest methods to count elements in an array is by utilizing the `.Count` property. This property returns the total number of elements present in the array.
For example:
$myArray = @("Apple", "Banana", "Cherry")
$count = $myArray.Count
Write-Output "Number of items in array: $count"
In this scenario, `$count` will return `3`, as there are three items in the `$myArray`. It's important to note that `.Count` will always give you a valid count, even for empty arrays, which will return `0`.
Using the `Measure-Object` Cmdlet
Another powerful tool for counting objects in PowerShell is the `Measure-Object` cmdlet. This cmdlet is particularly handy when dealing with data piped from commands or when you want to count specific types of objects.
Here's an example of using `Measure-Object`:
$myArray = @("Apple", "Banana", "Cherry")
$result = $myArray | Measure-Object
Write-Output "Number of items in array: $($result.Count)"
Here, `$result.Count` retrieves the number of items in the `$myArray`, demonstrating how you can use piping to perform additional operations efficiently. While `.Count` is quick and direct, `Measure-Object` provides a more flexible approach, especially when working with filtered datasets.
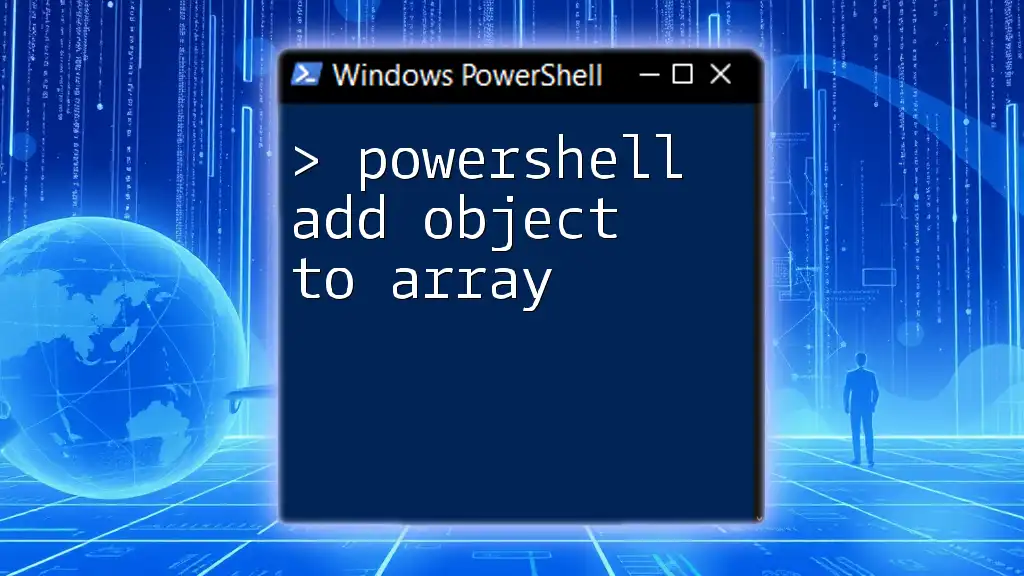
Practical Applications and Examples
Counting Strings in an Array
Consider a common scenario where you need to count specific strings within an array. For example, let's count how many "Apple" entries exist in the fruit array:
$fruits = @("Apple", "Banana", "Cherry", "Apple")
$appleCount = ($fruits | Where-Object { $_ -eq "Apple" }).Count
Write-Output "Number of Apples: $appleCount"
In this case, `$appleCount` will return `2`, as "Apple" appears twice in the `$fruits` array. This demonstrates the effectiveness of combining filtering and counting to derive meaningful insights from data.
Counting Numeric Values in an Array
Another practical example can involve counting numeric values in an array, particularly when duplicates are present. Let’s look at how to count unique numbers:
$numbers = @(1, 2, 2, 3, 4)
$distinctCount = ($numbers | Sort-Object -Unique).Count
Write-Output "Unique numbers count: $distinctCount"
Here, `Sort-Object -Unique` returns a new array of unique numbers from `$numbers`, and `.Count` gives us the number of distinct values (`4` in this case). This is a valuable technique for datasets where duplicates might skew analysis results.
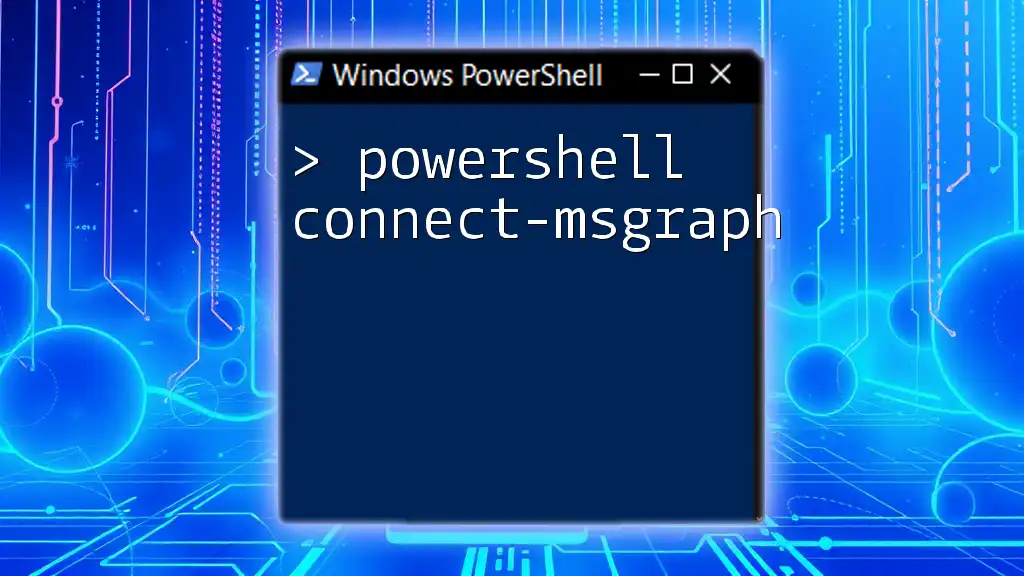
Handling Edge Cases
Counting Empty Arrays
When working with arrays in PowerShell, it’s important to recognize how they behave when empty. For example, an empty array will return a count of zero:
$emptyArray = @()
$emptyCount = $emptyArray.Count
Write-Output "Number of items in empty array: $emptyCount"
In this case, `$emptyCount` will yield `0`, making it clear that the array contains no elements. This behavior is predictable and should be considered to avoid errors in scripts.
Counting Objects in Multi-Dimensional Arrays
PowerShell supports multi-dimensional arrays, which can complicate counting. You can calculate the total number of items in a multi-dimensional array as follows:
$multiDimArray = @(@("Red", "Green"), @("Blue", "Yellow"))
$totalCount = $multiDimArray.Count * $multiDimArray[0].Count
Write-Output "Total items in multi-dimensional array: $totalCount"
This multiplication gives you the complete item count (`4` in this case). Understanding how to manage and count elements in multi-dimensional arrays is crucial when handling complex data structures.
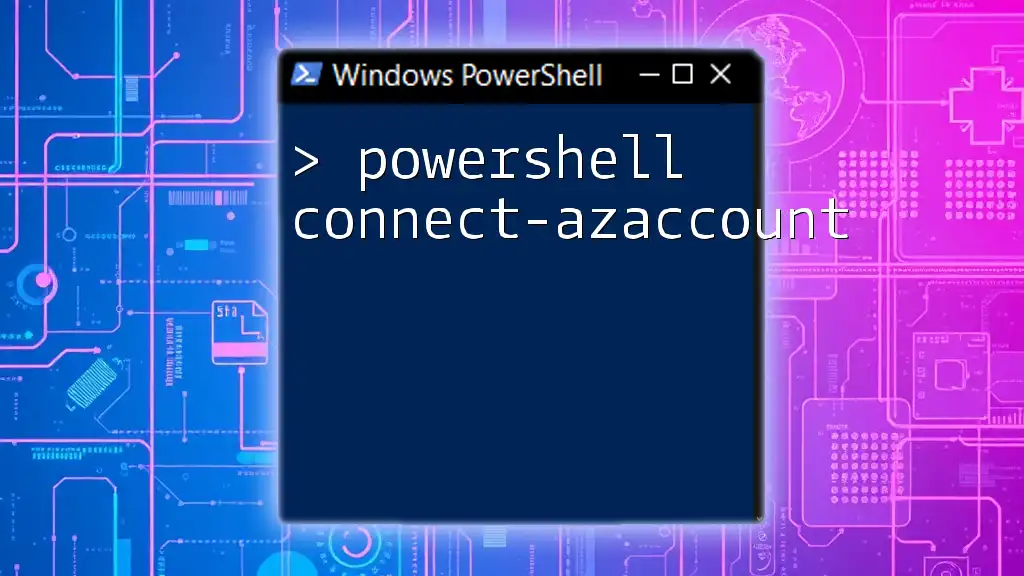
Best Practices for Counting Objects in Arrays
Efficient Counting Methods
While both `.Count` and `Measure-Object` are effective for counting objects, consider using `.Count` when you need a quick tally of items, especially in simple arrays. Use `Measure-Object` for more complex operations, such as when you might filter or group data.
Common Pitfalls to Avoid
One common mistake is neglecting to handle empty arrays or assuming the array always contains data. Always implement checks to confirm that arrays contain elements before attempting operations. For example:
if ($myArray.Count -gt 0) {
# Proceed with processing
} else {
Write-Output "Array is empty."
}
Following best practices will yield cleaner, more robust PowerShell scripts.
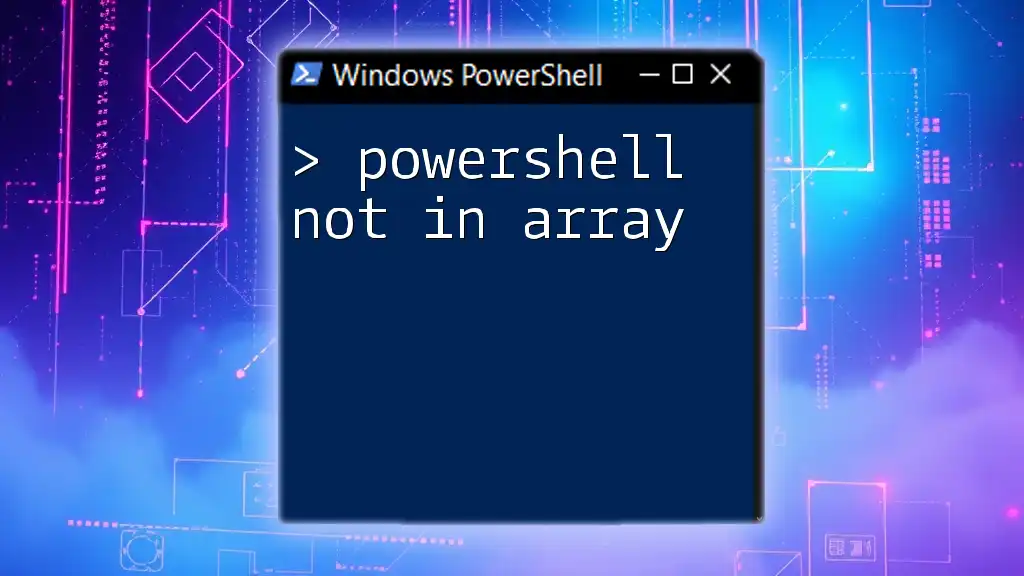
Conclusion
Understanding how to effectively count objects in an array in PowerShell is essential for any script writer. This guide covered the fundamental principles for working with arrays, utilizing properties like `.Count`, and leveraging cmdlets like `Measure-Object`. By mastering these techniques, you will enhance your PowerShell skills and ability to analyze and manipulate data efficiently. As you continue your learning journey, practice with diverse examples to become more proficient in leveraging the power of PowerShell arrays.
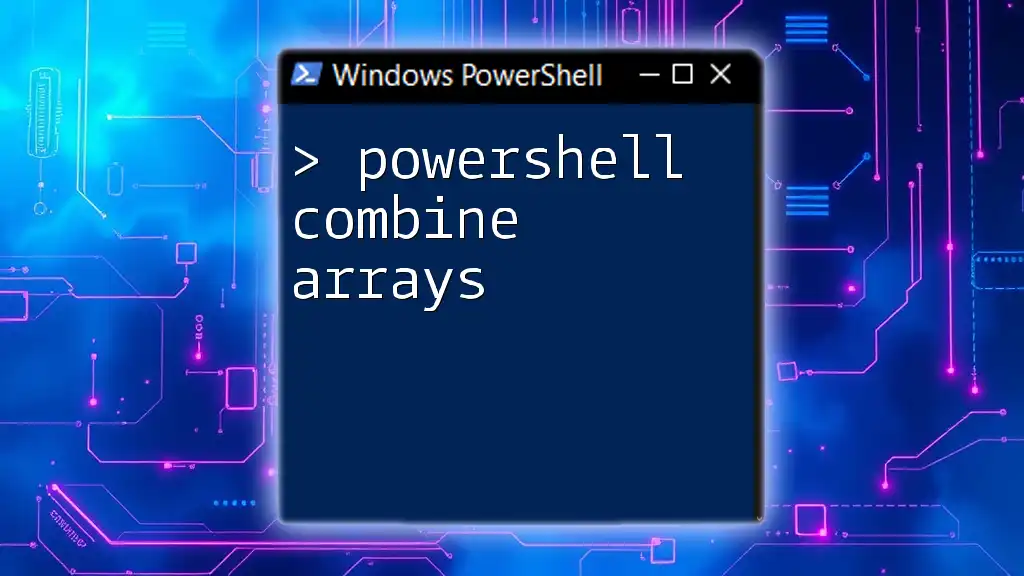
Additional Resources
For more information, refer to the PowerShell documentation on arrays and the `Measure-Object` cmdlet. As you dive deeper into scripting, consider exploring more advanced topics to enrich your PowerShell skills and capabilities.